Demystifying Functions: How to Create and Use Them Effectively
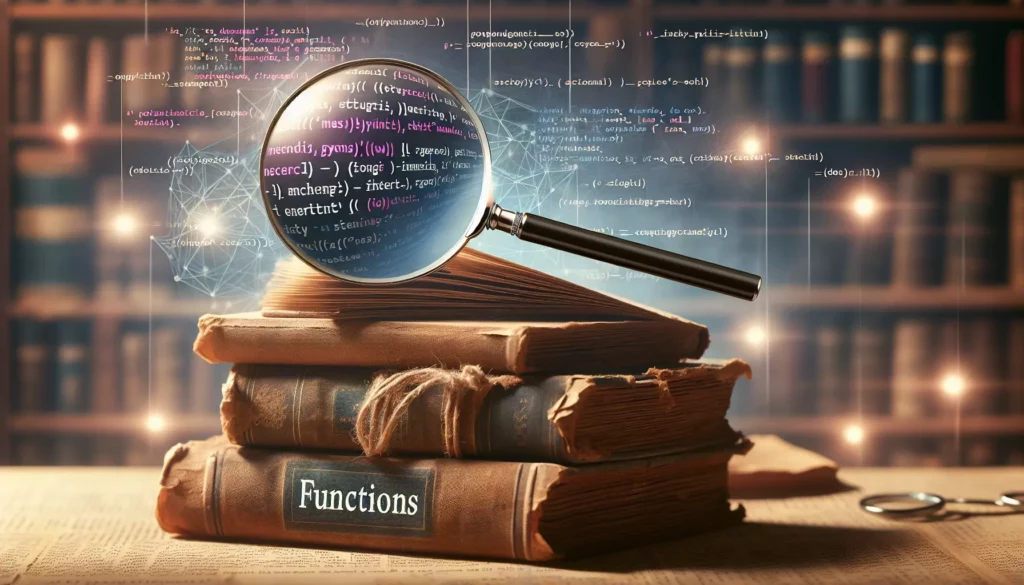
Functions are the building blocks of modern programming. They allow us to organize code, promote reusability, and make our programs more manageable. Whether you’re a beginner just starting your coding journey or an experienced developer looking to refine your skills, understanding how to create and use functions effectively is crucial. In this comprehensive guide, we’ll demystify functions and explore how to harness their power in your code.
Table of Contents
- What Are Functions?
- Why Use Functions?
- Anatomy of a Function
- Creating Functions
- Function Parameters
- Return Values
- Function Scope
- Anonymous Functions and Lambda Expressions
- Higher-Order Functions
- Recursion
- Best Practices for Writing Functions
- Common Pitfalls and How to Avoid Them
- Real-World Examples
- Conclusion
1. What Are Functions?
At its core, a function is a reusable block of code that performs a specific task. It’s like a mini-program within your main program. Functions can take inputs (called parameters or arguments), process them, and return outputs. They serve as the fundamental unit of code organization in most programming languages.
Here’s a simple example of a function in Python:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice")) # Output: Hello, Alice!
In this example, greet
is a function that takes a name
as input and returns a greeting message.
2. Why Use Functions?
Functions offer several benefits that make them indispensable in programming:
- Code Reusability: Write once, use many times. Functions allow you to avoid repeating code.
- Modularity: Break down complex problems into smaller, manageable pieces.
- Abstraction: Hide complex implementations behind simple interfaces.
- Readability: Well-named functions make code self-documenting and easier to understand.
- Maintainability: Isolate changes to specific functions, making updates and bug fixes easier.
- Testing: Functions provide natural units for unit testing.
3. Anatomy of a Function
Let’s break down the components of a typical function:
def function_name(parameter1, parameter2):
"""
Docstring: A brief description of what the function does.
"""
# Function body
# Code that performs the task
result = parameter1 + parameter2
return result # Return statement (optional)
- Function Declaration: Starts with
def
in Python (other languages may use different keywords). - Function Name: A descriptive name for the function.
- Parameters: Input values the function can work with (optional).
- Docstring: A string that describes what the function does (optional but recommended).
- Function Body: The actual code that performs the task.
- Return Statement: Specifies the output of the function (optional).
4. Creating Functions
Creating functions is a fundamental skill in programming. Let’s explore how to create functions in different programming languages:
Python
def calculate_area(length, width):
"""Calculate the area of a rectangle."""
return length * width
area = calculate_area(5, 3)
print(f"The area is: {area}") # Output: The area is: 15
JavaScript
function calculateArea(length, width) {
// Calculate the area of a rectangle
return length * width;
}
const area = calculateArea(5, 3);
console.log(`The area is: ${area}`); // Output: The area is: 15
Java
public class AreaCalculator {
public static int calculateArea(int length, int width) {
// Calculate the area of a rectangle
return length * width;
}
public static void main(String[] args) {
int area = calculateArea(5, 3);
System.out.println("The area is: " + area); // Output: The area is: 15
}
}
As you can see, while the syntax may differ, the concept of creating functions remains consistent across languages.
5. Function Parameters
Parameters are the inputs that a function can accept. They allow functions to be more flexible and reusable. Let’s explore different types of parameters:
Required Parameters
def greet(name):
return f"Hello, {name}!"
print(greet("Alice")) # Output: Hello, Alice!
print(greet()) # Error: missing required argument
Optional Parameters (Default Arguments)
def greet(name="Guest"):
return f"Hello, {name}!"
print(greet("Alice")) # Output: Hello, Alice!
print(greet()) # Output: Hello, Guest!
Variable Number of Arguments
def sum_all(*args):
return sum(args)
print(sum_all(1, 2, 3)) # Output: 6
print(sum_all(1, 2, 3, 4, 5)) # Output: 15
Keyword Arguments
def create_profile(**kwargs):
return kwargs
profile = create_profile(name="Alice", age=30, city="New York")
print(profile) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York'}
Understanding these different parameter types allows you to create more flexible and powerful functions.
6. Return Values
The return
statement is used to specify the output of a function. It’s important to understand how return values work:
def divide(a, b):
if b == 0:
return "Error: Division by zero"
return a / b
result = divide(10, 2)
print(result) # Output: 5.0
result = divide(10, 0)
print(result) # Output: Error: Division by zero
Functions can return multiple values in some languages:
def min_max(numbers):
return min(numbers), max(numbers)
minimum, maximum = min_max([1, 2, 3, 4, 5])
print(f"Min: {minimum}, Max: {maximum}") # Output: Min: 1, Max: 5
7. Function Scope
Function scope refers to the visibility and lifetime of variables within a function. Understanding scope is crucial for writing clean and bug-free code:
x = 10 # Global variable
def modify_global():
global x
x = 20
def create_local():
x = 30 # Local variable
print(f"Local x: {x}")
print(f"Global x: {x}") # Output: Global x: 10
modify_global()
print(f"Modified global x: {x}") # Output: Modified global x: 20
create_local() # Output: Local x: 30
print(f"Global x after create_local: {x}") # Output: Global x after create_local: 20
It’s generally a good practice to avoid modifying global variables within functions, as it can lead to unexpected behavior and make code harder to debug.
8. Anonymous Functions and Lambda Expressions
Anonymous functions, often called lambda functions in some languages, are small, unnamed functions that can be defined inline. They’re useful for short, one-time operations:
Python Lambda
square = lambda x: x ** 2
print(square(5)) # Output: 25
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x ** 2, numbers))
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
JavaScript Arrow Functions
const square = x => x ** 2;
console.log(square(5)); // Output: 25
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map(x => x ** 2);
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
While anonymous functions can be convenient, they should be used judiciously. For complex operations, named functions are often more readable and maintainable.
9. Higher-Order Functions
Higher-order functions are functions that can accept other functions as arguments or return functions. They’re a powerful feature in many programming languages and are particularly useful in functional programming paradigms.
def apply_operation(func, x, y):
return func(x, y)
def add(a, b):
return a + b
def multiply(a, b):
return a * b
print(apply_operation(add, 5, 3)) # Output: 8
print(apply_operation(multiply, 5, 3)) # Output: 15
# Using lambda functions
print(apply_operation(lambda a, b: a - b, 5, 3)) # Output: 2
Higher-order functions enable more flexible and modular code designs, allowing for powerful abstractions and code reuse.
10. Recursion
Recursion is a technique where a function calls itself to solve a problem. It’s particularly useful for tasks that can be broken down into smaller, similar sub-problems. Here’s a classic example of calculating factorial using recursion:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
print(factorial(5)) # Output: 120
While powerful, recursion should be used carefully as it can lead to stack overflow errors for large inputs. In many cases, an iterative solution might be more efficient.
11. Best Practices for Writing Functions
To write clean, efficient, and maintainable functions, consider the following best practices:
- Single Responsibility Principle: Each function should do one thing and do it well.
- Keep Functions Short: Aim for functions that are 20-30 lines or less. If a function grows too large, consider breaking it into smaller functions.
- Use Descriptive Names: Function names should clearly describe what the function does.
- Limit the Number of Parameters: If a function requires many parameters, consider using a configuration object instead.
- Use Default Arguments Wisely: Provide sensible defaults for optional parameters.
- Return Early: Use guard clauses to handle edge cases and return early from the function.
- Avoid Side Effects: Functions should not modify global state or have unexpected side effects.
- Write Pure Functions When Possible: Pure functions always produce the same output for the same input and have no side effects.
- Use Type Hints (in languages that support them): Type hints improve code readability and catch potential errors early.
- Write Documentation: Include docstrings or comments explaining what the function does, its parameters, and return values.
12. Common Pitfalls and How to Avoid Them
When working with functions, be aware of these common pitfalls:
Modifying Mutable Default Arguments
def add_item(item, list=[]): # Problematic
list.append(item)
return list
print(add_item(1)) # Output: [1]
print(add_item(2)) # Output: [1, 2] (Unexpected!)
# Correct way:
def add_item_correct(item, list=None):
if list is None:
list = []
list.append(item)
return list
print(add_item_correct(1)) # Output: [1]
print(add_item_correct(2)) # Output: [2]
Returning None Implicitly
def divide(a, b):
if b != 0:
return a / b
# Implicitly returns None if b is 0
result = divide(10, 2)
print(result) # Output: 5.0
result = divide(10, 0)
print(result) # Output: None (Might lead to errors if not handled)
Overusing Global Variables
count = 0
def increment():
global count
count += 1
# Better approach:
def increment(count):
return count + 1
Ignoring Return Values
def process_data(data):
# Process the data
return processed_data
process_data(my_data) # Return value is ignored
# Better:
result = process_data(my_data)
# Do something with the result
13. Real-World Examples
Let’s look at some real-world examples of how functions can be used effectively in programming:
Data Processing Pipeline
import pandas as pd
def load_data(file_path):
return pd.read_csv(file_path)
def clean_data(df):
df = df.dropna()
df['date'] = pd.to_datetime(df['date'])
return df
def calculate_metrics(df):
df['total_sales'] = df['quantity'] * df['price']
df['profit'] = df['total_sales'] - df['cost']
return df
def generate_report(df):
report = {
'total_profit': df['profit'].sum(),
'best_selling_product': df.groupby('product')['quantity'].sum().idxmax(),
'average_order_value': df['total_sales'].mean()
}
return report
# Usage
data = load_data('sales_data.csv')
cleaned_data = clean_data(data)
data_with_metrics = calculate_metrics(cleaned_data)
final_report = generate_report(data_with_metrics)
print(final_report)
This example demonstrates how breaking down a complex data processing task into smaller functions makes the code more readable and maintainable.
Web Scraping Utility
import requests
from bs4 import BeautifulSoup
def fetch_webpage(url):
response = requests.get(url)
response.raise_for_status()
return response.text
def parse_html(html):
return BeautifulSoup(html, 'html.parser')
def extract_titles(soup):
titles = soup.find_all('h2', class_='post-title')
return [title.text.strip() for title in titles]
def scrape_blog_titles(url):
html = fetch_webpage(url)
soup = parse_html(html)
return extract_titles(soup)
# Usage
blog_url = 'https://example.com/blog'
titles = scrape_blog_titles(blog_url)
for title in titles:
print(title)
This example shows how functions can be composed to create a simple web scraping tool, with each function handling a specific part of the process.
14. Conclusion
Functions are a fundamental concept in programming that allow us to write more organized, reusable, and maintainable code. By mastering the creation and use of functions, you’ll be able to tackle complex programming challenges more effectively and write cleaner, more efficient code.
Remember these key takeaways:
- Functions should have a single, well-defined purpose.
- Use parameters to make functions flexible and reusable.
- Return values to pass results back to the caller.
- Be mindful of scope and avoid unnecessary global variables.
- Use higher-order functions and lambda expressions for more expressive code.
- Apply best practices to write clean, readable functions.
- Be aware of common pitfalls and how to avoid them.
As you continue your programming journey, you’ll find that effective use of functions is key to solving complex problems and building robust applications. Practice creating and using functions in your projects, and you’ll soon see improvements in your code quality and problem-solving skills.
Happy coding!