Mastering Stack-Based Problems in Interviews: A Comprehensive Guide
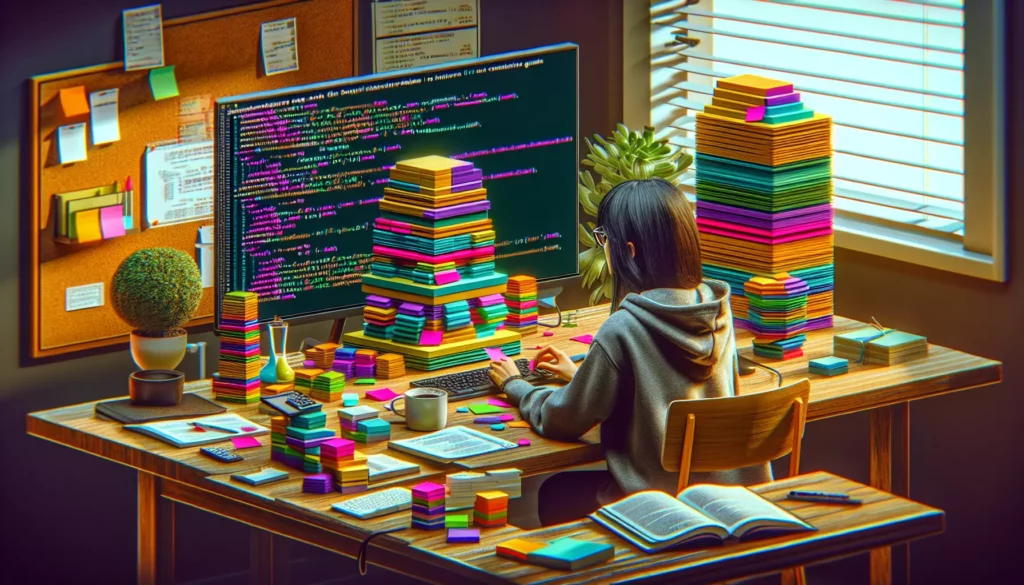
Welcome to our in-depth guide on mastering stack-based problems in coding interviews. If you’re preparing for technical interviews, especially with major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding and solving stack-based problems is crucial. In this article, we’ll explore the concept of stacks, common stack-based problems, and strategies to tackle them effectively.
Table of Contents
- What is a Stack?
- Importance of Stacks in Coding Interviews
- Common Stack Operations
- Implementing a Stack in Different Programming Languages
- Common Stack-Based Problems in Interviews
- Techniques for Solving Stack-Based Problems
- Practice Problems and Solutions
- Interview Tips for Stack-Based Questions
- Conclusion
What is a Stack?
A stack is a fundamental data structure in computer science that follows the Last-In-First-Out (LIFO) principle. This means that the last element added to the stack is the first one to be removed. Think of a stack of plates – you add plates to the top and remove them from the top.
The stack data structure supports two primary operations:
- Push: Add an element to the top of the stack
- Pop: Remove the top element from the stack
Additionally, most stack implementations include a peek operation, which allows you to view the top element without removing it.
Importance of Stacks in Coding Interviews
Stacks are essential in coding interviews for several reasons:
- Fundamental Data Structure: Understanding stacks demonstrates your grasp of basic computer science concepts.
- Problem-Solving Tool: Many complex problems can be elegantly solved using stacks.
- Real-World Applications: Stacks are used in various real-world scenarios, such as managing function calls in programming languages, undo mechanisms in text editors, and parsing expressions.
- Algorithmic Thinking: Stack-based problems often require creative thinking and can reveal your ability to approach problems algorithmically.
Common Stack Operations
Before diving into complex problems, let’s review the basic operations of a stack:
- push(element): Adds an element to the top of the stack
- pop(): Removes and returns the top element from the stack
- peek() or top(): Returns the top element without removing it
- isEmpty(): Returns true if the stack is empty, false otherwise
- size(): Returns the number of elements in the stack
Implementing a Stack in Different Programming Languages
Let’s look at how to implement a basic stack in some popular programming languages:
Python
In Python, we can use a list to implement a stack:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
Java
In Java, we can use an ArrayList to implement a stack:
import java.util.ArrayList;
public class Stack<T> {
private ArrayList<T> items;
public Stack() {
items = new ArrayList<>();
}
public void push(T item) {
items.add(item);
}
public T pop() {
if (!isEmpty()) {
return items.remove(items.size() - 1);
}
return null;
}
public T peek() {
if (!isEmpty()) {
return items.get(items.size() - 1);
}
return null;
}
public boolean isEmpty() {
return items.isEmpty();
}
public int size() {
return items.size();
}
}
JavaScript
In JavaScript, we can use an array to implement a stack:
class Stack {
constructor() {
this.items = [];
}
push(element) {
this.items.push(element);
}
pop() {
if (!this.isEmpty()) {
return this.items.pop();
}
}
peek() {
if (!this.isEmpty()) {
return this.items[this.items.length - 1];
}
}
isEmpty() {
return this.items.length === 0;
}
size() {
return this.items.length;
}
}
Common Stack-Based Problems in Interviews
Now that we’ve covered the basics, let’s explore some common stack-based problems you might encounter in coding interviews:
1. Valid Parentheses
Problem: Given a string containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid. An input string is valid if:
- Open brackets must be closed by the same type of brackets.
- Open brackets must be closed in the correct order.
Solution Approach: Use a stack to keep track of opening brackets. When you encounter a closing bracket, check if it matches the most recent opening bracket (top of the stack).
2. Evaluate Reverse Polish Notation
Problem: Evaluate the value of an arithmetic expression in Reverse Polish Notation (RPN). Valid operators are +, -, *, and /. Each operand may be an integer or another expression.
Solution Approach: Use a stack to store operands. When you encounter an operator, pop the required number of operands, perform the operation, and push the result back onto the stack.
3. Implement Min Stack
Problem: Design a stack that supports push, pop, top, and retrieving the minimum element in constant time.
Solution Approach: Use two stacks – one for regular stack operations and another to keep track of the minimum element at each state of the stack.
4. Largest Rectangle in Histogram
Problem: Given an array of integers representing the histogram’s bar height where the width of each bar is 1, find the area of the largest rectangle in the histogram.
Solution Approach: Use a stack to keep track of indices of histogram bars. Calculate areas when you find a bar shorter than the bar at top of stack.
Techniques for Solving Stack-Based Problems
When approaching stack-based problems in interviews, keep these techniques in mind:
- Recognize Stack Patterns: Many problems involving matching, balancing, or processing elements in reverse order can be solved using stacks.
- Use Multiple Stacks: Some problems require maintaining multiple stacks or using a stack alongside other data structures.
- Implement Monotonic Stacks: A monotonic stack is a stack whose elements are always sorted. This can be useful for problems involving finding the next greater/smaller element.
- Consider Time and Space Complexity: While stacks often provide elegant solutions, be mindful of the time and space complexity of your approach.
- Practice Visualization: Drawing out the state of the stack as you process elements can help you understand the problem better.
Practice Problems and Solutions
Let’s walk through a couple of practice problems to reinforce our understanding:
Problem 1: Next Greater Element
Problem Statement: Given an array, print the Next Greater Element (NGE) for every element. The NGE for an element x is the first greater element on the right side of x in the array. Elements for which no greater element exists, consider the next greater element as -1.
Solution:
def nextGreaterElements(arr):
n = len(arr)
stack = []
result = [-1] * n
for i in range(n-1, -1, -1):
while stack and stack[-1] <= arr[i]:
stack.pop()
if stack:
result[i] = stack[-1]
stack.append(arr[i])
return result
# Example usage
arr = [4, 5, 2, 25, 7, 8]
print(nextGreaterElements(arr)) # Output: [5, 25, 25, -1, 8, -1]
Problem 2: Simplify Directory Path
Problem Statement: Given an absolute path for a file (Unix-style), simplify it. Note that absolute path always begin with ‘/’ (root directory), a dot in path represent current directory, double dot represents parent directory.
Solution:
def simplifyPath(path):
stack = []
components = path.split('/')
for component in components:
if component == '.' or not component:
continue
elif component == '..':
if stack:
stack.pop()
else:
stack.append(component)
return '/' + '/'.join(stack)
# Example usage
path = "/home//foo/"
print(simplifyPath(path)) # Output: "/home/foo"
Interview Tips for Stack-Based Questions
When tackling stack-based problems in an interview setting, keep these tips in mind:
- Clarify the Problem: Make sure you understand all aspects of the problem before starting to code.
- Think Aloud: Explain your thought process as you work through the problem. This gives the interviewer insight into your problem-solving approach.
- Consider Edge Cases: Think about and discuss potential edge cases, such as empty input, single-element input, or maximum input size.
- Analyze Time and Space Complexity: Be prepared to discuss the time and space complexity of your solution.
- Optimize: If you come up with a working solution, think about ways to optimize it further.
- Test Your Solution: Walk through your code with a simple example to ensure it works as expected.
Conclusion
Mastering stack-based problems is an essential skill for coding interviews, especially when aiming for positions at top tech companies. By understanding the fundamental concepts of stacks, practicing common problems, and developing effective problem-solving strategies, you’ll be well-prepared to tackle stack-related questions in your interviews.
Remember, the key to success is consistent practice. Use platforms like AlgoCademy to work through a variety of stack problems, leverage the AI-powered assistance for guidance, and gradually build your confidence in solving these types of questions.
As you continue your interview preparation journey, don’t forget to explore other crucial data structures and algorithms. Stack problems often intersect with other concepts, so a well-rounded understanding of computer science fundamentals will serve you well in your technical interviews and future career in software development.
Happy coding, and best of luck in your interviews!