Understanding Object-Oriented Programming Fundamentals
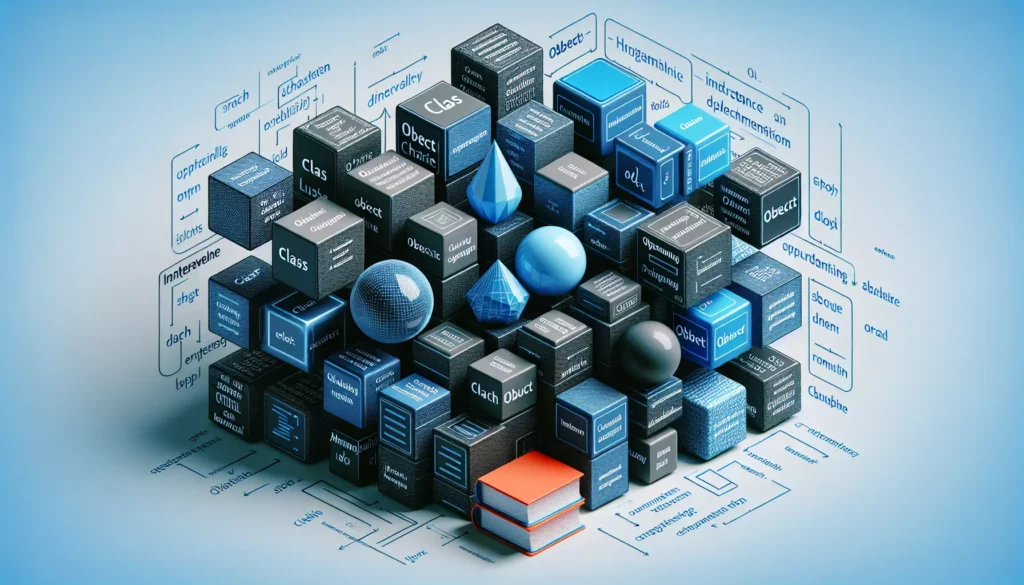
Object-Oriented Programming (OOP) is a fundamental paradigm in modern software development. It’s an essential concept for aspiring programmers to master, especially those aiming to excel in technical interviews at major tech companies. In this comprehensive guide, we’ll explore the core principles of OOP, its benefits, and how to apply these concepts in your coding projects.
What is Object-Oriented Programming?
Object-Oriented Programming is a programming paradigm based on the concept of “objects,” which can contain data in the form of fields (often known as attributes or properties) and code in the form of procedures (often known as methods). OOP is designed to increase flexibility and maintainability in programming, and it’s widely used in large-scale software engineering.
The main idea behind OOP is to bind together the data and the functions that operate on them so that no other part of the code can access this data except that function.
The Four Pillars of OOP
There are four fundamental concepts in object-oriented programming:
1. Encapsulation
Encapsulation is the bundling of data with the methods that operate on that data. It restricts direct access to some of an object’s components, which is a means of preventing accidental interference and misuse of the methods and data.
Here’s a simple example of encapsulation in Python:
class BankAccount:
def __init__(self):
self.__balance = 0 # Private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
return True
return False
def withdraw(self, amount):
if amount > 0 and amount <= self.__balance:
self.__balance -= amount
return True
return False
def get_balance(self):
return self.__balance
# Usage
account = BankAccount()
account.deposit(100)
print(account.get_balance()) # Output: 100
print(account.__balance) # This will raise an AttributeError
In this example, the balance is kept private (denoted by the double underscore), and can only be accessed or modified through the public methods provided.
2. Abstraction
Abstraction is the process of hiding the complex reality while exposing only the necessary parts. It helps in reducing programming complexity and effort.
Here’s an example of abstraction in Python using abstract base classes:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
@abstractmethod
def perimeter(self):
pass
class Rectangle(Shape):
def __init__(self, length, width):
self.length = length
self.width = width
def area(self):
return self.length * self.width
def perimeter(self):
return 2 * (self.length + self.width)
# Usage
rect = Rectangle(5, 3)
print(rect.area()) # Output: 15
print(rect.perimeter()) # Output: 16
In this example, Shape is an abstract base class that defines a common interface for all shapes, without implementing the methods. Rectangle is a concrete class that implements the abstract methods.
3. Inheritance
Inheritance is a mechanism where you can to derive a class from another class for a hierarchy of classes that share a set of attributes and methods.
Here’s an example of inheritance in Python:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
# Usage
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Output: Buddy says Woof!
print(cat.speak()) # Output: Whiskers says Meow!
In this example, Dog and Cat inherit from Animal, and override the speak method to provide their own implementations.
4. Polymorphism
Polymorphism is the provision of a single interface to entities of different types. A polymorphic type is one whose operations can also be applied to values of some other type, or types.
Here’s an example of polymorphism in Python:
class Shape:
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius ** 2
def print_area(shape):
print(f"The area is: {shape.area()}")
# Usage
rect = Rectangle(5, 3)
circ = Circle(4)
print_area(rect) # Output: The area is: 15
print_area(circ) # Output: The area is: 50.24
In this example, both Rectangle and Circle implement the area method, but in different ways. The print_area function can work with any Shape object, demonstrating polymorphism.
Benefits of Object-Oriented Programming
OOP offers several advantages that make it a popular choice for software development:
- Modularity: Encapsulation enables objects to be self-contained, making troubleshooting and collaborative development easier.
- Reusability: Through inheritance, you can reuse code from existing classes when creating new classes, saving time and effort.
- Flexibility and extensibility: Polymorphism allows for the addition of new classes without changing existing code, making programs more flexible and scalable.
- Data hiding: The encapsulation mechanism ensures data security by keeping the data and code safe within the object.
- Easy troubleshooting: When an issue occurs in an OOP program, you can usually isolate the cause within a particular object.
Applying OOP Concepts in Real-World Scenarios
Understanding OOP concepts is crucial, but applying them effectively in real-world scenarios is where the true power of OOP shines. Let’s explore some practical applications:
1. Game Development
Game development is an excellent example of where OOP concepts are extensively used. Consider a simple game with different types of characters:
class Character:
def __init__(self, name, health, damage):
self.name = name
self.health = health
self.damage = damage
def attack(self, target):
target.health -= self.damage
print(f"{self.name} attacks {target.name} for {self.damage} damage!")
class Warrior(Character):
def __init__(self, name):
super().__init__(name, health=100, damage=10)
def shield_block(self):
print(f"{self.name} blocks the next attack!")
class Mage(Character):
def __init__(self, name):
super().__init__(name, health=80, damage=15)
def cast_spell(self, target):
spell_damage = self.damage * 1.5
target.health -= spell_damage
print(f"{self.name} casts a spell on {target.name} for {spell_damage} damage!")
# Game simulation
warrior = Warrior("Aragorn")
mage = Mage("Gandalf")
warrior.attack(mage)
mage.cast_spell(warrior)
warrior.shield_block()
print(f"{warrior.name}'s health: {warrior.health}")
print(f"{mage.name}'s health: {mage.health}")
In this example, we use inheritance to create specific character types (Warrior and Mage) from a base Character class. Each character type has its unique abilities, demonstrating polymorphism.
2. E-commerce System
OOP is also widely used in building e-commerce systems. Here’s a simplified example:
class Product:
def __init__(self, name, price, stock):
self.name = name
self.price = price
self.stock = stock
def display_info(self):
return f"{self.name}: ${self.price} (Stock: {self.stock})"
class ShoppingCart:
def __init__(self):
self.items = {}
def add_item(self, product, quantity):
if product.stock >= quantity:
if product in self.items:
self.items[product] += quantity
else:
self.items[product] = quantity
product.stock -= quantity
print(f"Added {quantity} {product.name}(s) to cart.")
else:
print(f"Sorry, only {product.stock} {product.name}(s) available.")
def remove_item(self, product, quantity):
if product in self.items:
if self.items[product] >= quantity:
self.items[product] -= quantity
product.stock += quantity
print(f"Removed {quantity} {product.name}(s) from cart.")
if self.items[product] == 0:
del self.items[product]
else:
print(f"Error: Trying to remove more items than in cart.")
else:
print(f"Error: {product.name} not in cart.")
def display_cart(self):
if not self.items:
print("Your cart is empty.")
else:
total = 0
for product, quantity in self.items.items():
subtotal = product.price * quantity
total += subtotal
print(f"{product.name}: {quantity} x ${product.price} = ${subtotal}")
print(f"Total: ${total}")
# Usage
laptop = Product("Laptop", 999.99, 10)
phone = Product("Smartphone", 499.99, 20)
cart = ShoppingCart()
cart.add_item(laptop, 2)
cart.add_item(phone, 1)
cart.display_cart()
cart.remove_item(laptop, 1)
cart.display_cart()
This example demonstrates encapsulation (Product and ShoppingCart classes encapsulate their data and behaviors) and abstraction (the internal workings of the ShoppingCart are hidden from the user).
OOP Design Patterns
Design patterns are typical solutions to common problems in software design. They are like pre-made blueprints that you can customize to solve a recurring design problem in your code. Let’s look at a few popular OOP design patterns:
1. Singleton Pattern
The Singleton pattern ensures a class has only one instance and provides a global point of access to it. This is useful when exactly one object is needed to coordinate actions across the system.
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
# Usage
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
2. Factory Method Pattern
The Factory Method pattern provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created.
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
class AnimalFactory:
def create_animal(self, animal_type):
if animal_type == "Dog":
return Dog()
elif animal_type == "Cat":
return Cat()
else:
raise ValueError("Unknown animal type")
# Usage
factory = AnimalFactory()
dog = factory.create_animal("Dog")
cat = factory.create_animal("Cat")
print(dog.speak()) # Output: Woof!
print(cat.speak()) # Output: Meow!
3. Observer Pattern
The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
class Subject:
def __init__(self):
self._observers = []
self._state = None
def attach(self, observer):
self._observers.append(observer)
def detach(self, observer):
self._observers.remove(observer)
def notify(self):
for observer in self._observers:
observer.update(self._state)
def set_state(self, state):
self._state = state
self.notify()
class Observer(ABC):
@abstractmethod
def update(self, state):
pass
class ConcreteObserver(Observer):
def update(self, state):
print(f"Observer: My new state is {state}")
# Usage
subject = Subject()
observer1 = ConcreteObserver()
observer2 = ConcreteObserver()
subject.attach(observer1)
subject.attach(observer2)
subject.set_state(123) # This will update all observers
Best Practices in OOP
To make the most of OOP, consider following these best practices:
- Keep it DRY (Don’t Repeat Yourself): Avoid duplicating code. Use inheritance and composition to reuse code effectively.
- SOLID Principles: Follow the SOLID principles (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion) for creating more maintainable and scalable software.
- Favor Composition Over Inheritance: While inheritance is powerful, it can lead to inflexible designs. Composition often provides more flexibility.
- Program to an Interface, Not an Implementation: Depend on abstractions rather than concrete classes to make your code more flexible and easier to change.
- Keep Classes Focused: Each class should have a single, well-defined purpose. If a class is trying to do too much, it might be time to split it into smaller, more focused classes.
- Use Descriptive Names: Choose clear, descriptive names for your classes, methods, and variables. This makes your code self-documenting and easier to understand.
- Encapsulate What Varies: Identify the aspects of your application that vary and separate them from what stays the same.
Conclusion
Object-Oriented Programming is a powerful paradigm that, when used correctly, can lead to more organized, flexible, and maintainable code. By understanding and applying the four pillars of OOP – encapsulation, abstraction, inheritance, and polymorphism – you can create robust and scalable software systems.
As you continue your journey in programming, particularly if you’re preparing for technical interviews at major tech companies, mastering OOP concepts will be crucial. Practice implementing these concepts in your projects, explore design patterns, and always strive to write clean, efficient code.
Remember, becoming proficient in OOP is not just about understanding the theory, but also about applying these concepts practically. As you gain more experience, you’ll develop an intuition for when and how to best use OOP principles to solve complex programming challenges.
Keep coding, keep learning, and embrace the object-oriented mindset in your programming journey!