Understanding Regular Expressions for Pattern Matching
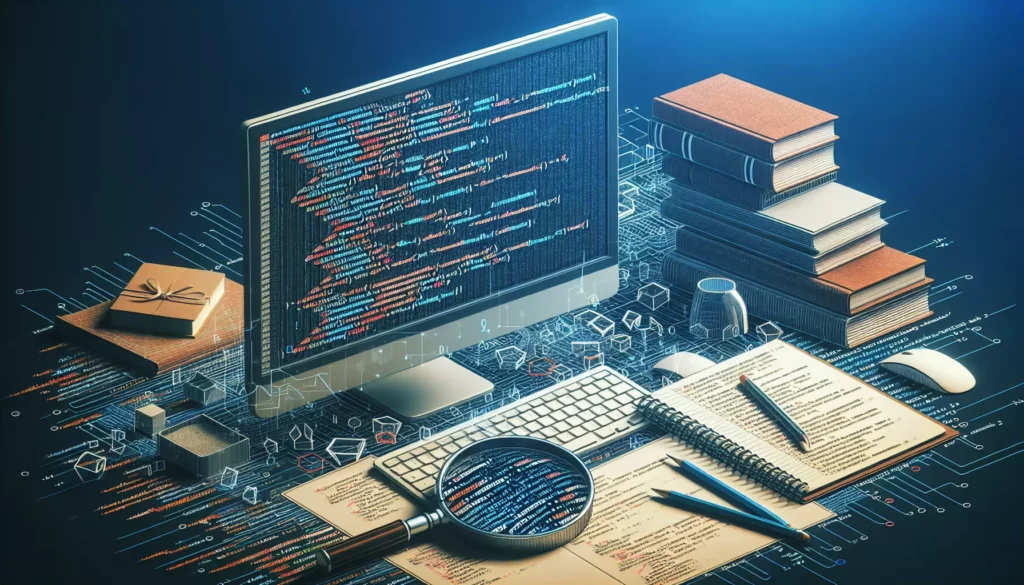
Regular expressions, often abbreviated as regex or regexp, are powerful tools for pattern matching and text manipulation in programming. They provide a concise and flexible means to search, extract, and manipulate strings based on specific patterns. In this comprehensive guide, we’ll dive deep into the world of regular expressions, exploring their syntax, applications, and best practices. Whether you’re a beginner looking to grasp the basics or an experienced developer aiming to refine your skills, this article will equip you with the knowledge to leverage regex effectively in your coding projects.
What are Regular Expressions?
Regular expressions are sequences of characters that define a search pattern. They are used in string searching algorithms for “find” or “find and replace” operations on strings, or for input validation. Regular expressions are supported in many programming languages and text editors, making them a versatile tool for developers across different platforms.
Why Learn Regular Expressions?
- Powerful text processing: Regex allows you to perform complex string operations with concise syntax.
- Versatility: Regular expressions can be used in various programming languages and tools.
- Efficiency: Well-crafted regex can significantly reduce the amount of code needed for string manipulation tasks.
- Data validation: Regex is excellent for validating user input, such as email addresses or phone numbers.
- Search and replace: Regex enables complex search and replace operations in text editors and programming environments.
Basic Syntax and Metacharacters
Regular expressions consist of ordinary characters and special characters known as metacharacters. Let’s explore some fundamental elements of regex syntax:
Literal Characters
Most characters in a regular expression are treated as literal characters, meaning they match themselves. For example, the regex pattern “hello” will match the exact string “hello” in the text.
Metacharacters
Metacharacters have special meanings in regex. Here are some common metacharacters:
- . (dot): Matches any single character except newline.
- ^ (caret): Matches the start of a line.
- $ (dollar): Matches the end of a line.
- * (asterisk): Matches zero or more occurrences of the previous character or group.
- + (plus): Matches one or more occurrences of the previous character or group.
- ? (question mark): Matches zero or one occurrence of the previous character or group.
- [ ] (square brackets): Defines a character set. Matches any single character within the brackets.
- ( ) (parentheses): Groups characters together and creates a capturing group.
- | (pipe): Acts as an OR operator, matching either the expression before or after it.
Example: Basic Pattern Matching
Let’s look at a simple example of using regex to match an email address pattern:
\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b
This pattern breaks down as follows:
- \b: Word boundary
- [A-Za-z0-9._%+-]+: One or more letters, digits, or certain special characters
- @: Literal “@” symbol
- [A-Za-z0-9.-]+: One or more letters, digits, dots, or hyphens
- \.: Literal dot
- [A-Z|a-z]{2,}: Two or more letters (domain extension)
- \b: Word boundary
Character Classes and Quantifiers
Character classes and quantifiers are essential components of regex that allow for more flexible and powerful pattern matching.
Character Classes
Character classes define a set of characters that can match at a particular position in the regex. Some common character classes include:
- [abc]: Matches any single character a, b, or c
- [^abc]: Matches any single character except a, b, or c
- [a-z]: Matches any single lowercase letter from a to z
- [A-Z]: Matches any single uppercase letter from A to Z
- [0-9]: Matches any single digit from 0 to 9
Predefined Character Classes
Regex also provides shorthand notation for commonly used character classes:
- \d: Matches any digit (equivalent to [0-9])
- \D: Matches any non-digit (equivalent to [^0-9])
- \w: Matches any word character (letters, digits, or underscore)
- \W: Matches any non-word character
- \s: Matches any whitespace character (space, tab, newline)
- \S: Matches any non-whitespace character
Quantifiers
Quantifiers specify how many times a character or group should occur. Common quantifiers include:
- *: Matches zero or more occurrences
- +: Matches one or more occurrences
- ?: Matches zero or one occurrence
- {n}: Matches exactly n occurrences
- {n,}: Matches n or more occurrences
- {n,m}: Matches between n and m occurrences
Example: Using Character Classes and Quantifiers
Let’s create a regex pattern to match a simple date format (DD/MM/YYYY):
\b(0[1-9]|[12][0-9]|3[01])\/(0[1-9]|1[0-2])\/\d{4}\b
This pattern uses character classes and quantifiers to ensure:
- The day is between 01 and 31
- The month is between 01 and 12
- The year is exactly four digits
Anchors and Boundaries
Anchors and boundaries in regex help specify the position of a match within the text. They don’t match any characters themselves but rather assert a position in the string.
Common Anchors
- ^: Matches the start of a line
- $: Matches the end of a line
- \b: Matches a word boundary
- \B: Matches a non-word boundary
Example: Using Anchors
Let’s create a regex pattern to match lines that start with “Hello” and end with “World”:
^Hello.*World$
This pattern will match lines like:
- “Hello World”
- “Hello, how are you? Nice World”
But it won’t match:
- “Say Hello to the World” (doesn’t start with “Hello”)
- “Hello Universe” (doesn’t end with “World”)
Grouping and Capturing
Grouping and capturing are powerful features in regex that allow you to treat multiple characters as a single unit and extract specific parts of a match.
Parentheses for Grouping
Parentheses ( ) are used to group parts of a regex together. This is useful for applying quantifiers to a group of characters or creating alternative matches.
Capturing Groups
By default, parentheses create capturing groups. These groups allow you to extract specific parts of a match for further processing.
Non-Capturing Groups
If you want to group characters without creating a capturing group, you can use the syntax (?:…).
Example: Grouping and Capturing
Let’s create a regex pattern to match and extract information from a simple log entry:
(\d{4}-\d{2}-\d{2}) (\d{2}:\d{2}:\d{2}) \[(\w+)\] (.+)
This pattern will match log entries like:
2023-05-15 14:30:45 [INFO] User logged in successfully
And capture the following groups:
- Date: 2023-05-15
- Time: 14:30:45
- Log level: INFO
- Message: User logged in successfully
Lookahead and Lookbehind Assertions
Lookahead and lookbehind assertions are advanced regex features that allow you to match a pattern only if it’s followed by or preceded by another pattern, without including the latter in the match itself.
Positive Lookahead
Syntax: (?=…)
Matches if the pattern inside the parentheses occurs next, without consuming it.
Negative Lookahead
Syntax: (?!…)
Matches if the pattern inside the parentheses does not occur next.
Positive Lookbehind
Syntax: (?<=…)
Matches if the pattern inside the parentheses occurs before, without consuming it.
Negative Lookbehind
Syntax: (?<!…)
Matches if the pattern inside the parentheses does not occur before.
Example: Using Lookahead and Lookbehind
Let’s create a regex pattern to match numbers that are followed by “px” but not preceded by a “$” sign:
(?<!\$)\d+(?=px)
This pattern will match:
- “The width is 100px” (matches “100”)
- “Height: 50px” (matches “50”)
But it won’t match:
- “The price is $20px” (preceded by “$”)
- “It’s 30 pixels wide” (not followed by “px”)
Regex in Different Programming Languages
While the core concepts of regular expressions are universal, the syntax and available features can vary slightly between programming languages. Let’s look at how to use regex in some popular languages:
Python
Python provides the re
module for working with regular expressions:
import re
pattern = r'\b\w+@\w+\.\w+\b'
text = "Contact us at info@example.com or support@company.org"
matches = re.findall(pattern, text)
print(matches) # Output: ['info@example.com', 'support@company.org']
JavaScript
JavaScript has built-in support for regular expressions:
const pattern = /\b\w+@\w+\.\w+\b/g;
const text = "Contact us at info@example.com or support@company.org";
const matches = text.match(pattern);
console.log(matches); // Output: ['info@example.com', 'support@company.org']
Java
Java provides the java.util.regex
package for working with regular expressions:
import java.util.regex.*;
public class RegexExample {
public static void main(String[] args) {
String pattern = "\\b\\w+@\\w+\\.\\w+\\b";
String text = "Contact us at info@example.com or support@company.org";
Pattern r = Pattern.compile(pattern);
Matcher m = r.matcher(text);
while (m.find()) {
System.out.println(m.group());
}
}
}
Common Regex Patterns and Their Uses
Here are some commonly used regex patterns for various purposes:
Email Validation
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
URL Matching
https?:\/\/(www\.)?[-a-zA-Z0-9@:%._\+~#=]{2,256}\.[a-z]{2,6}\b([-a-zA-Z0-9@:%_\+.~#?&//=]*)
Phone Number (US Format)
\(?([0-9]{3})\)?[-. ]?([0-9]{3})[-. ]?([0-9]{4})
Strong Password
^(?=.*[A-Za-z])(?=.*\d)(?=.*[@$!%*#?&])[A-Za-z\d@$!%*#?&]{8,}$
IP Address
\b(?:(?:25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.){3}(?:25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\b
Best Practices and Performance Considerations
While regular expressions are powerful, they can also be complex and potentially impact performance if not used correctly. Here are some best practices to keep in mind:
1. Keep it Simple
Try to use the simplest regex that meets your requirements. Complex patterns can be hard to maintain and may have unexpected behavior.
2. Use Anchors
Whenever possible, use anchors (^ and $) to specify the start and end of the string. This can prevent unwanted partial matches.
3. Avoid Excessive Backtracking
Be cautious with patterns that can lead to excessive backtracking, especially when using nested quantifiers. This can cause performance issues with large inputs.
4. Use Non-Capturing Groups
If you don’t need to capture a group, use non-capturing groups (?:…) to improve performance.
5. Compile Regex Patterns
In languages that support it, compile your regex patterns once and reuse them, rather than creating new regex objects for each use.
6. Test Thoroughly
Always test your regex patterns with a variety of inputs, including edge cases and potentially problematic inputs.
7. Use Verbose Mode
For complex patterns, consider using verbose mode (if supported by your language) to make the regex more readable and maintainable.
8. Limit Use of Lookahead and Lookbehind
While powerful, excessive use of lookahead and lookbehind can impact performance. Use them judiciously.
Tools and Resources for Learning and Testing Regex
To help you master regular expressions, here are some valuable tools and resources:
Online Regex Testers
- Regex101: A powerful online regex tester with syntax highlighting and explanation.
- RegExr: An online tool to learn, build, and test regular expressions.
- Debuggex: A visual regex tester that provides a railroad diagram of your pattern.
Learning Resources
- Regular-Expressions.info: A comprehensive resource for learning about regex.
- RegexOne: An interactive tutorial for learning regex.
- RexEgg: A regex tutorial with a focus on advanced techniques.
Books
- “Mastering Regular Expressions” by Jeffrey Friedl
- “Regular Expressions Cookbook” by Jan Goyvaerts and Steven Levithan
Conclusion
Regular expressions are an indispensable tool in a programmer’s toolkit. They offer a powerful and flexible way to work with text patterns, enabling efficient string manipulation, validation, and searching. While the syntax may seem daunting at first, with practice and understanding, you’ll find that regex can significantly simplify many text-processing tasks.
As you continue to work with regular expressions, remember to balance their power with readability and performance considerations. Start with simple patterns and gradually build up to more complex ones as you gain confidence. Utilize the tools and resources mentioned to practice and refine your regex skills.
Whether you’re parsing log files, validating user input, or searching through large text datasets, mastering regular expressions will make you a more effective and efficient programmer. Keep experimenting, testing, and learning, and you’ll soon find regex becoming an invaluable part of your coding repertoire.