How to Prepare for AI and Machine Learning Questions in Tech Interviews
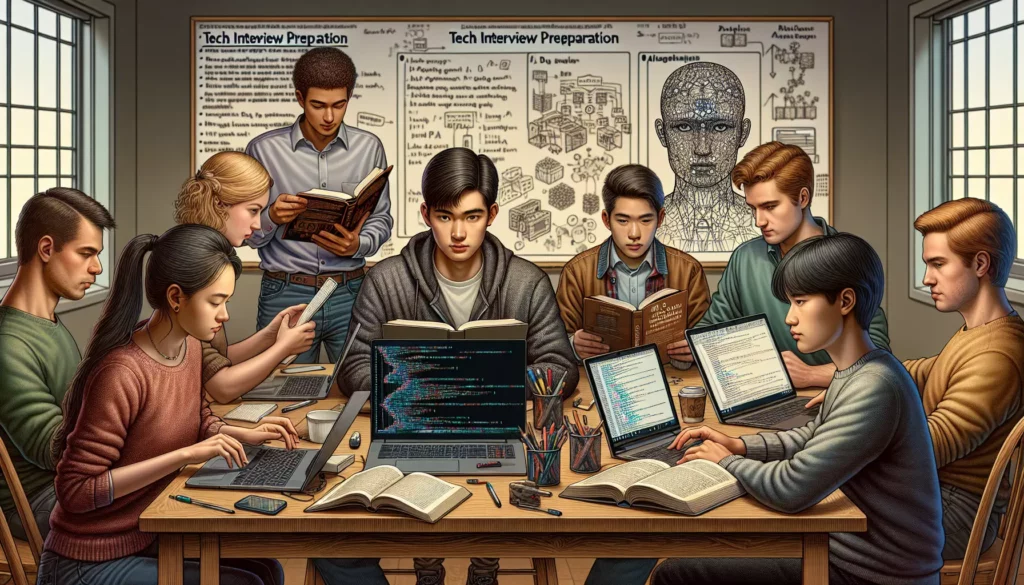
As artificial intelligence (AI) and machine learning (ML) continue to revolutionize the tech industry, more companies are incorporating these topics into their interview processes. Whether you’re aiming for a position at a FAANG company (Facebook/Meta, Amazon, Apple, Netflix, Google) or any tech firm leveraging AI and ML, being well-prepared for these questions is crucial. In this comprehensive guide, we’ll explore effective strategies to help you ace AI and ML questions in your next tech interview.
1. Understand the Fundamentals
Before diving into complex AI and ML concepts, it’s essential to have a solid grasp of the fundamentals. This includes:
- Basic statistics and probability
- Linear algebra
- Calculus
- Programming skills (particularly in Python or R)
- Data structures and algorithms
These foundational skills form the bedrock of AI and ML. Make sure you’re comfortable with concepts like:
- Descriptive statistics (mean, median, mode, standard deviation)
- Probability distributions (normal, binomial, Poisson)
- Matrix operations
- Derivatives and gradients
- Basic data structures (arrays, linked lists, trees, graphs)
- Common algorithms (sorting, searching, graph traversal)
2. Familiarize Yourself with Key AI and ML Concepts
Once you have a strong foundation, focus on understanding the core concepts of AI and ML. Some key areas to study include:
2.1 Machine Learning Algorithms
- Supervised Learning:
- Linear Regression
- Logistic Regression
- Decision Trees
- Random Forests
- Support Vector Machines (SVM)
- Unsupervised Learning:
- K-means Clustering
- Hierarchical Clustering
- Principal Component Analysis (PCA)
- Reinforcement Learning:
- Q-Learning
- Policy Gradient Methods
2.2 Deep Learning
- Neural Network Architectures
- Convolutional Neural Networks (CNNs)
- Recurrent Neural Networks (RNNs)
- Long Short-Term Memory (LSTM) Networks
- Transformers and Attention Mechanisms
2.3 Natural Language Processing (NLP)
- Text preprocessing techniques
- Tokenization
- Named Entity Recognition (NER)
- Sentiment Analysis
- Language Models (e.g., BERT, GPT)
2.4 Computer Vision
- Image preprocessing
- Object Detection
- Image Segmentation
- Face Recognition
3. Practice Implementing Algorithms
Theory is important, but practical implementation is crucial. Practice coding AI and ML algorithms from scratch. This will deepen your understanding and prepare you for coding challenges during interviews. Here’s a simple example of implementing a basic linear regression using Python:
import numpy as np
def linear_regression(X, y):
# Add bias term
X_b = np.c_[np.ones((X.shape[0], 1)), X]
# Calculate coefficients
theta = np.linalg.inv(X_b.T.dot(X_b)).dot(X_b.T).dot(y)
return theta
# Example usage
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 5, 4, 5])
theta = linear_regression(X, y)
print("Intercept:", theta[0])
print("Slope:", theta[1])
This simple implementation helps you understand the mathematics behind linear regression and how to translate it into code.
4. Understand Model Evaluation and Validation
Knowing how to evaluate and validate machine learning models is crucial. Familiarize yourself with:
- Cross-validation techniques
- Metrics for classification (accuracy, precision, recall, F1-score)
- Metrics for regression (Mean Squared Error, R-squared)
- ROC curves and AUC
- Confusion matrices
Here’s an example of how to calculate accuracy and create a confusion matrix using scikit-learn:
from sklearn.metrics import accuracy_score, confusion_matrix
import numpy as np
# Assuming y_true and y_pred are your true and predicted labels
y_true = np.array([0, 1, 1, 0, 1, 0])
y_pred = np.array([0, 1, 0, 0, 1, 1])
# Calculate accuracy
accuracy = accuracy_score(y_true, y_pred)
print("Accuracy:", accuracy)
# Create confusion matrix
cm = confusion_matrix(y_true, y_pred)
print("Confusion Matrix:")
print(cm)
5. Stay Updated with Recent Advancements
AI and ML are rapidly evolving fields. Stay current with the latest developments by:
- Reading research papers on arXiv
- Following AI/ML conferences (NeurIPS, ICML, CVPR)
- Subscribing to AI/ML newsletters and blogs
- Participating in online communities (Reddit r/MachineLearning, Stack Overflow)
Being aware of recent breakthroughs like GPT-3, DALL-E, or advancements in reinforcement learning can impress interviewers and demonstrate your passion for the field.
6. Prepare for Common Interview Questions
While every interview is different, some questions come up frequently. Prepare answers for questions like:
- Explain the difference between supervised and unsupervised learning.
- What is overfitting, and how can you prevent it?
- Describe the process of feature selection and its importance.
- How does backpropagation work in neural networks?
- Explain the concept of regularization in machine learning.
- What is the bias-variance tradeoff?
- How do you handle imbalanced datasets?
Practice articulating your answers clearly and concisely. Use examples to illustrate your points when possible.
7. Work on Projects and Build a Portfolio
Practical experience is invaluable. Work on personal projects or contribute to open-source AI/ML projects. This will not only enhance your skills but also provide concrete examples to discuss during interviews. Some project ideas include:
- Developing a sentiment analysis tool for social media posts
- Creating an image classification model for a specific domain (e.g., plant species identification)
- Building a recommendation system for movies or products
- Implementing a chatbot using natural language processing techniques
Document your projects on platforms like GitHub, and be prepared to discuss your approach, challenges faced, and lessons learned during interviews.
8. Practice with Mock Interviews
Participate in mock interviews to get comfortable with the interview process. You can:
- Use platforms like LeetCode or HackerRank for coding practice
- Join AI/ML study groups or find a study partner for mock interviews
- Record yourself answering questions to improve your communication skills
Pay attention to your problem-solving process, as interviewers are often more interested in how you approach a problem than whether you get the perfect answer immediately.
9. Understand the Company and Role
Research the company you’re interviewing with and the specific role you’re applying for. Different companies may emphasize different aspects of AI and ML. For example:
- Google might focus more on large-scale machine learning and search algorithms
- Facebook (Meta) might emphasize social network analysis and recommendation systems
- Amazon could focus on natural language processing for Alexa or machine learning for product recommendations
Tailor your preparation to align with the company’s focus areas and the job description.
10. Develop Your Soft Skills
While technical skills are crucial, don’t neglect soft skills. AI and ML roles often involve:
- Collaborating with cross-functional teams
- Communicating complex ideas to non-technical stakeholders
- Problem-solving in ambiguous situations
- Staying adaptable in a rapidly changing field
Prepare examples that demonstrate these skills from your past experiences or projects.
11. Be Ready to Discuss Ethical Considerations
As AI and ML technologies become more pervasive, ethical considerations are increasingly important. Be prepared to discuss topics such as:
- Bias in AI systems and how to mitigate it
- Privacy concerns in data collection and usage
- Transparency and explainability of AI models
- Potential societal impacts of AI and ML technologies
Demonstrating awareness of these issues shows maturity and responsibility as an AI/ML practitioner.
12. Practice Explaining Complex Concepts Simply
A key skill for AI and ML professionals is the ability to explain complex concepts in simple terms. Practice explaining AI and ML concepts to friends or family members who aren’t in the tech field. This will help you develop the skill of breaking down complex ideas into understandable components, which is valuable both in interviews and on the job.
13. Familiarize Yourself with AI/ML Tools and Frameworks
While understanding the underlying concepts is crucial, familiarity with popular AI and ML tools and frameworks is also important. Some key ones to know include:
- TensorFlow and Keras
- PyTorch
- Scikit-learn
- Pandas for data manipulation
- NumPy for numerical computing
- Matplotlib and Seaborn for data visualization
Here’s a simple example of using TensorFlow to create a basic neural network:
import tensorflow as tf
from tensorflow.keras import layers
model = tf.keras.Sequential([
layers.Dense(64, activation='relu', input_shape=(10,)),
layers.Dense(64, activation='relu'),
layers.Dense(1)
])
model.compile(optimizer='adam',
loss='mean_squared_error',
metrics=['mae'])
# Assuming X_train and y_train are your training data
model.fit(X_train, y_train, epochs=100, batch_size=32, validation_split=0.2)
Being able to quickly prototype models using these tools can be a valuable skill in interviews and on the job.
14. Understand Big Data and Distributed Computing
Many AI and ML applications deal with large datasets that require distributed computing. Familiarize yourself with concepts and tools like:
- Hadoop and MapReduce
- Apache Spark
- Distributed training of neural networks
- Cloud computing platforms (AWS, Google Cloud, Azure)
Understanding how to scale AI and ML solutions is increasingly important as datasets grow larger and models become more complex.
15. Prepare to Discuss Real-World Applications
Be ready to discuss how AI and ML are applied in real-world scenarios. This could include:
- Recommendation systems in e-commerce or streaming services
- Fraud detection in financial services
- Predictive maintenance in manufacturing
- Disease diagnosis in healthcare
- Autonomous vehicles in transportation
Being able to connect theoretical concepts to practical applications demonstrates a deeper understanding of the field and its potential impact.
Conclusion
Preparing for AI and machine learning questions in tech interviews requires a multifaceted approach. It involves building a strong foundation in the fundamentals, understanding key AI and ML concepts, gaining practical experience through projects, staying updated with the latest advancements, and developing both technical and soft skills.
Remember that interviewers are not just looking for someone who can recite algorithms or implement models. They want to see how you think, how you approach problems, and how you can apply your knowledge to real-world situations. By following this comprehensive guide and consistently practicing, you’ll be well-prepared to tackle AI and ML questions in your next tech interview.
Lastly, don’t forget to showcase your passion for the field. AI and ML are exciting and rapidly evolving areas, and demonstrating genuine enthusiasm can set you apart from other candidates. Good luck with your preparation and upcoming interviews!