How to Use Debuggers and IDE Tools Effectively: A Comprehensive Guide
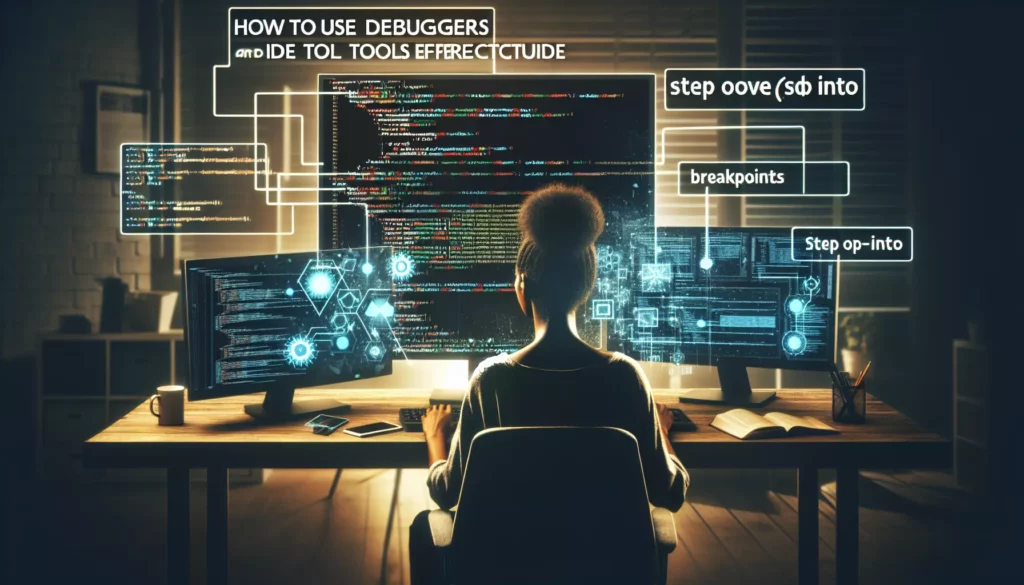
As a programmer, one of the most valuable skills you can develop is the ability to effectively use debuggers and Integrated Development Environment (IDE) tools. These powerful resources can significantly enhance your coding efficiency, help you identify and fix bugs faster, and ultimately make you a more proficient developer. In this comprehensive guide, we’ll explore the ins and outs of using debuggers and IDE tools effectively, providing you with the knowledge and techniques to level up your coding game.
1. Understanding Debuggers
Before we dive into the specifics of using debuggers effectively, let’s first understand what a debugger is and why it’s such an essential tool in a programmer’s arsenal.
What is a Debugger?
A debugger is a software tool that allows developers to monitor the execution of their code, examine the state of variables and memory at different points during runtime, and control the flow of the program. It’s like having X-ray vision for your code, enabling you to see what’s happening under the hood as your program runs.
Why Use a Debugger?
Debuggers offer several advantages over traditional debugging methods like print statements:
- They allow you to pause execution at specific points in your code.
- You can step through your code line by line, observing changes in variables and program state.
- Debuggers provide a way to set conditional breakpoints, which trigger only when certain conditions are met.
- They offer the ability to modify variable values on the fly during debugging sessions.
- Many debuggers allow you to evaluate expressions and execute arbitrary code during a debugging session.
2. Getting Started with Debuggers
Now that we understand the importance of debuggers, let’s look at how to start using them effectively.
Setting Breakpoints
Breakpoints are the foundation of debugging. They allow you to pause program execution at specific lines of code. To set a breakpoint:
- Identify the line of code where you want to pause execution.
- Click in the margin next to that line in your IDE, or use a keyboard shortcut (often F9 or Cmd/Ctrl + B).
- A red dot or highlight should appear, indicating the breakpoint.
Running in Debug Mode
To use your breakpoints, you need to run your program in debug mode:
- Look for a “Debug” option in your IDE (often represented by a bug icon).
- Click this to start your program in debug mode.
- Your program will run normally until it hits a breakpoint.
Stepping Through Code
Once your program is paused at a breakpoint, you can step through your code:
- Step Over (F10): Execute the current line and move to the next one.
- Step Into (F11): If the current line contains a function call, step into that function.
- Step Out (Shift + F11): If you’re inside a function, execute the rest of the function and return to the calling code.
3. Advanced Debugging Techniques
As you become more comfortable with basic debugging, you can start to leverage more advanced techniques to debug your code more efficiently.
Conditional Breakpoints
Conditional breakpoints only pause execution when a specific condition is met. This is useful when you’re dealing with loops or want to break only in certain scenarios. To set a conditional breakpoint:
- Set a normal breakpoint.
- Right-click on the breakpoint and select “Edit Breakpoint” or a similar option.
- Enter a condition (e.g., “i == 50” to break when a loop variable i equals 50).
Watch Expressions
Watch expressions allow you to monitor the value of variables or expressions as you step through your code. To add a watch expression:
- Look for a “Watch” or “Variables” pane in your IDE’s debug view.
- Add a new watch expression (often with a + button).
- Enter the variable name or expression you want to monitor.
Call Stack Navigation
The call stack shows you the sequence of function calls that led to the current point of execution. Understanding and navigating the call stack can help you trace the flow of your program:
- Look for a “Call Stack” or “Stack Trace” pane in your IDE’s debug view.
- Click on different entries in the call stack to jump to different points in your code’s execution history.
4. IDE Tools for Enhanced Productivity
Modern IDEs offer a wealth of tools beyond just debugging to enhance your productivity as a developer. Let’s explore some of these powerful features.
Code Completion and IntelliSense
Code completion (often called IntelliSense in Microsoft environments) is a feature that suggests completions for your code as you type. It can significantly speed up your coding process and help prevent typos. To use code completion effectively:
- Start typing a variable or function name, then look for the suggestion popup.
- Use arrow keys to navigate suggestions and Enter or Tab to accept them.
- Learn your IDE’s shortcut for manually triggering code completion (often Ctrl + Space).
Refactoring Tools
Refactoring tools help you restructure your code without changing its external behavior. Common refactoring operations include:
- Rename: Change the name of a variable, function, or class throughout your codebase.
- Extract Method: Turn a piece of code into its own function.
- Move: Relocate functions or classes to different files or modules.
To use refactoring tools:
- Select the code you want to refactor.
- Right-click and look for a “Refactor” option, or use your IDE’s refactoring shortcut.
- Choose the refactoring operation you want to perform.
Version Control Integration
Most modern IDEs integrate with version control systems like Git. This integration allows you to perform version control operations directly from your IDE. Key features include:
- Viewing file status and changes
- Committing changes
- Creating and switching branches
- Resolving merge conflicts
To use version control in your IDE:
- Look for a “Version Control” or “Git” pane in your IDE.
- Use this pane to stage, commit, and push changes.
- Learn your IDE’s shortcuts for common version control operations.
5. Optimizing Your IDE Setup
To get the most out of your IDE, it’s important to customize it to fit your workflow and preferences.
Keyboard Shortcuts
Learning and customizing keyboard shortcuts can significantly speed up your coding process. Some essential shortcuts to learn include:
- Navigate to Definition (often F12 or Cmd/Ctrl + Click)
- Find All References (often Shift + F12)
- Quick Fix / Show Intentions (often Alt + Enter)
- Rename (often F2)
To customize shortcuts:
- Look for a “Keyboard Shortcuts” or “Key Bindings” section in your IDE’s settings.
- Search for the action you want to customize.
- Assign or modify the shortcut for that action.
Code Snippets and Templates
Code snippets allow you to quickly insert commonly used code patterns. Many IDEs come with pre-defined snippets, and you can create your own. To use snippets:
- Start typing the snippet keyword (e.g., “for” for a for loop).
- Look for the snippet in the code completion suggestions.
- Select the snippet to insert it.
To create custom snippets:
- Look for a “Snippets” or “Templates” section in your IDE’s settings.
- Create a new snippet, giving it a name and a trigger keyword.
- Define the snippet’s code, using placeholders for parts you’ll want to customize when inserting.
Extensions and Plugins
Most IDEs support extensions or plugins that can add new features or enhance existing ones. Some popular categories of extensions include:
- Language support for additional programming languages
- Linters and code quality tools
- Themes and visual customizations
- Productivity tools (e.g., advanced clipboard managers, TODO list integrations)
To find and install extensions:
- Look for an “Extensions” or “Plugins” marketplace in your IDE.
- Browse or search for extensions that interest you.
- Click “Install” to add the extension to your IDE.
6. Debugging Best Practices
To make the most of your debugging sessions, consider adopting these best practices:
Reproduce the Bug Consistently
Before diving into debugging, ensure you can consistently reproduce the bug. This might involve:
- Creating a specific test case that triggers the bug
- Documenting the exact steps to reproduce the issue
- Identifying any relevant input data or environmental factors
Use Logging Effectively
While debuggers are powerful, sometimes traditional logging is still useful, especially for production issues. Consider:
- Using a logging framework that allows different log levels (e.g., DEBUG, INFO, WARN, ERROR)
- Including relevant context in log messages (e.g., function parameters, current state)
- Avoiding logging sensitive information (e.g., passwords, personal data)
Leverage Unit Tests
Unit tests can be an excellent debugging tool. They allow you to:
- Isolate specific components or functions for testing
- Automatically verify that fixes don’t break existing functionality
- Create regression tests to prevent bugs from reoccurring
Use Version Control Wisely
Version control can be a powerful ally in debugging:
- Use meaningful commit messages to track changes
- Create branches for significant changes or experimental fixes
- Use git bisect or similar tools to identify when a bug was introduced
7. Common Debugging Scenarios and Solutions
Let’s look at some common debugging scenarios and how to approach them using the tools and techniques we’ve discussed.
Null Pointer Exceptions
Null pointer exceptions occur when you try to use a variable that hasn’t been initialized. To debug:
- Set a breakpoint where the exception occurs.
- Run in debug mode and examine the variables when execution pauses.
- Use the call stack to trace back and find where the null value was introduced.
Infinite Loops
Infinite loops can be tricky to debug because they can cause your program to hang. To tackle them:
- Set a breakpoint inside the suspected loop.
- Use conditional breakpoints to break after a certain number of iterations.
- Examine loop variables to see why the exit condition isn’t being met.
Race Conditions
Race conditions in multi-threaded programs can be some of the most challenging bugs to track down. Strategies include:
- Use thread-aware debuggers that can show the state of all threads.
- Set breakpoints in critical sections where shared resources are accessed.
- Use logging to capture the sequence of events leading to the race condition.
Memory Leaks
Memory leaks can cause your program to consume more and more memory over time. To debug:
- Use memory profiling tools provided by your IDE or external tools.
- Set breakpoints and examine object lifecycles to ensure proper cleanup.
- Look for objects that are unintentionally kept in scope.
8. Advanced IDE Features for Coding Interviews
When preparing for coding interviews, especially for major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), your IDE can be a powerful ally. Here are some advanced features that can help you excel in technical interviews:
Code Metrics and Analysis
Many IDEs offer built-in or plugin-based code analysis tools that can help you improve your code quality:
- Cyclomatic complexity analysis to identify overly complex methods
- Code duplication detection to encourage DRY (Don’t Repeat Yourself) principles
- Performance hints to spot potential bottlenecks
Use these tools to refine your solutions and ensure they meet the high standards expected in technical interviews.
Time Complexity Visualization
Some IDEs or plugins offer the ability to visualize the time complexity of your algorithms. This can be incredibly useful when optimizing your solutions for coding challenges:
- Look for extensions that provide Big O notation analysis.
- Use these tools to identify inefficient algorithms or data structures in your code.
- Practice refactoring your solutions to improve their time complexity.
Interactive Coding Environments
Many modern IDEs offer interactive coding environments or notebooks, similar to Jupyter notebooks. These can be excellent for interview preparation:
- Use them to quickly prototype and test algorithm ideas.
- Leverage their ability to mix code, documentation, and visualizations.
- Practice explaining your thought process by adding markdown comments alongside your code.
Integration with Online Judges
Some IDEs offer plugins that integrate with online coding platforms like LeetCode, HackerRank, or Codeforces. These integrations can streamline your interview preparation:
- Solve problems directly in your familiar IDE environment.
- Use your custom snippets and keyboard shortcuts while solving problems.
- Easily submit solutions and view results without leaving your IDE.
9. Debugging and IDE Best Practices for Different Programming Paradigms
Different programming paradigms often require different debugging approaches and IDE setups. Let’s explore some best practices for common paradigms:
Object-Oriented Programming (OOP)
When debugging OOP code:
- Use the debugger’s object inspector to examine object states and relationships.
- Leverage IDE features like “Go to Definition” to navigate complex class hierarchies.
- Use UML diagram generators to visualize class relationships.
Functional Programming
For functional programming:
- Use debuggers that support immutable data structures and pure functions.
- Leverage IDE features that help visualize function composition and data flow.
- Use REPL (Read-Eval-Print Loop) environments for quick function testing.
Concurrent and Parallel Programming
When working with concurrent or parallel code:
- Use thread-aware debuggers to pause and inspect multiple threads simultaneously.
- Leverage IDE features that help visualize thread interactions and synchronization points.
- Use static analysis tools to detect potential race conditions or deadlocks.
10. Continuous Learning and Improvement
The world of software development is constantly evolving, and so are the tools we use. To stay effective, it’s crucial to continuously learn and improve your debugging and IDE skills:
Stay Updated
- Regularly check for updates to your IDE and install them to get the latest features and bug fixes.
- Follow blogs, forums, or social media accounts related to your IDE to learn about new features and best practices.
- Attend webinars or workshops focused on advanced IDE usage and debugging techniques.
Experiment with New Tools
- Try out different IDEs or editors to expose yourself to new workflows and features.
- Experiment with specialized debugging tools for performance profiling, memory analysis, or specific languages/frameworks.
- Explore cloud-based IDEs and collaborative coding platforms to prepare for remote pair programming scenarios.
Share Knowledge
- Participate in coding communities and share your debugging experiences and IDE tips.
- Consider writing blog posts or creating video tutorials about debugging techniques you’ve found particularly useful.
- Mentor junior developers, teaching them effective debugging practices and IDE usage.
Conclusion
Mastering debuggers and IDE tools is an essential skill for any programmer, especially those aiming for positions at top tech companies. By understanding how to leverage these tools effectively, you can significantly improve your coding efficiency, problem-solving skills, and overall productivity.
Remember that becoming proficient with these tools takes time and practice. Don’t be discouraged if you find some advanced features challenging at first. Keep experimenting, learning, and refining your skills. As you become more comfortable with your debugging and IDE tools, you’ll find that they become an extension of your thinking process, allowing you to tackle even the most complex coding challenges with confidence.
Whether you’re preparing for a coding interview, working on a personal project, or contributing to a large-scale application, the skills you’ve learned in this guide will serve you well. Happy coding, and may your bugs be few and your debugging sessions short!