How to Use Version Control: An Introduction to Git
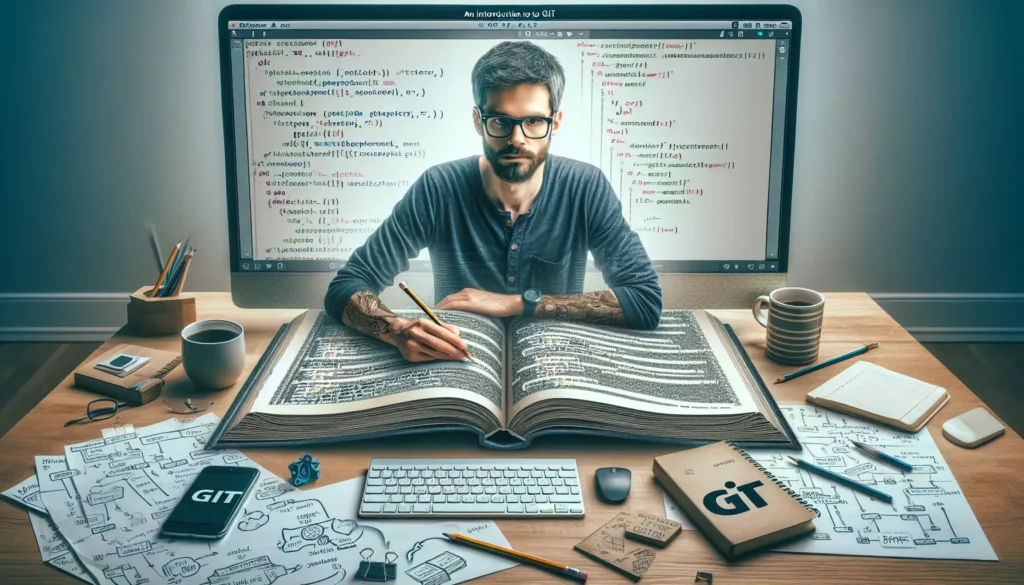
In the world of software development, version control is an indispensable tool that helps developers manage and track changes in their code over time. Among the various version control systems available, Git stands out as the most popular and widely used. Whether you’re a beginner programmer or an experienced developer, understanding how to use Git can significantly improve your workflow and collaboration with other developers. In this comprehensive guide, we’ll explore the fundamentals of Git and how to use it effectively in your projects.
What is Git?
Git is a distributed version control system created by Linus Torvalds in 2005. It was designed to handle everything from small to very large projects with speed and efficiency. Git allows multiple developers to work on the same project simultaneously, tracking changes and managing different versions of the codebase.
Some key features of Git include:
- Distributed development
- Strong support for non-linear development (thousands of parallel branches)
- Efficient handling of large projects
- Cryptographic authentication of history
- Toolkit-based design
Setting Up Git
Before we dive into using Git, let’s make sure it’s properly set up on your system.
1. Installing Git
If you haven’t already installed Git, you can download it from the official website: https://git-scm.com/downloads
Follow the installation instructions for your operating system.
2. Configuring Git
After installation, you need to set up your identity. Open a terminal or command prompt and run the following commands:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Replace “Your Name” and “your.email@example.com” with your actual name and email address.
Basic Git Concepts
Before we start using Git commands, let’s familiarize ourselves with some basic concepts:
Repository (Repo)
A repository is a directory where Git tracks changes to your files. It contains all of your project’s files and each file’s revision history.
Commit
A commit represents a specific point in your project’s history. It’s like a snapshot of your repository at a particular time.
Branch
A branch is a parallel version of your repository. It allows you to work on different features or experiments without affecting the main project.
Remote
A remote is a common repository that all team members use to exchange their changes. It’s typically hosted on a code hosting service like GitHub, GitLab, or Bitbucket.
Basic Git Workflow
Now that we understand the basic concepts, let’s go through a typical Git workflow:
1. Creating a New Repository
To create a new Git repository, navigate to your project directory in the terminal and run:
git init
This initializes a new Git repository in your current directory.
2. Checking the Status
To see which files have been changed and which are staged for commit, use:
git status
3. Adding Files to the Staging Area
Before you can commit changes, you need to add them to the staging area. To add a specific file:
git add filename.txt
To add all changed files:
git add .
4. Committing Changes
Once you’ve added files to the staging area, you can commit them:
git commit -m "Your commit message here"
Always write clear and descriptive commit messages to make it easier to understand the changes later.
5. Viewing Commit History
To see a log of all commits:
git log
For a more concise view:
git log --oneline
Working with Branches
Branches are an essential feature of Git that allow you to work on different versions of your project simultaneously.
1. Creating a New Branch
To create a new branch:
git branch branch-name
2. Switching Branches
To switch to a different branch:
git checkout branch-name
You can also create and switch to a new branch in one command:
git checkout -b new-branch-name
3. Merging Branches
To merge changes from one branch into another, first switch to the branch you want to merge into, then run:
git merge branch-to-merge
4. Deleting a Branch
Once you’ve merged a branch and no longer need it, you can delete it:
git branch -d branch-name
Working with Remotes
Remotes allow you to collaborate with other developers and share your code.
1. Adding a Remote
To add a remote repository:
git remote add origin https://github.com/username/repository.git
2. Pushing Changes to a Remote
To push your local commits to a remote repository:
git push origin branch-name
3. Pulling Changes from a Remote
To fetch and merge changes from a remote repository:
git pull origin branch-name
4. Cloning a Repository
To create a local copy of a remote repository:
git clone https://github.com/username/repository.git
Advanced Git Techniques
As you become more comfortable with Git, you might want to explore some advanced techniques:
1. Rebasing
Rebasing is an alternative to merging that can create a cleaner project history. To rebase your current branch onto another:
git rebase branch-name
2. Cherry-picking
Cherry-picking allows you to apply specific commits from one branch to another:
git cherry-pick commit-hash
3. Interactive Rebase
Interactive rebase lets you modify commits in various ways, such as squashing, editing, or reordering:
git rebase -i HEAD~n
Where ‘n’ is the number of commits you want to interact with.
4. Git Hooks
Git hooks are scripts that Git executes before or after events such as commit, push, and receive. They can be used to enforce certain workflows or coding standards.
Best Practices for Using Git
To make the most of Git and maintain a clean, efficient workflow, consider these best practices:
1. Commit Often
Make small, frequent commits rather than large, infrequent ones. This makes it easier to track changes and revert if necessary.
2. Write Clear Commit Messages
Use clear, concise commit messages that explain what changes were made and why. A common format is:
Short (50 chars or less) summary of changes
More detailed explanatory text, if necessary. Wrap it to about 72
characters or so. The blank line separating the summary from the body
is critical (unless you omit the body entirely).
Further paragraphs come after blank lines.
- Bullet points are okay, too
- Typically a hyphen or asterisk is used for the bullet, preceded
by a single space, with blank lines in between
3. Use Branches
Create a new branch for each feature or bug fix. This keeps your main branch clean and makes it easier to manage multiple features simultaneously.
4. Pull Before You Push
Always pull the latest changes from the remote repository before pushing your own changes. This helps avoid conflicts and keeps your local repository up-to-date.
5. Review Your Changes
Before committing, use git diff
to review your changes. This helps catch unintended modifications and ensures you’re only committing what you mean to.
6. Use .gitignore
Create a .gitignore file to specify which files or directories Git should ignore. This is useful for excluding build artifacts, temporary files, and sensitive information.
Git Tools and Integrations
While the command line is powerful, there are many tools and integrations that can enhance your Git experience:
1. GUI Clients
Git GUI clients provide a visual interface for Git operations. Some popular options include:
- GitKraken
- SourceTree
- GitHub Desktop
2. IDE Integrations
Many Integrated Development Environments (IDEs) come with built-in Git support or plugins:
- Visual Studio Code: Built-in Git support
- IntelliJ IDEA: Built-in Git support
- Eclipse: EGit plugin
3. Git Hosting Services
Git hosting services provide a platform for storing repositories and collaborating with others:
- GitHub
- GitLab
- Bitbucket
Troubleshooting Common Git Issues
Even experienced developers encounter Git issues from time to time. Here are some common problems and their solutions:
1. Merge Conflicts
Merge conflicts occur when Git can’t automatically merge changes. To resolve:
- Open the conflicting files and look for the conflict markers (<<<<<<<, =======, >>>>>>>)
- Manually edit the files to resolve the conflicts
- Add the resolved files using
git add
- Complete the merge by running
git commit
2. Undoing Changes
To undo the last commit while keeping the changes:
git reset HEAD~1
To completely discard the last commit and its changes:
git reset --hard HEAD~1
3. Detached HEAD State
If you find yourself in a detached HEAD state, you can create a new branch to save your work:
git checkout -b new-branch-name
4. Large Files in Repository
If you accidentally committed large files, you can remove them from Git history using:
git filter-branch --tree-filter 'rm -f path/to/large/file' HEAD
Conclusion
Git is a powerful tool that can greatly enhance your development workflow. By understanding its basic concepts and commands, you can efficiently manage your code, collaborate with others, and maintain a clean project history. As you continue to use Git, you’ll discover more advanced features and techniques that can further improve your productivity.
Remember, mastering Git takes time and practice. Don’t be afraid to experiment in a test repository, and always keep backups of important projects. With persistence and experience, you’ll soon find Git an indispensable part of your development toolkit.
Happy coding, and may your commits always be clean and your merges conflict-free!