How to Approach Distributed System Design Questions: A Comprehensive Guide
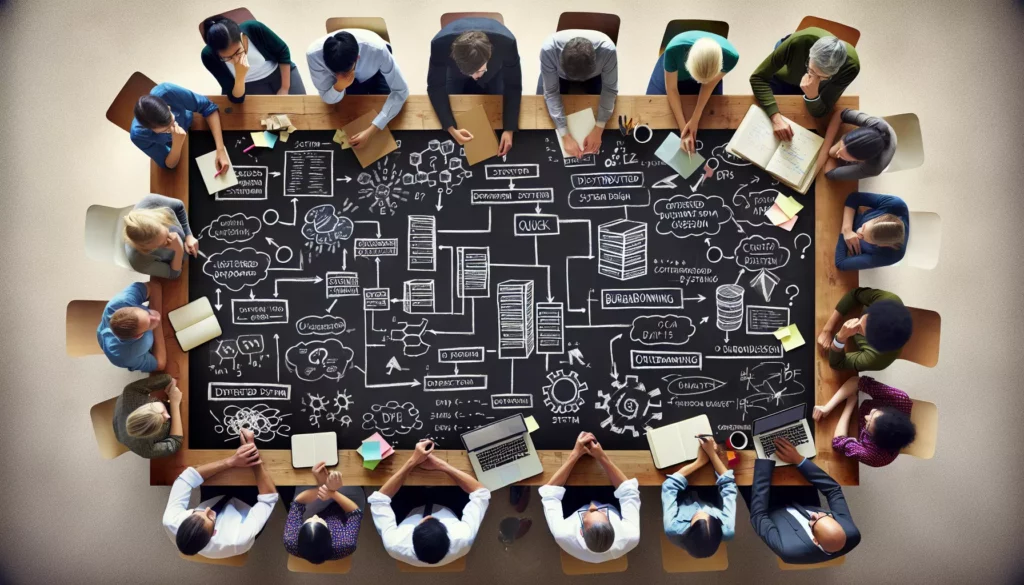
In today’s technology-driven world, distributed systems have become the backbone of many large-scale applications and services. As a result, distributed system design questions have become increasingly common in technical interviews, especially for positions at major tech companies. This comprehensive guide will walk you through the process of approaching distributed system design questions, providing you with the tools and strategies needed to excel in your next interview.
Table of Contents
- Understanding Distributed Systems
- The Importance of Distributed System Design Questions
- A Framework for Approaching Distributed System Design Questions
- Key Concepts in Distributed System Design
- Common Distributed System Design Questions
- Best Practices for Answering Distributed System Design Questions
- Resources for Further Learning
- Conclusion
1. Understanding Distributed Systems
Before diving into the specifics of answering distributed system design questions, it’s crucial to have a solid understanding of what distributed systems are and why they’re important.
What is a Distributed System?
A distributed system is a collection of independent computers that appear to its users as a single coherent system. These systems are designed to work together to achieve a common goal, such as processing large amounts of data or serving millions of users simultaneously.
Key Characteristics of Distributed Systems
- Scalability: The ability to handle increased load by adding more resources.
- Reliability: The system continues to function correctly even when some components fail.
- Availability: The system remains operational and accessible at all times.
- Consistency: All nodes in the system have the same view of the data at any given time.
- Partition Tolerance: The system continues to operate despite network partitions.
2. The Importance of Distributed System Design Questions
Distributed system design questions are a critical component of technical interviews for several reasons:
- Real-world relevance: Many modern applications and services are built on distributed systems.
- Problem-solving skills: These questions test a candidate’s ability to think critically and solve complex problems.
- System design knowledge: They assess a candidate’s understanding of system architecture and design principles.
- Trade-off analysis: Candidates must demonstrate their ability to evaluate and make decisions about trade-offs in system design.
- Communication skills: These questions often require candidates to explain their thought process and design decisions clearly.
3. A Framework for Approaching Distributed System Design Questions
When faced with a distributed system design question, it’s helpful to follow a structured approach. Here’s a framework you can use:
Step 1: Clarify Requirements
- Ask questions to understand the problem scope and constraints.
- Identify functional and non-functional requirements.
- Determine the scale of the system (e.g., number of users, data volume).
Step 2: Define System Interface
- Outline the main APIs or interfaces the system will expose.
- Define the input and output of these interfaces.
Step 3: Estimate Capacity and Constraints
- Calculate storage requirements.
- Estimate network bandwidth usage.
- Determine read/write ratios and query per second (QPS) for each component.
Step 4: Design High-Level Architecture
- Sketch out the main components of the system.
- Identify data storage solutions.
- Consider load balancing and caching strategies.
Step 5: Design Core Components
- Dive deeper into each component’s design.
- Consider algorithms and data structures for specific functionalities.
Step 6: Scale the Design
- Identify potential bottlenecks.
- Propose solutions for scaling (e.g., sharding, replication).
Step 7: Discuss Trade-offs and Alternatives
- Analyze the pros and cons of your design choices.
- Consider alternative approaches and explain why you didn’t choose them.
4. Key Concepts in Distributed System Design
To effectively answer distributed system design questions, you should be familiar with the following key concepts:
Load Balancing
Load balancing is the process of distributing network traffic across multiple servers to ensure no single server bears too much demand. This improves the distribution of workloads across multiple computing resources, maximizing throughput, minimizing response time, and avoiding overload of any single resource.
Caching
Caching involves storing copies of data in a cache, a temporary storage area, to allow faster access to this data in the future. This can significantly improve the performance of a distributed system by reducing the load on backend services and databases.
Database Sharding
Sharding is a database partitioning technique that involves breaking a large database into smaller, more manageable parts called shards. Each shard is held on a separate database server instance, which allows for better distribution of the data load across multiple machines.
Consistency Models
Consistency models define the rules for how changes to data are propagated through a distributed system. Common models include:
- Strong Consistency: All reads receive the most recent write or an error.
- Eventual Consistency: Given enough time, all updates will propagate through the system.
- Causal Consistency: Writes that are causally related must be read in the same order by all processes.
CAP Theorem
The CAP theorem states that it is impossible for a distributed data store to simultaneously provide more than two out of the following three guarantees:
- Consistency: Every read receives the most recent write or an error.
- Availability: Every request receives a (non-error) response, without the guarantee that it contains the most recent write.
- Partition tolerance: The system continues to operate despite an arbitrary number of messages being dropped (or delayed) by the network between nodes.
Message Queues
Message queues provide an asynchronous communications protocol, meaning that the sender and receiver of the message do not need to interact with the message queue at the same time. This is particularly useful for handling tasks that don’t need to be processed immediately or for balancing loads between workers.
5. Common Distributed System Design Questions
Here are some common distributed system design questions you might encounter in interviews:
- Design a URL shortening service like bit.ly
- Design a social media feed (like Facebook or Twitter)
- Design a distributed key-value store
- Design a real-time chat system
- Design a video streaming platform (like YouTube)
- Design a distributed file storage system (like Dropbox)
- Design a web crawler
- Design a notification system
- Design a distributed cache
- Design a content delivery network (CDN)
Let’s take a closer look at one of these questions to see how we might approach it using our framework.
Example: Designing a URL Shortening Service
Step 1: Clarify Requirements
- Functional Requirements:
- Given a long URL, generate a shorter, unique alias
- When users access the short URL, redirect to the original URL
- Users should be able to specify a custom short URL
- Non-Functional Requirements:
- High availability
- Low latency for URL redirection
- The system should be able to handle a high volume of requests
- Scale:
- Assume 100 million new URL shortenings per month
- 1 billion redirections per month
Step 2: Define System Interface
We’ll need two main APIs:
createShortURL(api_dev_key, original_url, custom_alias=None, user_name=None, expire_date=None)
-> Returns: short_url
getOriginalURL(api_dev_key, short_url)
-> Returns: original_url
Step 3: Estimate Capacity and Constraints
- New URLs: 100 million / (30 days * 24 hours * 3600 seconds) ≈ 40 URLs/second
- URL redirections: 1 billion / (30 days * 24 hours * 3600 seconds) ≈ 400 URLs/second
- Storage: Assuming each stored object is 500 bytes, we’ll need 100 million * 500 bytes = 50 GB/month
Step 4: Design High-Level Architecture
Our system will consist of:
- Application servers to handle incoming requests
- Database servers to store URL mappings
- Cache servers to store frequently accessed URLs
- Load balancers to distribute traffic
Step 5: Design Core Components
For URL generation, we could use a base62 encoding of an incrementing ID, which would give us a 7-character URL for up to 62^7 ≈ 3.5 trillion URLs.
Step 6: Scale the Design
- Use database sharding to distribute data across multiple machines
- Implement a cache (e.g., Redis) to store frequently accessed URLs
- Use multiple application servers behind a load balancer
Step 7: Discuss Trade-offs and Alternatives
- Trade-off between short URL length and total number of possible URLs
- Alternative: Use MD5 hash of the original URL, but this could lead to collisions
- Discuss consistency issues that might arise with caching and how to mitigate them
6. Best Practices for Answering Distributed System Design Questions
To excel in distributed system design questions, keep these best practices in mind:
- Start with the basics: Begin with a simple design and gradually add complexity as needed.
- Communicate clearly: Explain your thought process and reasoning behind each decision.
- Ask clarifying questions: Don’t hesitate to ask for more information or clarification about requirements.
- Consider trade-offs: Always discuss the pros and cons of your design choices.
- Be familiar with real-world systems: Understanding how existing distributed systems work can provide valuable insights.
- Practice, practice, practice: The more you practice, the more comfortable you’ll become with these types of questions.
- Stay up-to-date: Keep learning about new technologies and design patterns in distributed systems.
- Draw diagrams: Visual representations can help clarify your ideas and make your explanations more effective.
- Consider edge cases: Think about how your system would handle failures or unexpected scenarios.
- Be ready to iterate: Be open to feedback and be prepared to modify your design based on new information or requirements.
7. Resources for Further Learning
To deepen your understanding of distributed systems and improve your ability to answer design questions, consider exploring these resources:
Books
- “Designing Data-Intensive Applications” by Martin Kleppmann
- “System Design Interview – An Insider’s Guide” by Alex Xu
- “Designing Distributed Systems” by Brendan Burns
Online Courses
- MIT’s Distributed Systems course on edX
- Coursera’s Cloud Computing Specialization
- Udacity’s Scalable Microservices with Kubernetes
Websites and Blogs
- High Scalability (highscalability.com)
- System Design Primer (github.com/donnemartin/system-design-primer)
- Netflix Tech Blog (netflixtechblog.com)
Practice Platforms
- LeetCode’s System Design section
- Grokking the System Design Interview on Educative.io
- InterviewBit’s System Design problems
8. Conclusion
Mastering distributed system design questions is a valuable skill that can significantly boost your chances of success in technical interviews, especially for positions at major tech companies. By understanding the key concepts, following a structured approach, and practicing regularly, you can develop the confidence and expertise needed to tackle even the most challenging design questions.
Remember that distributed system design is as much an art as it is a science. There’s often no single “correct” answer, but rather a range of possible solutions with different trade-offs. The key is to demonstrate your ability to think critically about complex systems, make informed design decisions, and clearly communicate your reasoning.
As you continue to learn and practice, you’ll find that your ability to design scalable, reliable, and efficient distributed systems will improve. This skill set will not only help you in interviews but will also prove invaluable in your career as a software engineer or system architect.
Keep exploring, keep learning, and don’t be afraid to tackle complex design problems. With time and practice, you’ll be well-equipped to handle any distributed system design question that comes your way. Good luck in your interviews and your future endeavors in the world of distributed systems!