How to Debug Your Code: Techniques for Finding and Fixing Errors
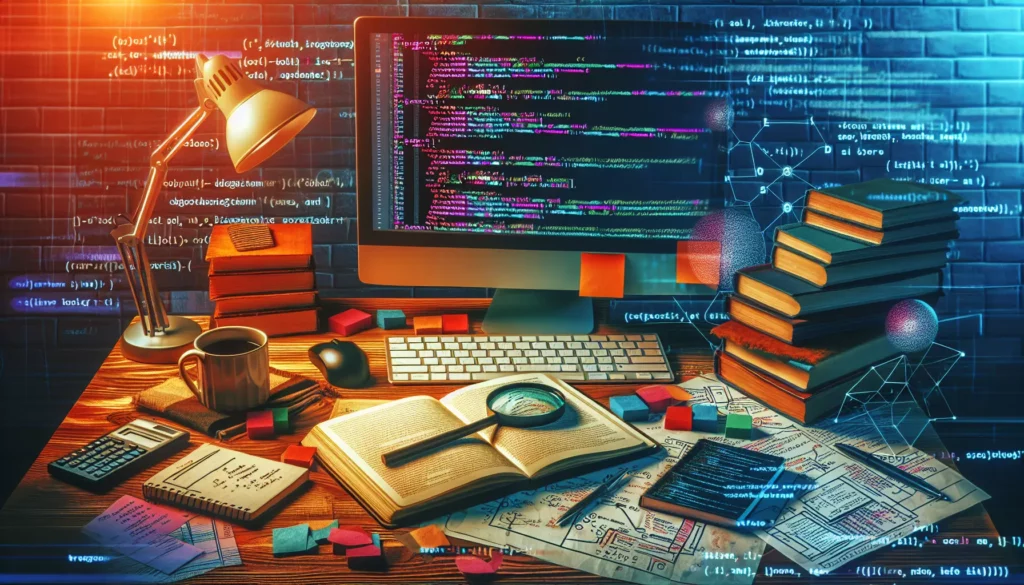
Debugging is an essential skill for every programmer, regardless of their experience level. It’s the process of identifying, isolating, and fixing errors or bugs in your code. As you progress in your coding journey, you’ll find that debugging becomes an integral part of your development process. In this comprehensive guide, we’ll explore various techniques and strategies to help you become a more efficient debugger, ultimately improving your coding skills and problem-solving abilities.
1. Understanding the Importance of Debugging
Before we dive into specific techniques, it’s crucial to understand why debugging is so important:
- Ensures code functionality: Debugging helps ensure that your code works as intended and produces the expected output.
- Improves code quality: Through debugging, you can identify and fix potential issues, leading to cleaner and more efficient code.
- Enhances problem-solving skills: Regular debugging practice sharpens your analytical and logical thinking abilities.
- Saves time in the long run: While debugging may seem time-consuming initially, it saves you from potential issues and bugs in the future.
- Prepares you for technical interviews: Many technical interviews include debugging tasks, so honing this skill is crucial for your career advancement.
2. Common Types of Errors
To effectively debug your code, it’s important to understand the different types of errors you might encounter:
2.1. Syntax Errors
Syntax errors occur when your code violates the rules of the programming language. These are usually caught by the compiler or interpreter and prevent your code from running. Examples include:
- Missing parentheses, brackets, or semicolons
- Misspelled keywords
- Incorrect indentation (in languages like Python)
2.2. Runtime Errors
Runtime errors occur when your code is syntactically correct but encounters an issue during execution. These can cause your program to crash or produce unexpected results. Common runtime errors include:
- Division by zero
- Accessing an array index out of bounds
- Null pointer exceptions
2.3. Logical Errors
Logical errors are the trickiest to identify because they don’t cause your program to crash or produce error messages. Instead, they result in incorrect output or unexpected behavior. These errors occur when your code doesn’t accurately implement the intended logic. Examples include:
- Using the wrong comparison operator (e.g., < instead of >)
- Incorrect algorithm implementation
- Off-by-one errors in loops
3. Essential Debugging Techniques
Now that we understand the types of errors, let’s explore some essential debugging techniques:
3.1. Print Statements
One of the simplest yet effective debugging techniques is using print statements to track the flow of your program and inspect variable values at different points. While this method may seem basic, it can be incredibly useful for quick debugging, especially in smaller programs.
Example in Python:
def calculate_sum(a, b):
print(f"Calculating sum of {a} and {b}")
result = a + b
print(f"Result: {result}")
return result
total = calculate_sum(5, 3)
print(f"Total: {total}")
3.2. Using a Debugger
Most modern Integrated Development Environments (IDEs) come with built-in debuggers. These powerful tools allow you to:
- Set breakpoints in your code
- Step through your code line by line
- Inspect variable values at different points of execution
- Evaluate expressions in real-time
Learning to use a debugger effectively can significantly speed up your debugging process and provide deeper insights into your code’s behavior.
3.3. Rubber Duck Debugging
This technique involves explaining your code, line by line, to an inanimate object (traditionally a rubber duck). The act of verbalizing your code often helps you spot errors or inconsistencies in your logic. This method is particularly useful for identifying logical errors.
3.4. Code Review
Having another programmer review your code can be invaluable in catching errors you might have missed. Fresh eyes can often spot issues that you’ve become blind to after staring at your code for hours.
3.5. Divide and Conquer
When dealing with complex issues, try to isolate the problem by commenting out sections of code or creating simplified test cases. This approach can help you narrow down the source of the error more quickly.
4. Advanced Debugging Strategies
As you become more proficient in coding, you’ll encounter more complex debugging scenarios. Here are some advanced strategies to tackle these challenges:
4.1. Logging
For larger applications, using a logging framework can be more efficient than print statements. Logging allows you to:
- Set different levels of logging (e.g., DEBUG, INFO, WARNING, ERROR)
- Log to files for later analysis
- Include timestamps and other metadata with your log messages
Example using Python’s logging module:
import logging
logging.basicConfig(level=logging.DEBUG, filename='app.log', filemode='w',
format='%(name)s - %(levelname)s - %(message)s')
def complex_function(x, y):
logging.debug(f"Entering complex_function with x={x}, y={y}")
# Function logic here
result = x * y
logging.info(f"complex_function result: {result}")
return result
complex_function(5, 3)
4.2. Unit Testing
Writing unit tests for your code can help you catch bugs early and ensure that your functions work as expected. Unit tests can also serve as documentation for how your code should behave.
Example using Python’s unittest module:
import unittest
def add_numbers(a, b):
return a + b
class TestAddNumbers(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add_numbers(-1, -1), -2)
def test_add_zero(self):
self.assertEqual(add_numbers(5, 0), 5)
if __name__ == '__main__':
unittest.main()
4.3. Debugging Multithreaded Code
Debugging multithreaded applications can be challenging due to race conditions and synchronization issues. Some strategies for debugging multithreaded code include:
- Using thread-safe logging
- Employing specialized debugging tools for concurrent programs
- Simplifying the code to run sequentially when possible
- Using synchronization primitives to control thread execution
4.4. Remote Debugging
When working with applications deployed on remote servers or in cloud environments, remote debugging becomes essential. Many IDEs and debugging tools support remote debugging, allowing you to step through code running on a different machine.
4.5. Performance Profiling
Sometimes, the bug you’re looking for isn’t a crash or incorrect output, but a performance issue. Profiling tools can help you identify bottlenecks in your code by measuring execution time and resource usage.
5. Best Practices for Effective Debugging
To become a more efficient debugger, consider adopting these best practices:
5.1. Reproduce the Bug Consistently
Before diving into debugging, ensure you can reproduce the bug consistently. This might involve creating a minimal test case that demonstrates the issue.
5.2. Read the Error Message Carefully
Error messages often contain valuable information about what went wrong and where. Take the time to read and understand the error message before starting your debugging process.
5.3. Use Version Control
Version control systems like Git allow you to track changes in your code over time. This can be invaluable when trying to identify when a bug was introduced or reverting to a working version of your code.
5.4. Keep Your Code Clean and Well-Organized
Well-structured code is easier to debug. Follow coding best practices, use meaningful variable names, and keep your functions small and focused.
5.5. Learn to Read Stack Traces
Stack traces provide a wealth of information about where an error occurred and the sequence of function calls that led to it. Learning to interpret stack traces can significantly speed up your debugging process.
5.6. Use Assertions
Assertions can help you catch bugs early by verifying that certain conditions are met during program execution. They’re especially useful for catching logical errors.
Example in Python:
def calculate_average(numbers):
assert len(numbers) > 0, "List cannot be empty"
return sum(numbers) / len(numbers)
# This will raise an AssertionError
calculate_average([])
5.7. Document Your Debugging Process
Keep notes on the bugs you encounter and how you solved them. This documentation can be invaluable when facing similar issues in the future or when collaborating with team members.
6. Debugging Tools and Resources
Familiarize yourself with these popular debugging tools and resources:
6.1. IDE Debuggers
- PyCharm Debugger (for Python)
- Visual Studio Debugger (for C#, C++)
- IntelliJ IDEA Debugger (for Java)
- Chrome DevTools (for JavaScript in web applications)
6.2. Standalone Debuggers
- GDB (GNU Debugger) for C, C++, and Fortran
- LLDB for macOS and iOS development
- WinDbg for Windows applications
6.3. Profiling Tools
- cProfile (for Python)
- Valgrind (for memory debugging, memory leak detection, and profiling)
- Java VisualVM (for Java applications)
6.4. Online Resources
- Stack Overflow: A vast community of developers sharing knowledge and solutions
- GitHub Issues: Check if others have reported similar issues in open-source projects
- Language-specific documentation and forums
7. Debugging in Different Programming Paradigms
Different programming paradigms may require slightly different debugging approaches:
7.1. Object-Oriented Programming (OOP)
When debugging OOP code, pay attention to:
- Object state and how it changes over time
- Inheritance and method overriding
- Proper encapsulation and access control
7.2. Functional Programming
In functional programming, focus on:
- Ensuring functions are pure (no side effects)
- Proper handling of immutable data structures
- Correct implementation of recursion
7.3. Asynchronous Programming
Debugging asynchronous code can be challenging. Pay attention to:
- Proper use of async/await or Promises
- Handling of race conditions
- Correct error propagation in asynchronous operations
8. Debugging in a Team Environment
When working in a team, consider these additional debugging strategies:
8.1. Collaborative Debugging
Pair programming or group debugging sessions can be effective for tackling complex issues. Multiple perspectives can lead to faster problem-solving.
8.2. Using Issue Tracking Systems
Tools like JIRA or GitHub Issues can help teams track and manage bugs systematically. Use these systems to document bug reports, steps to reproduce, and solutions.
8.3. Code Review as a Debugging Tool
Implement a code review process in your team. This not only catches potential bugs before they make it to production but also serves as a learning opportunity for all team members.
9. Conclusion
Debugging is an art as much as it is a science. It requires patience, analytical thinking, and a systematic approach. By mastering the techniques and strategies outlined in this guide, you’ll be well-equipped to tackle even the most challenging bugs in your code.
Remember that becoming an expert debugger takes time and practice. Don’t get discouraged when facing difficult bugs – each debugging session is an opportunity to learn and improve your skills. As you continue to develop your debugging abilities, you’ll find that you’re not only fixing errors more efficiently but also writing better, more robust code from the start.
Keep exploring new tools and techniques, stay curious about how things work under the hood, and don’t hesitate to seek help when you’re stuck. With persistence and the right approach, you’ll be able to conquer any bug that comes your way and become a more confident, skilled programmer in the process.