The Role of Algorithmic Intuition in Technical Interviews: A Comprehensive Guide
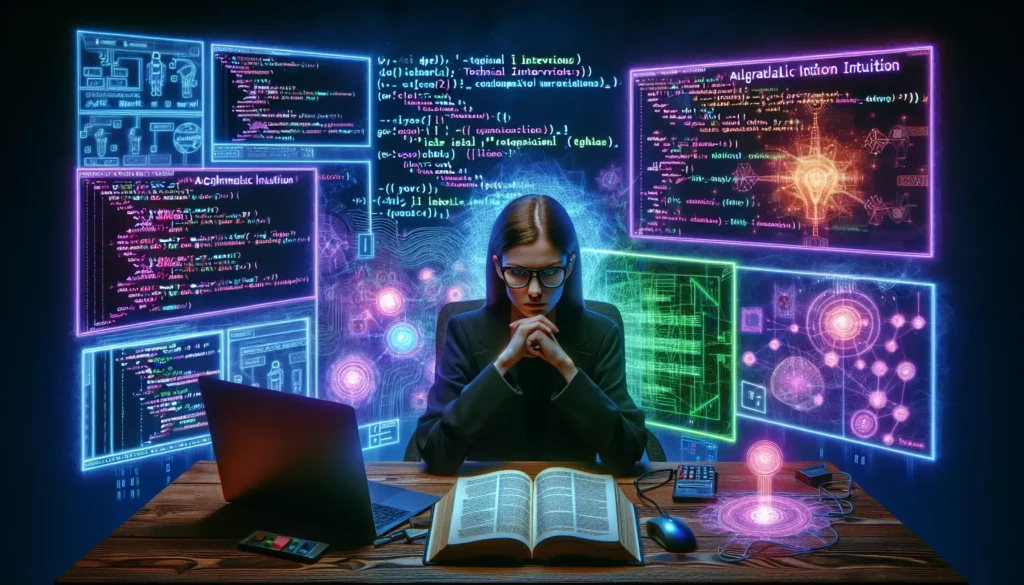
In the competitive landscape of software engineering, technical interviews have become a crucial gateway for landing coveted positions at top tech companies. Among the various skills assessed during these interviews, algorithmic intuition stands out as a fundamental attribute that can make or break a candidate’s performance. This blog post delves deep into the concept of algorithmic intuition, its significance in technical interviews, and how aspiring developers can cultivate this essential skill.
Understanding Algorithmic Intuition
Algorithmic intuition refers to the ability to quickly grasp the underlying patterns and optimal approaches to solving computational problems. It’s not just about memorizing algorithms or data structures; rather, it’s about developing a keen sense for problem-solving strategies that can be applied across a wide range of scenarios.
This intuition allows developers to:
- Quickly identify the most suitable algorithm for a given problem
- Recognize patterns that link seemingly disparate problems
- Estimate the time and space complexity of potential solutions
- Adapt known algorithms to novel situations
- Optimize solutions efficiently
The Importance of Algorithmic Intuition in Technical Interviews
Technical interviews, especially those conducted by major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), place a significant emphasis on algorithmic problem-solving. Here’s why algorithmic intuition is crucial in these high-stakes situations:
1. Time Constraints
Interviews typically last 45-60 minutes, during which candidates are expected to solve one or more complex problems. Strong algorithmic intuition allows candidates to quickly assess the problem, consider multiple approaches, and settle on an optimal solution within the given time frame.
2. Novel Problems
Interviewers often present unique or modified versions of classic problems to test a candidate’s ability to apply their knowledge in new contexts. Algorithmic intuition helps in recognizing underlying patterns and adapting known solutions to these novel scenarios.
3. Optimization Requirements
It’s rarely enough to provide a working solution; candidates are often asked to optimize their initial approach. Intuition about algorithmic efficiency helps in identifying bottlenecks and suggesting improvements.
4. Communication of Thought Process
Interviewers are interested not just in the final solution, but in how candidates arrive at it. Strong algorithmic intuition allows for clearer articulation of reasoning, trade-offs, and decision-making processes.
5. Handling Pressure
The interview environment can be stressful. Having a well-developed intuition serves as a fallback, allowing candidates to rely on their instincts when under pressure.
Cultivating Algorithmic Intuition
Developing algorithmic intuition is a gradual process that requires consistent practice and exposure to a variety of problems. Here are some strategies to help cultivate this skill:
1. Solve Diverse Problems
Expose yourself to a wide range of algorithmic problems. Platforms like LeetCode, HackerRank, and CodeForces offer thousands of problems across various difficulty levels and topics.
2. Study Classic Algorithms and Data Structures
Gain a deep understanding of fundamental algorithms and data structures. This knowledge forms the building blocks of algorithmic intuition. Some essential topics include:
- Sorting algorithms (Quick Sort, Merge Sort, Heap Sort)
- Searching algorithms (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Graph algorithms (Dijkstra’s, Floyd-Warshall, Minimum Spanning Tree)
- Tree traversals and manipulations
- Hash tables and collision resolution techniques
3. Analyze Multiple Solutions
For each problem you solve, don’t stop at your first working solution. Explore alternative approaches, compare their time and space complexities, and understand the trade-offs involved.
4. Practice Problem Classification
As you solve more problems, try to classify them based on the underlying patterns or algorithms they employ. This helps in quickly recognizing similar problems in the future.
5. Implement Algorithms from Scratch
Don’t rely solely on built-in functions or libraries. Implement classic algorithms and data structures from scratch to gain a deeper understanding of their inner workings.
6. Time Your Problem-Solving Sessions
Simulate interview conditions by setting time limits for solving problems. This helps in developing the ability to think quickly and manage time effectively.
7. Engage in Coding Competitions
Participate in coding contests on platforms like CodeForces or TopCoder. These competitions often feature problems that require quick thinking and efficient solutions, which can sharpen your algorithmic intuition.
8. Review and Reflect
After solving a problem, take time to reflect on your approach. Ask yourself:
- Could the solution be optimized further?
- Are there any edge cases I didn’t consider initially?
- How does this problem relate to others I’ve solved?
- What new concept or technique did I learn from this problem?
9. Study Solution Patterns
Familiarize yourself with common solution patterns like Two Pointers, Sliding Window, or the Fast and Slow Pointer technique. Recognizing these patterns can significantly speed up your problem-solving process.
10. Leverage Visualization Tools
Use algorithm visualization tools to better understand how different algorithms work. Websites like VisuAlgo offer interactive visualizations of various data structures and algorithms.
Applying Algorithmic Intuition in Interviews
Having developed your algorithmic intuition, it’s crucial to effectively apply it during technical interviews. Here are some tips:
1. Think Aloud
Verbalize your thought process as you approach the problem. This not only demonstrates your intuition but also allows the interviewer to provide guidance if needed.
2. Start with a Brute Force Approach
Begin by outlining a simple, perhaps inefficient solution. This shows you can at least solve the problem and provides a starting point for optimization.
3. Consider Multiple Approaches
Don’t commit to the first solution that comes to mind. Briefly consider alternative approaches and discuss their trade-offs with the interviewer.
4. Analyze Time and Space Complexity
Before diving into coding, discuss the expected time and space complexity of your proposed solution. This demonstrates your understanding of algorithmic efficiency.
5. Use Analogies and Visualizations
If struggling to explain an concept, try using analogies or drawing diagrams. Visual representations can often clarify complex ideas.
6. Break Down the Problem
If faced with a complex problem, break it down into smaller, manageable sub-problems. This approach often reveals familiar patterns or algorithms that can be applied.
7. Optimize Incrementally
After implementing a working solution, look for opportunities to optimize. Discuss potential improvements with the interviewer, showing your ability to refine and enhance your initial approach.
Common Pitfalls to Avoid
While developing and applying algorithmic intuition, be aware of these common pitfalls:
1. Over-reliance on Memorization
While it’s important to know classic algorithms, relying solely on memorized solutions can backfire when faced with novel problems. Focus on understanding the underlying principles rather than rote memorization.
2. Neglecting Edge Cases
Strong intuition involves anticipating and handling edge cases. Always consider scenarios like empty inputs, negative numbers, or maximum values when designing your solution.
3. Jumping to Code Too Quickly
Resist the urge to start coding immediately. Take time to fully understand the problem and plan your approach. Premature coding often leads to suboptimal solutions and wasted time.
4. Ignoring Problem Constraints
Pay close attention to the given constraints, such as input size or time/space complexity requirements. These often provide clues about the expected solution approach.
5. Overlooking Simple Solutions
Sometimes, a problem that seems complex at first glance can be solved with a surprisingly simple approach. Don’t discount straightforward solutions in favor of more complex ones.
Advanced Techniques for Enhancing Algorithmic Intuition
As you progress in your journey to develop strong algorithmic intuition, consider these advanced techniques:
1. Study Algorithm Design Paradigms
Familiarize yourself with broader algorithm design paradigms such as:
- Divide and Conquer
- Dynamic Programming
- Greedy Algorithms
- Backtracking
Understanding these paradigms can help you approach a wide variety of problems more systematically.
2. Analyze Space-Time Tradeoffs
Practice identifying situations where you can trade space for time, or vice versa. This often involves techniques like precomputation, caching, or using additional data structures to speed up operations.
3. Explore Amortized Analysis
Learn about amortized analysis to understand the performance of algorithms that have occasional expensive operations but perform well on average. This is particularly useful for data structures like dynamic arrays or splay trees.
4. Study Randomized Algorithms
Familiarize yourself with randomized algorithms and their analysis. Understanding concepts like expected running time and probabilistic guarantees can broaden your problem-solving toolkit.
5. Implement Lower-Level Data Structures
Go beyond basic data structures and implement more complex ones like:
- Segment Trees
- Fenwick Trees (Binary Indexed Trees)
- Trie (Prefix Tree)
- Disjoint Set Union (Union-Find)
Understanding these structures can provide powerful tools for solving certain classes of problems efficiently.
6. Practice Problem Reduction
Hone your ability to reduce one problem to another. This skill allows you to leverage known efficient solutions for seemingly unrelated problems.
7. Study Bit Manipulation Techniques
Mastering bit manipulation can lead to highly optimized solutions for certain problems. Practice problems that involve bitwise operations and learn common bit manipulation tricks.
Leveraging AI for Algorithmic Intuition Development
In the age of artificial intelligence, several AI-powered tools can aid in developing algorithmic intuition:
1. AI-Powered Code Assistants
Tools like GitHub Copilot or TabNine can suggest code completions and even entire functions. While these shouldn’t be relied upon exclusively, they can expose you to different coding patterns and approaches.
2. Automated Problem Generators
Some platforms use AI to generate unique coding problems based on your skill level and areas for improvement. This can provide a more personalized learning experience.
3. AI-Driven Code Review
AI systems can analyze your code for efficiency and suggest optimizations. This immediate feedback can help reinforce good practices and identify areas for improvement.
4. Natural Language Problem Solving
Experiment with describing algorithmic solutions in natural language to AI models like GPT-3. This can help you practice articulating your thought process and may even provide insights into alternative approaches.
Conclusion
Algorithmic intuition is a powerful skill that can significantly enhance your performance in technical interviews and your overall capabilities as a software engineer. It’s not an innate talent, but rather a skill that can be developed through consistent practice, exposure to diverse problems, and a deep understanding of fundamental concepts.
Remember that developing strong algorithmic intuition is a journey, not a destination. Even experienced engineers continue to refine and expand their intuition throughout their careers. Embrace the learning process, stay curious, and always be open to new approaches and perspectives.
As you prepare for technical interviews, particularly those at top tech companies, focus on not just solving problems, but understanding the underlying patterns and principles. Cultivate the ability to quickly recognize problem types, consider multiple approaches, and articulate your reasoning clearly.
With dedication and the right approach, you can develop the kind of algorithmic intuition that will not only help you excel in interviews but also make you a more effective and efficient problem solver in your professional career. Happy coding, and best of luck in your interview preparation!