Understanding Asynchronous Programming Concepts
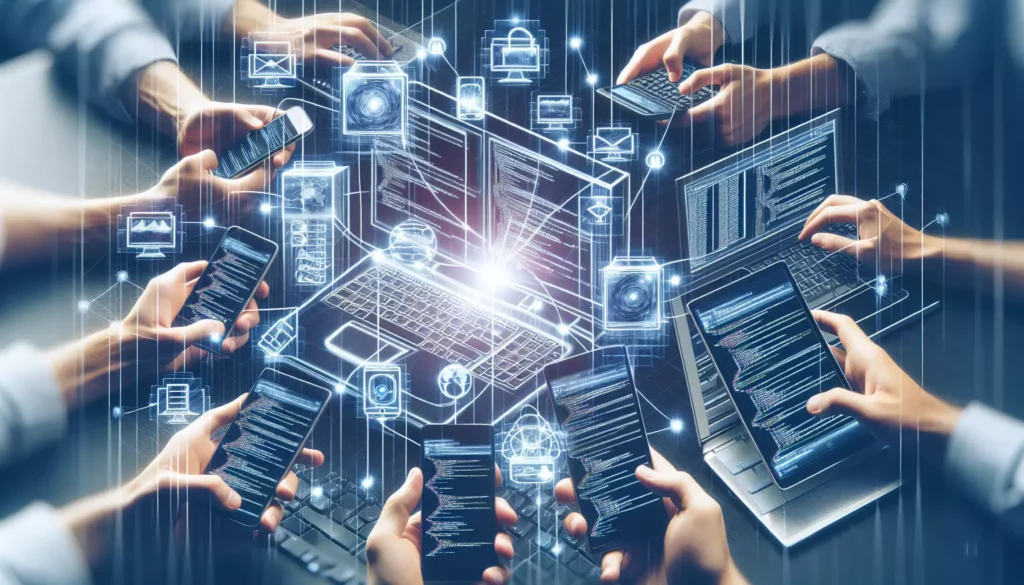
In the world of modern software development, asynchronous programming has become an essential concept that every programmer should master. As applications grow more complex and user expectations for responsiveness increase, the ability to handle multiple tasks concurrently without blocking the main execution thread has become crucial. This article will dive deep into asynchronous programming concepts, exploring their importance, implementation, and best practices across various programming languages.
What is Asynchronous Programming?
Asynchronous programming is a programming paradigm that allows operations to be executed independently of the main program flow. In contrast to synchronous programming, where tasks are executed sequentially one after another, asynchronous programming enables multiple operations to be initiated and processed concurrently.
The primary goal of asynchronous programming is to improve the overall efficiency and responsiveness of applications, particularly in scenarios involving I/O operations, network requests, or any tasks that may take an unpredictable amount of time to complete.
Key Concepts in Asynchronous Programming
- Non-blocking execution: Operations are initiated without waiting for their completion, allowing the program to continue executing other tasks.
- Callbacks: Functions that are passed as arguments to asynchronous operations and are executed once the operation completes.
- Promises: Objects representing the eventual completion or failure of an asynchronous operation.
- Async/Await: Syntactic sugar built on top of promises, providing a more readable and maintainable way to write asynchronous code.
- Event Loop: A programming construct that waits for and dispatches events or messages in a program.
The Importance of Asynchronous Programming
Asynchronous programming is crucial for several reasons:
- Improved Performance: By allowing multiple operations to run concurrently, asynchronous programming can significantly improve the overall performance of applications, especially those that are I/O-bound.
- Enhanced User Experience: Asynchronous operations prevent the user interface from freezing during long-running tasks, resulting in a more responsive and smoother user experience.
- Scalability: Asynchronous programming enables better resource utilization, allowing applications to handle a larger number of concurrent operations efficiently.
- Real-time Applications: For applications that require real-time updates or continuous data streaming, asynchronous programming is essential to maintain responsiveness and handle multiple data sources simultaneously.
Asynchronous Programming in Different Languages
While the core concepts of asynchronous programming remain consistent across languages, the implementation details and syntax can vary. Let’s explore how asynchronous programming is handled in some popular programming languages:
JavaScript
JavaScript, being single-threaded, heavily relies on asynchronous programming to maintain responsiveness. It provides several mechanisms for handling asynchronous operations:
1. Callbacks
Callbacks are the most basic form of asynchronous programming in JavaScript. They are functions passed as arguments to other functions and are executed once the asynchronous operation completes.
function fetchData(callback) {
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
callback(data);
}, 1000);
}
fetchData((result) => {
console.log(result);
});
While callbacks are simple to understand, they can lead to callback hell when dealing with multiple nested asynchronous operations, making the code hard to read and maintain.
2. Promises
Promises provide a more structured way to handle asynchronous operations. They represent a value that may not be available immediately but will be resolved at some point in the future.
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
resolve(data);
}, 1000);
});
}
fetchData()
.then((result) => {
console.log(result);
})
.catch((error) => {
console.error(error);
});
3. Async/Await
Async/Await is syntactic sugar built on top of promises, providing a more synchronous-looking way to write asynchronous code. It makes asynchronous code easier to read and maintain.
async function fetchData() {
return new Promise((resolve) => {
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
resolve(data);
}, 1000);
});
}
async function processData() {
try {
const result = await fetchData();
console.log(result);
} catch (error) {
console.error(error);
}
}
processData();
Python
Python supports asynchronous programming through its asyncio module and the async/await syntax introduced in Python 3.5+.
import asyncio
async def fetch_data():
await asyncio.sleep(1) # Simulating an asynchronous operation
return {"id": 1, "name": "John Doe"}
async def main():
result = await fetch_data()
print(result)
asyncio.run(main())
C#
C# provides robust support for asynchronous programming through its Task-based Asynchronous Pattern (TAP) and the async/await keywords.
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main()
{
var result = await FetchDataAsync();
Console.WriteLine(result);
}
static async Task<string> FetchDataAsync()
{
await Task.Delay(1000); // Simulating an asynchronous operation
return "{"id": 1, "name": "John Doe"}";
}
}
Best Practices for Asynchronous Programming
To effectively utilize asynchronous programming, consider the following best practices:
- Use async/await when possible: It provides cleaner and more readable code compared to callbacks or raw promises.
- Avoid blocking operations in asynchronous code: Blocking operations can negate the benefits of asynchronous programming.
- Handle errors properly: Use try-catch blocks or .catch() methods to handle errors in asynchronous operations.
- Be mindful of concurrency issues: When multiple asynchronous operations access shared resources, ensure proper synchronization to avoid race conditions.
- Use cancellation tokens: Implement cancellation mechanisms to allow long-running asynchronous operations to be terminated gracefully.
- Avoid excessive nesting: Use promise chaining or async/await to flatten nested asynchronous calls and improve readability.
- Consider using libraries: Utilize well-established libraries for complex asynchronous scenarios, such as RxJS for reactive programming.
Common Pitfalls in Asynchronous Programming
While asynchronous programming offers numerous benefits, it also comes with its own set of challenges. Here are some common pitfalls to watch out for:
1. Callback Hell
Callback hell, also known as the “pyramid of doom,” occurs when multiple nested callbacks are used, making the code difficult to read and maintain.
fetchData((result1) => {
processData(result1, (result2) => {
updateDatabase(result2, (result3) => {
displayResults(result3, () => {
// More nested callbacks...
});
});
});
});
To avoid callback hell, use promises, async/await, or break down the code into smaller, more manageable functions.
2. Forgetting to Handle Errors
Failing to handle errors in asynchronous code can lead to silent failures or unhandled promise rejections. Always include error handling in your asynchronous operations.
async function fetchData() {
try {
const response = await fetch("https://api.example.com/data");
const data = await response.json();
return data;
} catch (error) {
console.error("Error fetching data:", error);
throw error;
}
}
3. Blocking the Event Loop
Performing CPU-intensive operations or using synchronous functions in asynchronous code can block the event loop, defeating the purpose of asynchronous programming.
async function processLargeData(data) {
// BAD: This will block the event loop
for (let i = 0; i < 1000000000; i++) {
data[i] = data[i] * 2;
}
return data;
}
// GOOD: Use web workers or break the task into smaller chunks
async function processLargeDataAsync(data) {
return new Promise((resolve) => {
const worker = new Worker("worker.js");
worker.postMessage(data);
worker.onmessage = (event) => {
resolve(event.data);
};
});
}
4. Race Conditions
When multiple asynchronous operations access shared resources, race conditions can occur, leading to unexpected behavior.
let count = 0;
async function incrementCount() {
// BAD: This can lead to race conditions
count++;
console.log(count);
}
// GOOD: Use proper synchronization mechanisms
let count = 0;
const mutex = new Mutex();
async function incrementCount() {
await mutex.acquire();
try {
count++;
console.log(count);
} finally {
mutex.release();
}
}
Advanced Asynchronous Patterns
As you become more comfortable with basic asynchronous programming, you may encounter more advanced patterns and concepts:
1. Generators and Coroutines
Generators and coroutines provide a way to pause and resume the execution of a function, allowing for more complex asynchronous flows.
function* numberGenerator() {
yield 1;
yield 2;
yield 3;
}
const gen = numberGenerator();
console.log(gen.next().value); // 1
console.log(gen.next().value); // 2
console.log(gen.next().value); // 3
2. Observables and Reactive Programming
Observables, popularized by libraries like RxJS, provide a powerful way to work with asynchronous data streams and events.
import { fromEvent } from "rxjs";
import { debounceTime, map } from "rxjs/operators";
const input = document.getElementById("search-input");
const keyup$ = fromEvent(input, "keyup");
keyup$
.pipe(
map((event) => event.target.value),
debounceTime(300)
)
.subscribe((value) => {
console.log("Search query:", value);
});
3. Asynchronous Iterators
Asynchronous iterators allow you to iterate over asynchronously generated data, combining the power of iterators with asynchronous programming.
async function* asyncNumberGenerator() {
yield await Promise.resolve(1);
yield await Promise.resolve(2);
yield await Promise.resolve(3);
}
(async () => {
for await (const num of asyncNumberGenerator()) {
console.log(num);
}
})();
Testing Asynchronous Code
Testing asynchronous code presents its own set of challenges. Here are some strategies for effectively testing asynchronous operations:
1. Use Async/Await in Tests
Leverage async/await in your test cases to handle asynchronous operations cleanly.
describe("fetchData function", () => {
it("should return user data", async () => {
const result = await fetchData();
expect(result).toEqual({ id: 1, name: "John Doe" });
});
});
2. Mock Asynchronous Dependencies
Use mocking libraries to simulate asynchronous dependencies and control their behavior in tests.
jest.mock("./api");
const api = require("./api");
describe("processUserData function", () => {
it("should process user data correctly", async () => {
api.fetchUserData.mockResolvedValue({ id: 1, name: "John Doe" });
const result = await processUserData(1);
expect(result).toBe("Processed: John Doe");
});
});
3. Test Error Handling
Ensure that your asynchronous error handling is properly tested.
describe("fetchData function", () => {
it("should handle network errors", async () => {
fetch.mockRejectedValue(new Error("Network error"));
await expect(fetchData()).rejects.toThrow("Network error");
});
});
Conclusion
Asynchronous programming is a fundamental concept in modern software development, enabling the creation of responsive, efficient, and scalable applications. By understanding the core principles, implementing best practices, and avoiding common pitfalls, developers can harness the full power of asynchronous programming to build robust and high-performance software.
As you continue to explore and master asynchronous programming, remember that practice is key. Experiment with different patterns, explore advanced concepts, and always strive to write clean, maintainable asynchronous code. With time and experience, you’ll develop a strong intuition for when and how to leverage asynchronous programming to solve complex problems and create exceptional user experiences.
Keep in mind that asynchronous programming is an evolving field, with new patterns and tools emerging regularly. Stay updated with the latest developments in your programming language of choice and the broader software development community to continually refine your asynchronous programming skills.
By mastering asynchronous programming concepts, you’ll be well-equipped to tackle the challenges of modern software development and create applications that can handle the demands of today’s fast-paced, interconnected world.