Mastering Nested Loops: Understanding Two For Loops in Programming
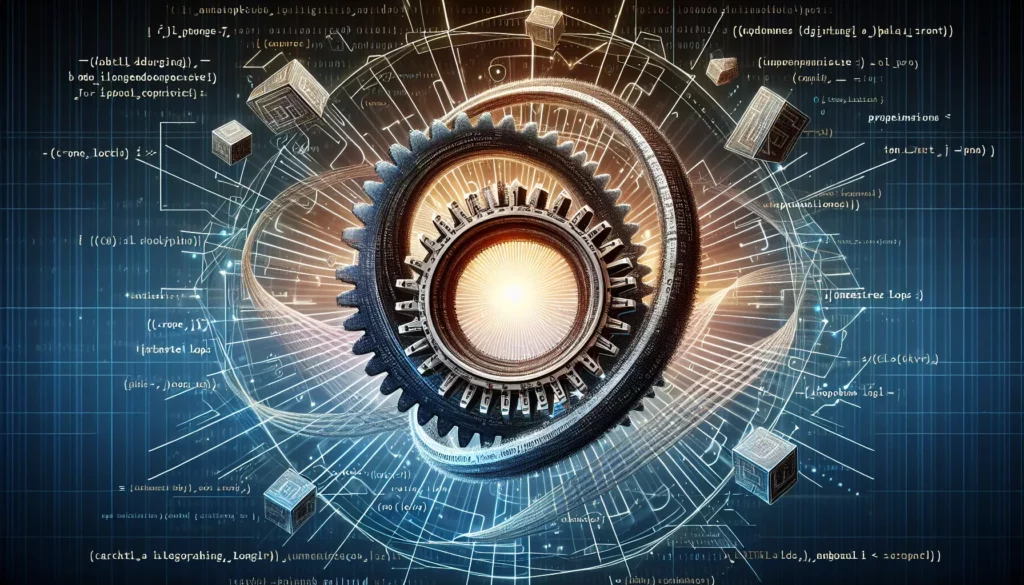
In the world of programming, loops are essential tools that allow developers to execute a block of code repeatedly. While single loops are powerful on their own, nested loops—loops within loops—open up a whole new realm of possibilities. In this comprehensive guide, we’ll dive deep into the concept of nested loops, focusing specifically on the use of two for loops. Whether you’re a beginner looking to strengthen your foundational skills or an experienced coder preparing for technical interviews at top tech companies, mastering nested loops is crucial for your programming journey.
What Are Nested Loops?
Before we delve into the intricacies of two for loops, let’s start with the basics. A nested loop occurs when one loop is placed inside another. The inner loop completes all its iterations for each iteration of the outer loop. This structure allows programmers to work with multi-dimensional data structures, perform complex calculations, and solve intricate problems efficiently.
The Anatomy of a Nested For Loop
A typical nested for loop structure looks like this:
for (initialization; condition; update) {
// Outer loop code
for (initialization; condition; update) {
// Inner loop code
}
// More outer loop code
}
In this structure, the inner loop will execute all its iterations for each single iteration of the outer loop. This pattern creates a multiplicative effect on the number of times the innermost code is executed.
Why Use Nested Loops?
Nested loops are incredibly versatile and find applications in various programming scenarios. Here are some common use cases:
- Working with 2D arrays or matrices: Nested loops are ideal for traversing and manipulating two-dimensional data structures.
- Pattern printing: Creating complex patterns or shapes in console output often requires nested loops.
- Comparing elements: When you need to compare each element of a collection with every other element, nested loops come in handy.
- Generating combinations: Nested loops are excellent for generating all possible combinations of elements from different sets.
- Solving mathematical problems: Many mathematical algorithms, especially in fields like linear algebra, rely heavily on nested loops.
Understanding the Execution Flow of Two For Loops
To truly master nested loops, it’s crucial to understand how they execute. Let’s break down the process step by step:
- The outer loop initializes its counter variable.
- The outer loop’s condition is checked.
- If the condition is true, the outer loop’s body begins to execute.
- The inner loop is encountered and initializes its counter variable.
- The inner loop’s condition is checked.
- If the inner loop’s condition is true, its body executes.
- After the inner loop’s body executes, its update statement runs.
- Steps 5-7 repeat until the inner loop’s condition becomes false.
- Once the inner loop completes, execution returns to the outer loop.
- The outer loop’s update statement runs.
- Steps 2-10 repeat until the outer loop’s condition becomes false.
Let’s illustrate this with a simple example:
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 2; j++) {
System.out.println("i = " + i + ", j = " + j);
}
}
The output of this code would be:
i = 0, j = 0
i = 0, j = 1
i = 1, j = 0
i = 1, j = 1
i = 2, j = 0
i = 2, j = 1
As you can see, for each value of i, the inner loop completes all its iterations before moving to the next value of i.
Common Patterns and Use Cases
Now that we understand the basics, let’s explore some common patterns and use cases for nested loops with two for loops.
1. Traversing 2D Arrays
One of the most common applications of nested loops is traversing 2D arrays or matrices. Here’s an example of how to print all elements of a 2D array:
int[][] matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println(); // Move to the next line after each row
}
2. Generating Multiplication Tables
Nested loops are perfect for creating multiplication tables. Here’s how you can generate a multiplication table from 1 to 10:
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 10; j++) {
System.out.printf("%4d", i * j);
}
System.out.println();
}
3. Pattern Printing
Creating patterns is another classic use case for nested loops. Here’s an example of how to print a right-angled triangle pattern:
int n = 5;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= i; j++) {
System.out.print("* ");
}
System.out.println();
}
This will output:
*
* *
* * *
* * * *
* * * * *
4. Finding Prime Numbers
Nested loops can be used to implement algorithms for finding prime numbers. Here’s a simple implementation of the Sieve of Eratosthenes algorithm:
boolean[] isPrime = new boolean[100];
Arrays.fill(isPrime, true);
isPrime[0] = isPrime[1] = false;
for (int i = 2; i * i < 100; i++) {
if (isPrime[i]) {
for (int j = i * i; j < 100; j += i) {
isPrime[j] = false;
}
}
}
// Print prime numbers
for (int i = 2; i < 100; i++) {
if (isPrime[i]) {
System.out.print(i + " ");
}
}
Optimizing Nested Loops
While nested loops are powerful, they can also be computationally expensive, especially when dealing with large datasets. Here are some tips to optimize your nested loops:
1. Minimize Work Inside the Inner Loop
Try to move as much computation as possible to the outer loops. Any operation that doesn’t depend on the inner loop’s variables should be placed in the outer loop.
2. Use Break and Continue Statements Wisely
The break
statement can be used to exit a loop early when a certain condition is met. The continue
statement skips the rest of the current iteration and moves to the next one. Used judiciously, these can significantly improve performance.
3. Consider Loop Unrolling
For small, fixed-size loops, unrolling (i.e., manually repeating the loop body) can sometimes be more efficient. However, this should be done carefully as it can make the code less readable.
4. Use Appropriate Data Structures
Sometimes, using a different data structure or algorithm can eliminate the need for nested loops altogether. Always consider if there’s a more efficient approach to your problem.
Common Pitfalls and How to Avoid Them
When working with nested loops, there are several common mistakes that programmers often make. Being aware of these can help you write more efficient and bug-free code:
1. Infinite Loops
One of the most common issues with nested loops is accidentally creating an infinite loop. This usually happens when the loop conditions are not properly updated. Always ensure that your loop variables are being correctly modified and that your loop conditions will eventually become false.
// Incorrect - Infinite loop
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
// Oops! We're modifying i instead of j
i++;
}
}
// Correct
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
// j is correctly incremented in the loop header
}
}
2. Off-by-One Errors
Off-by-one errors occur when loop boundaries are miscalculated, often due to confusion about whether to use < or <= in loop conditions. Always double-check your loop boundaries, especially when working with arrays or other indexed structures.
// Incorrect - Accessing out of bounds array element
int[][] matrix = new int[5][5];
for (int i = 0; i <= matrix.length; i++) { // Should be <, not <=
for (int j = 0; j < matrix[i].length; j++) {
// This will throw an ArrayIndexOutOfBoundsException
}
}
// Correct
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
// Correct loop boundaries
}
}
3. Unnecessary Computations
Performing unnecessary calculations inside nested loops can significantly impact performance, especially for large datasets. Try to move any calculations that don’t depend on the inner loop variables outside of the inner loop.
// Inefficient
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
result += Math.pow(2, i) * j; // Math.pow(2, i) is recalculated unnecessarily
}
}
// More efficient
for (int i = 0; i < n; i++) {
double powerOfTwo = Math.pow(2, i); // Calculate once per outer loop iteration
for (int j = 0; j < n; j++) {
result += powerOfTwo * j;
}
}
4. Incorrect Nesting Order
The order of nested loops can significantly affect performance. In general, you want to minimize the number of times the inner loop is executed. This often means putting the loop with the largest number of iterations on the inside.
// Less efficient for a 1000x10 matrix
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 1000; j++) {
// Process matrix[j][i]
}
}
// More efficient
for (int j = 0; j < 1000; j++) {
for (int i = 0; i < 10; i++) {
// Process matrix[j][i]
}
}
Advanced Techniques with Nested Loops
As you become more comfortable with basic nested loops, you can start exploring more advanced techniques. These can help you solve complex problems more efficiently and write more sophisticated algorithms.
1. Dynamic Programming with Nested Loops
Dynamic programming often involves using nested loops to build up solutions to complex problems from simpler subproblems. Here’s an example of using nested loops to solve the classic “0/1 Knapsack” problem:
int[] values = {60, 100, 120};
int[] weights = {10, 20, 30};
int W = 50;
int n = values.length;
int[][] K = new int[n + 1][W + 1];
for (int i = 0; i <= n; i++) {
for (int w = 0; w <= W; w++) {
if (i == 0 || w == 0)
K[i][w] = 0;
else if (weights[i - 1] <= w)
K[i][w] = Math.max(values[i - 1] + K[i - 1][w - weights[i - 1]], K[i - 1][w]);
else
K[i][w] = K[i - 1][w];
}
}
System.out.println("Maximum value: " + K[n][W]);
2. Nested Loops with Recursion
Combining nested loops with recursion can lead to powerful algorithms. Here’s an example of generating all possible subsets of a set using nested loops and recursion:
public static void generateSubsets(int[] nums) {
List<Integer> subset = new ArrayList<>();
generateSubsetsHelper(nums, 0, subset);
}
private static void generateSubsetsHelper(int[] nums, int index, List<Integer> subset) {
if (index == nums.length) {
System.out.println(subset);
return;
}
// Don't include nums[index]
generateSubsetsHelper(nums, index + 1, new ArrayList<>(subset));
// Include nums[index]
subset.add(nums[index]);
generateSubsetsHelper(nums, index + 1, new ArrayList<>(subset));
}
// Usage
int[] nums = {1, 2, 3};
generateSubsets(nums);
3. Nested Loops in Multithreading
When working with multithreading, nested loops can be used to parallelize computations. Here’s a simple example using Java’s parallel streams:
int[][] matrix = new int[1000][1000];
// Fill matrix with some values...
long sum = IntStream.range(0, matrix.length)
.parallel()
.mapToLong(i -> IntStream.range(0, matrix[i].length)
.map(j -> matrix[i][j])
.sum())
.sum();
System.out.println("Sum of all elements: " + sum);
Preparing for Technical Interviews
Understanding nested loops is crucial for technical interviews, especially at top tech companies. Here are some tips to help you prepare:
1. Practice, Practice, Practice
Solve problems that involve nested loops regularly. Platforms like LeetCode, HackerRank, and AlgoCademy offer a wealth of problems that can help you hone your skills.
2. Analyze Time and Space Complexity
Be prepared to discuss the time and space complexity of your nested loop solutions. For two nested loops, the time complexity is often O(n^2), but this can vary depending on the specific implementation.
3. Consider Edge Cases
When solving problems with nested loops, always consider edge cases. What happens if the input is empty? What if it contains negative numbers? Anticipating these scenarios will impress your interviewer.
4. Optimize Your Solutions
After implementing a solution with nested loops, think about ways to optimize it. Can you reduce the number of iterations? Is there a more efficient data structure you could use?
5. Explain Your Thought Process
During interviews, explain your thought process as you work through problems involving nested loops. This gives the interviewer insight into your problem-solving skills and allows them to provide hints if needed.
Conclusion
Mastering nested loops, particularly two for loops, is a fundamental skill for any programmer. They are powerful tools that allow you to solve complex problems efficiently and elegantly. By understanding their execution flow, recognizing common patterns, avoiding pitfalls, and practicing advanced techniques, you’ll be well-equipped to tackle a wide range of programming challenges.
Remember, the key to mastery is practice. Challenge yourself with increasingly complex problems, analyze your solutions, and always look for ways to optimize your code. Whether you’re preparing for technical interviews at top tech companies or simply aiming to become a better programmer, your proficiency with nested loops will serve you well throughout your coding journey.
Happy coding, and may your loops always terminate!