How to Learn from Each Interview Experience: A Comprehensive Guide
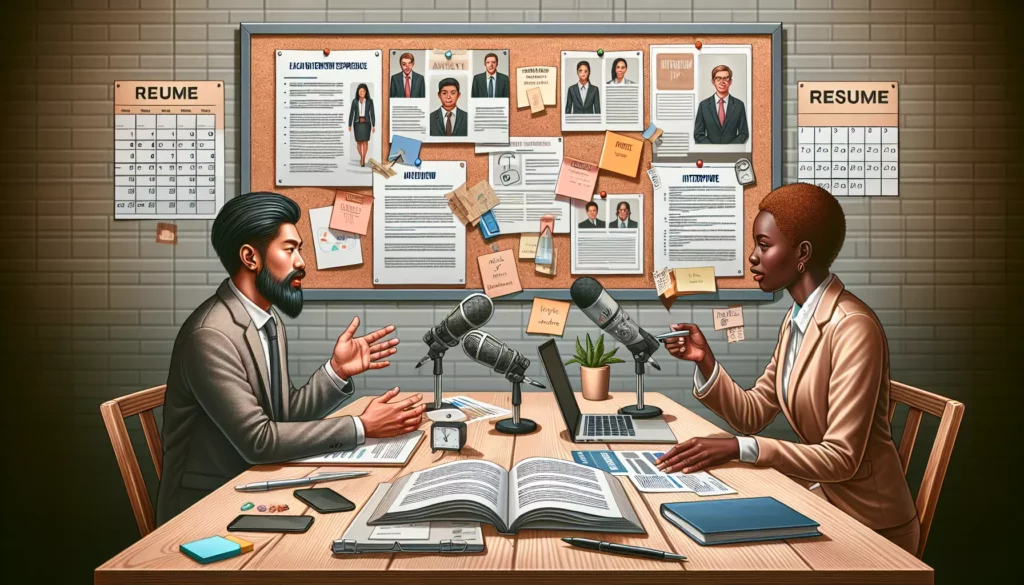
Interviewing for software engineering positions, especially at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), can be a challenging and nerve-wracking experience. However, each interview, regardless of its outcome, presents a valuable learning opportunity. In this comprehensive guide, we’ll explore how to extract maximum value from every interview experience, turning each encounter into a stepping stone towards your dream job.
1. Preparation is Key
Before we dive into post-interview learning, it’s crucial to emphasize the importance of thorough preparation. A well-prepared candidate is more likely to have a positive interview experience and gain more insights from it.
1.1 Review Fundamental Concepts
Brush up on core computer science concepts, data structures, and algorithms. Platforms like AlgoCademy offer interactive coding tutorials and resources specifically designed to help you prepare for technical interviews.
1.2 Practice Coding Problems
Solve a variety of coding problems on platforms like LeetCode, HackerRank, or AlgoCademy. Focus on understanding the underlying patterns and techniques rather than memorizing solutions.
1.3 Mock Interviews
Conduct mock interviews with friends, mentors, or through online platforms. This helps simulate the interview environment and reduces anxiety.
2. During the Interview: Stay Alert and Engaged
To learn effectively from your interview, you need to be fully present and engaged during the process.
2.1 Active Listening
Pay close attention to the interviewer’s questions, comments, and feedback. Often, they provide subtle hints or additional information that can be crucial for solving the problem at hand.
2.2 Ask Clarifying Questions
Don’t hesitate to ask for clarification if something is unclear. This shows your attentiveness and helps ensure you’re solving the right problem.
2.3 Think Aloud
Verbalize your thought process as you work through problems. This gives the interviewer insight into your problem-solving approach and allows them to provide guidance if needed.
3. Post-Interview Reflection
The learning process doesn’t end when you walk out of the interview room. In fact, some of the most valuable insights come from post-interview reflection.
3.1 Immediate Documentation
As soon as possible after the interview, write down everything you can remember. This includes:
- Questions asked
- Your responses
- Challenges you faced
- Feedback received
- Your overall impressions
3.2 Analyze Your Performance
Review your notes and critically assess your performance. Consider the following questions:
- Which questions did you handle well?
- Where did you struggle?
- Were there any concepts you need to review?
- How was your communication throughout the interview?
3.3 Research Unfamiliar Concepts
If you encountered any unfamiliar concepts or technologies during the interview, make a list and research them thoroughly. This not only prepares you for future interviews but also expands your knowledge base.
4. Revisiting Interview Questions
One of the most effective ways to learn from your interview experience is to revisit and solve the questions you faced.
4.1 Recreate the Problems
Try to recreate the coding problems or system design questions you were asked. Solve them again, this time without the pressure of the interview setting.
4.2 Explore Alternative Solutions
Once you’ve solved the problem, explore alternative solutions. There’s often more than one way to solve a problem, and understanding different approaches can greatly enhance your problem-solving skills.
4.3 Optimize Your Solutions
Look for ways to optimize your solutions in terms of time and space complexity. This exercise helps deepen your understanding of algorithmic efficiency.
5. Improving Your Weak Areas
Use the insights gained from your interview to identify and work on your weak areas.
5.1 Create a Personalized Study Plan
Based on your post-interview analysis, create a study plan that focuses on your weak areas. This could involve:
- Reviewing specific data structures or algorithms
- Practicing certain types of problems more intensively
- Improving your system design skills
- Enhancing your communication skills for technical discussions
5.2 Utilize Learning Resources
Take advantage of online learning platforms like AlgoCademy, which offer targeted resources for interview preparation. These platforms often provide:
- Interactive coding tutorials
- Problem-solving exercises with step-by-step guidance
- AI-powered assistance for personalized learning
5.3 Join Coding Communities
Engage with coding communities, either online or in-person. Discussing problems and solutions with peers can provide new perspectives and deepen your understanding.
6. Enhancing Your Soft Skills
Technical skills are crucial, but soft skills can often make or break an interview. Reflect on and improve these aspects of your performance.
6.1 Communication Skills
Practice explaining complex technical concepts clearly and concisely. This skill is crucial for both coding interviews and system design discussions.
6.2 Handling Pressure
If you felt overwhelmed during the interview, work on stress management techniques such as deep breathing or mindfulness exercises.
6.3 Time Management
If you struggled with time management during the interview, practice solving problems under timed conditions to improve your pacing.
7. Learning from Successful Candidates
Gain insights from those who have successfully navigated the interview process at your target companies.
7.1 Read Interview Experiences
Many successful candidates share their interview experiences online. Read these accounts to gain insights into the interview process and preparation strategies.
7.2 Network with Professionals
Connect with professionals working at your target companies. They can provide valuable insights into the company culture and interview process.
7.3 Mentorship
If possible, find a mentor who has experience with technical interviews. Their guidance can be invaluable in your preparation process.
8. Continuous Learning and Improvement
Remember that interview preparation is an ongoing process. Even after you land your dream job, continue to hone your skills.
8.1 Stay Updated with Industry Trends
Keep abreast of new technologies, programming languages, and industry best practices. This knowledge can give you an edge in interviews and in your career.
8.2 Contribute to Open Source Projects
Contributing to open source projects can enhance your coding skills, expose you to different coding styles, and demonstrate your passion for programming to potential employers.
8.3 Build Personal Projects
Work on personal coding projects. These not only improve your skills but also provide great talking points during interviews.
9. Leveraging Technology for Interview Preparation
Make use of technology to enhance your interview preparation process.
9.1 Code Execution Environments
Use online code execution environments to practice coding problems. These platforms allow you to write, run, and debug code in various programming languages. Here’s an example of how you might use such an environment:
// Example: Solving a Two Sum problem in Python
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Test the function
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(f"Indices of two numbers that add up to {target}: {result}")
9.2 Version Control Systems
Familiarize yourself with version control systems like Git. Many coding interviews may involve questions about version control or require you to demonstrate your knowledge. Here’s a basic example of Git commands:
// Basic Git commands
git init // Initialize a new Git repository
git add . // Stage all changes
git commit -m "Initial commit" // Commit staged changes
git push origin main // Push commits to remote repository
9.3 AI-Powered Learning Assistants
Utilize AI-powered learning assistants, like those offered by AlgoCademy, to get personalized feedback on your code and problem-solving approach. These tools can provide hints, explain concepts, and even simulate an interviewer’s perspective.
10. Maintaining a Growth Mindset
Perhaps the most important aspect of learning from each interview experience is maintaining a growth mindset.
10.1 Embrace Challenges
View each difficult question or challenging interview as an opportunity to learn and grow, rather than a setback.
10.2 Learn from Feedback
If you receive constructive feedback, whether from the interviewer or through self-reflection, embrace it as a chance to improve.
10.3 Persistence is Key
Remember that even the most successful software engineers have faced rejection. Persistence and continuous improvement are key to eventual success.
Conclusion
Every interview, regardless of its outcome, is a valuable learning experience. By thoroughly preparing, staying engaged during the interview, reflecting on your performance, addressing your weak areas, and maintaining a growth mindset, you can turn each interview into a stepping stone towards your career goals.
Remember, the journey to becoming a skilled software engineer and acing technical interviews is a marathon, not a sprint. Platforms like AlgoCademy are designed to support you throughout this journey, providing the resources, practice, and guidance you need to succeed.
As you continue to learn and grow, you’ll find that not only do your technical skills improve, but your confidence in tackling challenging problems increases as well. This combination of skills and confidence is what will ultimately lead you to success in your technical interviews and your career as a software engineer.
Keep coding, keep learning, and approach each interview as an opportunity to showcase your skills and learn something new. With persistence and the right mindset, you’ll be well on your way to landing your dream job in the tech industry.