The Significance of Software Testing Principles: A Comprehensive Guide
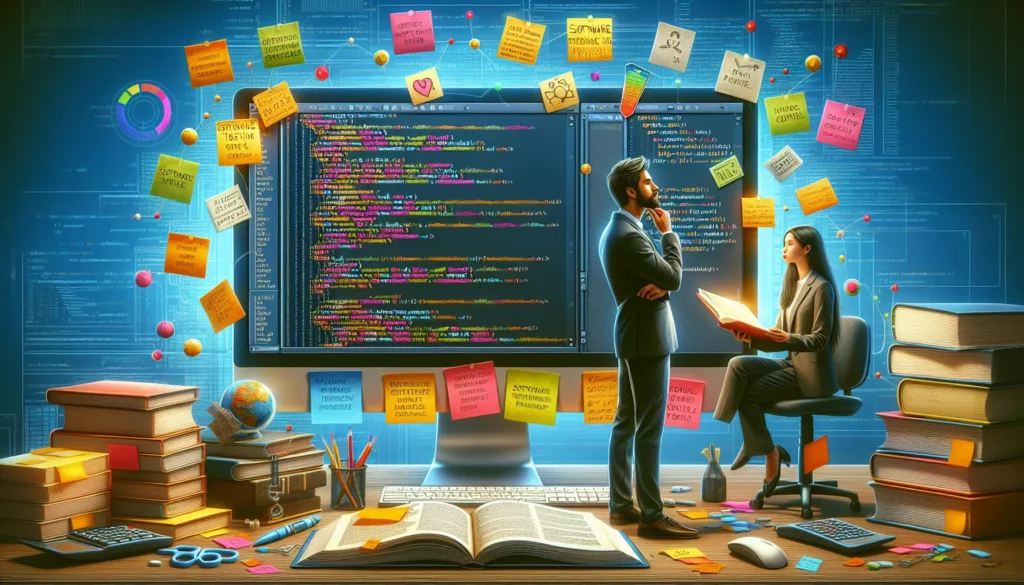
In the ever-evolving world of software development, the importance of robust testing cannot be overstated. As we dive deeper into the realm of coding education and programming skills development, it’s crucial to understand the fundamental principles that guide effective software testing. This comprehensive guide will explore the significance of software testing principles, their impact on code quality, and how they contribute to the overall success of software projects.
Table of Contents
- Introduction to Software Testing
- Key Principles of Software Testing
- Types of Software Testing
- Best Practices in Software Testing
- Popular Software Testing Tools
- Common Challenges in Software Testing
- The Future of Software Testing
- Conclusion
1. Introduction to Software Testing
Software testing is an integral part of the software development lifecycle (SDLC) that involves evaluating and verifying that a software application or program works as expected. It’s a process of executing a program or application with the intent of finding software bugs, errors, or defects. The primary goal of software testing is to ensure the delivery of high-quality software that meets the specified requirements and provides a seamless user experience.
In the context of coding education and programming skills development, understanding software testing principles is crucial for several reasons:
- It helps developers write more reliable and robust code
- It improves the overall quality of software products
- It reduces the cost of maintenance and support in the long run
- It enhances the user experience by identifying and fixing issues before release
- It prepares aspiring developers for real-world scenarios and technical interviews
As we delve deeper into the world of software testing, it’s important to note that testing is not just about finding bugs. It’s about building confidence in the software’s functionality, performance, and reliability. Let’s explore the key principles that guide effective software testing practices.
2. Key Principles of Software Testing
Understanding and adhering to the fundamental principles of software testing is essential for developing high-quality software. These principles serve as a foundation for creating effective test strategies and ensuring comprehensive coverage. Let’s examine some of the most important software testing principles:
2.1 Testing Shows the Presence of Defects
This principle emphasizes that testing can only prove the existence of errors, not their absence. No matter how thoroughly you test a software application, you can never guarantee that it’s 100% bug-free. The goal of testing is to uncover as many defects as possible and reduce the risk of undiscovered issues.
2.2 Exhaustive Testing is Impossible
It’s practically impossible to test every possible input, user scenario, and path through the software. Instead, testers should focus on risk-based testing, prioritizing the most critical functionalities and high-risk areas of the application.
2.3 Early Testing
The earlier you start testing in the software development lifecycle, the more cost-effective it becomes to fix defects. This principle aligns with the shift-left testing approach, which emphasizes testing activities earlier in the development process.
2.4 Defect Clustering
This principle suggests that a small number of modules in a software application usually contain most of the defects. By identifying these problematic areas, testers can focus their efforts more efficiently.
2.5 Pesticide Paradox
If you repeatedly run the same tests, they will eventually stop finding new bugs. To overcome this, it’s important to regularly review and update test cases, adding variety to the testing process.
2.6 Testing is Context Dependent
Different types of software require different testing approaches. For example, testing a mobile app is different from testing a web application or an embedded system. The testing strategy should be tailored to the specific context of the software being developed.
2.7 Absence of Errors Fallacy
Just because a software application is free of bugs doesn’t mean it meets user requirements or is usable. Testing should also focus on verifying that the software fulfills its intended purpose and provides value to the end-users.
By understanding and applying these principles, developers and testers can create more effective testing strategies and improve the overall quality of their software products. Let’s now explore the various types of software testing that put these principles into practice.
3. Types of Software Testing
Software testing can be categorized into various types, each serving a specific purpose in the quality assurance process. Understanding these different types of testing is crucial for comprehensive test coverage and ensuring that all aspects of the software are thoroughly evaluated. Here are some of the most common types of software testing:
3.1 Unit Testing
Unit testing involves testing individual components or functions of the software in isolation. It’s typically performed by developers as they write code to ensure that each unit of the software performs as designed. Unit tests are usually automated and run frequently as part of the development process.
Example of a simple unit test in Python using the unittest framework:
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add(-1, -1), -2)
def test_add_zero(self):
self.assertEqual(add(0, 5), 5)
if __name__ == '__main__':
unittest.main()
3.2 Integration Testing
Integration testing focuses on verifying that different components or modules of the software work together correctly. This type of testing ensures that the interfaces between components function as expected and that data is properly passed between different parts of the system.
3.3 Functional Testing
Functional testing verifies that the software meets the specified functional requirements. It involves testing the software’s features and functionality to ensure they work as intended from the user’s perspective.
3.4 Performance Testing
Performance testing evaluates how well the software performs under various conditions, such as high load or concurrent users. It includes testing for responsiveness, scalability, and stability under different scenarios.
3.5 Security Testing
Security testing aims to identify vulnerabilities in the software that could be exploited by malicious users. It involves testing for common security issues such as SQL injection, cross-site scripting (XSS), and authentication flaws.
3.6 Usability Testing
Usability testing focuses on the user experience, evaluating how easy and intuitive the software is to use. It often involves real users interacting with the software and providing feedback.
3.7 Regression Testing
Regression testing ensures that new changes or additions to the software don’t negatively impact existing functionality. It involves re-running previously executed tests to verify that previously developed and tested software still performs correctly.
3.8 Acceptance Testing
Acceptance testing is typically performed by the client or end-user to determine if the software meets the business requirements and is ready for deployment. It’s the final stage of testing before the software is released to production.
By employing a combination of these testing types, development teams can ensure comprehensive coverage and improve the overall quality of their software products. In the next section, we’ll explore best practices for implementing effective software testing strategies.
4. Best Practices in Software Testing
Implementing effective software testing practices is crucial for delivering high-quality software products. Here are some best practices that can help improve the testing process and overall software quality:
4.1 Start Testing Early
Incorporate testing from the beginning of the software development lifecycle. This approach, known as “shift-left testing,” helps identify and fix issues earlier, reducing the cost and effort of resolving problems later in the development process.
4.2 Use Test-Driven Development (TDD)
Test-Driven Development is an approach where tests are written before the actual code. This practice encourages developers to think about the requirements and expected behavior of the software before implementation, leading to more robust and well-designed code.
Example of TDD workflow:
- Write a failing test for a new feature
- Run the test to ensure it fails
- Write the minimum amount of code to make the test pass
- Run the test again to ensure it passes
- Refactor the code if necessary
- Repeat the process for the next feature or requirement
4.3 Automate Testing Where Possible
Automated testing can significantly improve the efficiency and consistency of the testing process. While not all tests can be automated, focus on automating repetitive and time-consuming tests, such as regression tests and unit tests.
4.4 Maintain a Comprehensive Test Suite
Develop and maintain a diverse set of test cases that cover various scenarios, including edge cases and potential error conditions. Regularly review and update the test suite to ensure it remains relevant as the software evolves.
4.5 Implement Continuous Integration and Continuous Testing
Integrate testing into your continuous integration (CI) pipeline to automatically run tests whenever code changes are pushed. This practice helps catch issues early and ensures that the main branch of your codebase always remains in a releasable state.
4.6 Use Code Coverage Tools
Employ code coverage tools to measure the extent to which your source code is tested. While 100% code coverage doesn’t guarantee bug-free software, it can help identify areas of the codebase that lack sufficient test coverage.
4.7 Conduct Regular Code Reviews
Code reviews can help identify potential issues, improve code quality, and ensure adherence to coding standards. Include reviewing test cases and testing strategies as part of the code review process.
4.8 Document Test Cases and Results
Maintain clear and detailed documentation of test cases, test results, and any issues discovered during testing. This documentation can be invaluable for tracking progress, reproducing issues, and onboarding new team members.
4.9 Prioritize Testing Based on Risk
Focus testing efforts on high-risk areas of the application, such as critical functionality, frequently used features, or components with a history of defects. This risk-based approach helps allocate testing resources more effectively.
4.10 Foster a Quality-Focused Culture
Encourage a culture where quality is everyone’s responsibility, not just the testers’. Promote collaboration between developers, testers, and other stakeholders to ensure a shared understanding of quality goals and testing strategies.
By implementing these best practices, development teams can significantly improve their testing processes and ultimately deliver higher-quality software products. In the next section, we’ll explore some popular tools that can assist in implementing these practices effectively.
5. Popular Software Testing Tools
The right tools can significantly enhance the efficiency and effectiveness of software testing efforts. Here’s an overview of some popular software testing tools categorized by their primary functions:
5.1 Test Management Tools
- TestRail: A web-based test case management tool that helps teams organize, track, and coordinate their testing efforts.
- Zephyr: An end-to-end test management tool that integrates with Jira and other popular development tools.
- qTest: A test management platform that supports agile testing methodologies and integrates with various CI/CD tools.
5.2 Automated Testing Tools
- Selenium: An open-source tool for automating web browsers, widely used for web application testing.
- Appium: An open-source tool for automating mobile application testing on both Android and iOS platforms.
- JUnit: A popular unit testing framework for Java applications.
- PyTest: A testing framework for Python that simplifies test writing and execution.
5.3 Performance Testing Tools
- Apache JMeter: An open-source tool for load testing and measuring performance.
- Gatling: A highly capable load testing tool that focuses on web applications.
- LoadRunner: A comprehensive performance testing tool by Micro Focus.
5.4 Security Testing Tools
- OWASP ZAP (Zed Attack Proxy): An open-source web application security scanner.
- Burp Suite: A popular platform for performing security testing of web applications.
- Acunetix: An automated web vulnerability scanner that detects and reports on a wide range of web security issues.
5.5 Continuous Integration/Continuous Deployment (CI/CD) Tools
- Jenkins: An open-source automation server that supports building, deploying, and automating any project.
- GitLab CI/CD: A CI/CD tool integrated into the GitLab platform.
- Travis CI: A hosted continuous integration service used to build and test software projects hosted on GitHub.
5.6 Code Coverage Tools
- Codecov: A tool that integrates with CI/CD pipelines to measure and report code coverage.
- Coveralls: A web service that helps track code coverage over time and ensures that all new code is fully covered.
- JaCoCo: A free code coverage library for Java.
5.7 API Testing Tools
- Postman: A popular tool for API development and testing.
- SoapUI: An open-source tool for testing SOAP and REST APIs.
- REST Assured: A Java library for testing and validating REST services.
When selecting tools for your testing process, consider factors such as:
- The specific needs of your project
- Integration capabilities with your existing tech stack
- Learning curve and ease of use
- Community support and documentation
- Cost (for commercial tools)
Remember that while tools can greatly enhance the testing process, they are not a substitute for a well-thought-out testing strategy and skilled testers. The most effective testing approaches combine the right tools with solid testing principles and practices.
6. Common Challenges in Software Testing
While software testing is crucial for delivering high-quality products, it comes with its own set of challenges. Understanding these challenges can help teams better prepare and develop strategies to overcome them. Here are some common challenges faced in software testing:
6.1 Insufficient Testing Time
Often, testing is squeezed into tight project timelines, leading to inadequate testing coverage. This can result in undetected bugs making their way into production.
Solution: Advocate for testing throughout the development process (shift-left testing) and automate where possible to increase efficiency.
6.2 Incomplete Requirements
Unclear or constantly changing requirements can make it difficult to design comprehensive test cases and determine what constitutes a “pass” or “fail” scenario.
Solution: Encourage clear communication between stakeholders, developers, and testers. Use techniques like behavior-driven development (BDD) to create shared understanding.
6.3 Test Environment Management
Setting up and maintaining test environments that accurately reflect production can be challenging, especially for complex systems.
Solution: Utilize containerization technologies like Docker to create consistent and reproducible test environments. Implement infrastructure-as-code practices for managing test environments.
6.4 Handling Test Data
Creating, managing, and maintaining test data that covers various scenarios without violating data privacy regulations can be complex.
Solution: Implement data masking techniques, use synthetic data generation tools, and establish clear processes for test data management.
6.5 Testing Complex Integrations
Modern applications often involve multiple integrations with third-party services, making end-to-end testing challenging.
Solution: Use service virtualization to simulate the behavior of external services. Implement contract testing to verify interactions between services.
6.6 Keeping Up with Rapid Development Cycles
Agile and DevOps practices have accelerated development cycles, making it challenging for testing to keep pace.
Solution: Implement continuous testing practices, automate regression tests, and use risk-based testing to prioritize critical areas.
6.7 Mobile Device Fragmentation
The wide variety of mobile devices, operating systems, and screen sizes makes comprehensive mobile testing difficult.
Solution: Use cloud-based mobile device farms for testing on real devices. Implement responsive design testing and focus on the most common device configurations.
6.8 Security Testing Complexity
With increasing cyber threats, security testing has become more critical and complex.
Solution: Integrate security testing tools into the CI/CD pipeline. Conduct regular security audits and penetration testing. Train developers in secure coding practices.
6.9 Balancing Manual and Automated Testing
Determining which tests to automate and which to keep manual can be challenging, especially with limited resources.
Solution: Prioritize automation for repetitive, high-value tests. Use a risk-based approach to determine which tests provide the most value when automated.
6.10 Lack of Skilled Testers
The demand for skilled testers, especially those familiar with modern testing practices and tools, often exceeds supply.
Solution: Invest in training and upskilling programs. Foster a culture of quality where developers are also involved in testing. Consider partnering with testing service providers for specialized skills.
By recognizing these challenges and implementing appropriate solutions, teams can improve their testing processes and deliver higher-quality software products. In the next section, we’ll look at emerging trends and the future of software testing.
7. The Future of Software Testing
As technology continues to evolve at a rapid pace, so does the field of software testing. Understanding emerging trends and future directions can help teams prepare for the changing landscape of software quality assurance. Here are some key trends shaping the future of software testing:
7.1 Artificial Intelligence and Machine Learning in Testing
AI and ML are increasingly being applied to various aspects of software testing:
- Test Case Generation: AI can analyze application behavior and automatically generate test cases, reducing the manual effort required in test design.
- Predictive Analytics: ML algorithms can predict potential areas of failure based on historical data, allowing testers to focus on high-risk areas.
- Self-Healing Tests: AI-powered tools can automatically update test scripts when the application under test changes, reducing maintenance efforts.
7.2 Shift-Right Testing
While shift-left testing (moving testing earlier in the development cycle) has been a trend, there’s also a growing focus on shift-right testing:
- Continuous monitoring and testing in production environments
- Real-time analytics to detect and respond to issues in live systems
- Canary releases and feature toggles to safely test new features with real users
7.3 IoT and Edge Computing Testing
As Internet of Things (IoT) devices and edge computing become more prevalent, testing strategies will need to adapt:
- Testing for diverse hardware and software configurations
- Simulating real-world conditions and scenarios for IoT devices
- Performance testing for edge computing environments
7.4 Increased Focus on Non-Functional Testing
While functional testing remains crucial, there’s growing emphasis on non-functional aspects:
- Accessibility testing to ensure applications are usable by people with disabilities
- Usability and user experience (UX) testing
- Performance testing under various network conditions (especially for mobile and IoT applications)
7.5 Blockchain Testing
As blockchain technology gains adoption, specialized testing approaches are emerging:
- Smart contract testing
- Consensus mechanism testing
- Performance and scalability testing for blockchain networks
7.6 Quantum Computing and Testing
As quantum computing advances, it will bring new challenges and opportunities for testing:
- Testing quantum algorithms and their classical counterparts
- Verifying the correctness of quantum computations
- Developing new testing methodologies for quantum software
7.7 Continuous Testing in DevOps and DevSecOps
The integration of testing into DevOps practices will continue to evolve:
- Further automation of the testing process
- Closer collaboration between developers, testers, and operations teams
- Integration of security testing throughout the development lifecycle (DevSecOps)
7.8 Testing for AI and ML Systems
As AI and ML become more prevalent in software systems, new testing challenges emerge:
- Testing AI model accuracy and bias
- Ensuring the explainability and interpretability of AI decisions
- Testing the robustness of ML models against adversarial attacks
7.9 Low-Code/No-Code Testing
The rise of low-code and no-code development platforms will influence testing practices:
- Visual, drag-and-drop test creation tools
- Automated testing of applications built with low-code platforms
- Simplified test case management for non-technical stakeholders
7.10 Ethical and Responsible AI Testing
As AI systems become more prevalent, ensuring they operate ethically and responsibly will be crucial:
- Testing for fairness and non-discrimination in AI decisions
- Verifying compliance with AI ethics guidelines and regulations
- Assessing the societal impact of AI systems
As these trends evolve, software testing professionals will need to continuously update their skills and adapt their practices. The future of software testing promises to be both challenging and exciting, with new technologies offering both novel testing challenges and innovative solutions.
8. Conclusion
Software testing principles form the backbone of quality assurance in software development. As we’ve explored throughout this comprehensive guide, these principles are not just theoretical concepts but practical guidelines that shape how we approach testing in real-world scenarios.
From understanding the key principles like early testing and defect clustering to exploring various types of testing and best practices, we’ve seen how a systematic approach to testing can significantly improve software quality. The challenges in software testing, while numerous, can be overcome with the right strategies and tools.
Looking to the future, it’s clear that software testing will continue to evolve alongside technological advancements. The integration of AI and ML in testing, the rise of IoT and edge computing, and the increasing focus on ethical AI are just a few of the trends that will shape the future of software quality assurance.
For aspiring developers and those looking to enhance their programming skills, understanding and applying these testing principles is crucial. It not only helps in writing more robust and reliable code but also prepares you for the challenges of real-world software development and technical interviews at major tech companies.
Remember, effective testing is not just about finding bugs; it’s about building confidence in the software’s quality and reliability. By embracing these principles and staying abreast of emerging trends, you can contribute to creating software that not only meets specifications but truly delights users.
As you continue your journey in coding education and skills development, keep these testing principles in mind. They will serve as valuable guideposts, helping you create better software and become a more well-rounded developer. The world of software testing is vast and ever-changing, offering endless opportunities for learning and growth. Embrace these challenges, and you’ll be well-prepared for the exciting future of software development.