75 Strategies for Substring and Subsequence Problems: Mastering String Manipulation
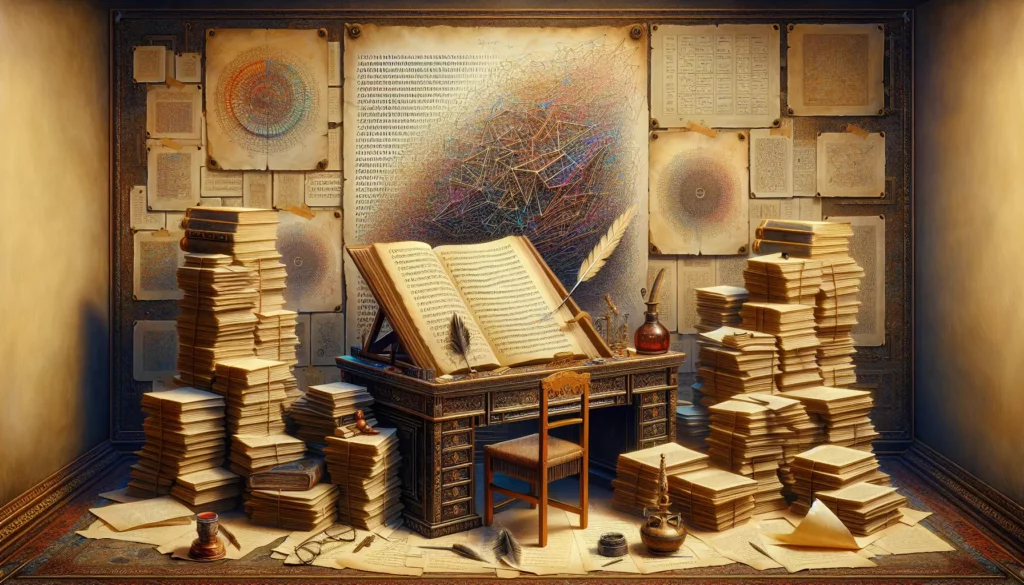
Welcome to our comprehensive guide on strategies for tackling substring and subsequence problems in coding interviews and algorithmic challenges. As part of our AlgoCademy series, this article aims to equip you with the knowledge and techniques necessary to excel in string manipulation tasks, a crucial skill for any programmer preparing for technical interviews at top tech companies.
String manipulation, particularly working with substrings and subsequences, is a fundamental concept in computer science and a common theme in coding interviews. Whether you’re a beginner looking to build a strong foundation or an experienced developer aiming to refine your skills, this guide will provide you with a wealth of strategies to approach these problems efficiently.
Table of Contents
- Understanding the Basics
- The Sliding Window Technique
- Two Pointers Approach
- Dynamic Programming for Subsequences
- Hashing and Hash Tables
- KMP Algorithm for Pattern Matching
- Suffix Arrays and LCP Arrays
- Trie Data Structure
- Rabin-Karp Algorithm
- Manacher’s Algorithm for Palindromes
- Z Algorithm for Pattern Matching
- Bit Manipulation Techniques
- Divide and Conquer Strategies
- Greedy Algorithms for Substrings
- Binary Search on Strings
1. Understanding the Basics
Before diving into advanced strategies, it’s crucial to have a solid grasp of the fundamental concepts:
Substring vs. Subsequence
- Substring: A contiguous sequence of characters within a string.
- Subsequence: A sequence of characters in the same relative order but not necessarily contiguous.
For example, in the string “algorithm”:
- “gor” is a substring
- “agtm” is a subsequence, but not a substring
Basic String Operations
Familiarize yourself with basic string operations in your preferred programming language:
// Python example
s = "algorithm"
print(s[2:5]) # Output: gor
print(s[::-1]) # Output: mhtirogla (reverse)
print(len(s)) # Output: 9
print(s.find("ith")) # Output: 5
2. The Sliding Window Technique
The sliding window technique is a powerful method for solving substring problems, especially when dealing with contiguous sequences.
Key Concepts:
- Maintain a window that expands or contracts
- Use two pointers: start and end of the window
- Efficiently process substrings in linear time
Example Problem: Longest Substring Without Repeating Characters
def longest_substring_without_repeats(s):
char_set = set()
max_length = 0
left = right = 0
while right < len(s):
if s[right] not in char_set:
char_set.add(s[right])
max_length = max(max_length, right - left + 1)
right += 1
else:
char_set.remove(s[left])
left += 1
return max_length
# Test
print(longest_substring_without_repeats("abcabcbb")) # Output: 3
3. Two Pointers Approach
The two pointers technique is versatile and can be applied to various string problems, especially those involving palindromes or comparing characters at different positions.
Key Concepts:
- Use two pointers that move towards each other or in the same direction
- Efficiently compare characters or substrings
- Often used in conjunction with other techniques
Example Problem: Valid Palindrome
def is_palindrome(s):
left, right = 0, len(s) - 1
while left < right:
while left < right and not s[left].isalnum():
left += 1
while left < right and not s[right].isalnum():
right -= 1
if s[left].lower() != s[right].lower():
return False
left += 1
right -= 1
return True
# Test
print(is_palindrome("A man, a plan, a canal: Panama")) # Output: True
4. Dynamic Programming for Subsequences
Dynamic Programming (DP) is a powerful technique for solving subsequence problems, especially when optimal substructure and overlapping subproblems are present.
Key Concepts:
- Break down the problem into smaller subproblems
- Store and reuse solutions to subproblems
- Build up the solution iteratively or recursively
Example Problem: Longest Common Subsequence
def longest_common_subsequence(text1, text2):
m, n = len(text1), len(text2)
dp = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if text1[i-1] == text2[j-1]:
dp[i][j] = dp[i-1][j-1] + 1
else:
dp[i][j] = max(dp[i-1][j], dp[i][j-1])
return dp[m][n]
# Test
print(longest_common_subsequence("abcde", "ace")) # Output: 3
5. Hashing and Hash Tables
Hashing is an essential technique for quickly storing and retrieving string information, making it valuable for various substring and subsequence problems.
Key Concepts:
- Use hash functions to map strings to integer values
- Employ hash tables for O(1) average-case lookup time
- Handle collisions effectively
Example Problem: Group Anagrams
from collections import defaultdict
def group_anagrams(strs):
anagram_groups = defaultdict(list)
for s in strs:
sorted_s = ''.join(sorted(s))
anagram_groups[sorted_s].append(s)
return list(anagram_groups.values())
# Test
print(group_anagrams(["eat", "tea", "tan", "ate", "nat", "bat"]))
# Output: [['eat', 'tea', 'ate'], ['tan', 'nat'], ['bat']]
6. KMP Algorithm for Pattern Matching
The Knuth-Morris-Pratt (KMP) algorithm is an efficient string matching algorithm that utilizes the failure function to avoid unnecessary comparisons.
Key Concepts:
- Preprocess the pattern to compute the failure function
- Use the failure function to skip unnecessary comparisons
- Achieve O(n + m) time complexity for pattern matching
Example Implementation:
def kmp_search(text, pattern):
def compute_lps(pattern):
lps = [0] * len(pattern)
length = 0
i = 1
while i < len(pattern):
if pattern[i] == pattern[length]:
length += 1
lps[i] = length
i += 1
elif length != 0:
length = lps[length - 1]
else:
lps[i] = 0
i += 1
return lps
lps = compute_lps(pattern)
i = j = 0
while i < len(text):
if pattern[j] == text[i]:
i += 1
j += 1
if j == len(pattern):
return i - j
elif j != 0:
j = lps[j - 1]
else:
i += 1
return -1
# Test
print(kmp_search("ABABDABACDABABCABAB", "ABABCABAB")) # Output: 10
7. Suffix Arrays and LCP Arrays
Suffix arrays and Longest Common Prefix (LCP) arrays are powerful data structures for efficient string processing, particularly useful for substring-related problems.
Key Concepts:
- Suffix array: sorted array of all suffixes of a string
- LCP array: lengths of longest common prefixes between adjacent suffixes in the suffix array
- Enables efficient substring searches and comparisons
Example: Building a Suffix Array
def build_suffix_array(s):
suffixes = [(s[i:], i) for i in range(len(s))]
suffixes.sort()
return [suffix[1] for suffix in suffixes]
# Test
print(build_suffix_array("banana")) # Output: [5, 3, 1, 0, 4, 2]
8. Trie Data Structure
A Trie (prefix tree) is an efficient data structure for storing and searching strings, particularly useful for problems involving prefixes and word dictionaries.
Key Concepts:
- Tree-like structure where each node represents a character
- Efficient for prefix-based operations
- Space-efficient for storing large sets of strings with common prefixes
Example: Implementing a Trie
class TrieNode:
def __init__(self):
self.children = {}
self.is_end = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
# Test
trie = Trie()
trie.insert("apple")
print(trie.search("apple")) # Output: True
print(trie.search("app")) # Output: False
print(trie.starts_with("app")) # Output: True
9. Rabin-Karp Algorithm
The Rabin-Karp algorithm is a string-searching algorithm that uses hashing to find patterns in strings.
Key Concepts:
- Calculate hash values for the pattern and substrings of the text
- Use rolling hash technique for efficient hash computation
- Useful for multiple pattern searching
Example Implementation:
def rabin_karp(text, pattern):
n, m = len(text), len(pattern)
if m > n:
return -1
prime = 101
d = 256
pattern_hash = 0
text_hash = 0
h = 1
for i in range(m-1):
h = (h * d) % prime
for i in range(m):
pattern_hash = (d * pattern_hash + ord(pattern[i])) % prime
text_hash = (d * text_hash + ord(text[i])) % prime
for i in range(n - m + 1):
if pattern_hash == text_hash:
if text[i:i+m] == pattern:
return i
if i < n - m:
text_hash = (d * (text_hash - ord(text[i]) * h) + ord(text[i + m])) % prime
if text_hash < 0:
text_hash += prime
return -1
# Test
print(rabin_karp("ABABDABACDABABCABAB", "ABABCABAB")) # Output: 10
10. Manacher’s Algorithm for Palindromes
Manacher’s algorithm is an efficient technique for finding all palindromic substrings in a string in linear time.
Key Concepts:
- Transform the string to handle even-length palindromes
- Use previously computed information to avoid redundant computations
- Achieve O(n) time complexity for finding all palindromic substrings
Example Implementation:
def manacher(s):
t = '#' + '#'.join(s) + '#'
n = len(t)
p = [0] * n
c = r = 0
for i in range(n):
mirror = 2 * c - i
if i < r:
p[i] = min(r - i, p[mirror])
while i + 1 + p[i] < n and i - 1 - p[i] >= 0 and t[i + 1 + p[i]] == t[i - 1 - p[i]]:
p[i] += 1
if i + p[i] > r:
c, r = i, i + p[i]
max_len, center = max((p[i], i) for i in range(n))
start = (center - max_len) // 2
return s[start:start + max_len]
# Test
print(manacher("babad")) # Output: "bab" or "aba"
11. Z Algorithm for Pattern Matching
The Z algorithm is a linear-time string matching algorithm that can be used to find all occurrences of a pattern in a text.
Key Concepts:
- Construct the Z array, which stores the length of the longest substring starting from each index that is also a prefix of the string
- Use the Z array to efficiently find pattern matches
- Achieve O(n + m) time complexity for pattern matching
Example Implementation:
def z_function(s):
n = len(s)
z = [0] * n
l, r = 0, 0
for i in range(1, n):
if i <= r:
z[i] = min(r - i + 1, z[i - l])
while i + z[i] < n and s[z[i]] == s[i + z[i]]:
z[i] += 1
if i + z[i] - 1 > r:
l, r = i, i + z[i] - 1
return z
def z_algorithm_search(text, pattern):
combined = pattern + "$" + text
z = z_function(combined)
return [i - len(pattern) - 1 for i in range(len(pattern) + 1, len(combined)) if z[i] == len(pattern)]
# Test
print(z_algorithm_search("ABABDABACDABABCABAB", "ABABCABAB")) # Output: [10]
12. Bit Manipulation Techniques
Bit manipulation can be a powerful tool for certain string problems, especially when dealing with character sets or optimizing space usage.
Key Concepts:
- Use bitwise operations to represent and manipulate character sets
- Optimize space usage by encoding information in bits
- Perform fast operations on small alphabets
Example: Check if a string has all unique characters
def has_unique_chars(s):
seen = 0
for char in s:
val = ord(char) - ord('a')
if seen & (1 << val):
return False
seen |= (1 << val)
return True
# Test
print(has_unique_chars("abcdefg")) # Output: True
print(has_unique_chars("abcdefga")) # Output: False
13. Divide and Conquer Strategies
Divide and conquer is a problem-solving paradigm that can be applied to various string problems, especially those involving recursion or splitting the problem into smaller subproblems.
Key Concepts:
- Break down the problem into smaller, manageable subproblems
- Solve the subproblems recursively
- Combine the solutions to subproblems to solve the original problem
Example: Longest Palindromic Subsequence
def longest_palindromic_subsequence(s):
def lps(s, i, j):
if i > j:
return 0
if i == j:
return 1
if s[i] == s[j]:
return lps(s, i+1, j-1) + 2
return max(lps(s, i+1, j), lps(s, i, j-1))
return lps(s, 0, len(s) - 1)
# Test
print(longest_palindromic_subsequence("bbbab")) # Output: 4
14. Greedy Algorithms for Substrings
Greedy algorithms can be effective for certain substring problems where making locally optimal choices leads to a globally optimal solution.
Key Concepts:
- Make the locally optimal choice at each step
- Hope that these local choices lead to a global optimum
- Often used in optimization problems
Example: Minimum Window Substring
from collections import Counter
def min_window(s, t):
need = Counter(t)
missing = len(t)
left = start = end = 0
for right, char in enumerate(s, 1):
if need[char] > 0:
missing -= 1
need[char] -= 1
if missing == 0:
while left < right and need[s[left]] < 0:
need[s[left]] += 1
left += 1
if not end or right - left <= end - start:
start, end = left, right
need[s[left]] += 1
missing += 1
left += 1
return s[start:end]
# Test
print(min_window("ADOBECODEBANC", "ABC")) # Output: "BANC"
15. Binary Search on Strings
Binary search can be applied to string problems, especially when dealing with sorted strings or when searching for a specific property in a range of substrings.
Key Concepts:
- Apply binary search on the length or index of substrings
- Use binary search to find the optimal substring satisfying certain conditions
- Combine with other techniques for efficient solutions
Example: Longest Repeating Substring
def longest_repeating_substring(s):
def is_repeating(length):
seen = set()
for i in range(len(s) - length + 1):
substr = s[i:i+length]
if substr in seen:
return True
seen.add(substr)
return False
left, right = 1, len(s)
while left <= right:
mid = (left + right) // 2
if is_repeating(mid):
left = mid + 1
else:
right = mid - 1
return right
# Test
print(longest_repeating_substring("abcabcabc")) # Output: 6
Conclusion
Mastering these 15 strategies for substring and subsequence problems will significantly enhance your ability to tackle a wide range of string manipulation challenges. Remember that the key to success in coding interviews and algorithmic problem-solving is not just knowing these techniques, but also understanding when and how to apply them effectively.
As you practice with AlgoCademy, you’ll encounter various problems that will help you refine your skills in applying these strategies. Don’t forget to leverage our AI-powered assistance and step-by-step guidance to deepen your understanding and improve your problem-solving capabilities.
Keep practicing, stay curious, and happy coding!