How to Optimize Your Code for Performance: A Comprehensive Guide
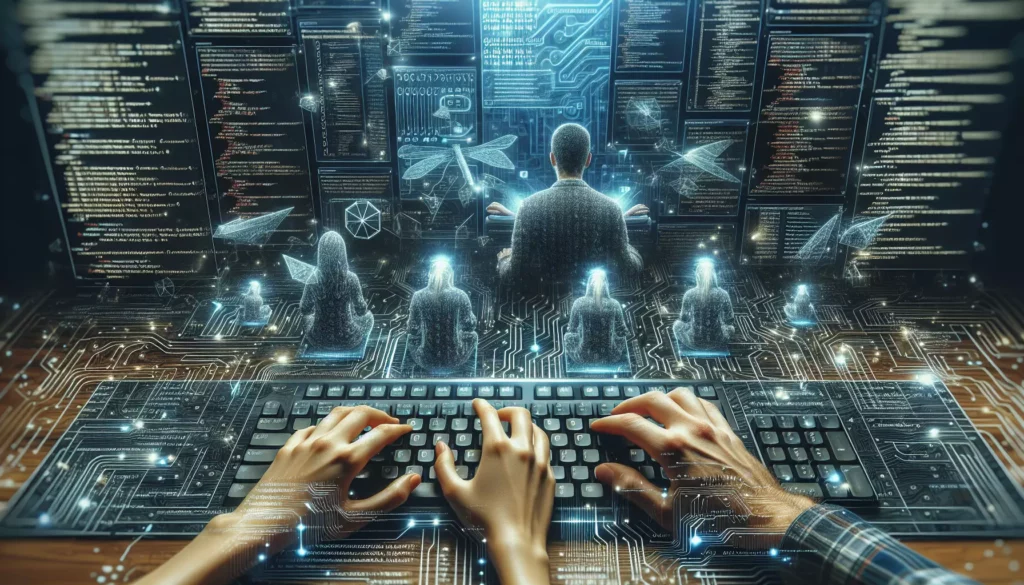
In the world of software development, writing code that works is just the first step. The next crucial phase is optimizing that code for maximum performance. Whether you’re a beginner programmer or an experienced developer preparing for technical interviews at top tech companies, understanding how to optimize your code is an essential skill. This comprehensive guide will walk you through various techniques and best practices to enhance your code’s efficiency and speed.
1. Understanding the Importance of Code Optimization
Before diving into specific optimization techniques, it’s crucial to understand why code optimization matters:
- Improved User Experience: Faster code leads to quicker response times and smoother application performance.
- Resource Efficiency: Optimized code uses fewer system resources, allowing for better scalability.
- Cost Savings: Efficient code can reduce infrastructure costs, especially in cloud environments.
- Environmental Impact: Less computational power means reduced energy consumption.
2. Profiling Your Code
The first step in optimization is identifying where your code needs improvement. Profiling tools help you pinpoint performance bottlenecks:
- Time Profilers: Measure how long each function or method takes to execute.
- Memory Profilers: Track memory usage and identify potential leaks.
- CPU Profilers: Show which parts of your code are consuming the most processing power.
Popular profiling tools include:
- Python: cProfile, memory_profiler
- Java: JProfiler, VisualVM
- JavaScript: Chrome DevTools, Node.js built-in profiler
3. Algorithmic Optimization
Often, the most significant performance gains come from improving your algorithms:
3.1. Time Complexity Analysis
Understand and optimize the time complexity of your algorithms. Aim for lower complexity classes:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time (avoid when possible)
- O(2^n) – Exponential time (avoid)
3.2. Space-Time Tradeoffs
Sometimes, using more memory can significantly speed up your algorithm. For example, caching results or using a hash table for quick lookups.
3.3. Example: Optimizing Fibonacci Sequence Calculation
Consider the following naive recursive implementation of Fibonacci:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
This has a time complexity of O(2^n). We can optimize it using dynamic programming:
def fibonacci_optimized(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
This optimized version has a time complexity of O(n) and space complexity of O(n).
4. Data Structure Optimization
Choosing the right data structure can dramatically improve performance:
4.1. Arrays vs. Linked Lists
- Arrays: Fast random access, slower insertions/deletions
- Linked Lists: Fast insertions/deletions, slower random access
4.2. Hash Tables
Use hash tables (dictionaries in Python, objects in JavaScript) for fast lookups, insertions, and deletions with average-case O(1) time complexity.
4.3. Trees and Graphs
For hierarchical data or complex relationships, consider using tree or graph structures. Binary search trees offer O(log n) search, insertion, and deletion in the average case.
4.4. Example: Optimizing Search
Consider searching for an element in a sorted list:
def linear_search(arr, target):
for i, num in enumerate(arr):
if num == target:
return i
return -1
# Optimized version using binary search
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
The binary search reduces time complexity from O(n) to O(log n).
5. Language-Specific Optimizations
Each programming language has its own set of optimization techniques:
5.1. Python
- Use list comprehensions instead of loops when possible.
- Leverage built-in functions and libraries (e.g.,
map()
,filter()
,numpy
). - Use generators for memory efficiency with large datasets.
5.2. JavaScript
- Avoid global variables to prevent slower scope resolution.
- Use
const
andlet
instead ofvar
for better scoping. - Leverage modern array methods like
map()
,filter()
, andreduce()
.
5.3. Java
- Use StringBuilder for string concatenation in loops.
- Leverage the Stream API for efficient data processing.
- Use primitive types instead of wrapper classes when possible.
6. Memory Management
Efficient memory usage is crucial for performance:
6.1. Garbage Collection
In languages with automatic garbage collection (like Java and Python), understand how it works and write code that helps the garbage collector:
- Nullify references to large objects when no longer needed.
- Be cautious with circular references.
6.2. Memory Leaks
Prevent memory leaks by:
- Closing resources (files, database connections) properly.
- Using weak references for caching.
- Implementing proper cleanup in destructors or finalizers.
6.3. Example: Optimizing Memory Usage in Python
import sys
# Unoptimized
def get_large_list():
return [i for i in range(1000000)]
# Memory-optimized using a generator
def get_large_list_optimized():
return (i for i in range(1000000))
unoptimized = get_large_list()
optimized = get_large_list_optimized()
print(f"Unoptimized size: {sys.getsizeof(unoptimized)} bytes")
print(f"Optimized size: {sys.getsizeof(optimized)} bytes")
The optimized version uses significantly less memory by creating a generator instead of a full list.
7. Concurrency and Parallelism
Leveraging multiple cores or processors can greatly enhance performance:
7.1. Multithreading
Use threads for I/O-bound tasks or to maintain responsiveness in user interfaces.
7.2. Multiprocessing
For CPU-bound tasks, use multiprocessing to utilize multiple cores.
7.3. Asynchronous Programming
Use async/await patterns for efficient I/O operations without blocking.
7.4. Example: Parallel Processing in Python
import multiprocessing
def process_chunk(chunk):
return [x * 2 for x in chunk]
def parallel_processing(data):
num_cores = multiprocessing.cpu_count()
chunk_size = len(data) // num_cores
chunks = [data[i:i + chunk_size] for i in range(0, len(data), chunk_size)]
with multiprocessing.Pool(num_cores) as pool:
results = pool.map(process_chunk, chunks)
return [item for sublist in results for item in sublist]
# Usage
data = list(range(1000000))
result = parallel_processing(data)
This example demonstrates how to split a large task into chunks and process them in parallel using multiple CPU cores.
8. Caching and Memoization
Caching can significantly speed up repetitive computations:
8.1. Function-Level Caching
Use memoization to cache function results based on input parameters.
8.2. Application-Level Caching
Implement caching systems like Redis or Memcached for distributed applications.
8.3. Example: Memoization in Python
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
# Usage
print(fibonacci(100)) # Fast, even for large n
The @lru_cache
decorator automatically caches function results, dramatically speeding up recursive calls.
9. I/O Optimization
I/O operations are often the bottleneck in many applications:
9.1. Buffering
Use buffered I/O to reduce the number of system calls.
9.2. Asynchronous I/O
Implement asynchronous I/O for non-blocking operations, especially in network-heavy applications.
9.3. Batch Processing
Group multiple I/O operations together to reduce overhead.
9.4. Example: Optimized File Reading in Python
def read_large_file(file_path):
with open(file_path, 'r') as file:
while True:
data = file.read(8192) # Read in 8KB chunks
if not data:
break
yield data
# Usage
for chunk in read_large_file('large_file.txt'):
process_chunk(chunk)
This example reads a large file in chunks, reducing memory usage and improving performance for large files.
10. Code-Level Optimizations
Small optimizations can add up to significant improvements:
10.1. Loop Optimization
- Minimize work inside loops.
- Use loop unrolling for small, fixed-size loops.
- Consider using
break
orcontinue
to skip unnecessary iterations.
10.2. Conditional Optimization
- Order conditions by likelihood (most likely first).
- Use switch statements or lookup tables instead of long if-else chains.
10.3. Function Inlining
For small, frequently called functions, consider inlining to reduce function call overhead.
10.4. Example: Loop Optimization in JavaScript
// Unoptimized
function sumArray(arr) {
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
// Optimized
function sumArrayOptimized(arr) {
let sum = 0;
let len = arr.length;
for (let i = 0; i < len; i++) {
sum += arr[i];
}
return sum;
}
The optimized version caches the array length, reducing property lookups in each iteration.
11. Compiler and Interpreter Optimizations
Understand how your language’s compiler or interpreter works to write optimization-friendly code:
11.1. Just-In-Time (JIT) Compilation
For languages with JIT compilation (like JavaScript), write predictable code that allows for effective optimization.
11.2. Ahead-of-Time (AOT) Compilation
For AOT-compiled languages, use compiler optimization flags and understand their effects.
11.3. Example: JavaScript JIT Optimization
// Less optimizable
function add(a, b) {
if (typeof a === 'number' && typeof b === 'number') {
return a + b;
}
return String(a) + String(b);
}
// More JIT-friendly
function addNumbers(a, b) {
return a + b;
}
function concatenateStrings(a, b) {
return String(a) + String(b);
}
The second approach allows the JIT compiler to optimize each function separately, potentially leading to better performance.
12. Testing and Benchmarking
Always measure the impact of your optimizations:
12.1. Unit Testing
Ensure optimizations don’t break existing functionality.
12.2. Performance Testing
Use benchmarking tools to measure performance improvements:
- Python: timeit module
- JavaScript: console.time() and console.timeEnd()
- Java: JMH (Java Microbenchmark Harness)
12.3. Example: Python Benchmarking
import timeit
def original_function():
return sum(range(10000))
def optimized_function():
return sum(range(10000))
original_time = timeit.timeit(original_function, number=1000)
optimized_time = timeit.timeit(optimized_function, number=1000)
print(f"Original: {original_time:.6f} seconds")
print(f"Optimized: {optimized_time:.6f} seconds")
print(f"Improvement: {(original_time - optimized_time) / original_time * 100:.2f}%")
This script benchmarks two functions and compares their performance.
Conclusion
Optimizing code for performance is a crucial skill for any programmer, especially those aiming for positions at top tech companies. By understanding and applying these optimization techniques, you can significantly improve the efficiency and speed of your code. Remember, optimization is an iterative process – always measure, optimize, and test to ensure your changes are having the desired effect.
As you continue your journey in programming and prepare for technical interviews, keep these optimization principles in mind. They will not only help you write better code but also demonstrate your deep understanding of software engineering principles to potential employers.
Practice these techniques regularly, and don’t hesitate to explore more advanced optimization strategies as you grow in your programming career. With dedication and consistent application of these principles, you’ll be well-equipped to tackle performance challenges in any software development role.