Understanding Recursion: When and How to Use It
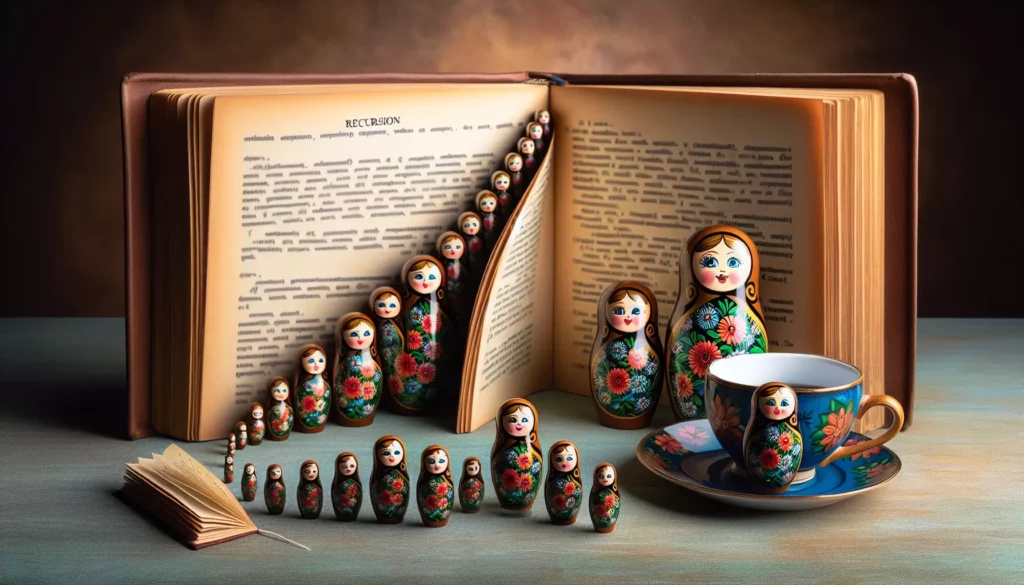
Recursion is a powerful programming concept that can solve complex problems elegantly and efficiently. However, it can also be a source of confusion for many programmers, especially those new to the concept. In this comprehensive guide, we’ll dive deep into the world of recursion, exploring what it is, when to use it, and how to implement it effectively in your code.
What is Recursion?
Recursion is a programming technique where a function calls itself to solve a problem. It’s based on the principle of breaking down a complex problem into smaller, more manageable subproblems. Each recursive call works on a smaller instance of the original problem until a base case is reached, at which point the function stops calling itself and starts returning results back up the chain of calls.
The key components of a recursive function are:
- Base case: The condition that stops the recursion
- Recursive case: The part where the function calls itself with a modified input
When to Use Recursion
Recursion is particularly useful in scenarios where a problem can be naturally divided into smaller, similar subproblems. Here are some situations where recursion shines:
1. Tree-like Data Structures
Recursion is excellent for traversing and manipulating tree-like data structures, such as binary trees, file systems, or HTML DOM. The recursive nature of these structures aligns well with recursive algorithms.
2. Divide and Conquer Algorithms
Many divide and conquer algorithms, like quicksort and merge sort, use recursion to break down the problem into smaller parts and then combine the results.
3. Backtracking Problems
Recursion is often used in backtracking algorithms, where you need to explore all possible solutions and backtrack when a solution is not valid.
4. Mathematical Problems
Certain mathematical concepts, like factorial calculations or Fibonacci sequences, have natural recursive definitions that translate well into code.
5. Dynamic Programming
While dynamic programming often uses iteration for efficiency, the initial recursive solution can provide a clear understanding of the problem before optimization.
How to Implement Recursion
Let’s look at some examples to understand how to implement recursion effectively:
Example 1: Factorial Calculation
The factorial of a non-negative integer n, denoted as n!, is the product of all positive integers less than or equal to n. Here’s a recursive implementation:
def factorial(n):
# Base case
if n == 0 or n == 1:
return 1
# Recursive case
else:
return n * factorial(n - 1)
# Example usage
print(factorial(5)) # Output: 120
In this example, the base case is when n is 0 or 1, as the factorial of these numbers is 1. For any other number, we multiply n with the factorial of (n-1), which is our recursive case.
Example 2: Binary Tree Traversal
Recursion is particularly useful for traversing tree-like structures. Here’s an example of an in-order traversal of a binary tree:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def inorder_traversal(root):
if root is None:
return []
return (inorder_traversal(root.left) +
[root.value] +
inorder_traversal(root.right))
# Example usage
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print(inorder_traversal(root)) # Output: [4, 2, 5, 1, 3]
In this example, we recursively traverse the left subtree, process the current node, and then recursively traverse the right subtree. The base case is when we reach a null node.
Best Practices for Using Recursion
While recursion can be powerful, it’s important to use it correctly to avoid common pitfalls:
1. Always Define a Base Case
Ensure that your recursive function has a clearly defined base case that will eventually be reached. Without this, your function will continue calling itself indefinitely, resulting in a stack overflow error.
2. Ensure Progress Towards the Base Case
Make sure that each recursive call brings you closer to the base case. This usually involves modifying the input in some way with each call.
3. Be Mindful of Stack Space
Recursive calls consume stack space. For very deep recursions, you might run out of stack space, causing a stack overflow. In such cases, consider using tail recursion (if your language supports it) or iterative solutions.
4. Avoid Redundant Calculations
In some recursive algorithms, you might end up calculating the same subproblems multiple times. Use techniques like memoization to store and reuse results of subproblems.
5. Consider Readability
While recursion can lead to elegant solutions, sometimes an iterative approach might be more readable or intuitive. Choose the approach that makes your code easier to understand and maintain.
Common Recursive Algorithms
Let’s explore some common algorithms that are often implemented using recursion:
1. Fibonacci Sequence
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. Here’s a recursive implementation:
def fibonacci(n):
if n
Note that while this implementation is simple and intuitive, it’s not efficient for large values of n due to redundant calculations. For better performance, you’d typically use dynamic programming or iteration.
2. Binary Search
Binary search is an efficient algorithm for searching a sorted array. Here’s a recursive implementation:
def binary_search(arr, target, low, high):
if high >= low:
mid = (high + low) // 2
if arr[mid] == target:
return mid
elif arr[mid] > target:
return binary_search(arr, target, low, mid - 1)
else:
return binary_search(arr, target, mid + 1, high)
else:
return -1
# Example usage
arr = [2, 3, 4, 10, 40]
target = 10
result = binary_search(arr, target, 0, len(arr)-1)
print(f"Element is present at index {result}") # Output: Element is present at index 3
This recursive implementation divides the search space in half with each recursive call, efficiently narrowing down the location of the target element.
3. Quicksort
Quicksort is a divide-and-conquer sorting algorithm that uses recursion to sort an array. Here’s a basic implementation:
def quicksort(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
# Example usage
arr = [3, 6, 8, 10, 1, 2, 1]
print(quicksort(arr)) # Output: [1, 1, 2, 3, 6, 8, 10]
This implementation recursively sorts the subarrays to the left and right of the pivot element.
Recursion vs. Iteration
While recursion can lead to elegant and intuitive solutions for certain problems, it’s not always the best choice. Let’s compare recursion with iteration:
Advantages of Recursion:
- Can lead to cleaner, more readable code for certain problems
- Natural fit for problems with recursive structures (like trees)
- Can be easier to prove correct using mathematical induction
Disadvantages of Recursion:
- Can be less efficient due to function call overhead
- Risk of stack overflow for deep recursions
- May be harder to understand for those unfamiliar with the concept
When to Choose Recursion or Iteration:
- Use recursion when the problem has a natural recursive structure and the depth of recursion is manageable
- Use iteration when you need to optimize for performance or when the recursive solution would be too deep
- Consider the readability and maintainability of your code when making the choice
Advanced Recursion Techniques
As you become more comfortable with basic recursion, you can explore more advanced techniques:
1. Tail Recursion
Tail recursion is a special form of recursion where the recursive call is the last operation in the function. Some programming languages and compilers can optimize tail-recursive functions to be as efficient as loops.
def factorial_tail(n, accumulator=1):
if n == 0:
return accumulator
else:
return factorial_tail(n - 1, n * accumulator)
# Example usage
print(factorial_tail(5)) # Output: 120
2. Mutual Recursion
Mutual recursion involves two or more functions that call each other recursively. This can be useful for problems that have a natural back-and-forth structure.
def is_even(n):
if n == 0:
return True
else:
return is_odd(n - 1)
def is_odd(n):
if n == 0:
return False
else:
return is_even(n - 1)
# Example usage
print(is_even(4)) # Output: True
print(is_odd(4)) # Output: False
3. Memoization
Memoization is a technique used to optimize recursive functions by storing the results of expensive function calls and returning the cached result when the same inputs occur again. This is particularly useful for problems with overlapping subproblems, like the Fibonacci sequence.
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
# Example usage
print(fibonacci_memo(100)) # Output: 354224848179261915075
Common Pitfalls and How to Avoid Them
While recursion can be powerful, it’s easy to fall into certain traps. Here are some common pitfalls and how to avoid them:
1. Infinite Recursion
This occurs when the base case is never reached, causing the function to call itself indefinitely.
How to avoid: Always ensure that your recursive calls are moving towards the base case. Double-check your base case conditions.
2. Stack Overflow
This happens when the recursion depth is too great for the available stack space.
How to avoid: Consider using tail recursion if your language supports it, or switch to an iterative solution for very deep recursions.
3. Excessive Memory Usage
Recursive solutions can sometimes use more memory than necessary, especially if they’re not tail-recursive.
How to avoid: Use techniques like memoization to avoid redundant calculations, or consider an iterative approach if memory usage is a concern.
4. Poor Performance
Naive recursive solutions to problems like Fibonacci can have exponential time complexity.
How to avoid: Use memoization or dynamic programming to optimize recursive solutions with overlapping subproblems.
Conclusion
Recursion is a powerful tool in a programmer’s toolkit. When used appropriately, it can lead to elegant, intuitive solutions to complex problems. However, it’s important to understand both its strengths and limitations. By mastering recursion, you’ll be better equipped to tackle a wide range of programming challenges, from tree traversals to divide-and-conquer algorithms.
Remember, the key to effective use of recursion lies in identifying problems with a natural recursive structure, ensuring a clear base case, and being mindful of performance implications. With practice, you’ll develop an intuition for when and how to apply recursive techniques to solve problems efficiently and elegantly.
As you continue your journey in programming, don’t shy away from recursive solutions, but also don’t force them where an iterative approach might be more appropriate. The ability to choose the right tool for the job is a hallmark of an experienced programmer.
Happy coding, and may your recursive adventures always reach their base case!