How to Effectively Use Comments in Your Code: A Comprehensive Guide
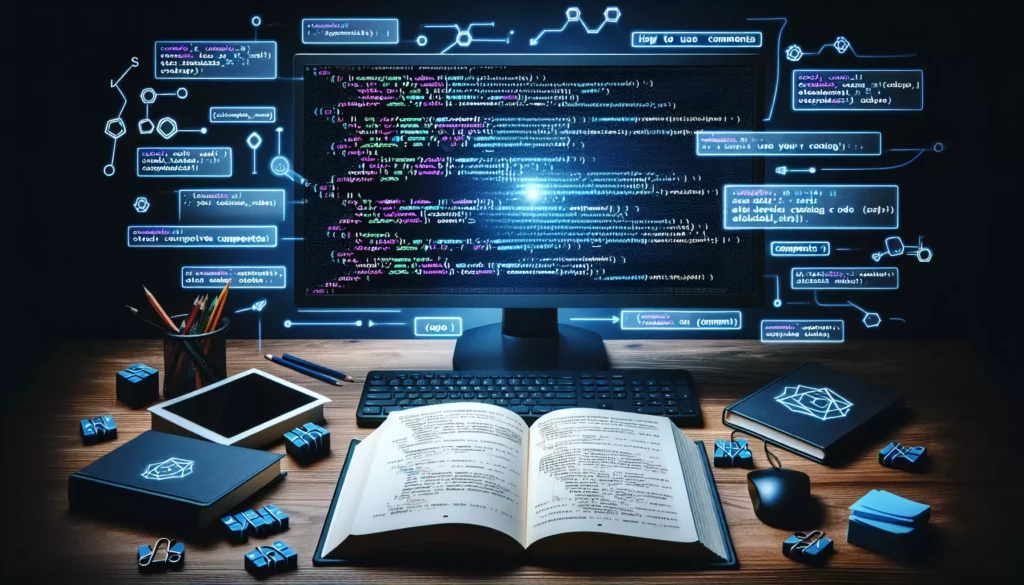
In the world of programming, writing clean and understandable code is a crucial skill. One of the most powerful tools at a developer’s disposal for enhancing code readability and maintainability is the effective use of comments. In this comprehensive guide, we’ll explore the art of commenting your code, discussing best practices, common pitfalls, and how to leverage comments to improve your overall coding skills.
1. Understanding the Purpose of Comments
Before diving into the specifics of how to write effective comments, it’s essential to understand their primary purposes:
- Explanation: Comments help explain complex logic or algorithms that may not be immediately obvious from the code itself.
- Documentation: They can serve as inline documentation, providing context and usage instructions for functions, classes, or modules.
- Clarification: Comments can clarify the intent behind certain code decisions, especially when the implementation might seem counterintuitive.
- TODO markers: They can be used to mark areas of code that need future attention or improvement.
- Debugging aids: Comments can be useful for temporarily disabling code sections during debugging or development.
2. Types of Comments
Most programming languages support two main types of comments:
2.1. Single-line Comments
Single-line comments are typically used for brief explanations or notes. In many languages, they start with a double forward slash (//).
// This is a single-line comment
int x = 5; // Initializing x with the value 5
2.2. Multi-line Comments
Multi-line comments are used for longer explanations or when commenting out larger blocks of code. They often start with /* and end with */.
/*
This is a multi-line comment.
It can span several lines and is useful for longer explanations.
*/
3. Best Practices for Writing Effective Comments
Now that we understand the basics, let’s explore some best practices for writing effective comments:
3.1. Be Clear and Concise
Write comments that are easy to understand and to the point. Avoid unnecessary verbosity.
// Good
// Calculate the average of the array elements
double average = calculateAverage(numbers);
// Bad
// This line of code is responsible for computing the arithmetic mean of all the numerical values contained within the array by summing them up and dividing by the total count of elements present in the aforementioned array structure
double average = calculateAverage(numbers);
3.2. Comment on the Why, Not the What
Focus on explaining why certain code decisions were made rather than restating what the code does.
// Good
// Use a HashMap for O(1) lookup time
Map<String, Integer> userScores = new HashMap<>();
// Bad
// Create a new HashMap
Map<String, Integer> userScores = new HashMap<>();
3.3. Keep Comments Updated
Ensure that your comments remain accurate as your code evolves. Outdated comments can be more harmful than no comments at all.
3.4. Use Proper Grammar and Spelling
Treat comments as an integral part of your code. Use correct grammar, spelling, and punctuation to maintain professionalism and clarity.
3.5. Avoid Redundant Comments
Don’t state the obvious. If the code is self-explanatory, additional comments may not be necessary.
// Bad
int x = 5; // Set x to 5
// Good
int maxAttempts = 5; // Limit login attempts to prevent brute-force attacks
3.6. Use TODO Comments Wisely
TODO comments are helpful for marking areas that need future attention, but don’t overuse them. Make sure to address these comments in a timely manner.
// TODO: Implement error handling for network failures
public void fetchUserData() {
// ...
}
4. Commenting Different Code Elements
Different parts of your code may require different commenting approaches:
4.1. Function/Method Comments
For functions or methods, focus on describing the purpose, parameters, return values, and any exceptions that might be thrown.
/**
* Calculates the factorial of a given number.
*
* @param n The number to calculate the factorial for.
* @return The factorial of n.
* @throws IllegalArgumentException if n is negative.
*/
public int factorial(int n) {
if (n < 0) {
throw new IllegalArgumentException("n must be non-negative");
}
// Implementation...
}
4.2. Class Comments
Class comments should provide an overview of the class’s purpose, its main functionalities, and any important usage notes.
/**
* Represents a bank account with basic operations like deposit and withdraw.
* This class is thread-safe and can be used in concurrent environments.
*/
public class BankAccount {
// Class implementation...
}
4.3. Inline Comments
Use inline comments sparingly, only when necessary to explain complex logic or non-obvious decisions.
public void processData(List<String> data) {
for (String item : data) {
// Skip empty items to avoid unnecessary processing
if (item.isEmpty()) {
continue;
}
// Process the item...
}
}
5. Language-Specific Comment Conventions
Different programming languages may have specific conventions for comments. Here are a few examples:
5.1. Java
Java uses Javadoc comments for documenting classes, methods, and fields. These comments start with /** and end with */.
/**
* This class represents a simple calculator.
* @author John Doe
* @version 1.0
*/
public class Calculator {
/**
* Adds two numbers.
* @param a the first number
* @param b the second number
* @return the sum of a and b
*/
public int add(int a, int b) {
return a + b;
}
}
5.2. Python
Python uses docstrings for function and class documentation. These are enclosed in triple quotes (”’ or “””).
def calculate_area(radius):
"""
Calculate the area of a circle.
Args:
radius (float): The radius of the circle.
Returns:
float: The area of the circle.
"""
return 3.14159 * radius ** 2
5.3. JavaScript
JavaScript often uses JSDoc comments for documenting functions and classes. These are similar to Javadoc comments.
/**
* Represents a book.
* @constructor
* @param {string} title - The title of the book.
* @param {string} author - The author of the book.
*/
function Book(title, author) {
this.title = title;
this.author = author;
}
6. Tools for Generating Documentation from Comments
Many programming languages have tools that can generate documentation from properly formatted comments. Here are a few examples:
- JavaDoc: For Java, generates HTML documentation from Java source code and comments.
- Doxygen: A documentation generator for various languages including C++, C, Python, and more.
- JSDoc: Generates documentation for JavaScript code.
- Sphinx: A popular documentation generator for Python projects.
These tools can significantly enhance the value of your comments by creating comprehensive, navigable documentation for your codebase.
7. Common Pitfalls to Avoid
While comments are generally beneficial, there are some common mistakes to avoid:
7.1. Over-commenting
Don’t comment on every line of code. This can make the code harder to read and maintain.
// Bad
int sum = 0; // Initialize sum to 0
for (int i = 0; i < 10; i++) { // Loop from 0 to 9
sum += i; // Add i to sum
}
// The sum is now calculated
7.2. Commenting Out Code
Avoid leaving large blocks of commented-out code in your codebase. If you need to temporarily disable code, consider using version control systems instead.
7.3. Misleading Comments
Ensure your comments accurately reflect the code. Misleading comments can be worse than no comments at all.
// Bad
// Check if the number is even
if (number % 2 == 1) {
// ...
}
7.4. Using Comments to Explain Poor Code
If you find yourself writing extensive comments to explain confusing code, consider refactoring the code instead to make it more self-explanatory.
8. Comments and Code Reviews
Comments play a crucial role in code reviews. They can help reviewers understand your thought process and the reasons behind certain implementation decisions. Here are some tips for using comments effectively in code reviews:
- Use comments to explain complex algorithms or business logic that might not be immediately obvious.
- Add comments to highlight areas where you’re particularly interested in feedback.
- If you’ve made a decision between multiple approaches, briefly comment on why you chose the implemented solution.
9. Comments in Different Programming Paradigms
The approach to commenting can vary depending on the programming paradigm you’re using:
9.1. Object-Oriented Programming (OOP)
In OOP, focus on commenting classes, interfaces, and public methods. Explain the purpose of each class and how it fits into the larger system.
9.2. Functional Programming
In functional programming, comments often focus on explaining the transformations being applied to data and the purpose of pure functions.
9.3. Procedural Programming
In procedural programming, comments might focus more on explaining the steps of algorithms and the flow of control through the program.
10. Comments and Clean Code
While comments are valuable, it’s important to remember that the best code is self-documenting. Here are some principles of clean code that can reduce the need for extensive commenting:
- Use Descriptive Names: Choose clear and descriptive names for variables, functions, and classes.
- Keep Functions Small: Smaller functions are often self-explanatory and require fewer comments.
- Use Design Patterns: Familiar design patterns can convey a lot of information without the need for extensive comments.
- Follow the Single Responsibility Principle: When each function or class has a single, well-defined purpose, it’s often easier to understand without extensive comments.
11. Comments in Open Source Projects
When contributing to open source projects, clear and comprehensive comments become even more crucial. They help other contributors understand your code and the project maintainers to review your contributions effectively. Here are some additional considerations for commenting in open source projects:
- Follow the project’s existing commenting style and conventions.
- Use comments to explain any workarounds or temporary solutions, especially if they address specific issues or pull requests.
- Include links to relevant issues, pull requests, or external resources in your comments when appropriate.
12. The Future of Code Comments
As programming languages and development tools evolve, so too does the role of comments in code. Some emerging trends include:
- Interactive Documentation: Tools that allow developers to run and interact with code examples directly from the comments.
- AI-Assisted Commenting: AI tools that can suggest or generate comments based on the code context.
- Living Documentation: Systems that keep comments and documentation in sync with the code automatically.
Conclusion
Effective use of comments is a skill that develops over time. By following best practices, avoiding common pitfalls, and consistently working to improve your commenting style, you can significantly enhance the quality and maintainability of your code. Remember, the goal of comments is to clarify and explain, making your code more accessible to others (and to your future self). As you continue to grow as a developer, pay attention to how you use comments and always look for ways to make your code more self-explanatory and your comments more meaningful.
By mastering the art of commenting, you’ll not only improve your own coding skills but also contribute to creating more robust, maintainable, and collaborative software projects. Whether you’re working on personal projects, contributing to open source, or developing in a professional environment, effective commenting is a valuable skill that will serve you well throughout your programming career.