The Use of Pseudocode in Structuring Your Solutions
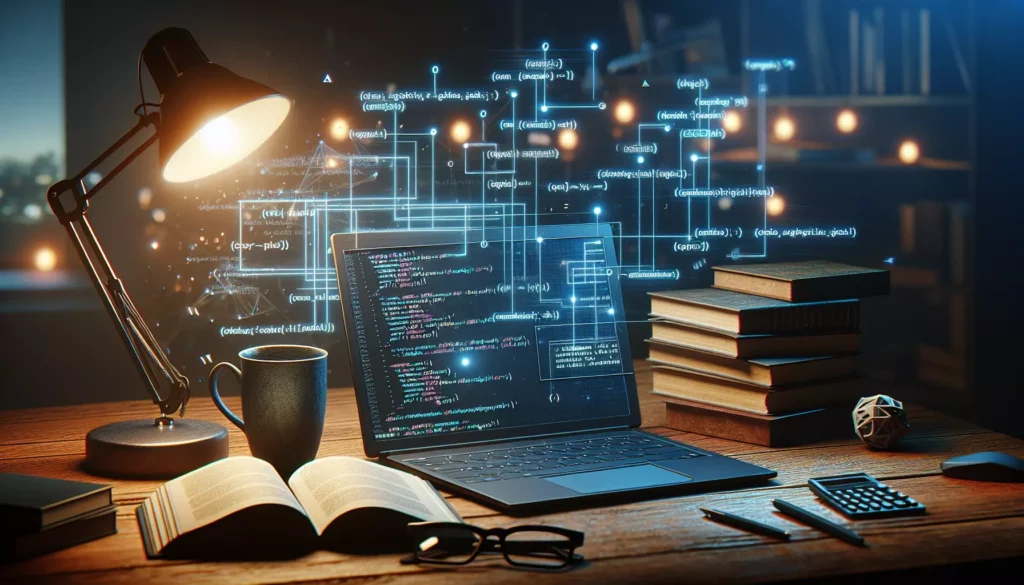
In the world of programming and algorithmic problem-solving, one of the most valuable tools at a developer’s disposal is pseudocode. This powerful technique bridges the gap between human thought processes and machine-executable code, serving as an essential stepping stone in the journey from concept to implementation. In this comprehensive guide, we’ll explore the ins and outs of pseudocode, its importance in structuring solutions, and how it can significantly enhance your coding skills and problem-solving abilities.
What is Pseudocode?
Pseudocode is a informal, high-level description of a computer program or algorithm. It uses structured English-like statements to outline the logic and flow of a program without adhering to the strict syntax rules of any particular programming language. The primary purpose of pseudocode is to create a readable and easily understandable outline of a program’s logic before diving into the actual coding process.
Unlike actual code, pseudocode is not meant to be executed by a computer. Instead, it serves as a planning tool and a communication medium between programmers, allowing them to focus on the problem-solving aspect of programming without getting bogged down in language-specific details.
The Importance of Pseudocode in Problem-Solving
Pseudocode plays a crucial role in the problem-solving process for several reasons:
- Clarity of Thought: Writing pseudocode forces you to think through the logic of your solution step-by-step, helping to clarify your thoughts and identify potential issues early in the development process.
- Language Independence: Since pseudocode is not tied to any specific programming language, it allows you to focus on the algorithm itself rather than syntax details.
- Easy Modification: It’s much simpler to modify and refine your approach when working with pseudocode compared to fully written code.
- Collaboration Tool: Pseudocode serves as an excellent medium for discussing and sharing ideas with team members or mentors.
- Documentation: Well-written pseudocode can serve as documentation for your algorithm, making it easier for others (or yourself in the future) to understand the logic behind your solution.
Key Elements of Effective Pseudocode
While there’s no strict standard for writing pseudocode, there are some common elements and best practices that can make your pseudocode more effective:
- Use of Indentation: Proper indentation helps to show the structure and hierarchy of your algorithm, making it easier to follow the flow of logic.
- Clear and Concise Statements: Each line of pseudocode should represent a single action or decision in your algorithm.
- Use of Control Structures: Incorporate familiar programming constructs like loops, conditionals, and function calls to outline the flow of your algorithm.
- Consistency: Maintain a consistent style throughout your pseudocode to enhance readability.
- Appropriate Level of Detail: Strike a balance between being too vague and too specific. Your pseudocode should provide enough detail to understand the logic without delving into language-specific implementation details.
Common Pseudocode Conventions
While pseudocode doesn’t follow strict syntax rules, there are some common conventions that many programmers use:
- INPUT: Used to indicate data input
- OUTPUT: Used to indicate data output
- IF…THEN…ELSE: For conditional statements
- WHILE: For while loops
- FOR: For for loops
- FUNCTION: To define functions or procedures
- RETURN: To indicate a return value from a function
- //: For comments
Example: Writing Pseudocode for a Simple Problem
Let’s walk through the process of writing pseudocode for a simple problem: finding the maximum number in an array.
FUNCTION findMaximum(arr)
IF arr is empty THEN
RETURN null
END IF
SET max = first element of arr
FOR each element in arr
IF element > max THEN
SET max = element
END IF
END FOR
RETURN max
END FUNCTION
// Main program
INPUT array of numbers
CALL findMaximum with array as argument
OUTPUT the result
This pseudocode outlines the logic for finding the maximum number in an array. It’s easy to read and understand, and it can be easily translated into any programming language.
From Pseudocode to Code
Once you have your pseudocode, translating it into actual code becomes much easier. Let’s see how our pseudocode example could be implemented in Python:
def find_maximum(arr):
if not arr:
return None
max_num = arr[0]
for num in arr:
if num > max_num:
max_num = num
return max_num
# Main program
numbers = input("Enter a list of numbers separated by spaces: ").split()
numbers = [int(num) for num in numbers] # Convert string inputs to integers
result = find_maximum(numbers)
print(f"The maximum number is: {result}")
As you can see, the structure of the code closely follows the pseudocode, making the implementation process straightforward.
Pseudocode in Technical Interviews
Pseudocode is particularly valuable in technical interviews, especially when applying for positions at major tech companies. Here’s why:
- Demonstrates Thought Process: Writing pseudocode allows you to show your problem-solving approach and thought process to the interviewer.
- Facilitates Discussion: It provides a clear outline that you can discuss with the interviewer, allowing for collaborative problem-solving.
- Helps Manage Time: By quickly sketching out your approach in pseudocode, you can ensure you have a solid plan before diving into actual coding.
- Showcases Planning Skills: Using pseudocode demonstrates your ability to plan and structure your solutions effectively, a crucial skill for any developer.
Best Practices for Using Pseudocode
To make the most of pseudocode in your problem-solving toolkit, consider the following best practices:
- Start with High-Level Steps: Begin by outlining the main steps of your algorithm before diving into details.
- Refine Iteratively: Start with a rough outline and gradually refine your pseudocode as you clarify your thoughts.
- Use Meaningful Variable Names: Choose clear and descriptive names for variables and functions in your pseudocode.
- Include Error Handling: Consider and document potential error cases in your pseudocode.
- Practice Regularly: The more you use pseudocode, the more natural and effective it becomes.
- Review and Refine: After implementing your solution, compare it with your pseudocode and refine your pseudocode writing skills based on what you’ve learned.
Common Pitfalls to Avoid
While pseudocode is a powerful tool, there are some common mistakes to watch out for:
- Being Too Vague: While pseudocode shouldn’t be as detailed as actual code, it should still provide enough information to guide your implementation.
- Being Too Language-Specific: Avoid using syntax or constructs that are specific to a particular programming language.
- Neglecting Edge Cases: Make sure your pseudocode accounts for potential edge cases and error conditions.
- Overcomplicating: Keep your pseudocode as simple and straightforward as possible while still capturing the essential logic of your algorithm.
- Ignoring Efficiency: While pseudocode doesn’t need to be optimized, it’s good to consider efficiency in your high-level approach.
Pseudocode and Algorithmic Thinking
Pseudocode is not just a tool for planning code; it’s also an excellent way to develop and refine your algorithmic thinking skills. By regularly practicing pseudocode writing, you can:
- Improve Problem Decomposition: Break down complex problems into smaller, manageable steps.
- Enhance Logical Thinking: Develop a more structured approach to problem-solving.
- Boost Abstraction Skills: Learn to focus on the essential aspects of a problem without getting lost in implementation details.
- Increase Efficiency: Identify potential optimizations and improvements in your algorithms before writing any code.
Advanced Pseudocode Techniques
As you become more comfortable with basic pseudocode, you can incorporate more advanced techniques to make your pseudocode even more effective:
- Modularization: Break your pseudocode into smaller, reusable modules or functions to improve readability and reusability.
- Data Structure Representation: Use simple representations of complex data structures in your pseudocode to clarify how data is being stored and manipulated.
- Time and Space Complexity Annotations: Include brief notes about the time and space complexity of different parts of your algorithm.
- Parallel Processing Notation: For algorithms that involve parallelism, use notation to indicate which parts can be executed concurrently.
- State Diagrams: For complex algorithms, consider incorporating simple state diagrams alongside your pseudocode to visualize the flow of your algorithm.
Tools and Resources for Pseudocode
While pseudocode is typically written by hand or in a simple text editor, there are some tools and resources that can aid in the process:
- Flowchart Tools: Tools like Lucidchart or Draw.io can be used to create visual representations of your pseudocode.
- Text Editors with Syntax Highlighting: Some text editors offer pseudocode syntax highlighting, which can make your pseudocode more readable.
- Online Pseudocode Editors: Websites like PseudoCode.io provide environments specifically designed for writing and sharing pseudocode.
- Pseudocode to Code Converters: While not always accurate, these tools can provide a starting point for converting your pseudocode to actual code.
- Pseudocode Style Guides: Many educational institutions and tech companies have their own pseudocode style guides, which can be valuable references.
Pseudocode in Team Collaboration
Pseudocode isn’t just valuable for individual problem-solving; it’s also an excellent tool for team collaboration:
- Design Discussions: Use pseudocode to outline and discuss high-level designs with team members before implementation.
- Code Reviews: Including pseudocode in your code comments can help reviewers understand your logic more quickly.
- Documentation: Pseudocode can serve as a form of documentation, helping new team members understand the logic behind complex algorithms.
- Cross-Functional Communication: Pseudocode can help bridge the gap between technical and non-technical team members by providing a more accessible representation of algorithms.
Conclusion
Pseudocode is a powerful tool in the programmer’s arsenal, bridging the gap between human thought processes and machine-executable code. By mastering the art of writing clear, concise, and effective pseudocode, you can significantly enhance your problem-solving skills, improve your coding efficiency, and become a more effective communicator in the world of software development.
Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, incorporating pseudocode into your problem-solving routine can yield significant benefits. It forces you to think through your solutions thoroughly, helps you identify potential issues early, and provides a clear roadmap for implementation.
Remember, the goal of pseudocode is not to write perfect, executable code, but to create a clear, logical outline of your solution. With practice, you’ll find that writing pseudocode becomes second nature, and you’ll be able to tackle even the most complex programming challenges with confidence and clarity.
So the next time you’re faced with a coding problem, take a step back, grab a pen and paper (or open your favorite text editor), and start sketching out your solution in pseudocode. You might be surprised at how much it clarifies your thinking and streamlines your coding process. Happy coding!