Understanding Design Patterns: Singleton, Factory, and Observer
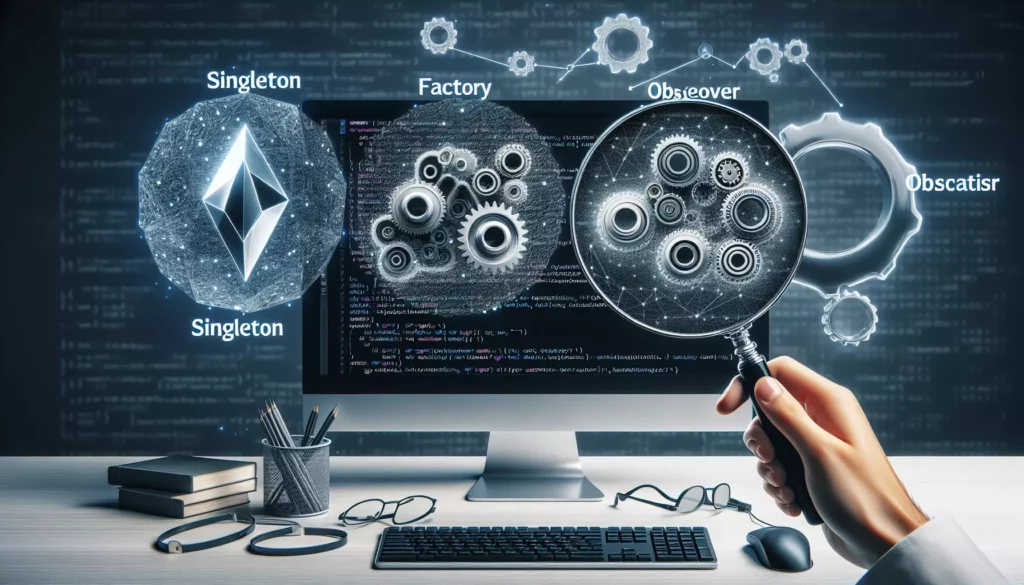
Design patterns are essential tools in a programmer’s toolkit, offering tried-and-true solutions to common software design problems. They provide reusable templates for structuring code that can improve the flexibility, maintainability, and scalability of your applications. In this comprehensive guide, we’ll dive deep into three fundamental design patterns: Singleton, Factory, and Observer. We’ll explore their concepts, implementations, use cases, and best practices to help you leverage these patterns effectively in your coding projects.
1. The Singleton Pattern
The Singleton pattern is one of the simplest yet most controversial design patterns. Its primary purpose is to ensure that a class has only one instance and provide a global point of access to that instance.
1.1 Concept and Implementation
The key idea behind the Singleton pattern is to:
- Make the constructor private to prevent direct instantiation
- Create a static method that returns the single instance of the class
- Ensure that only one instance is created throughout the application’s lifecycle
Here’s a basic implementation of the Singleton pattern in Java:
public class Singleton {
private static Singleton instance;
private Singleton() {
// Private constructor to prevent direct instantiation
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
// Other methods of the Singleton class
}
1.2 Use Cases
The Singleton pattern is useful in scenarios where you need:
- Exactly one instance of a class to coordinate actions across the system
- A global point of access to a resource (e.g., a configuration manager)
- To control concurrent access to a shared resource
Common examples include:
- Logger classes
- Database connection pools
- Configuration managers
- Thread pools
1.3 Pros and Cons
Pros:
- Ensures a class has only one instance
- Provides a global access point to that instance
- The singleton object is initialized only when it’s requested for the first time
Cons:
- Can make unit testing difficult
- Violates the Single Responsibility Principle
- Requires special treatment in multithreaded environments
1.4 Best Practices
When using the Singleton pattern:
- Consider using dependency injection instead, if possible
- Implement thread-safety if used in a multi-threaded environment
- Be cautious of overuse, as it can lead to tight coupling
2. The Factory Pattern
The Factory pattern is a creational pattern that provides an interface for creating objects in a superclass, allowing subclasses to decide which class to instantiate. It encapsulates object creation logic, promoting loose coupling and flexibility in your code.
2.1 Concept and Implementation
The Factory pattern involves:
- A factory interface or abstract class defining the method for object creation
- Concrete factory classes implementing the creation method
- Product interfaces or abstract classes defining the objects to be created
- Concrete product classes implementing the product interfaces
Here’s a simple implementation of the Factory pattern in Java:
// Product interface
interface Animal {
void makeSound();
}
// Concrete products
class Dog implements Animal {
public void makeSound() {
System.out.println("Woof!");
}
}
class Cat implements Animal {
public void makeSound() {
System.out.println("Meow!");
}
}
// Factory interface
interface AnimalFactory {
Animal createAnimal();
}
// Concrete factories
class DogFactory implements AnimalFactory {
public Animal createAnimal() {
return new Dog();
}
}
class CatFactory implements AnimalFactory {
public Animal createAnimal() {
return new Cat();
}
}
// Client code
public class FactoryPatternDemo {
public static void main(String[] args) {
AnimalFactory dogFactory = new DogFactory();
Animal dog = dogFactory.createAnimal();
dog.makeSound();
AnimalFactory catFactory = new CatFactory();
Animal cat = catFactory.createAnimal();
cat.makeSound();
}
}
2.2 Use Cases
The Factory pattern is useful when:
- A class can’t anticipate the type of objects it needs to create
- A class wants its subclasses to specify the objects it creates
- You want to encapsulate object creation logic in a separate class
Common examples include:
- UI libraries creating different types of UI elements
- Database systems supporting multiple database types
- Game engines creating different types of game objects
2.3 Pros and Cons
Pros:
- Promotes loose coupling between classes
- Simplifies object creation and allows for flexibility in creating different types
- Adheres to the Open/Closed Principle, allowing easy extension
Cons:
- Can lead to an explosion of subclasses for factories and products
- May introduce unnecessary complexity for simple object creation scenarios
2.4 Best Practices
When implementing the Factory pattern:
- Use it when you have a set of related classes that you need to instantiate
- Consider using Abstract Factory for families of related objects
- Combine with other patterns like Singleton or Builder for more complex scenarios
3. The Observer Pattern
The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. It’s a behavioral pattern that promotes loose coupling between objects.
3.1 Concept and Implementation
The Observer pattern consists of:
- Subject: The object holding the state and managing a list of observers
- Observer: An interface defining the update method for observers
- Concrete Observers: Classes implementing the Observer interface
Here’s a basic implementation of the Observer pattern in Java:
import java.util.ArrayList;
import java.util.List;
// Observer interface
interface Observer {
void update(String message);
}
// Subject class
class Subject {
private List<Observer> observers = new ArrayList<>();
private String state;
public void attach(Observer observer) {
observers.add(observer);
}
public void detach(Observer observer) {
observers.remove(observer);
}
public void setState(String state) {
this.state = state;
notifyObservers();
}
private void notifyObservers() {
for (Observer observer : observers) {
observer.update(state);
}
}
}
// Concrete Observer
class ConcreteObserver implements Observer {
private String name;
public ConcreteObserver(String name) {
this.name = name;
}
@Override
public void update(String message) {
System.out.println(name + " received message: " + message);
}
}
// Client code
public class ObserverPatternDemo {
public static void main(String[] args) {
Subject subject = new Subject();
Observer observer1 = new ConcreteObserver("Observer 1");
Observer observer2 = new ConcreteObserver("Observer 2");
subject.attach(observer1);
subject.attach(observer2);
subject.setState("New State");
}
}
3.2 Use Cases
The Observer pattern is useful when:
- A change in one object requires changing others, and you don’t know how many objects need to be changed
- An object should be able to notify other objects without making assumptions about who these objects are
- You need to maintain consistency between related objects without making them tightly coupled
Common examples include:
- Event handling systems in user interfaces
- Publish-subscribe systems in messaging platforms
- Model-View-Controller (MVC) architectures
3.3 Pros and Cons
Pros:
- Promotes loose coupling between the subject and observers
- Supports broadcast communication
- Makes it easy to add new observers without modifying the subject
Cons:
- Observers are notified in random order
- If not implemented carefully, it may cause memory leaks (forgotten subscriptions)
- In complex scenarios, it can be challenging to track the flow of updates
3.4 Best Practices
When implementing the Observer pattern:
- Use it when you need to maintain consistency across related objects
- Be cautious of circular references between subjects and observers
- Consider using weak references to prevent memory leaks
- Implement a mechanism for observers to unsubscribe when they’re no longer needed
4. Comparing the Patterns
While these three patterns serve different purposes, they can sometimes be used in conjunction or as alternatives to each other:
- Singleton vs. Factory: A Singleton can be used to implement a Factory when you need a single point of object creation. However, this can lead to tight coupling and should be used judiciously.
- Factory vs. Observer: These patterns often complement each other. A Factory can be used to create different types of Observers, while the Observer pattern can be used to notify objects created by a Factory.
- Singleton vs. Observer: A Singleton can implement the Observer pattern to provide a global point for event management. However, this approach should be used carefully to avoid creating a “god object” that does too much.
5. Real-world Applications
Let’s explore some real-world applications of these patterns in popular frameworks and libraries:
5.1 Singleton Pattern
- Java’s Runtime class: The
Runtime.getRuntime()
method returns the singleton instance of the Runtime class, which represents the runtime environment of the application. - Logger in Log4j: The Logger instances in Log4j are typically implemented as singletons to ensure consistent logging across an application.
5.2 Factory Pattern
- Java’s Calendar class: The
Calendar.getInstance()
method uses the Factory pattern to create the appropriate calendar object based on the locale and timezone. - Spring Framework’s BeanFactory: This is a sophisticated implementation of the Factory pattern used for creating and managing application components (beans).
5.3 Observer Pattern
- Java’s Event Handling: The event listener mechanism in Java Swing and JavaFX is based on the Observer pattern.
- React’s State Management: React’s state updates and re-rendering mechanism is inspired by the Observer pattern, where components “observe” state changes and update accordingly.
6. Advanced Considerations
6.1 Thread Safety
When implementing these patterns in multi-threaded environments, consider the following:
- Singleton: Use double-checked locking or initialize-on-demand holder idiom for thread-safe lazy initialization.
- Factory: Ensure that the object creation process is thread-safe if the factory is shared across threads.
- Observer: Use thread-safe collections for managing observers and consider synchronization when notifying observers.
6.2 Performance Implications
While these patterns offer significant benefits, they can impact performance:
- Singleton: Can lead to resource contention in high-concurrency scenarios.
- Factory: May introduce a slight overhead due to the additional abstraction layer.
- Observer: Can cause performance issues if there are many observers or if the update process is complex.
6.3 Testing Considerations
Design patterns can sometimes complicate unit testing:
- Singleton: Makes it difficult to isolate tests and can lead to test interdependence.
- Factory: May require mocking or stubbing of factory methods.
- Observer: Can make it challenging to test individual components in isolation.
Conclusion
Understanding and effectively implementing design patterns like Singleton, Factory, and Observer can significantly improve the structure and maintainability of your code. These patterns offer solutions to common design problems and promote best practices in software development.
However, it’s crucial to remember that design patterns are tools, not rules. Always consider the specific requirements of your project and use patterns judiciously. Overusing or misapplying design patterns can lead to unnecessary complexity and reduced maintainability.
As you continue your journey in software development, practice implementing these patterns in your projects. Experiment with different scenarios and combinations to gain a deeper understanding of their strengths and limitations. Remember, the goal is to write clean, efficient, and maintainable code that solves real-world problems effectively.
By mastering these fundamental design patterns, you’ll be better equipped to tackle complex software design challenges and create robust, scalable applications. Keep exploring, practicing, and refining your skills to become a more proficient and versatile developer.