Preparing for Coding Interviews in Different Languages: A Comprehensive Guide
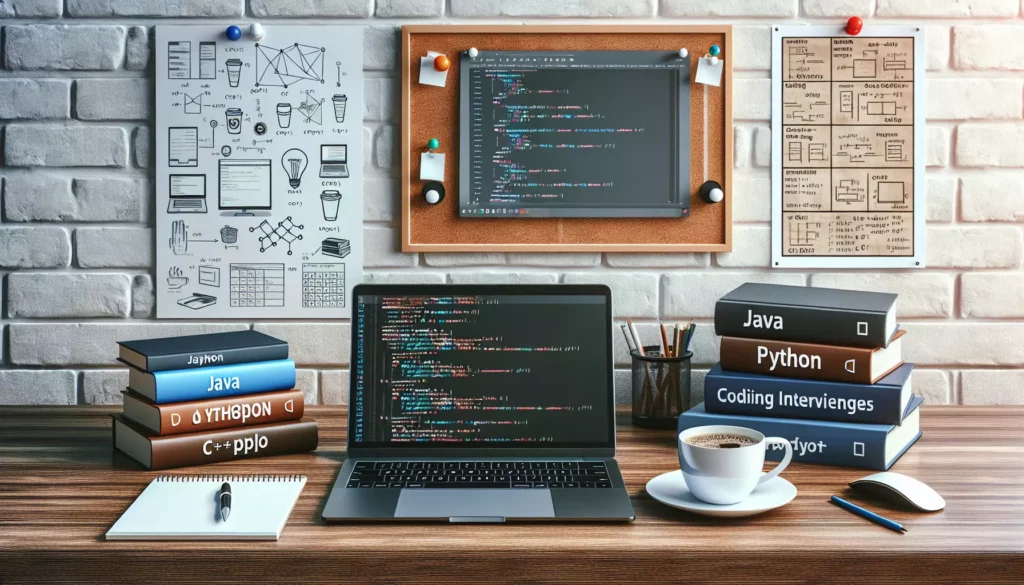
Coding interviews are a crucial step in the hiring process for many tech companies, especially the giants like FAANG (Facebook, Amazon, Apple, Netflix, Google). These interviews test not only your coding skills but also your problem-solving abilities, algorithmic thinking, and ability to communicate your thought process. While the core concepts remain the same, preparing for coding interviews in different programming languages can present unique challenges and opportunities. In this comprehensive guide, we’ll explore how to effectively prepare for coding interviews across various popular languages, focusing on strategies that will help you excel regardless of your chosen language.
1. Understanding the Importance of Language Choice
Before diving into specific preparation strategies, it’s crucial to understand why your choice of programming language matters in coding interviews:
- Company preferences: Some companies may have a preferred language based on their tech stack.
- Problem suitability: Certain languages may be better suited for specific types of problems.
- Your comfort level: Using a language you’re comfortable with can help you focus on problem-solving rather than syntax.
- Showcasing versatility: Proficiency in multiple languages can demonstrate your adaptability as a developer.
While many companies allow candidates to choose their preferred language, it’s always a good idea to research the company’s tech stack and any language requirements before the interview.
2. Core Concepts to Master Across All Languages
Regardless of the programming language you choose, there are fundamental concepts that you should master for coding interviews:
2.1 Data Structures
Understanding and implementing common data structures is crucial. Focus on:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, etc.)
- Graphs
- Hash Tables
- Heaps
2.2 Algorithms
Familiarize yourself with key algorithmic concepts and techniques:
- Sorting algorithms (Quick Sort, Merge Sort, etc.)
- Searching algorithms (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Recursion
- Divide and Conquer
2.3 Time and Space Complexity Analysis
Being able to analyze and optimize the efficiency of your solutions is crucial. Make sure you understand:
- Big O notation
- Time complexity analysis
- Space complexity analysis
- Trade-offs between time and space
3. Language-Specific Preparation Strategies
While the core concepts remain consistent, each programming language has its own quirks and strengths. Let’s explore how to prepare for coding interviews in some popular languages:
3.1 Python
Python is known for its simplicity and readability, making it a popular choice for coding interviews.
Key Focus Areas:
- List comprehensions and generator expressions
- Built-in functions like
map()
,filter()
, andreduce()
- Dictionary and set operations
- String manipulation methods
- Lambda functions
Example: Using list comprehension for concise code
# Traditional approach
squares = []
for i in range(10):
squares.append(i**2)
# List comprehension
squares = [i**2 for i in range(10)]
3.2 Java
Java’s strong typing and object-oriented nature make it a robust choice for interviews, especially for backend and enterprise-focused roles.
Key Focus Areas:
- Collections framework (ArrayList, HashMap, etc.)
- Stream API for functional-style operations
- Object-oriented design principles
- Exception handling
- Generics
Example: Using Stream API for efficient operations
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Traditional approach
int sum = 0;
for (int num : numbers) {
if (num % 2 == 0) {
sum += num;
}
}
// Using Stream API
int sum = numbers.stream()
.filter(num -> num % 2 == 0)
.mapToInt(Integer::intValue)
.sum();
3.3 C++
C++ is favored for its performance and low-level control, making it ideal for system-level programming and competitive coding.
Key Focus Areas:
- STL containers and algorithms
- Memory management and pointers
- Object-oriented features (inheritance, polymorphism)
- Template programming
- Operator overloading
Example: Using STL algorithms for efficient operations
#include <vector>
#include <algorithm>
std::vector<int> numbers = {5, 2, 8, 1, 9};
// Sorting
std::sort(numbers.begin(), numbers.end());
// Finding an element
auto it = std::find(numbers.begin(), numbers.end(), 8);
if (it != numbers.end()) {
// Element found
}
3.4 JavaScript
JavaScript’s versatility makes it a popular choice, especially for front-end and full-stack roles.
Key Focus Areas:
- Closures and scope
- Promises and async/await for asynchronous programming
- Functional programming concepts
- ES6+ features (arrow functions, destructuring, etc.)
- DOM manipulation (for front-end roles)
Example: Using async/await for asynchronous operations
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
4. General Interview Preparation Tips
Regardless of the programming language you choose, these general tips will help you prepare effectively for coding interviews:
4.1 Practice, Practice, Practice
Consistent practice is key to success in coding interviews. Here are some ways to practice effectively:
- Solve coding problems regularly: Use platforms like LeetCode, HackerRank, or AlgoCademy to practice a wide range of problems.
- Time yourself: Most coding interviews have time constraints, so practice solving problems within a set time limit.
- Mock interviews: Conduct mock interviews with friends or use online platforms that offer this service.
- Implement data structures from scratch: This deepens your understanding and prepares you for questions that require custom implementations.
4.2 Develop a Problem-Solving Approach
Having a structured approach to problem-solving can help you tackle unfamiliar problems during interviews:
- Understand the problem: Clarify any ambiguities and ask questions if needed.
- Plan your approach: Think about potential solutions before starting to code.
- Write pseudocode: Outline your solution in plain language before writing actual code.
- Implement the solution: Write clean, readable code.
- Test your solution: Consider edge cases and test your code with various inputs.
- Optimize: Look for ways to improve the time or space complexity of your solution.
4.3 Improve Your Communication Skills
Coding interviews aren’t just about writing code; they’re also about communicating your thought process:
- Think out loud: Explain your reasoning as you work through the problem.
- Ask clarifying questions: Don’t hesitate to seek clarification on problem details or requirements.
- Explain your approach: Before coding, outline your planned approach to the interviewer.
- Discuss trade-offs: Be prepared to discuss the pros and cons of different solutions.
4.4 Study System Design (for Senior Roles)
For more senior positions, system design questions are often part of the interview process:
- Understand scalability concepts
- Learn about distributed systems
- Study common architectures (microservices, event-driven, etc.)
- Practice designing high-level system architectures
5. Language-Specific Resources and Tools
To further enhance your preparation, here are some language-specific resources and tools:
5.1 Python
- Books: “Python Crash Course” by Eric Matthes, “Fluent Python” by Luciano Ramalho
- Online Courses: Coursera’s “Python for Everybody” specialization, edX’s “Introduction to Computer Science and Programming Using Python”
- Tools: PyCharm (IDE), Jupyter Notebooks for interactive coding
5.2 Java
- Books: “Effective Java” by Joshua Bloch, “Java: The Complete Reference” by Herbert Schildt
- Online Courses: Coursera’s “Java Programming and Software Engineering Fundamentals” specialization, Udacity’s “Java Programming Basics”
- Tools: IntelliJ IDEA or Eclipse (IDEs), JUnit for unit testing
5.3 C++
- Books: “C++ Primer” by Stanley B. Lippman, “Effective Modern C++” by Scott Meyers
- Online Courses: edX’s “Introduction to C++”, Coursera’s “C++ For C Programmers”
- Tools: Visual Studio Code with C++ extensions, CLion (IDE)
5.4 JavaScript
- Books: “Eloquent JavaScript” by Marijn Haverbeke, “You Don’t Know JS” series by Kyle Simpson
- Online Courses: freeCodeCamp’s JavaScript Algorithms and Data Structures certification, Udacity’s “JavaScript and the DOM”
- Tools: Visual Studio Code, Chrome DevTools for debugging
6. Adapting to Different Interview Formats
Coding interviews can take various formats, and it’s essential to be prepared for each:
6.1 Whiteboard Coding
Traditional whiteboard interviews require you to write code on a whiteboard or piece of paper:
- Practice writing code by hand to get comfortable with this format
- Focus on clear, readable code structure
- Be prepared to explain your thought process as you write
6.2 Online Coding Platforms
Many companies use online coding platforms for remote interviews:
- Familiarize yourself with common platforms like HackerRank, CoderPad, or LeetCode
- Practice using these platforms to get comfortable with their interfaces
- Ensure you have a stable internet connection for online interviews
6.3 Take-Home Coding Assignments
Some companies provide take-home assignments to be completed over a longer period:
- Manage your time effectively
- Focus on code quality, documentation, and testing
- Be prepared to explain and defend your design decisions
7. Handling Common Interview Scenarios
Being prepared for various scenarios can help you stay calm and focused during the interview:
7.1 When You’re Stuck
- Don’t panic – it’s normal to encounter challenging problems
- Break down the problem into smaller, manageable parts
- Consider solving a simpler version of the problem first
- Ask for hints if you’re truly stuck – interviewers often appreciate proactive communication
7.2 Optimizing Your Solution
- Always consider the time and space complexity of your initial solution
- Think about edge cases and how to handle them
- If time allows, discuss potential optimizations with your interviewer
7.3 Debugging Your Code
- Stay calm if your code doesn’t work on the first try
- Use a systematic approach to identify and fix bugs
- Consider using print statements or debugger tools if available
- Explain your debugging process to the interviewer
8. Post-Interview Reflection and Improvement
After each interview, take time to reflect and improve:
- Review your performance: Identify areas where you struggled or excelled
- Research questions you found challenging: Study optimal solutions and practice similar problems
- Seek feedback: If possible, ask the interviewer or recruiter for constructive feedback
- Adjust your preparation strategy: Focus on areas that need improvement
Conclusion
Preparing for coding interviews in different languages requires a combination of strong fundamental knowledge, language-specific expertise, and effective problem-solving skills. By mastering core concepts, practicing regularly, and adapting to various interview formats, you can confidently approach coding interviews regardless of the programming language required.
Remember that the key to success lies not just in writing perfect code, but in demonstrating your thought process, problem-solving approach, and ability to communicate effectively. With dedication and consistent practice, you can develop the skills and confidence needed to excel in coding interviews across different programming languages.
As you continue your preparation journey, platforms like AlgoCademy can provide valuable resources, interactive coding tutorials, and AI-powered assistance to help you progress from beginner-level coding to mastering the skills needed for technical interviews at major tech companies. Embrace the learning process, stay persistent, and approach each interview as an opportunity to showcase your skills and passion for programming.