Techniques for Optimizing Recursive Algorithms: Enhancing Efficiency in Coding
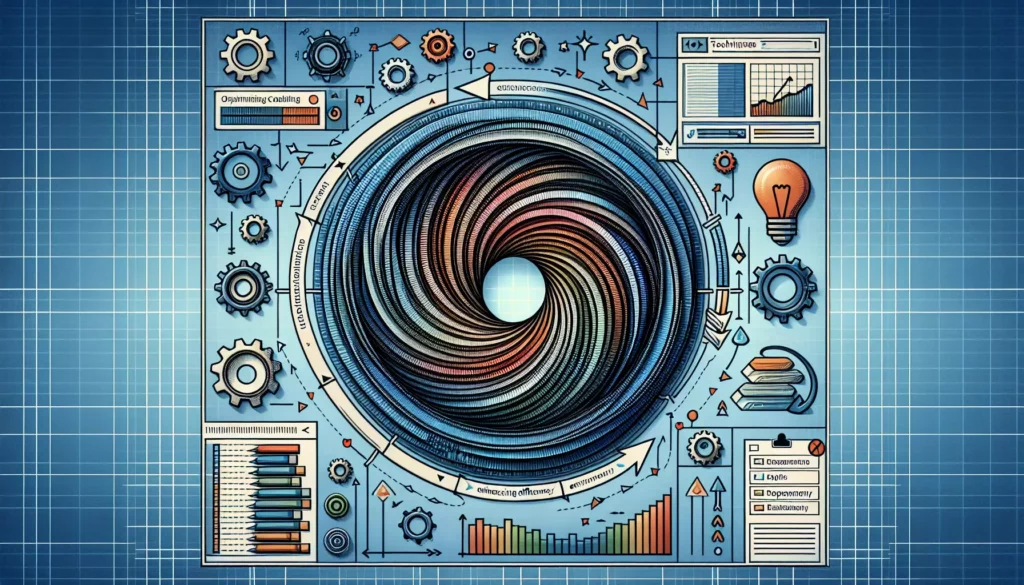
Recursive algorithms are a fundamental concept in computer science and programming, often used to solve complex problems by breaking them down into smaller, more manageable subproblems. While recursion can lead to elegant and intuitive solutions, it can also introduce performance issues if not implemented carefully. In this comprehensive guide, we’ll explore various techniques for optimizing recursive algorithms, helping you write more efficient code and improve your problem-solving skills.
Understanding Recursion
Before diving into optimization techniques, let’s briefly review what recursion is and why it’s important in programming:
- Recursion is a method where a function calls itself to solve a problem by breaking it down into smaller instances of the same problem.
- It consists of a base case (the simplest form of the problem that can be solved directly) and a recursive case (where the function calls itself with a simpler version of the problem).
- Recursive algorithms are often used for tasks like traversing tree-like data structures, implementing divide-and-conquer algorithms, and solving problems with a naturally recursive structure.
While recursion can lead to elegant solutions, it can also be inefficient if not implemented properly. Let’s explore some techniques to optimize recursive algorithms and make them more efficient.
1. Tail Recursion Optimization
Tail recursion is a special form of recursion where the recursive call is the last operation in the function. Many modern compilers can optimize tail-recursive functions to be as efficient as iterative loops.
Example of Tail Recursion:
def factorial(n, accumulator=1):
if n == 0:
return accumulator
return factorial(n - 1, n * accumulator)
In this example, the recursive call to factorial
is the last operation, making it tail-recursive. Some compilers can optimize this to use constant stack space, effectively turning it into an iterative solution.
Benefits of Tail Recursion:
- Reduces stack overflow risks
- Can be as efficient as iterative solutions
- Maintains the clarity and elegance of recursive code
2. Memoization
Memoization is a technique used to store the results of expensive function calls and return the cached result when the same inputs occur again. This is particularly useful for recursive algorithms that solve overlapping subproblems.
Example of Memoization:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
result = fibonacci(n - 1, memo) + fibonacci(n - 2, memo)
memo[n] = result
return result
In this Fibonacci sequence implementation, we use a dictionary to store previously computed values, dramatically reducing the number of recursive calls for larger inputs.
Benefits of Memoization:
- Significantly reduces redundant calculations
- Can change time complexity from exponential to polynomial in many cases
- Useful for problems with overlapping subproblems
3. Dynamic Programming
Dynamic programming is an extension of the memoization concept, where we solve a problem by breaking it down into simpler subproblems and storing their solutions to avoid redundant computations. While not strictly a recursion optimization, it often replaces recursive solutions with more efficient iterative ones.
Example of Dynamic Programming:
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i - 1] + dp[i - 2]
return dp[n]
This dynamic programming approach to the Fibonacci sequence eliminates recursion entirely, solving the problem iteratively while storing intermediate results.
Benefits of Dynamic Programming:
- Often more efficient than recursive solutions for problems with overlapping subproblems
- Can significantly improve time and space complexity
- Useful for optimization problems and certain types of counting problems
4. Divide and Conquer
The divide and conquer approach involves breaking a problem into smaller subproblems, solving them independently, and then combining their solutions. While this is often inherently recursive, optimizing the division and combination steps can lead to significant performance improvements.
Example of Divide and Conquer:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
This merge sort implementation demonstrates the divide and conquer approach. The problem of sorting an array is divided into sorting smaller subarrays, which are then merged.
Benefits of Divide and Conquer:
- Can lead to efficient algorithms for large-scale problems
- Often results in logarithmic time complexity for certain operations
- Suitable for parallel processing, as subproblems can be solved independently
5. Recursion Elimination
In some cases, recursive algorithms can be rewritten as iterative ones, eliminating recursion altogether. This can lead to performance improvements and reduced stack usage.
Example of Recursion Elimination:
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
This iterative version of the factorial function eliminates recursion, potentially improving performance and reducing stack usage.
Benefits of Recursion Elimination:
- Reduces stack overflow risks
- Can improve performance by eliminating function call overhead
- Often leads to more straightforward and easier-to-optimize code
6. Tail Call Optimization (TCO)
Tail Call Optimization is a compiler optimization technique that transforms recursive calls in tail position into jumps, effectively eliminating the need for additional stack frames. While not all programming languages or compilers support TCO, understanding it can help in writing more optimizable recursive code.
Example of a Tail-Call Optimizable Function:
def sum_to_n(n, accumulator=0):
if n == 0:
return accumulator
return sum_to_n(n - 1, accumulator + n)
In languages that support TCO, this function could be optimized to use constant stack space, regardless of the input size.
Benefits of Tail Call Optimization:
- Allows for writing recursive functions that don’t consume stack space linearly
- Enables recursive solutions to problems that would otherwise cause stack overflow
- Can make recursive code as efficient as iterative code in terms of space usage
7. Caching and Precomputation
For recursive algorithms that repeatedly perform the same calculations, caching results or precomputing values can significantly improve performance.
Example of Caching:
class Solution:
def climbStairs(self, n: int) -> int:
cache = {}
def climb(i):
if i <= 1:
return 1
if i in cache:
return cache[i]
cache[i] = climb(i-1) + climb(i-2)
return cache[i]
return climb(n)
This solution to the “Climbing Stairs” problem uses a cache to store previously computed results, significantly reducing the number of recursive calls.
Benefits of Caching and Precomputation:
- Reduces redundant calculations in recursive calls
- Can dramatically improve time complexity for certain problems
- Useful for problems with repeated subproblems or calculations
8. Hybrid Approaches
Sometimes, combining recursive and iterative approaches can lead to more efficient algorithms. This hybrid approach can leverage the clarity of recursion for the overall structure while using iteration for performance-critical parts.
Example of a Hybrid Approach:
def quick_sort(arr):
def partition(low, high):
pivot = arr[high]
i = low - 1
for j in range(low, high):
if arr[j] <= pivot:
i += 1
arr[i], arr[j] = arr[j], arr[i]
arr[i + 1], arr[high] = arr[high], arr[i + 1]
return i + 1
def quick_sort_recursive(low, high):
if low < high:
pi = partition(low, high)
quick_sort_recursive(low, pi - 1)
quick_sort_recursive(pi + 1, high)
quick_sort_recursive(0, len(arr) - 1)
return arr
This quicksort implementation uses recursion for the overall divide-and-conquer strategy but implements the partition step iteratively for better performance.
Benefits of Hybrid Approaches:
- Combines the clarity of recursion with the efficiency of iteration
- Can lead to more optimized solutions for complex problems
- Allows for fine-tuning performance in critical sections of the algorithm
9. Recursion Depth Limiting
In some cases, especially when dealing with potentially infinite recursion or very deep recursive calls, it can be beneficial to implement a depth limit to prevent stack overflow.
Example of Recursion Depth Limiting:
def depth_limited_search(graph, start, goal, max_depth):
def dls(node, depth):
if depth == 0:
return None
if node == goal:
return [node]
if depth > 0:
for neighbor in graph[node]:
path = dls(neighbor, depth - 1)
if path is not None:
return [node] + path
return None
return dls(start, max_depth)
This depth-limited search algorithm prevents excessive recursion by limiting the depth of the search tree.
Benefits of Recursion Depth Limiting:
- Prevents stack overflow in potentially infinite or very deep recursions
- Can be used to implement iterative deepening search strategies
- Useful for graph traversal algorithms in large or cyclic graphs
10. Parallelization of Recursive Algorithms
For recursive algorithms that work on independent subproblems, parallelization can significantly improve performance on multi-core systems.
Example of Parallelized Recursion:
import multiprocessing
def parallel_merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
with multiprocessing.Pool(processes=2) as pool:
left, right = pool.map(parallel_merge_sort, [arr[:mid], arr[mid:]])
return merge(left, right)
def merge(left, right):
# Merge implementation (same as before)
pass
This parallel merge sort implementation uses multiprocessing to sort subarrays concurrently, potentially speeding up the sorting process on multi-core systems.
Benefits of Parallelization:
- Can significantly speed up recursive algorithms on multi-core systems
- Particularly effective for divide-and-conquer algorithms
- Allows for better utilization of available computing resources
Conclusion
Optimizing recursive algorithms is a crucial skill for any programmer looking to write efficient and scalable code. By applying techniques such as tail recursion, memoization, dynamic programming, and others discussed in this article, you can significantly improve the performance of your recursive algorithms.
Remember that the best optimization technique depends on the specific problem and context. Sometimes, a simple recursive solution might be more readable and maintainable than a highly optimized one. Always consider the trade-offs between performance, readability, and maintainability when optimizing your code.
As you continue to develop your programming skills, practice implementing these optimization techniques in your recursive algorithms. This will not only improve your code’s efficiency but also deepen your understanding of algorithmic thinking and problem-solving strategies.
Keep exploring, practicing, and refining your skills in algorithm optimization. With time and experience, you’ll be able to choose the most appropriate optimization techniques for any given recursive problem, leading to more efficient and effective solutions in your coding journey.