How to Interpret Complex Problem Statements Quickly: A Comprehensive Guide
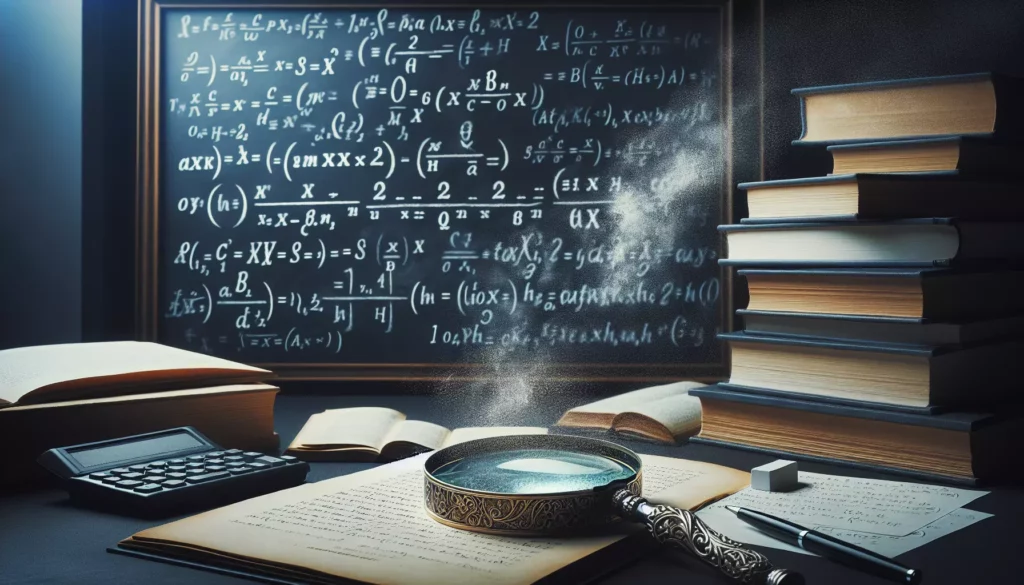
In the world of coding and software development, the ability to quickly and accurately interpret complex problem statements is a crucial skill. Whether you’re preparing for technical interviews at top tech companies or working on challenging projects, mastering this skill can significantly boost your problem-solving capabilities. In this comprehensive guide, we’ll explore effective strategies and techniques to help you decode and understand complex problem statements with speed and precision.
1. Understanding the Importance of Problem Statement Interpretation
Before diving into the techniques, it’s essential to recognize why interpreting problem statements quickly is so vital:
- Time Management: In coding interviews and timed coding challenges, every second counts. The faster you can understand the problem, the more time you’ll have to devise and implement a solution.
- Accuracy: Misinterpreting a problem can lead to incorrect solutions, wasting valuable time and effort.
- Efficiency: Quick interpretation allows you to focus on problem-solving rather than getting stuck on understanding the question.
- Confidence: Being able to rapidly grasp complex problems boosts your confidence, reducing anxiety during high-pressure situations like interviews.
2. Breaking Down the Problem Statement
The first step in interpreting complex problem statements is to break them down into manageable parts:
2.1. Identify Key Components
Look for these essential elements in the problem statement:
- Input: What data or information is provided?
- Output: What is the expected result or solution?
- Constraints: Are there any limitations or specific conditions?
- Examples: Are sample inputs and outputs provided?
2.2. Highlight Keywords and Phrases
Pay special attention to words that indicate:
- Data structures (e.g., array, linked list, tree)
- Algorithms (e.g., sorting, searching, dynamic programming)
- Time or space complexity requirements
- Special conditions or edge cases
2.3. Visualize the Problem
Create a mental image or quick sketch of the problem. This can help you understand the relationships between different elements and see the big picture.
3. Techniques for Quick Interpretation
Now that we’ve covered the basics, let’s explore some advanced techniques to speed up your interpretation process:
3.1. Skimming and Scanning
Develop the ability to quickly skim through the problem statement to get an overall idea, then scan for specific details:
- Skimming: Read the first and last sentences of each paragraph to grasp the main ideas.
- Scanning: Look for key terms, numbers, and special characters that might indicate important information.
3.2. Active Reading
Engage with the text actively to improve comprehension and retention:
- Ask yourself questions as you read
- Summarize each paragraph in your own words
- Make notes or annotations
3.3. Pattern Recognition
Train yourself to recognize common patterns in problem statements:
- Identify similarities to problems you’ve encountered before
- Look for clues that suggest specific algorithmic approaches
- Recognize standard problem types (e.g., optimization, graph traversal)
3.4. Chunking Information
Group related pieces of information together to make them easier to process and remember:
- Organize constraints into categories
- Group similar examples or test cases
- Combine related steps or requirements
4. Practicing Interpretation Skills
Like any skill, interpreting complex problem statements improves with practice. Here are some ways to hone your abilities:
4.1. Timed Reading Exercises
Set a timer and challenge yourself to interpret problem statements within a specific timeframe. Gradually decrease the time as you improve.
4.2. Diverse Problem Types
Expose yourself to a wide variety of problem statements from different domains and difficulty levels. This will help you become more adaptable and quick in your interpretations.
4.3. Peer Discussion
Discuss problem statements with fellow programmers or study groups. Explaining your interpretation to others and hearing their perspectives can deepen your understanding and reveal alternative viewpoints.
4.4. Mock Interviews
Participate in mock coding interviews where you have to interpret and solve problems under time pressure. This simulates real interview conditions and helps you improve your speed and accuracy.
5. Common Pitfalls to Avoid
Be aware of these common mistakes when interpreting problem statements:
5.1. Overlooking Details
Don’t rush through the statement so quickly that you miss crucial information. Pay attention to every word, as small details can significantly impact the solution.
5.2. Making Assumptions
Avoid filling in gaps with your own assumptions. If something is unclear, it’s better to ask for clarification (in an interview setting) or re-read the statement carefully.
5.3. Fixating on Familiar Elements
Don’t let familiarity with certain aspects of the problem blind you to its unique characteristics. Each problem is distinct, even if it shares similarities with others you’ve encountered.
5.4. Ignoring Constraints
Always consider the constraints provided in the problem statement. These often guide you towards the expected solution and help you avoid inefficient approaches.
6. Advanced Strategies for Complex Problems
For particularly complex or lengthy problem statements, consider these advanced strategies:
6.1. Multi-pass Reading
Read the problem statement multiple times, each time with a different focus:
- First pass: Get a general overview
- Second pass: Identify key components and constraints
- Third pass: Look for subtle details and edge cases
6.2. Problem Restatement
Try restating the problem in your own words. This can help you confirm your understanding and sometimes reveal insights you might have missed.
6.3. Decomposition
Break down complex problems into smaller, more manageable sub-problems. This can make the overall task less daunting and easier to approach.
6.4. Analogies and Metaphors
Try to relate the problem to real-world scenarios or familiar concepts. This can make abstract problems more concrete and easier to grasp.
7. Leveraging Tools and Resources
While developing your interpretation skills, don’t hesitate to use available tools and resources:
7.1. Problem Banks
Platforms like LeetCode, HackerRank, and AlgoCademy offer extensive collections of coding problems. Use these to practice interpreting a wide variety of problem statements.
7.2. Syntax Highlighters
When problem statements include code snippets, syntax highlighters can help you quickly identify different components of the code. For example:
function findMaxElement(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
7.3. Visualization Tools
For problems involving data structures or algorithms, visualization tools can help you better understand the problem. For instance, you might use a tool to visualize a binary tree or a graph algorithm.
7.4. AI-powered Assistants
Some platforms offer AI-powered assistants that can help break down problem statements or provide hints. While these can be useful learning aids, remember to develop your own interpretation skills as well.
8. Applying Interpretation Skills in Real-world Scenarios
The ability to quickly interpret complex problem statements isn’t just useful for coding interviews. It’s a valuable skill in various real-world scenarios:
8.1. Project Requirements Analysis
In professional settings, you’ll often need to interpret complex project requirements or specifications. The skills you develop in interpreting coding problems can be directly applied here.
8.2. Debugging and Troubleshooting
When faced with bug reports or system issues, quick interpretation skills can help you rapidly understand the problem and start working on a solution.
8.3. Code Reviews
During code reviews, you’ll need to quickly understand the purpose and implementation of code written by others. Your interpretation skills will be invaluable in this process.
8.4. Technical Documentation
Whether you’re reading or writing technical documentation, the ability to quickly grasp and communicate complex ideas is crucial.
9. Continuous Improvement
Interpreting complex problem statements is a skill that can always be improved. Here are some strategies for continuous enhancement:
9.1. Reflect on Your Process
After each problem you tackle, take a moment to reflect on your interpretation process. What worked well? Where did you struggle? Use these insights to refine your approach.
9.2. Seek Feedback
Don’t hesitate to ask for feedback from mentors, peers, or interviewers on your problem interpretation skills. Their insights can be invaluable for improvement.
9.3. Stay Updated
Keep abreast of new problem-solving techniques, algorithms, and data structures. The more knowledge you have, the easier it becomes to interpret and categorize new problems.
9.4. Cross-disciplinary Learning
Explore problem-solving techniques from other fields, such as mathematics, logic, or even linguistics. These can often provide new perspectives on interpreting and approaching complex problems.
Conclusion
Mastering the art of quickly interpreting complex problem statements is a journey that requires practice, patience, and perseverance. By applying the techniques and strategies outlined in this guide, you can significantly enhance your ability to understand and tackle challenging coding problems.
Remember, the goal is not just to solve problems faster, but to develop a deeper, more intuitive understanding of the problems you encounter. This skill will serve you well beyond coding interviews, becoming an invaluable asset throughout your career in software development.
As you continue to hone your interpretation skills, you’ll find that what once seemed like impenetrable problem statements become clear, manageable challenges. Embrace the process, stay curious, and keep pushing your limits. With time and dedication, you’ll be able to confidently face even the most complex problem statements, unlocking new levels of problem-solving prowess.