Understanding Dependency Injection and Its Benefits in Software Development
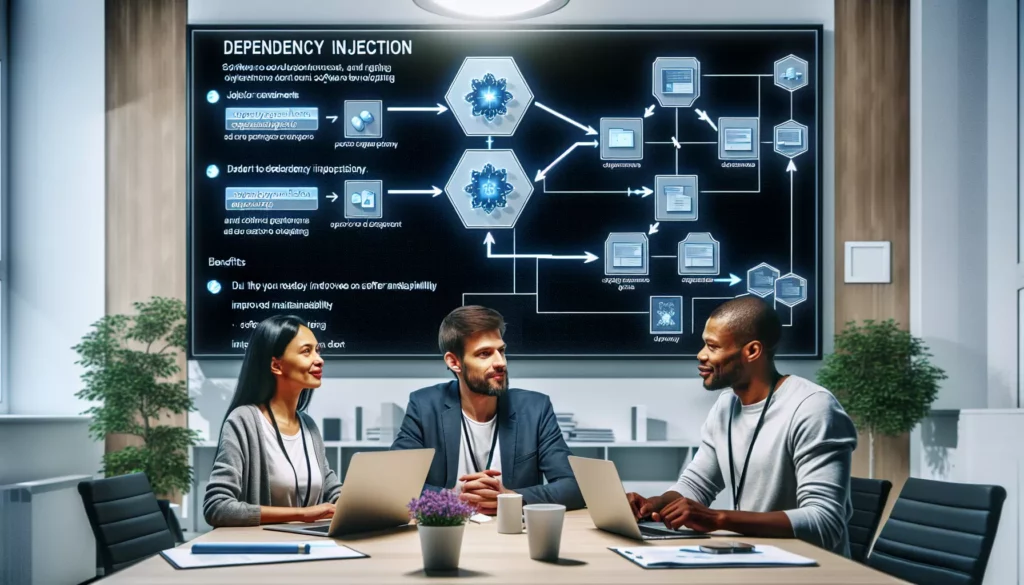
In the world of software development, creating clean, maintainable, and scalable code is paramount. One of the design patterns that significantly contributes to achieving these goals is Dependency Injection (DI). This powerful technique has become increasingly popular among developers, especially those working on large-scale applications. In this comprehensive guide, we’ll dive deep into the concept of Dependency Injection, explore its benefits, and learn how to implement it effectively in your projects.
What is Dependency Injection?
Dependency Injection is a design pattern that allows us to develop loosely coupled code by removing the responsibility of creating and managing dependencies from a class. Instead, these dependencies are “injected” into the class from the outside. This inversion of control is the core principle behind Dependency Injection.
To understand Dependency Injection better, let’s break it down:
- Dependency: An object that another object depends on to function correctly.
- Injection: The process of providing a dependency to an object that needs it.
In simpler terms, instead of a class creating its own dependencies, they are provided to the class when it’s instantiated or when they’re needed. This approach leads to more flexible, testable, and maintainable code.
The Problem Dependency Injection Solves
To appreciate the value of Dependency Injection, it’s essential to understand the problem it solves. Consider the following example without Dependency Injection:
public class UserService {
private DatabaseConnection dbConnection;
public UserService() {
this.dbConnection = new DatabaseConnection();
}
public User getUser(int userId) {
// Use dbConnection to fetch user
}
}
In this example, the UserService
class is tightly coupled with the DatabaseConnection
class. This coupling introduces several issues:
- Tight Coupling:
UserService
is directly responsible for creating itsDatabaseConnection
dependency, making it harder to change or replace the database implementation. - Reduced Testability: It’s challenging to unit test
UserService
in isolation because it always uses a real database connection. - Reduced Flexibility: If we want to use a different type of database or a mock database for testing, we’d need to modify the
UserService
class.
Dependency Injection addresses these issues by inverting the control of dependency creation and management.
Types of Dependency Injection
There are three main types of Dependency Injection:
1. Constructor Injection
In constructor injection, dependencies are provided through a class constructor. This is often considered the most robust form of Dependency Injection.
public class UserService {
private DatabaseConnection dbConnection;
public UserService(DatabaseConnection dbConnection) {
this.dbConnection = dbConnection;
}
// Rest of the class implementation
}
2. Setter Injection
Setter injection involves using setter methods to inject dependencies.
public class UserService {
private DatabaseConnection dbConnection;
public void setDatabaseConnection(DatabaseConnection dbConnection) {
this.dbConnection = dbConnection;
}
// Rest of the class implementation
}
3. Interface Injection
Interface injection is less common but involves the dependency providing an injector method that will inject the dependency into any client passed to it. Clients must implement an interface that exposes a setter method for receiving the dependency.
public interface DatabaseClient {
void setDatabaseConnection(DatabaseConnection connection);
}
public class UserService implements DatabaseClient {
private DatabaseConnection dbConnection;
@Override
public void setDatabaseConnection(DatabaseConnection connection) {
this.dbConnection = connection;
}
// Rest of the class implementation
}
Benefits of Dependency Injection
Now that we understand what Dependency Injection is and how it works, let’s explore its numerous benefits:
1. Loose Coupling
Dependency Injection promotes loose coupling between classes. When a class is not responsible for creating its dependencies, it becomes less dependent on the specific implementations of those dependencies. This makes the code more flexible and easier to maintain.
2. Improved Testability
With Dependency Injection, it becomes much easier to write unit tests. You can easily mock or stub dependencies, allowing you to test classes in isolation. This leads to more comprehensive and reliable test coverage.
3. Increased Modularity
By separating the concerns of object creation and object use, Dependency Injection naturally leads to more modular code. Each module or class has a clear, single responsibility, adhering to the Single Responsibility Principle of SOLID design principles.
4. Enhanced Code Reusability
When classes are not tightly coupled to their dependencies, they become more reusable. You can easily use the same class with different implementations of its dependencies without modifying the class itself.
5. Easier Maintenance
Loosely coupled code is generally easier to maintain. Changes in one part of the system are less likely to require changes in other parts, reducing the risk of introducing bugs when modifying or extending the codebase.
6. Flexibility in Development
Dependency Injection allows for more flexibility in development. You can develop against interfaces rather than concrete implementations, making it easier to swap out components or add new features without affecting existing code.
7. Better Separation of Concerns
By moving the responsibility of creating and managing dependencies out of the class that uses them, Dependency Injection promotes a better separation of concerns. This makes the code more organized and easier to understand.
Implementing Dependency Injection
While the concept of Dependency Injection is relatively simple, implementing it effectively requires some consideration. Here are some approaches to implementing Dependency Injection:
Manual Dependency Injection
The simplest form of Dependency Injection is manual injection, where you create and inject dependencies yourself. This is suitable for small projects or when you’re just starting with DI.
public class UserController {
private UserService userService;
public UserController() {
DatabaseConnection dbConnection = new DatabaseConnection();
this.userService = new UserService(dbConnection);
}
// Rest of the controller implementation
}
Dependency Injection Containers
For larger projects, using a Dependency Injection container (also known as an Inversion of Control container) can be beneficial. These containers manage the creation and lifetime of dependencies and inject them where needed. Popular DI containers include:
- Spring (for Java)
- Guice (for Java)
- Unity (for .NET)
- Ninject (for .NET)
Here’s a simple example using Spring:
@Configuration
public class AppConfig {
@Bean
public DatabaseConnection databaseConnection() {
return new DatabaseConnection();
}
@Bean
public UserService userService(DatabaseConnection dbConnection) {
return new UserService(dbConnection);
}
}
@Service
public class UserService {
private DatabaseConnection dbConnection;
@Autowired
public UserService(DatabaseConnection dbConnection) {
this.dbConnection = dbConnection;
}
// Rest of the service implementation
}
Best Practices for Dependency Injection
To get the most out of Dependency Injection, consider these best practices:
1. Prefer Constructor Injection
Constructor injection is generally considered the best form of Dependency Injection. It ensures that a class always has all its required dependencies and makes it clear what dependencies a class needs.
2. Use Interfaces
Depend on interfaces rather than concrete implementations. This makes it easier to swap out implementations and adhere to the Dependency Inversion Principle.
3. Keep Injection Simple
Avoid injecting too many dependencies into a single class. If a class requires many dependencies, it might be a sign that the class has too many responsibilities and should be split.
4. Use a DI Container for Complex Applications
For larger applications with many dependencies, consider using a Dependency Injection container to manage dependency creation and injection.
5. Avoid Service Locator Pattern
While the Service Locator pattern can seem similar to Dependency Injection, it’s generally considered an anti-pattern. It can make code harder to test and reason about.
Common Pitfalls and How to Avoid Them
While Dependency Injection offers many benefits, there are some common pitfalls to be aware of:
1. Overuse of DI
Pitfall: Trying to inject every single dependency, even when it’s not necessary.
Solution: Use DI for dependencies that might change or need to be mocked for testing. For simple, stable dependencies, direct instantiation might be fine.
2. Circular Dependencies
Pitfall: Creating a situation where Class A depends on Class B, and Class B depends on Class A.
Solution: Redesign your classes to avoid circular dependencies. Often, this indicates a design flaw that can be resolved by introducing a new abstraction.
3. Constructor Over-injection
Pitfall: Injecting too many dependencies through a constructor, making the class hard to instantiate and maintain.
Solution: If a class requires many dependencies, consider whether it has too many responsibilities and should be split into multiple classes.
4. Ignoring Lifecycle Management
Pitfall: Not properly managing the lifecycle of dependencies, leading to resource leaks or unexpected behavior.
Solution: Use a DI container that provides lifecycle management, or ensure you’re properly handling the creation and disposal of dependencies.
Dependency Injection in Different Programming Languages
While the principles of Dependency Injection remain the same across languages, the implementation details can vary. Let’s look at how DI is typically implemented in some popular programming languages:
Java
In Java, the Spring Framework is the most popular choice for Dependency Injection. It provides comprehensive support for DI through annotations and XML configuration.
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Service methods
}
C#
In the .NET ecosystem, built-in Dependency Injection support is available in ASP.NET Core, while third-party containers like Ninject are popular for other .NET applications.
public class UserController : Controller
{
private readonly IUserService _userService;
public UserController(IUserService userService)
{
_userService = userService;
}
// Controller actions
}
Python
Python doesn’t have built-in DI support, but libraries like Dependency Injector provide DI capabilities:
from dependency_injector import containers, providers
class Container(containers.DeclarativeContainer):
config = providers.Configuration()
db = providers.Singleton(Database, config.db_url)
user_service = providers.Factory(UserService, db=db)
# Usage
container = Container()
user_service = container.user_service()
JavaScript
In JavaScript, especially in Node.js applications, DI can be implemented manually or using libraries like InversifyJS:
import { injectable, inject } from "inversify";
@injectable()
class UserService {
constructor(@inject(TYPES.UserRepository) private userRepository: UserRepository) {}
// Service methods
}
Dependency Injection and Microservices
Dependency Injection plays a crucial role in microservices architecture. In a microservices environment, where services need to be independently deployable and scalable, DI helps in:
- Decoupling Services: DI allows services to depend on abstractions rather than concrete implementations, making it easier to evolve and replace services independently.
- Configuration Management: DI containers can help manage different configurations for different environments (development, staging, production).
- Testing: DI makes it easier to mock dependencies, allowing for more comprehensive unit and integration testing of individual microservices.
- Service Discovery: In some implementations, DI can be used in conjunction with service discovery mechanisms to dynamically inject service endpoints.
Future Trends in Dependency Injection
As software development practices evolve, so does the application of Dependency Injection. Some emerging trends include:
- Compile-time DI: Frameworks like Dagger in Java are moving towards compile-time dependency injection, which can improve performance and catch errors earlier.
- Serverless and DI: As serverless architectures become more popular, new patterns for applying DI in serverless functions are emerging.
- AI-assisted DI: Future IDEs and coding assistants may provide AI-powered suggestions for optimal dependency injection patterns based on code analysis.
Conclusion
Dependency Injection is a powerful design pattern that promotes loose coupling, improves testability, and enhances the overall maintainability of software systems. By understanding and applying DI principles, developers can create more flexible, scalable, and robust applications.
As you continue your journey in software development, remember that Dependency Injection is not just a technique, but a mindset. It encourages you to think about the relationships between components in your system and how to design them for maximum flexibility and reusability.
Whether you’re working on a small project or a large-scale enterprise application, the principles of Dependency Injection can help you write better, more maintainable code. As with any design pattern, the key is to understand when and how to apply it effectively, always considering the specific needs and constraints of your project.
Keep exploring, practicing, and refining your understanding of Dependency Injection, and you’ll find it becomes an invaluable tool in your software development toolkit.