How to Design User Interfaces: Basics of UI/UX in Programming
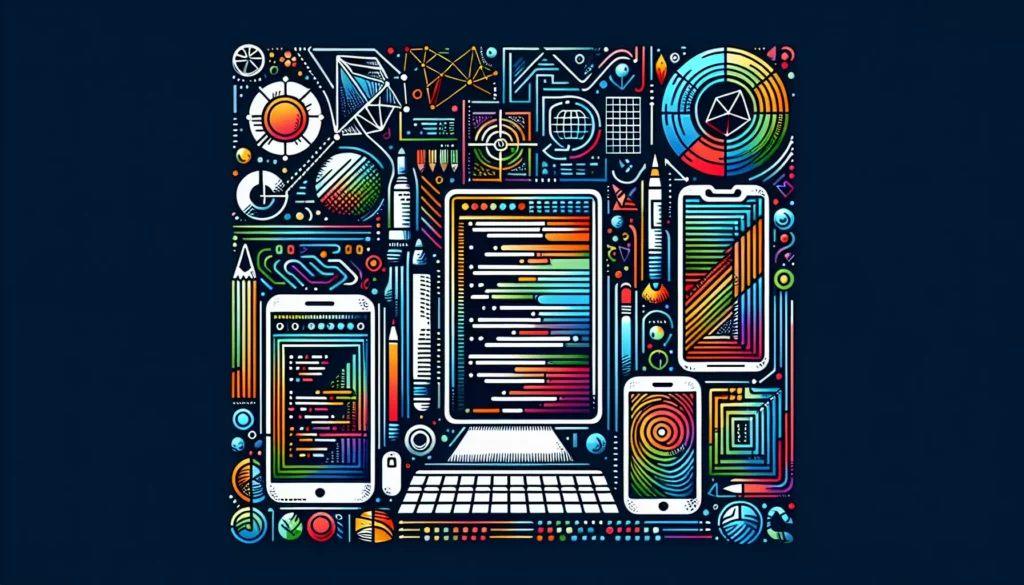
In the world of software development, creating functional and efficient code is only half the battle. The other crucial aspect is designing user interfaces (UI) that are intuitive, engaging, and provide an excellent user experience (UX). As programmers, understanding the basics of UI/UX design is essential for creating applications that users love to interact with. In this comprehensive guide, we’ll explore the fundamentals of UI/UX design in programming, providing you with the knowledge and tools to enhance your skills and create more user-friendly applications.
1. Understanding UI/UX Design
Before diving into the specifics of designing user interfaces, it’s important to understand the difference between UI and UX:
- User Interface (UI): This refers to the visual elements of an application that users interact with, such as buttons, menus, forms, and layout.
- User Experience (UX): This encompasses the overall experience a user has while interacting with an application, including ease of use, efficiency, and satisfaction.
While UI focuses on the look and feel of an application, UX is concerned with how users interact with and perceive the application. Both are crucial for creating successful software products.
2. Key Principles of UI/UX Design
To create effective user interfaces, it’s essential to follow some fundamental principles:
2.1. Simplicity
Keep your designs simple and intuitive. Users should be able to navigate your application without confusion or frustration. Avoid cluttered interfaces and unnecessary elements.
2.2. Consistency
Maintain consistency in design elements, such as colors, fonts, and button styles throughout your application. This helps users learn and remember how to interact with your interface.
2.3. Hierarchy
Use visual hierarchy to guide users’ attention to the most important elements first. This can be achieved through size, color, contrast, and positioning of elements.
2.4. Feedback
Provide clear feedback to users when they interact with your interface. This can include visual cues, animations, or messages that confirm actions or indicate errors.
2.5. Accessibility
Design your interface to be accessible to all users, including those with disabilities. This involves considering factors such as color contrast, font sizes, and keyboard navigation.
3. The Design Process
When approaching UI/UX design in programming, it’s helpful to follow a structured process:
3.1. Research and Analysis
Begin by understanding your target users, their needs, and the context in which they’ll use your application. Conduct user research, create user personas, and analyze competitor products.
3.2. Wireframing
Create low-fidelity sketches or wireframes of your interface to outline the basic structure and layout. This allows you to experiment with different ideas quickly.
3.3. Prototyping
Develop interactive prototypes to test your design concepts. This can be done using tools like Figma, Adobe XD, or InVision.
3.4. User Testing
Conduct user testing sessions to gather feedback on your prototypes. This helps identify usability issues and areas for improvement.
3.5. Iteration
Based on user feedback, refine and iterate on your designs. This process may involve multiple rounds of prototyping and testing.
3.6. Implementation
Once you’re satisfied with your design, implement it in your chosen programming language or framework.
4. UI Design Elements
When designing user interfaces, you’ll work with various elements that make up the visual and interactive aspects of your application:
4.1. Layout
The overall structure and arrangement of elements on the screen. Consider using grid systems and whitespace to create balanced and organized layouts.
4.2. Typography
Choose appropriate fonts and text styles that are legible and consistent with your design aesthetic. Pay attention to font sizes, line heights, and spacing.
4.3. Color
Select a color palette that aligns with your brand and enhances usability. Use color to create visual hierarchy and draw attention to important elements.
4.4. Icons and Images
Incorporate icons and images to enhance visual communication and make your interface more engaging. Ensure that these elements are consistent in style and easily recognizable.
4.5. Forms and Input Controls
Design user-friendly forms and input controls, such as text fields, dropdown menus, and checkboxes. Make sure they are clearly labeled and easy to interact with.
4.6. Buttons and Call-to-Actions
Create visually distinct buttons and call-to-action elements that guide users towards important actions or next steps in your application.
5. Implementing UI/UX in Code
As a programmer, you’ll need to translate your UI/UX designs into functional code. Here are some tips for implementing UI/UX principles in your programming:
5.1. Use CSS Effectively
Cascading Style Sheets (CSS) are crucial for implementing visual designs. Learn to use CSS effectively to control layout, typography, colors, and responsive design.
Example of using CSS for a responsive layout:
<style>
.container {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
}
.item {
flex-basis: calc(33.33% - 20px);
margin-bottom: 20px;
}
@media (max-width: 768px) {
.item {
flex-basis: calc(50% - 10px);
}
}
@media (max-width: 480px) {
.item {
flex-basis: 100%;
}
}
</style>
5.2. Implement Responsive Design
Ensure your interface adapts to different screen sizes and devices. Use responsive design techniques such as fluid grids, flexible images, and media queries.
5.3. Use JavaScript for Interactivity
Leverage JavaScript to add interactivity and dynamic behavior to your interface. This can include form validation, animations, and AJAX requests for smoother user experiences.
Example of form validation using JavaScript:
function validateForm() {
const emailInput = document.getElementById('email');
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!emailRegex.test(emailInput.value)) {
alert('Please enter a valid email address');
return false;
}
return true;
}
document.querySelector('form').addEventListener('submit', function(event) {
if (!validateForm()) {
event.preventDefault();
}
});
5.4. Utilize UI Frameworks and Libraries
Consider using UI frameworks and libraries like React, Vue.js, or Angular for building complex user interfaces. These tools provide pre-built components and structures that can speed up development and ensure consistency.
5.5. Implement Accessibility Features
Use semantic HTML elements and ARIA attributes to improve accessibility. Ensure your application can be navigated using a keyboard and is compatible with screen readers.
Example of using ARIA attributes for accessibility:
<button aria-label="Close dialog" aria-pressed="false" onclick="closeDialog()">
<span class="icon-close"></span>
</button>
6. Testing and Optimization
Once you’ve implemented your UI/UX design, it’s crucial to test and optimize it for the best user experience:
6.1. Usability Testing
Conduct usability tests with real users to identify any issues or areas for improvement in your interface. Observe how users interact with your application and gather feedback.
6.2. A/B Testing
Use A/B testing to compare different versions of your interface and determine which performs better. This can help you make data-driven decisions about design choices.
6.3. Performance Optimization
Optimize your application’s performance to ensure fast loading times and smooth interactions. This may involve minimizing HTTP requests, optimizing images, and using efficient coding practices.
6.4. Cross-browser and Cross-device Testing
Test your application across different browsers and devices to ensure consistent functionality and appearance.
7. Staying Updated with UI/UX Trends
The field of UI/UX design is constantly evolving. To stay current, consider the following practices:
7.1. Follow Design Blogs and Publications
Stay informed about the latest trends and best practices by following design blogs, publications, and thought leaders in the UI/UX field.
7.2. Attend Workshops and Conferences
Participate in UI/UX workshops and conferences to learn from experts and network with other professionals in the field.
7.3. Experiment with New Tools and Technologies
Keep an eye on new design and prototyping tools, as well as emerging technologies like AR/VR that may impact UI/UX design.
8. Integrating UI/UX Design with Coding Education
As you progress in your coding education and prepare for technical interviews, it’s important to consider how UI/UX design principles can be integrated into your learning journey:
8.1. Practical Projects
When working on coding projects, pay attention to the user interface and experience aspects. Challenge yourself to create not just functional applications, but ones that are also visually appealing and user-friendly.
8.2. Design Thinking in Problem-Solving
Apply design thinking principles to problem-solving in your coding exercises. Consider the user’s perspective and needs when developing algorithms and solutions.
8.3. UI/UX in Technical Interviews
Be prepared to discuss UI/UX considerations in technical interviews, especially for front-end or full-stack positions. Demonstrate your understanding of how design choices impact user satisfaction and overall product success.
8.4. Collaboration Skills
Develop skills in collaborating with designers and other team members. Understanding UI/UX principles will help you communicate more effectively with design teams and contribute to creating better user experiences.
Conclusion
Mastering the basics of UI/UX design is an invaluable skill for programmers. By understanding and implementing these principles, you can create applications that not only function well but also provide exceptional user experiences. Remember that great UI/UX design is an iterative process that requires continuous learning and refinement.
As you continue your coding education and prepare for technical interviews, keep in mind the importance of UI/UX in modern software development. By combining your programming skills with a strong foundation in UI/UX design, you’ll be well-equipped to tackle complex projects and stand out in the competitive tech industry.
Practice implementing these UI/UX principles in your coding projects, seek feedback from users, and always strive to create interfaces that are intuitive, accessible, and enjoyable to use. With dedication and practice, you’ll be able to create applications that not only meet technical requirements but also delight users and solve real-world problems effectively.