Introduction to Sockets and Real-Time Communication
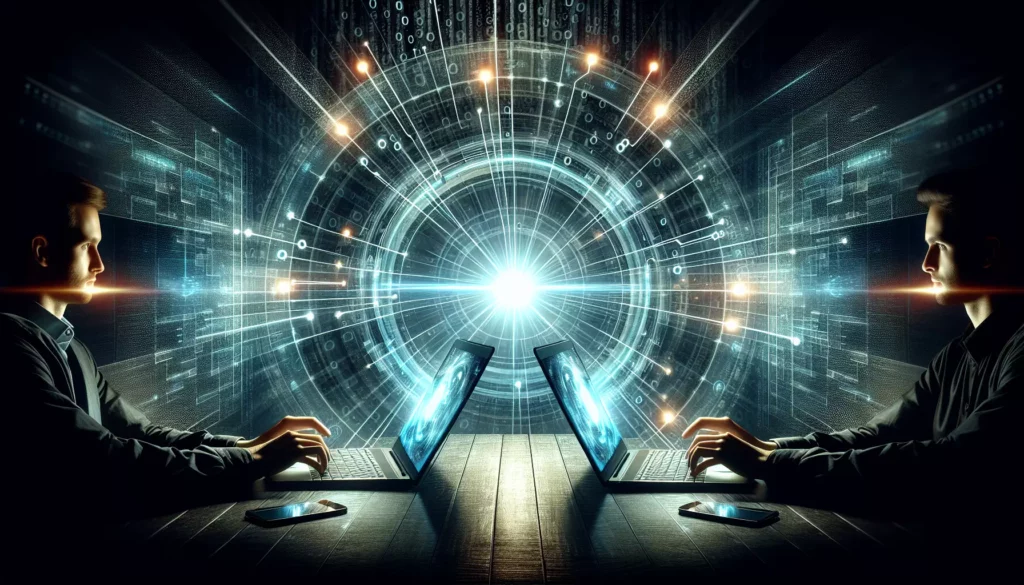
In the ever-evolving landscape of web development and networking, real-time communication has become an essential component of modern applications. From instant messaging platforms to live collaborative tools, the ability to exchange data instantly between clients and servers is crucial. At the heart of this real-time communication lies a powerful technology: sockets. In this comprehensive guide, we’ll dive deep into the world of sockets and explore how they enable real-time communication in various applications.
What are Sockets?
Sockets are the fundamental building blocks of network communication. They provide an endpoint for sending and receiving data across a computer network. In simpler terms, a socket is like a telephone that allows two programs to “talk” to each other, either on the same computer or across different machines connected via a network.
Sockets create a bidirectional communication channel between a client and a server, allowing data to flow freely between them. This bidirectional nature is what makes sockets particularly suitable for real-time applications, where instant updates and responses are crucial.
Types of Sockets
There are primarily two types of sockets used in network programming:
- Stream Sockets (TCP): These use the Transmission Control Protocol (TCP) and provide a reliable, ordered, and error-checked delivery of data between applications.
- Datagram Sockets (UDP): These use the User Datagram Protocol (UDP) and provide a connectionless point for sending and receiving data. While faster, they don’t guarantee delivery or order of data packets.
For most real-time applications, TCP sockets are preferred due to their reliability and built-in error handling mechanisms.
How Sockets Work
To understand how sockets facilitate communication, let’s break down the process:
- Socket Creation: Both the client and server create socket objects.
- Binding: The server binds its socket to a specific address and port.
- Listening: The server socket starts listening for incoming connections.
- Connection: The client initiates a connection to the server’s socket.
- Acceptance: The server accepts the connection, creating a new socket for this specific client.
- Data Exchange: Both sides can now send and receive data through the established connection.
- Closure: Either side can close the connection when communication is complete.
Implementing Sockets in Different Languages
Let’s look at how to implement basic socket communication in a few popular programming languages:
Python
Python provides a high-level socket module that makes it easy to work with sockets. Here’s a simple example of a server and client using TCP sockets:
Server:
import socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 12345))
server_socket.listen(1)
print("Server is listening...")
connection, address = server_socket.accept()
print(f"Connected by {address}")
while True:
data = connection.recv(1024)
if not data:
break
print(f"Received: {data.decode()}")
connection.sendall(data)
connection.close()
Client:
import socket
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(('localhost', 12345))
message = "Hello, server!"
client_socket.sendall(message.encode())
data = client_socket.recv(1024)
print(f"Received: {data.decode()}")
client_socket.close()
JavaScript (Node.js)
Node.js provides the ‘net’ module for working with TCP sockets. Here’s a simple example:
Server:
const net = require('net');
const server = net.createServer((socket) => {
console.log('Client connected');
socket.on('data', (data) => {
console.log(`Received: ${data}`);
socket.write(data);
});
socket.on('end', () => {
console.log('Client disconnected');
});
});
server.listen(12345, () => {
console.log('Server listening on port 12345');
});
Client:
const net = require('net');
const client = new net.Socket();
client.connect(12345, 'localhost', () => {
console.log('Connected to server');
client.write('Hello, server!');
});
client.on('data', (data) => {
console.log(`Received: ${data}`);
client.destroy();
});
client.on('close', () => {
console.log('Connection closed');
});
WebSockets: The Evolution of Real-Time Web Communication
While traditional sockets are powerful for general network programming, web browsers needed a specialized solution for real-time communication. This led to the development of WebSockets, a protocol that provides full-duplex communication channels over a single TCP connection.
WebSockets offer several advantages for web applications:
- Reduced latency compared to HTTP polling
- Full-duplex communication (both client and server can send messages at any time)
- Efficient for small messages, as there’s no need to send HTTP headers with each request
- Compatible with existing HTTP infrastructure (can use the same ports)
Implementing WebSockets
Let’s look at a simple WebSocket implementation using JavaScript on both the client and server side:
Server (Node.js with ‘ws’ library):
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
console.log('Client connected');
ws.on('message', (message) => {
console.log(`Received: ${message}`);
ws.send(`Echo: ${message}`);
});
ws.on('close', () => {
console.log('Client disconnected');
});
});
Client (Browser JavaScript):
const socket = new WebSocket('ws://localhost:8080');
socket.addEventListener('open', (event) => {
console.log('Connected to server');
socket.send('Hello, server!');
});
socket.addEventListener('message', (event) => {
console.log(`Received: ${event.data}`);
});
socket.addEventListener('close', (event) => {
console.log('Connection closed');
});
Real-World Applications of Sockets and WebSockets
The applications of sockets and WebSockets in modern software development are vast and varied. Here are some common use cases:
1. Chat Applications
Real-time chat applications heavily rely on WebSockets to provide instant messaging capabilities. When a user sends a message, it’s immediately pushed to all connected clients, creating a seamless chatting experience.
2. Multiplayer Games
Online multiplayer games use sockets to synchronize game states between players. This allows for real-time updates of player positions, actions, and game events.
3. Collaborative Tools
Applications like Google Docs use WebSockets to enable real-time collaboration. When one user makes a change, it’s instantly reflected on all other connected users’ screens.
4. Live Sports Updates
Sports websites and apps use WebSockets to provide live score updates, play-by-play commentary, and real-time statistics without requiring page refreshes.
5. Financial Trading Platforms
Stock trading platforms utilize sockets to stream real-time market data, allowing traders to make informed decisions based on up-to-the-second information.
6. IoT (Internet of Things) Devices
IoT devices often use sockets to communicate with central servers or other devices, enabling real-time monitoring and control of smart home appliances, industrial sensors, and more.
Challenges and Considerations
While sockets and WebSockets offer powerful capabilities for real-time communication, there are several challenges and considerations to keep in mind:
1. Scalability
As the number of concurrent connections increases, managing these connections efficiently becomes crucial. Techniques like connection pooling and load balancing are often employed to handle high traffic.
2. Security
Open connections can be vulnerable to attacks. Implementing proper authentication, encryption (such as SSL/TLS), and input validation is essential to protect against potential security threats.
3. Connection Management
Handling disconnections, reconnections, and timeout scenarios gracefully is important for maintaining a smooth user experience, especially in unreliable network conditions.
4. Browser Compatibility
While WebSockets are widely supported in modern browsers, you may need to provide fallback mechanisms for older browsers that don’t support WebSockets.
5. Data Synchronization
In applications where multiple clients can modify shared data simultaneously, implementing proper synchronization mechanisms is crucial to avoid conflicts and ensure data consistency.
Advanced Topics in Socket Programming
As you delve deeper into socket programming, you’ll encounter more advanced concepts and techniques:
1. Non-blocking I/O
Non-blocking sockets allow a program to continue executing while waiting for I/O operations to complete, improving efficiency in handling multiple connections.
2. Asynchronous Programming
Using asynchronous programming models (like async/await in many languages) can greatly simplify working with sockets, especially when dealing with multiple connections.
3. Protocol Design
For complex applications, designing a custom protocol on top of raw sockets can provide more structure and efficiency in communication.
4. Socket.IO
Socket.IO is a popular library that provides an abstraction layer over WebSockets (and other transport mechanisms), offering features like automatic reconnection, room support, and broadcasting.
5. WebRTC (Web Real-Time Communication)
While not directly related to sockets, WebRTC is another technology worth exploring for peer-to-peer real-time communication in web applications.
Conclusion
Sockets and WebSockets form the backbone of real-time communication in modern applications. By providing a direct, bidirectional channel between clients and servers, they enable the development of responsive, interactive applications that were once thought impossible.
As we’ve explored in this guide, implementing basic socket communication is relatively straightforward, but mastering the intricacies of efficient, scalable, and secure real-time systems requires deeper understanding and practice.
Whether you’re building a chat application, a collaborative tool, or a complex IoT system, a solid grasp of socket programming will be invaluable. As you continue your journey in software development, remember that real-time communication is not just about speed – it’s about creating seamless, engaging experiences that bring users closer to the information and interactions they need.
The world of socket programming is vast and ever-evolving. As new protocols and technologies emerge, staying updated and continuously experimenting will help you harness the full power of real-time communication in your applications. Happy coding, and may your sockets always remain open and responsive!