How to Tackle Rare and Unusual Coding Questions: A Comprehensive Guide
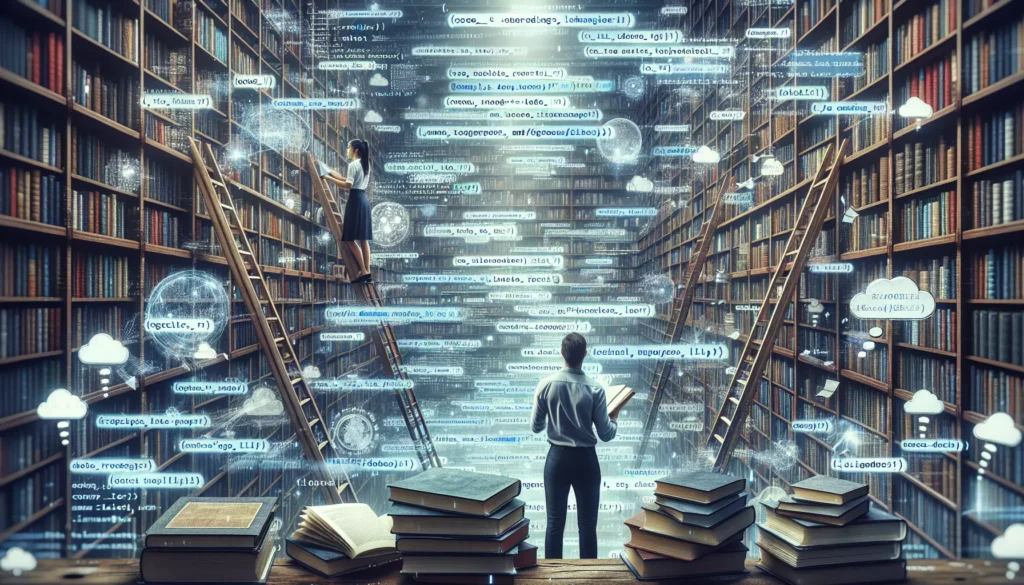
In the world of coding interviews and technical assessments, candidates often encounter a wide range of problems. While many questions fall into familiar categories like data structures, algorithms, and system design, there’s a special breed of challenges that can catch even experienced programmers off guard: rare and unusual coding questions. These questions are designed to test not just your coding skills, but your ability to think creatively, adapt quickly, and solve problems under pressure.
In this comprehensive guide, we’ll explore strategies for tackling these unconventional coding challenges. Whether you’re preparing for interviews with top tech companies or simply want to sharpen your problem-solving skills, mastering the art of handling rare and unusual coding questions can give you a significant edge.
1. Understanding the Nature of Rare and Unusual Coding Questions
Before diving into strategies, it’s essential to understand what makes a coding question “rare” or “unusual.” These types of questions often have one or more of the following characteristics:
- They involve concepts or algorithms that are not commonly used in day-to-day programming
- They require creative thinking or “out-of-the-box” solutions
- They may combine multiple concepts in unexpected ways
- They often have non-obvious optimal solutions
- They may involve domains or scenarios that are unfamiliar to most programmers
Examples of rare and unusual coding questions might include:
- Implementing a quantum algorithm in classical code
- Solving a complex geometric problem using only bitwise operations
- Designing a system to efficiently store and retrieve data from a hypothetical 4D space
- Creating an algorithm to solve a variation of a famous unsolved mathematical problem
2. Developing a Mindset for Tackling Unusual Problems
The key to successfully handling rare and unusual coding questions lies in cultivating the right mindset. Here are some mental approaches that can help:
2.1. Embrace the Challenge
Instead of feeling intimidated by an unfamiliar problem, try to see it as an exciting opportunity to learn and showcase your problem-solving skills. Remember, the interviewer is often more interested in your approach and thought process than in whether you arrive at the perfect solution.
2.2. Stay Calm and Composed
It’s natural to feel a surge of anxiety when faced with an unexpected question. Take a deep breath and remind yourself that it’s okay not to have an immediate answer. Your ability to remain calm and methodical under pressure is a valuable skill in itself.
2.3. Be Open to Learning
Approach each unusual question as a learning opportunity. Even if you don’t solve it perfectly during the interview, the experience of grappling with a novel problem can be incredibly valuable for your growth as a programmer.
2.4. Cultivate Curiosity
Develop a genuine curiosity about different areas of computer science and mathematics. The more diverse your knowledge base, the better equipped you’ll be to handle unusual questions.
3. Strategies for Approaching Rare and Unusual Coding Questions
Now that we’ve established the right mindset, let’s explore specific strategies for tackling these challenging problems:
3.1. Break Down the Problem
Even the most complex and unusual problems can often be broken down into smaller, more manageable sub-problems. Start by identifying the core components of the question and tackle them one at a time.
For example, if asked to implement a quantum algorithm classically, you might break it down into:
- Understanding the basic principles of quantum computing
- Identifying the key operations in the quantum algorithm
- Finding classical analogues for quantum operations
- Implementing these operations in your chosen programming language
3.2. Draw Analogies to Familiar Concepts
Try to connect the unusual problem to concepts you’re already familiar with. This can help you leverage your existing knowledge and problem-solving skills.
For instance, if faced with a problem involving 4D space, you might draw analogies to how we handle 3D space in computer graphics, and then extend those concepts to an additional dimension.
3.3. Start with a Brute Force Approach
When dealing with an unfamiliar problem, it’s often helpful to start with a simple, brute force solution. While this may not be efficient, it can help you understand the problem better and potentially lead to insights for optimization.
3.4. Use Visualization Techniques
Many unusual problems become clearer when visualized. Don’t hesitate to use diagrams, flowcharts, or even simple sketches to represent the problem and potential solutions.
3.5. Think Aloud
Verbalize your thought process as you work through the problem. This not only helps the interviewer understand your approach but can also lead you to new insights or catch mistakes early.
3.6. Leverage First Principles Thinking
Break down the problem to its most fundamental truths and build up from there. This approach can be particularly effective for questions that seem to have no obvious starting point.
3.7. Consider Edge Cases
Unusual problems often have interesting edge cases. Considering these can help you refine your understanding of the problem and improve your solution.
4. Techniques for Solving Specific Types of Unusual Questions
While every unusual question is unique, there are some common categories that you might encounter. Here are techniques for handling some of these:
4.1. Bitwise Operation Puzzles
Questions involving complex manipulations using only bitwise operations can be tricky. Here are some tips:
- Refresh your knowledge of bitwise operators (&, |, ^, ~, <<, >>)
- Practice visualizing numbers in binary
- Remember common bit manipulation tricks (e.g., XOR for toggling bits, AND with 1 for checking odd/even)
- Break down complex operations into a series of simpler bitwise operations
Here’s a simple example of using bitwise operations to check if a number is a power of 2:
bool isPowerOfTwo(int n) {
return n > 0 && (n & (n - 1)) == 0;
}
4.2. Mathematical Puzzles
For questions that involve complex mathematical concepts:
- Break down the mathematical problem into smaller, solvable parts
- Look for patterns or symmetries in the problem
- Consider using mathematical induction for problems involving sequences or series
- Don’t be afraid to use pen and paper to work out equations or draw diagrams
4.3. Geometric Problems
When dealing with unusual geometric questions:
- Visualize the problem, even if it involves higher dimensions
- Break complex shapes into simpler components
- Consider using coordinate systems or vector mathematics
- Look for symmetries or patterns that can simplify the problem
4.4. Algorithmic Puzzles
For questions that involve designing unusual algorithms:
- Start by solving small instances of the problem manually to identify patterns
- Consider how existing algorithms might be adapted or combined to solve the problem
- Think about the problem in terms of data structures – sometimes choosing the right data structure is key to an efficient solution
- Don’t forget about space complexity – sometimes trading space for time can lead to novel solutions
5. Practical Examples
Let’s look at a couple of examples of rare and unusual coding questions and how to approach them:
Example 1: The Skyline Problem
Problem: Given a set of buildings defined by (left, right, height), where left and right are x-coordinates, construct the skyline formed by these buildings.
Approach:
- Recognize this as a sweep line algorithm problem
- Break down the problem into events: start of a building and end of a building
- Use a priority queue to keep track of the highest current building
- Iterate through events, updating the skyline when the highest building changes
Here’s a basic implementation in Python:
import heapq
def getSkyline(buildings):
events = []
for l, r, h in buildings:
events.append((l, -h, r)) # start event
events.append((r, 0, 0)) # end event
events.sort()
res = [[0, 0]] # skyline result
heap = [(0, float('inf'))] # (height, ending position)
for pos, negH, r in events:
while heap[0][1] <= pos:
heapq.heappop(heap)
if negH != 0:
heapq.heappush(heap, (negH, r))
if res[-1][1] != -heap[0][0]:
res.append([pos, -heap[0][0]])
return res[1:]
Example 2: Implement Pow(x, n) without using multiplication or division
Problem: Implement pow(x, n), which calculates x raised to the power n (i.e., x^n), without using multiplication or division operations.
Approach:
- Recognize this as a problem that can be solved using bitwise operations
- Use the binary representation of the exponent
- Implement multiplication as repeated addition
- Use bit shifting for efficient computation
Here’s a C++ implementation:
class Solution {
public:
double myPow(double x, int n) {
long long N = n;
if (N < 0) {
x = 1 / x;
N = -N;
}
double ans = 1;
double current_product = x;
for (long long i = N; i; i /= 2) {
if ((i & 1) == 1) {
ans = fastMultiply(ans, current_product);
}
current_product = fastMultiply(current_product, current_product);
}
return ans;
}
private:
double fastMultiply(double a, double b) {
double result = 0;
while (b > 0) {
if (b & 1) {
result += a;
}
a += a;
b >>= 1;
}
return result;
}
};
6. Preparing for Rare and Unusual Coding Questions
While it’s impossible to prepare for every possible unusual question, there are steps you can take to improve your readiness:
6.1. Broaden Your Knowledge Base
Explore areas of computer science and mathematics beyond your usual focus. This might include:
- Advanced data structures (e.g., segment trees, Fenwick trees)
- Less common algorithms (e.g., Mo’s algorithm, heavy-light decomposition)
- Computational geometry
- Number theory
- Graph theory
- Probability and statistics
6.2. Practice Problem-Solving
Regularly challenge yourself with difficult problems from various sources:
- Competitive programming platforms (e.g., Codeforces, TopCoder)
- Online judges (e.g., LeetCode, HackerRank)
- Programming competitions (e.g., Google Code Jam, Facebook Hacker Cup)
- Mathematical olympiads and puzzles
6.3. Study Past Interview Questions
While you’re unlikely to get the exact same question, studying past unusual interview questions can help you understand the types of creative thinking that interviewers are looking for.
6.4. Improve Your Problem-Solving Process
Practice breaking down complex problems, identifying patterns, and explaining your thought process clearly. These skills are valuable regardless of the specific question you encounter.
6.5. Learn to Implement from Scratch
Practice implementing fundamental data structures and algorithms from scratch. This will deepen your understanding and prepare you for questions that require adapting or combining these basics in novel ways.
7. Handling Unusual Questions During an Interview
When you encounter a rare or unusual coding question during an actual interview, remember these key points:
7.1. Clarify the Problem
Don’t hesitate to ask questions to ensure you fully understand the problem. Clarify any ambiguities and confirm your understanding with the interviewer.
7.2. Think Aloud
Share your thought process with the interviewer. This gives them insight into your problem-solving approach and allows them to provide hints if needed.
7.3. Start with What You Know
Begin with the aspects of the problem that you’re familiar with. This can help build your confidence and may lead to insights about the more unusual aspects.
7.4. Be Open to Hints
If the interviewer offers a hint, take it! They’re often trying to guide you towards the solution, not trick you.
7.5. Don’t Give Up
Even if you can’t solve the entire problem, keep working and discussing your ideas. Interviewers often value persistence and problem-solving approach as much as getting the perfect solution.
8. Learning from Unusual Coding Questions
After encountering a rare or unusual coding question, whether in an interview or during practice, take time to reflect and learn:
8.1. Analyze the Solution
If you didn’t solve the problem completely, research the solution afterwards. Understand not just how it works, but why it’s effective.
8.2. Look for Underlying Principles
Try to identify the fundamental concepts or techniques that made the solution work. These principles might be applicable to other problems in the future.
8.3. Implement and Experiment
Code up the solution yourself and experiment with it. Try modifying the problem slightly and see how the solution needs to change.
8.4. Teach Others
Explaining the problem and solution to others can deepen your understanding and help you internalize the concepts.
9. Conclusion
Rare and unusual coding questions can be challenging, but they also present a unique opportunity to showcase your problem-solving skills and creativity. By developing the right mindset, employing effective strategies, and continuously expanding your knowledge, you can approach these questions with confidence.
Remember, the goal of these questions is often not just to test your coding ability, but to evaluate your thought process, creativity, and ability to tackle unfamiliar problems. Even if you don’t arrive at a perfect solution, demonstrating a logical approach, clear communication, and persistence can leave a strong positive impression.
As you continue your journey in software development, embrace these challenging questions as opportunities for growth. Each unusual problem you encounter expands your problem-solving toolkit and makes you a more versatile and resourceful programmer.
Keep practicing, stay curious, and don’t be afraid to think outside the box. With time and experience, you’ll find that even the most unusual coding questions become exciting puzzles to solve rather than daunting obstacles.