How to Implement Authentication and Authorization: A Comprehensive Guide
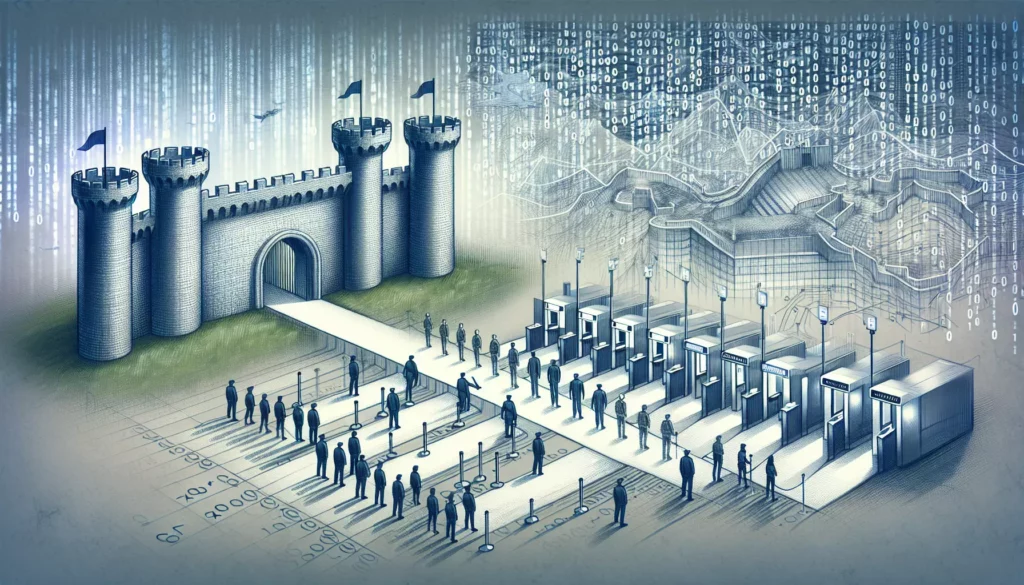
In the world of web development and application security, two crucial concepts stand out: authentication and authorization. These are the pillars that ensure only the right people can access the right resources at the right time. Whether you’re building a simple web application or a complex enterprise system, understanding and implementing these concepts correctly is essential for protecting sensitive data and maintaining user trust.
In this comprehensive guide, we’ll dive deep into the world of authentication and authorization, exploring what they are, why they’re important, and how to implement them effectively in your applications. We’ll cover various techniques, best practices, and even provide some code examples to help you get started.
Table of Contents
- What is Authentication?
- What is Authorization?
- Common Authentication Methods
- Authorization Techniques
- Implementing Authentication
- Implementing Authorization
- Best Practices for Authentication and Authorization
- Common Vulnerabilities and How to Avoid Them
- Conclusion
What is Authentication?
Authentication is the process of verifying the identity of a user, system, or entity. In simple terms, it’s about answering the question, “Are you who you say you are?” When you log into your email account or social media profile, you’re going through an authentication process.
The most common form of authentication is the username and password combination. However, as we’ll see later, there are many other methods of authentication, each with its own strengths and weaknesses.
What is Authorization?
Authorization, on the other hand, is the process of determining what an authenticated user is allowed to do. It answers the question, “Are you allowed to do this?” For example, in a content management system, a regular user might be authorized to create and edit their own posts, while an administrator might be authorized to edit and delete any post.
Authorization comes after authentication. Once a user’s identity is confirmed, the system then decides what resources or actions that user has permission to access or perform.
Common Authentication Methods
There are several methods of authentication, each with its own level of security and complexity. Here are some of the most common ones:
1. Password-based Authentication
This is the most basic and widely used form of authentication. Users provide a username (or email) and a secret password. While simple to implement, it’s also the most vulnerable to attacks if not implemented correctly.
2. Multi-factor Authentication (MFA)
MFA adds an extra layer of security by requiring two or more pieces of evidence (factors) to authenticate. These factors typically fall into three categories:
- Something you know (password, PIN)
- Something you have (smartphone, security token)
- Something you are (fingerprint, facial recognition)
3. Token-based Authentication
In this method, the server generates a token upon successful login, which the client then uses for subsequent requests. JSON Web Tokens (JWT) are a popular example of this approach.
4. Biometric Authentication
This method uses unique physical characteristics like fingerprints, facial features, or iris patterns to verify identity. While highly secure, it requires specialized hardware and software.
5. Single Sign-On (SSO)
SSO allows users to log in once and gain access to multiple applications without having to log in again. It’s commonly used in enterprise environments and with services like “Sign in with Google” or “Sign in with Facebook”.
Authorization Techniques
Once a user is authenticated, you need to determine what they’re allowed to do. Here are some common authorization techniques:
1. Role-Based Access Control (RBAC)
In RBAC, access rights are associated with roles, and users are assigned to appropriate roles. For example, you might have roles like “User”, “Editor”, and “Admin”, each with different permissions.
2. Attribute-Based Access Control (ABAC)
ABAC uses attributes associated with users, resources, and the environment to make access control decisions. This allows for more fine-grained and flexible access control policies.
3. Access Control Lists (ACL)
ACLs specify which users or system processes are granted access to objects, as well as what operations are allowed on given objects. They’re often used in file systems and networking.
4. Claims-based Authorization
In this approach, a user’s identity is represented by a set of claims. These claims are statements about the user that are trusted by both the identity provider and the application.
Implementing Authentication
Let’s look at how to implement a basic password-based authentication system using Node.js and Express. We’ll use bcrypt for password hashing and JSON Web Tokens (JWT) for session management.
Step 1: Set up your project
First, initialize a new Node.js project and install the necessary dependencies:
npm init -y
npm install express bcrypt jsonwebtoken
Step 2: Create the server and set up routes
Create a file named server.js
and add the following code:
const express = require('express');
const bcrypt = require('bcrypt');
const jwt = require('jsonwebtoken');
const app = express();
app.use(express.json());
const users = []; // This would be your database in a real application
const SECRET_KEY = 'your-secret-key'; // In a real app, use an environment variable
app.post('/register', async (req, res) => {
try {
const hashedPassword = await bcrypt.hash(req.body.password, 10);
const user = { username: req.body.username, password: hashedPassword };
users.push(user);
res.status(201).send('User registered successfully');
} catch {
res.status(500).send('Error registering user');
}
});
app.post('/login', async (req, res) => {
const user = users.find(user => user.username === req.body.username);
if (user == null) {
return res.status(400).send('Cannot find user');
}
try {
if (await bcrypt.compare(req.body.password, user.password)) {
const token = jwt.sign({ username: user.username }, SECRET_KEY);
res.json({ token: token });
} else {
res.send('Not Allowed');
}
} catch {
res.status(500).send('Error logging in');
}
});
app.listen(3000, () => console.log('Server started on port 3000'));
This code sets up two routes: /register
for creating new users, and /login
for authenticating users and providing a JWT.
Step 3: Implement a protected route
Now let’s add a protected route that requires authentication:
function authenticateToken(req, res, next) {
const authHeader = req.headers['authorization'];
const token = authHeader && authHeader.split(' ')[1];
if (token == null) return res.sendStatus(401);
jwt.verify(token, SECRET_KEY, (err, user) => {
if (err) return res.sendStatus(403);
req.user = user;
next();
});
}
app.get('/protected', authenticateToken, (req, res) => {
res.json({ message: 'This is a protected route', user: req.user });
});
This authenticateToken
middleware checks for a valid JWT in the Authorization header. If it’s valid, it adds the user information to the request object and allows the request to proceed.
Implementing Authorization
Now that we have authentication in place, let’s implement a simple role-based authorization system.
Step 1: Add roles to users
Modify the user registration to include a role:
app.post('/register', async (req, res) => {
try {
const hashedPassword = await bcrypt.hash(req.body.password, 10);
const user = {
username: req.body.username,
password: hashedPassword,
role: req.body.role || 'user' // Default to 'user' if no role is specified
};
users.push(user);
res.status(201).send('User registered successfully');
} catch {
res.status(500).send('Error registering user');
}
});
Step 2: Include role in JWT
Modify the login route to include the user’s role in the JWT:
app.post('/login', async (req, res) => {
const user = users.find(user => user.username === req.body.username);
if (user == null) {
return res.status(400).send('Cannot find user');
}
try {
if (await bcrypt.compare(req.body.password, user.password)) {
const token = jwt.sign({ username: user.username, role: user.role }, SECRET_KEY);
res.json({ token: token });
} else {
res.send('Not Allowed');
}
} catch {
res.status(500).send('Error logging in');
}
});
Step 3: Implement role-based authorization middleware
Create a middleware function to check user roles:
function authorize(roles = []) {
return (req, res, next) => {
if (!req.user) {
return res.sendStatus(401);
}
if (roles.length && !roles.includes(req.user.role)) {
return res.sendStatus(403);
}
next();
}
}
Step 4: Use the authorization middleware
Now you can use this middleware to protect routes based on roles:
app.get('/admin', authenticateToken, authorize(['admin']), (req, res) => {
res.json({ message: 'This is an admin route', user: req.user });
});
app.get('/user', authenticateToken, authorize(['user', 'admin']), (req, res) => {
res.json({ message: 'This is a user route', user: req.user });
});
In this example, only users with the ‘admin’ role can access the ‘/admin’ route, while both ‘user’ and ‘admin’ roles can access the ‘/user’ route.
Best Practices for Authentication and Authorization
- Use HTTPS: Always use HTTPS to encrypt data in transit, especially for login pages and API endpoints.
- Hash passwords: Never store passwords in plain text. Always use a strong, slow hashing algorithm like bcrypt.
- Implement MFA: When possible, offer multi-factor authentication to provide an extra layer of security.
- Use secure session management: Use techniques like JWT or secure session cookies to manage user sessions.
- Implement proper password policies: Enforce strong passwords and consider using a password strength meter.
- Use the principle of least privilege: Give users the minimum level of access they need to perform their tasks.
- Implement proper error handling: Don’t reveal too much information in error messages that could be used by attackers.
- Keep dependencies updated: Regularly update your libraries and frameworks to patch known vulnerabilities.
- Use security headers: Implement headers like Content Security Policy (CSP) to prevent attacks like XSS.
- Implement rate limiting: Prevent brute force attacks by limiting the number of requests from a single IP.
Common Vulnerabilities and How to Avoid Them
1. SQL Injection
SQL injection occurs when untrusted data is inserted into SQL queries. To prevent this:
- Use parameterized queries or prepared statements
- Validate and sanitize user input
- Use ORMs (Object-Relational Mapping) libraries
2. Cross-Site Scripting (XSS)
XSS allows attackers to inject client-side scripts into web pages. To prevent XSS:
- Sanitize and validate user input
- Use Content Security Policy (CSP) headers
- Encode output data
3. Cross-Site Request Forgery (CSRF)
CSRF tricks the victim into submitting a malicious request. To prevent CSRF:
- Use anti-CSRF tokens
- Implement SameSite cookie attribute
- Verify the origin header
4. Broken Authentication
This occurs when authentication mechanisms are implemented incorrectly. To prevent this:
- Use strong password policies
- Implement account lockout after failed attempts
- Use multi-factor authentication
5. Sensitive Data Exposure
This happens when sensitive data is not properly protected. To prevent this:
- Encrypt sensitive data at rest and in transit
- Use strong encryption algorithms and proper key management
- Don’t store sensitive data unnecessarily
Conclusion
Implementing robust authentication and authorization is crucial for the security of any application. By understanding the concepts, following best practices, and staying aware of common vulnerabilities, you can significantly enhance the security of your systems.
Remember, security is an ongoing process. Always stay updated with the latest security trends and regularly audit your systems for potential vulnerabilities. As you continue to develop your coding skills, make security a priority in your projects.
Whether you’re preparing for technical interviews or building your own applications, a solid understanding of authentication and authorization will serve you well. Keep practicing, stay curious, and always prioritize the security of your users and their data.