Introduction to Recursion Trees and Visualization
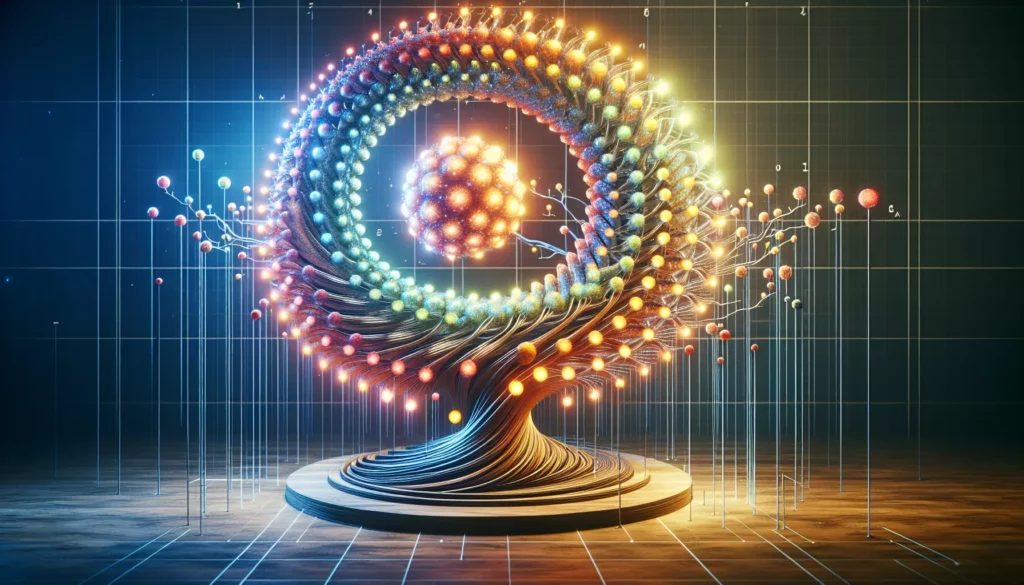
Welcome to AlgoCademy’s comprehensive guide on recursion trees and visualization! If you’re looking to level up your programming skills and prepare for technical interviews at top tech companies, understanding recursion and its visual representation is crucial. In this article, we’ll dive deep into the world of recursion trees, explore their importance in algorithm analysis, and learn how to visualize recursive processes effectively.
What is Recursion?
Before we delve into recursion trees, let’s quickly recap what recursion is. Recursion is a programming technique where a function calls itself to solve a problem by breaking it down into smaller, similar subproblems. It’s a powerful concept used in many algorithms and is often the key to solving complex problems elegantly.
Here’s a simple example of a recursive function that calculates the factorial of a number:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
While this code is concise and easy to understand, visualizing how it works for larger inputs can be challenging. This is where recursion trees come in handy.
What are Recursion Trees?
A recursion tree is a visual representation of the recursive calls made during the execution of a recursive algorithm. It helps us understand the flow of recursion, the number of recursive calls, and the overall structure of the problem-solving process.
In a recursion tree:
- Each node represents a function call
- Children of a node represent the recursive calls made by that function
- Leaf nodes represent base cases or non-recursive calls
Recursion trees are invaluable tools for:
- Analyzing the time complexity of recursive algorithms
- Debugging recursive functions
- Understanding the flow of recursive processes
- Optimizing recursive algorithms
How to Draw a Recursion Tree
Let’s walk through the process of drawing a recursion tree for our factorial function. We’ll use n = 4 as an example.
- Start with the initial function call as the root node: factorial(4)
- Draw a child node for the recursive call: factorial(3)
- Continue this process until you reach the base case
- Add the return values to each node
The resulting tree would look something like this:
factorial(4)
|
factorial(3)
|
factorial(2)
|
factorial(1)
|
1
As we move back up the tree, we can add the computed values:
factorial(4) = 24
|
factorial(3) = 6
|
factorial(2) = 2
|
factorial(1) = 1
|
1
Analyzing Recursion Trees
Recursion trees are powerful tools for analyzing the time complexity of recursive algorithms. By examining the structure of the tree, we can determine:
- The number of recursive calls
- The depth of recursion
- The work done at each level
For our factorial example, we can see that:
- The number of recursive calls is linear (n)
- The depth of recursion is also linear (n)
- The work done at each level is constant (O(1))
This analysis leads us to conclude that the time complexity of our factorial function is O(n).
Visualizing More Complex Recursive Algorithms
Let’s explore a more complex example to see how recursion trees can help us understand and analyze more sophisticated algorithms. We’ll use the classic problem of computing Fibonacci numbers.
Here’s a naive recursive implementation of the Fibonacci sequence:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2)
Now, let’s draw the recursion tree for fibonacci(5):
fib(5)
/ \
fib(4) fib(3)
/ \ / \
fib(3) fib(2) fib(2) fib(1)
/ \ / \ / \
fib(2) fib(1) fib(1) fib(0) fib(1) fib(0)
/ \
fib(1) fib(0)
This tree reveals several important insights:
- There are many duplicate calculations (e.g., fib(3) is computed twice)
- The tree is not linear but exponential in growth
- The depth of the tree is n
By analyzing this tree, we can deduce that the time complexity of this naive Fibonacci implementation is O(2^n), which is highly inefficient for large values of n.
Using Recursion Trees for Optimization
One of the most valuable aspects of recursion trees is their ability to highlight inefficiencies in our algorithms. In the Fibonacci example, we can clearly see the redundant calculations. This visualization can lead us to optimize our algorithm using techniques like memoization or dynamic programming.
Here’s an optimized version using memoization:
def fibonacci_memoized(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memoized(n - 1, memo) + fibonacci_memoized(n - 2, memo)
return memo[n]
The recursion tree for this optimized version would look much different:
fib(5)
/ \
fib(4) fib(3)*
/ \
fib(3) fib(2)*
/ \
fib(2) fib(1)*
/ \
fib(1) fib(0)*
* These values are retrieved from the memo, not recalculated
This optimized version has a linear structure, resulting in a time complexity of O(n), a significant improvement over the original O(2^n).
Recursion Trees in Algorithm Design
Understanding and utilizing recursion trees can be invaluable when designing recursive algorithms. They can help you:
- Identify the base cases for your recursion
- Understand the flow of your recursive calls
- Spot potential optimizations or redundancies
- Analyze the time and space complexity of your algorithm
When tackling a new problem that seems suitable for a recursive solution, try sketching out a recursion tree. This visual aid can often provide insights that might not be immediately apparent from the code alone.
Tools for Visualizing Recursion Trees
While drawing recursion trees by hand is an excellent exercise for understanding, there are also several tools available to help automate this process:
- Python libraries like
graphviz
ornetworkx
can be used to generate recursion trees programmatically. - Online visualization tools like Recursion Visualizer allow you to input your code and see the recursion tree generated in real-time.
- IDEs like PyCharm offer debugging features that can help you visualize the call stack, which is closely related to the structure of a recursion tree.
Here’s a simple example of how you might use graphviz
to generate a recursion tree:
from graphviz import Digraph
def visualize_factorial(n):
dot = Digraph(comment='Factorial Recursion Tree')
def add_nodes(m):
if m == 0 or m == 1:
dot.node(f'factorial({m})', f'factorial({m}) = 1')
return
dot.node(f'factorial({m})', f'factorial({m})')
dot.node(f'factorial({m-1})', f'factorial({m-1})')
dot.edge(f'factorial({m})', f'factorial({m-1})')
add_nodes(m-1)
add_nodes(n)
dot.render('factorial_tree', view=True)
visualize_factorial(5)
This code will generate a PDF visualization of the factorial recursion tree for n=5.
Common Pitfalls in Recursion and How Trees Can Help
Recursion, while powerful, can be tricky to get right. Here are some common pitfalls and how recursion trees can help avoid them:
- Infinite Recursion: If your base case is incorrect or missing, you might end up with infinite recursion. A recursion tree can quickly reveal if your function is making progress towards the base case with each call.
- Excessive Recursion Depth: Some problems can lead to very deep recursion, potentially causing a stack overflow. A recursion tree can help you visualize the depth and consider alternative approaches if needed.
- Redundant Calculations: As we saw with the Fibonacci example, naive recursive implementations can lead to many redundant calculations. A recursion tree makes these redundancies visually apparent, prompting optimizations.
- Incorrect Recursive Step: If your recursive step is not correctly reducing the problem, it may not converge to the base case. A recursion tree can help you verify that each recursive call is indeed moving towards the base case.
Advanced Topics in Recursion Trees
As you become more comfortable with basic recursion trees, there are several advanced topics you can explore:
- Master Theorem: This mathematical tool uses the structure of recursion trees to analyze the time complexity of divide-and-conquer algorithms.
- Recursion Trees for Divide-and-Conquer Algorithms: Algorithms like Merge Sort and Quick Sort have interesting recursion trees that can provide insights into their performance.
- Space Complexity Analysis: Recursion trees can also be used to analyze the space complexity of recursive algorithms by considering the maximum depth of the tree and the space used at each level.
- Tail Recursion: Understanding how tail recursion optimizations work can be aided by examining the structure of recursion trees for tail-recursive functions.
Practicing with Recursion Trees
To truly master the concept of recursion trees, practice is key. Here are some exercises you can try:
- Draw the recursion tree for the Merge Sort algorithm. How does it illustrate the algorithm’s O(n log n) time complexity?
- Implement and visualize the recursion tree for the Tower of Hanoi problem. How does the tree structure change as you increase the number of disks?
- Create a recursion tree for a recursive implementation of binary search. How does it differ from the trees we’ve seen for other algorithms?
- Implement a recursive solution for generating all permutations of a string, then draw its recursion tree. What insights can you gain about the algorithm’s complexity?
Conclusion
Recursion trees are powerful tools for understanding, analyzing, and optimizing recursive algorithms. They provide visual insights into the structure and complexity of recursive processes, making them invaluable for both learning and practical application in algorithm design.
As you continue your journey in computer science and prepare for technical interviews, remember that recursion trees are more than just a theoretical concept. They’re practical tools that can help you tackle complex problems, optimize your code, and communicate your thought process effectively to interviewers.
Keep practicing with different recursive algorithms, draw out their recursion trees, and use the insights you gain to write more efficient and elegant code. With time and practice, you’ll find that recursion trees become an indispensable part of your problem-solving toolkit.
Happy coding, and may your recursion always find its base case!