Understanding Memory Allocation: Stack vs. Heap
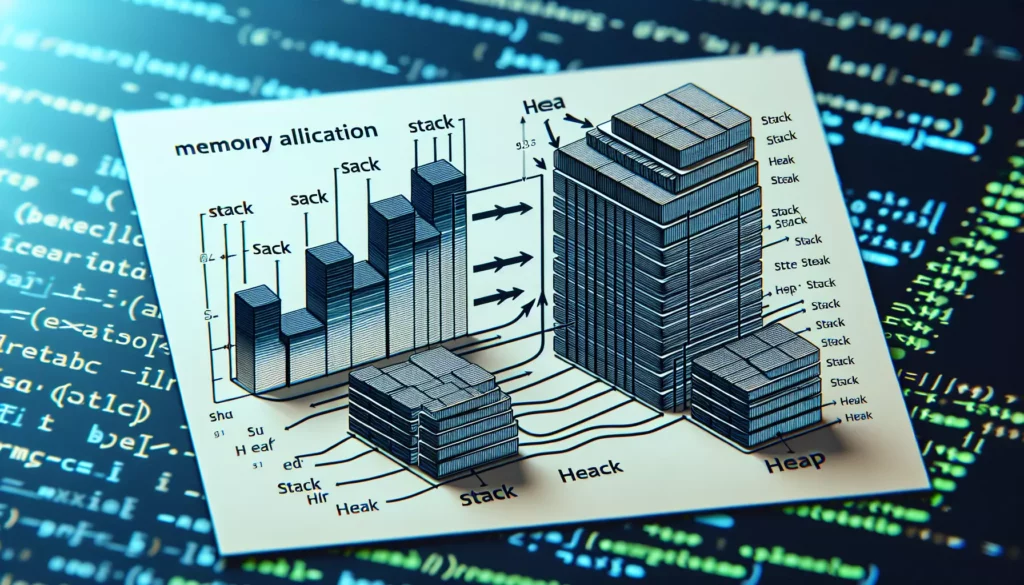
When it comes to programming, understanding how memory is allocated and managed is crucial for writing efficient and bug-free code. Two primary types of memory allocation in most programming languages are stack and heap. In this comprehensive guide, we’ll dive deep into the differences between stack and heap memory allocation, their characteristics, use cases, and how they impact your code’s performance and behavior.
What is Memory Allocation?
Before we delve into the specifics of stack and heap, let’s first understand what memory allocation means. Memory allocation is the process of reserving a portion of computer memory for storing data and instructions used by a program. Proper memory allocation ensures that your program has the necessary resources to run efficiently and prevents issues like memory leaks or crashes.
Stack Memory Allocation
Stack memory allocation is a fast and straightforward way of managing memory in a program. It follows a Last-In-First-Out (LIFO) structure, similar to a stack of plates where you add and remove items from the top.
Characteristics of Stack Memory:
- Fixed size: The stack has a predetermined size, which is typically set by the operating system or the compiler.
- Fast allocation and deallocation: Memory allocation and deallocation on the stack are very quick operations.
- Automatic memory management: The stack is automatically managed by the program, freeing memory when variables go out of scope.
- Limited lifetime: Variables stored on the stack have a limited lifetime, tied to the function or block in which they are declared.
- Contiguous memory: Stack memory is allocated in a contiguous block, making access faster.
When to Use Stack Memory:
- For storing local variables
- For function call management (storing return addresses and parameters)
- For storing small, fixed-size data structures
- When you need fast allocation and deallocation
Example of Stack Memory Allocation:
Here’s a simple example in C++ demonstrating stack memory allocation:
void exampleFunction() {
int x = 5; // Allocated on the stack
double y = 3.14; // Also allocated on the stack
// x and y will be automatically deallocated when the function ends
}
int main() {
exampleFunction();
// x and y are no longer accessible here
return 0;
}
In this example, variables x
and y
are allocated on the stack when exampleFunction()
is called and automatically deallocated when the function returns.
Heap Memory Allocation
Heap memory allocation is a more flexible but slower method of managing memory. It allows for dynamic allocation of memory during runtime.
Characteristics of Heap Memory:
- Dynamic size: The heap can grow or shrink as needed, limited only by the available system memory.
- Slower allocation and deallocation: Memory operations on the heap are generally slower compared to the stack.
- Manual memory management: In languages without garbage collection, the programmer is responsible for freeing heap memory to prevent memory leaks.
- Longer lifetime: Objects on the heap can persist for the entire duration of the program if not explicitly deallocated.
- Non-contiguous memory: Heap memory can be fragmented, potentially leading to slower access times.
When to Use Heap Memory:
- For storing large amounts of data
- When you need to allocate memory dynamically at runtime
- For objects that need to persist beyond the scope of a single function
- When working with complex data structures like trees or graphs
Example of Heap Memory Allocation:
Here’s an example in C++ demonstrating heap memory allocation:
int* createArray(int size) {
int* arr = new int[size]; // Allocated on the heap
return arr;
}
int main() {
int* dynamicArray = createArray(100);
// Use the array...
delete[] dynamicArray; // Manual deallocation required
return 0;
}
In this example, we allocate an array on the heap using new
. The memory persists even after the createArray
function returns, and we need to manually deallocate it using delete[]
to prevent memory leaks.
Key Differences Between Stack and Heap
Aspect | Stack | Heap |
---|---|---|
Size | Fixed | Dynamic |
Allocation Speed | Fast | Slower |
Memory Management | Automatic | Manual (in some languages) |
Lifetime | Function scope | Program lifetime (if not deallocated) |
Fragmentation | No fragmentation | Can be fragmented |
Access Speed | Faster | Slower |
Typical Use | Local variables, function calls | Dynamic data structures, large objects |
Memory Management in Different Programming Languages
The way stack and heap memory are managed can vary significantly between programming languages. Let’s look at how some popular languages handle memory allocation:
C and C++
In C and C++, programmers have direct control over memory allocation. Stack allocation is automatic for local variables, while heap allocation is done manually using functions like malloc()
in C or new
in C++. Developers are responsible for freeing heap memory to prevent leaks.
Java
Java uses automatic memory management with a garbage collector. Objects are allocated on the heap, and primitive types can be on the stack when they are local variables. The garbage collector automatically frees memory that is no longer in use.
Python
Python abstracts memory management from the developer. It uses a private heap to store objects and data structures. Memory management is handled by Python’s memory manager, and a garbage collector is used to free unused memory.
JavaScript
JavaScript, like Python, abstracts memory management. It uses a garbage collector to automatically free memory that is no longer being used. The specifics of stack vs. heap allocation can vary between JavaScript engines.
Common Memory-Related Issues
Understanding stack and heap memory allocation can help you avoid and debug common memory-related issues:
1. Stack Overflow
This occurs when the call stack exceeds its maximum size, often due to excessive recursion or allocating too many large objects on the stack.
Example of code that might cause stack overflow:
void infiniteRecursion() {
int largeArray[1000000]; // Large array on the stack
infiniteRecursion(); // Recursive call
}
int main() {
infiniteRecursion(); // This will likely cause a stack overflow
return 0;
}
2. Memory Leaks
Memory leaks occur when allocated heap memory is not properly freed, leading to gradual consumption of available memory.
Example of code that causes a memory leak:
void memoryLeakExample() {
int* ptr = new int[1000]; // Allocate memory
// ... use ptr ...
// Forgot to delete[] ptr; // Memory leak!
}
int main() {
while(true) {
memoryLeakExample(); // This will continuously leak memory
}
return 0;
}
3. Dangling Pointers
Dangling pointers occur when a pointer references memory that has been deallocated.
Example of a dangling pointer:
int* createAndDelete() {
int* ptr = new int(42);
delete ptr; // Memory is freed
return ptr; // Returning a dangling pointer
}
int main() {
int* danglingPtr = createAndDelete();
cout << *danglingPtr; // Undefined behavior, accessing freed memory
return 0;
}
Best Practices for Memory Management
To write efficient and bug-free code, consider these best practices for memory management:
- Use stack allocation when possible: For small, fixed-size objects with a clear lifetime, prefer stack allocation.
- Be mindful of object lifetimes: Understand the scope and lifetime requirements of your objects to choose the appropriate allocation method.
- Use smart pointers in C++: Smart pointers like
std::unique_ptr
andstd::shared_ptr
can help manage heap-allocated memory and prevent leaks. - Avoid large allocations on the stack: Large objects or arrays should typically be allocated on the heap to prevent stack overflow.
- Free memory properly: In languages without garbage collection, always free allocated memory when it’s no longer needed.
- Use memory profiling tools: Tools like Valgrind can help identify memory leaks and other issues.
- Consider using memory pools: For performance-critical applications, memory pools can provide efficient allocation and deallocation of frequently used objects.
Advanced Topics in Memory Allocation
Memory Alignment
Memory alignment refers to the way data is arranged and accessed in memory. Proper alignment can significantly impact performance and is especially important when working with low-level programming or specific hardware architectures.
Example of memory alignment in C++:
struct AlignedStruct {
char a;
int b;
char c;
};
struct PackedStruct {
char a;
int b;
char c;
} __attribute__((packed));
cout << "Size of AlignedStruct: " << sizeof(AlignedStruct) << endl;
cout << "Size of PackedStruct: " << sizeof(PackedStruct) << endl;
The AlignedStruct
will likely be larger due to padding, while PackedStruct
will be more compact but potentially slower to access.
Memory Fragmentation
Memory fragmentation occurs when free memory is split into small, non-contiguous blocks. This can lead to inefficient memory usage and allocation failures even when there’s technically enough free memory available.
There are two types of fragmentation:
- Internal fragmentation: Occurs when allocated memory blocks are larger than required.
- External fragmentation: Occurs when free memory is split into small, non-contiguous blocks.
To mitigate fragmentation:
- Use memory pools or custom allocators for frequently allocated objects of the same size.
- Implement periodic defragmentation in long-running applications.
- Consider using memory allocation strategies that minimize fragmentation, such as the buddy system or slab allocation.
Garbage Collection Algorithms
For languages with automatic memory management, understanding garbage collection algorithms can help you write more efficient code. Common garbage collection algorithms include:
- Mark-and-Sweep: Marks all reachable objects and then sweeps (frees) unmarked objects.
- Copying Collection: Divides heap into two spaces, copying live objects from one space to another.
- Generational Collection: Separates objects by age, focusing collection efforts on younger objects.
- Incremental Collection: Performs garbage collection in small increments to reduce pause times.
Understanding these algorithms can help you optimize your code for better performance in garbage-collected languages.
Conclusion
Understanding the differences between stack and heap memory allocation is crucial for writing efficient, robust, and bug-free code. The stack provides fast, automatic memory management for local variables and function calls, while the heap offers flexibility for dynamic allocation of larger or longer-lived objects. By choosing the appropriate allocation method and following best practices, you can optimize your program’s performance and avoid common memory-related issues.
As you continue to develop your programming skills, keep in mind that effective memory management is a key aspect of writing high-quality code. Whether you’re working on small scripts or large-scale applications, a solid understanding of memory allocation will serve you well in your journey as a programmer.
Remember to always consider the specific requirements of your project and the characteristics of your chosen programming language when making decisions about memory allocation. With practice and attention to detail, you’ll become proficient at managing memory effectively, leading to more efficient and reliable software.