The Beginner’s Guide to Variables and Data Types
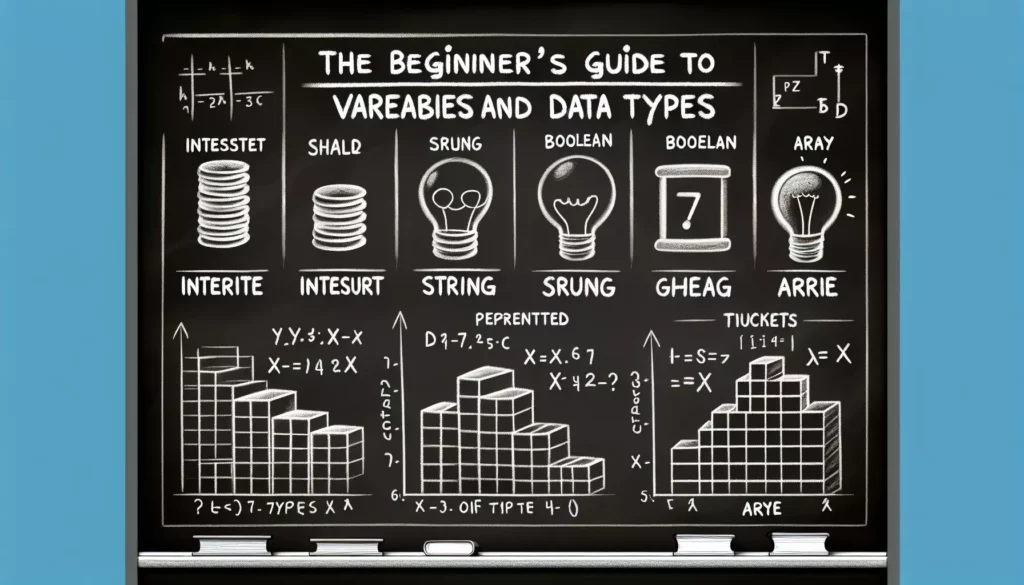
Welcome to AlgoCademy’s comprehensive guide on variables and data types! If you’re just starting your journey into the world of programming, understanding these fundamental concepts is crucial. This guide will walk you through the basics, providing you with a solid foundation for your coding adventures. Whether you’re aiming to ace technical interviews at major tech companies or simply want to enhance your problem-solving skills, mastering variables and data types is an essential first step.
Table of Contents
- What Are Variables?
- Declaring Variables
- Variable Naming Conventions
- Data Types: An Overview
- Primitive Data Types
- Complex Data Types
- Type Conversion and Casting
- How Variables Work in Memory
- Best Practices for Using Variables
- Common Mistakes and How to Avoid Them
- Practical Examples and Exercises
- Conclusion
What Are Variables?
In programming, a variable is a container for storing data values. Think of it as a labeled box where you can put information that you want to use or manipulate in your program. Variables are one of the most basic and essential concepts in coding, as they allow you to work with data in a dynamic and flexible way.
Here’s a simple analogy: Imagine you’re organizing a party, and you have boxes labeled “Decorations,” “Food,” and “Music.” These labels represent variables, and the contents of each box represent the data stored in those variables. Just as you can change the contents of these boxes, you can change the values stored in variables as your program runs.
Declaring Variables
Before you can use a variable, you need to declare it. The process of declaring a variable differs slightly depending on the programming language you’re using, but the general concept remains the same. Here are examples in a few popular languages:
Python
name = "Alice"
age = 30
height = 1.75
JavaScript
let name = "Alice";
const age = 30;
var height = 1.75;
Java
String name = "Alice";
int age = 30;
double height = 1.75;
In these examples, we’re declaring variables to store a person’s name, age, and height. Notice how different languages have different syntax for variable declaration, but the concept is similar across all of them.
Variable Naming Conventions
Choosing good variable names is crucial for writing clean, readable code. Here are some general guidelines for naming variables:
- Use descriptive names that indicate the purpose of the variable
- Start with a lowercase letter (in most languages)
- Use camelCase for multi-word names in many languages (e.g., firstName, lastLoginDate)
- Avoid using reserved keywords of the programming language
- Be consistent with your naming style throughout your code
Good variable names:
userAge
totalAmount
isLoggedIn
currentDate
Poor variable names:
a
x1
temp
myvar
Remember, your code should be easily understandable by others (and by you when you revisit it later). Clear, descriptive variable names go a long way in achieving this goal.
Data Types: An Overview
Data types are classifications that specify which type of value a variable can hold. Understanding data types is crucial because they determine how the data is stored in memory and what operations can be performed on that data. Data types can be broadly categorized into two groups: primitive data types and complex data types.
Primitive Data Types
Primitive data types are the most basic data types available in programming languages. They are usually built-in or predefined types. Here are some common primitive data types:
1. Integer (int)
Used for whole numbers, positive or negative, without decimals.
int age = 30;
int temperature = -5;
2. Float/Double
Used for numbers with decimal points. Float typically uses 32 bits, while double uses 64 bits for more precision.
float price = 19.99;
double pi = 3.14159265359;
3. Boolean
Represents true or false values.
boolean isStudent = true;
boolean hasLicense = false;
4. Character (char)
Represents a single character.
char grade = 'A';
char currency = '$';
5. String
While technically not always a primitive type (e.g., in Java), strings are used to represent text and are so commonly used that they’re often treated as a basic data type.
String name = "Alice";
String message = "Hello, World!";
Complex Data Types
Complex data types are derived from primitive data types and can hold multiple values. These include:
1. Arrays
An array is a collection of elements of the same data type.
int[] numbers = {1, 2, 3, 4, 5};
String[] fruits = {"apple", "banana", "orange"};
2. Lists
Similar to arrays but typically more flexible in size.
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
3. Objects
Objects are instances of classes and can contain multiple properties and methods.
class Person {
String name;
int age;
}
Person alice = new Person();
alice.name = "Alice";
alice.age = 30;
4. Dictionaries/Maps
These store key-value pairs.
Map<String, Integer> ages = new HashMap<>();
ages.put("Alice", 30);
ages.put("Bob", 25);
Understanding these data types is crucial for effective programming. Each type has its own use cases and limitations, and choosing the right data type for your variables can significantly impact your program’s performance and functionality.
Type Conversion and Casting
Sometimes, you need to convert a value from one data type to another. This process is known as type conversion or type casting. There are two types of type conversion:
1. Implicit Conversion (Widening)
This happens automatically when you assign a value of a smaller data type to a larger data type.
int myInt = 5;
double myDouble = myInt; // Automatically converts int to double
2. Explicit Conversion (Narrowing)
This is when you manually convert a larger data type to a smaller one. This can potentially lead to data loss and requires explicit syntax.
double myDouble = 5.7;
int myInt = (int) myDouble; // Explicitly convert double to int, result is 5
It’s important to be careful with type conversions, especially when converting from floating-point numbers to integers, as this can lead to loss of precision.
How Variables Work in Memory
Understanding how variables are stored in memory can help you write more efficient code and debug memory-related issues. Here’s a simplified explanation:
Stack vs Heap
Variables are typically stored in two areas of memory:
- Stack: Used for static memory allocation. This is where primitive data types and references to objects are stored.
- Heap: Used for dynamic memory allocation. This is where objects and more complex data structures are stored.
When you declare a primitive variable, it’s stored directly on the stack. When you create an object, the object itself is stored on the heap, but the reference to that object is stored on the stack.
int x = 5; // Stored directly on the stack
Person alice = new Person(); // Reference on stack, object on heap
This memory management system allows for efficient allocation and deallocation of memory, which is crucial for program performance.
Best Practices for Using Variables
To write clean, efficient, and maintainable code, follow these best practices when working with variables:
- Initialize variables: Always give your variables an initial value when you declare them.
- Use meaningful names: Choose variable names that clearly describe their purpose.
- Keep scope minimal: Declare variables in the smallest scope possible.
- Use constants for fixed values: If a value won’t change, declare it as a constant.
- Be consistent with naming conventions: Follow the standard conventions of your chosen programming language.
- Comment when necessary: If a variable’s purpose isn’t immediately clear from its name, add a comment explaining it.
- Avoid global variables: They can make code harder to understand and maintain.
- Use the appropriate data type: Choose the most suitable data type for your variable to optimize memory usage.
Common Mistakes and How to Avoid Them
Even experienced programmers can make mistakes with variables. Here are some common pitfalls and how to avoid them:
1. Using Uninitialized Variables
Always initialize your variables before using them.
// Incorrect
int x;
System.out.println(x); // This may cause an error or unexpected behavior
// Correct
int x = 0;
System.out.println(x);
2. Ignoring Scope
Be aware of variable scope to avoid unintended behavior.
// Incorrect
if (condition) {
int x = 5;
}
System.out.println(x); // x is not accessible here
// Correct
int x;
if (condition) {
x = 5;
}
System.out.println(x);
3. Forgetting Type Constraints
Remember the limitations of each data type.
// Incorrect
int bigNumber = 3000000000; // This will cause an error in many languages
// Correct
long bigNumber = 3000000000L;
4. Misusing = and ==
In many languages, = is for assignment, while == is for comparison.
// Incorrect (in an if statement)
if (x = 5) { // This assigns 5 to x, doesn't compare
// Correct
if (x == 5) { // This compares x to 5
5. Neglecting to Free Memory
In languages without automatic garbage collection, remember to free allocated memory.
// In C++
int* ptr = new int[10];
// Use the array
delete[] ptr; // Don't forget to free the memory
Practical Examples and Exercises
Let’s put our knowledge into practice with some examples and exercises. These will help reinforce your understanding of variables and data types.
Example 1: Temperature Converter
Create a program that converts temperature from Celsius to Fahrenheit.
double celsius = 25.0;
double fahrenheit = (celsius * 9/5) + 32;
System.out.println(celsius + " degrees Celsius is equal to " + fahrenheit + " degrees Fahrenheit.");
Example 2: Simple Interest Calculator
Calculate simple interest given principal, rate, and time.
double principal = 1000.0;
double rate = 0.05; // 5% annual interest rate
int time = 2; // 2 years
double interest = principal * rate * time;
double amount = principal + interest;
System.out.println("The simple interest is: $" + interest);
System.out.println("The total amount after " + time + " years is: $" + amount);
Exercise 1: Age Calculator
Write a program that calculates a person’s age given their birth year and the current year.
Exercise 2: BMI Calculator
Create a Body Mass Index (BMI) calculator that takes a person’s weight (in kilograms) and height (in meters) and calculates their BMI.
Exercise 3: String Manipulation
Write a program that takes a person’s full name as input and prints their initials.
Try solving these exercises on your own. They’ll help you practice working with different data types and performing calculations with variables.
Conclusion
Congratulations! You’ve just completed a comprehensive guide to variables and data types. This knowledge forms the foundation of programming and is crucial for your journey in becoming a proficient coder. Remember, practice is key to mastering these concepts. As you continue your learning journey with AlgoCademy, you’ll encounter more complex topics that build upon these fundamental ideas.
Here’s a quick recap of what we’ve covered:
- The concept and importance of variables in programming
- How to declare variables and follow naming conventions
- Different data types, both primitive and complex
- Type conversion and casting
- How variables work in memory
- Best practices for using variables
- Common mistakes to avoid
- Practical examples and exercises to reinforce your learning
As you progress in your coding journey, you’ll find that a solid understanding of variables and data types will make learning more advanced concepts much easier. Whether you’re aiming to ace technical interviews at major tech companies or simply want to enhance your problem-solving skills, this knowledge will serve as a strong foundation.
Keep practicing, stay curious, and don’t hesitate to experiment with different variables and data types in your code. Remember, every expert programmer started where you are now. With dedication and consistent practice, you’ll be well on your way to becoming a skilled developer.
Happy coding!