Understanding Common Algorithm Patterns: A Comprehensive Guide
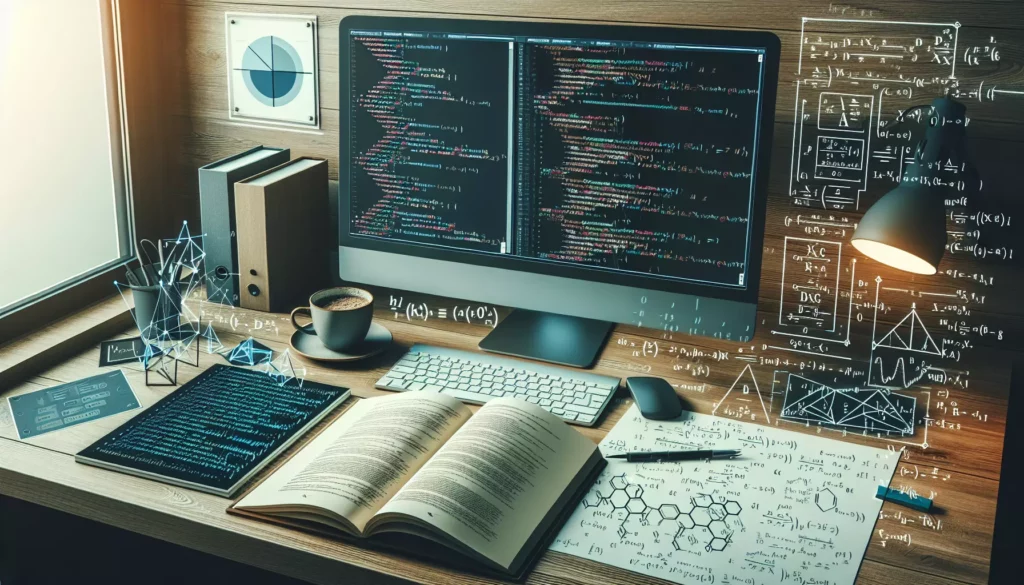
In the world of programming and software development, understanding common algorithm patterns is crucial for solving complex problems efficiently. Whether you’re a beginner looking to improve your coding skills or an experienced developer preparing for technical interviews at major tech companies, mastering these patterns can significantly enhance your problem-solving abilities. In this comprehensive guide, we’ll explore various algorithm patterns, their applications, and how to implement them effectively.
1. Two Pointer Technique
The Two Pointer technique is a simple yet powerful algorithm pattern used to solve various problems, particularly those involving arrays or linked lists. This method involves using two pointers that move through the data structure, often in opposite directions or at different speeds.
When to Use:
- Searching for pairs in a sorted array
- Reversing an array or string
- Removing duplicates from a sorted array
- Finding a subarray with a specific sum
Example: Reversing a String
function reverseString(str) {
let left = 0;
let right = str.length - 1;
let chars = str.split('');
while (left < right) {
// Swap characters
[chars[left], chars[right]] = [chars[right], chars[left]];
left++;
right--;
}
return chars.join('');
}
console.log(reverseString("hello")); // Output: "olleh"
2. Sliding Window
The Sliding Window technique is an efficient method for processing subarrays or sublists within a larger data structure. It involves maintaining a “window” that slides through the data, allowing for optimal time complexity in many scenarios.
When to Use:
- Finding the longest substring with certain properties
- Calculating the maximum/minimum sum of a subarray of fixed size
- Detecting patterns in strings
Example: Maximum Sum Subarray of Size K
function maxSubarraySum(arr, k) {
if (arr.length < k) return null;
let maxSum = 0;
let windowSum = 0;
// Calculate sum of first window
for (let i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
// Slide the window
for (let i = k; i < arr.length; i++) {
windowSum = windowSum - arr[i - k] + arr[i];
maxSum = Math.max(maxSum, windowSum);
}
return maxSum;
}
console.log(maxSubarraySum([1, 4, 2, 10, 23, 3, 1, 0, 20], 4)); // Output: 39
3. Fast and Slow Pointers (Floyd’s Cycle-Finding Algorithm)
This pattern, also known as the “tortoise and hare” algorithm, uses two pointers moving at different speeds to solve problems related to cyclic data structures or to find a specific element in a sequence.
When to Use:
- Detecting cycles in linked lists
- Finding the middle element of a linked list
- Determining if a number is happy (in the mathematical sense)
Example: Detecting a Cycle in a Linked List
class ListNode {
constructor(value) {
this.value = value;
this.next = null;
}
}
function hasCycle(head) {
if (!head || !head.next) return false;
let slow = head;
let fast = head.next;
while (slow !== fast) {
if (!fast || !fast.next) return false;
slow = slow.next;
fast = fast.next.next;
}
return true;
}
// Example usage
let head = new ListNode(1);
head.next = new ListNode(2);
head.next.next = new ListNode(3);
head.next.next.next = head.next; // Create a cycle
console.log(hasCycle(head)); // Output: true
4. Merge Intervals
The Merge Intervals pattern is used to deal with overlapping intervals. It’s particularly useful in scenarios involving time intervals, scheduling, or any situation where ranges might overlap.
When to Use:
- Merging overlapping intervals
- Finding free time slots in a schedule
- Determining if intervals conflict
Example: Merging Overlapping Intervals
function mergeIntervals(intervals) {
if (intervals.length <= 1) return intervals;
// Sort intervals based on start time
intervals.sort((a, b) => a[0] - b[0]);
const result = [intervals[0]];
for (let i = 1; i < intervals.length; i++) {
const currentInterval = intervals[i];
const lastMergedInterval = result[result.length - 1];
if (currentInterval[0] <= lastMergedInterval[1]) {
// Overlap found, merge intervals
lastMergedInterval[1] = Math.max(lastMergedInterval[1], currentInterval[1]);
} else {
// No overlap, add current interval to result
result.push(currentInterval);
}
}
return result;
}
console.log(mergeIntervals([[1,3],[2,6],[8,10],[15,18]]));
// Output: [[1,6],[8,10],[15,18]]
5. Binary Search
Binary Search is a highly efficient search algorithm used on sorted arrays. It repeatedly divides the search space in half, allowing for logarithmic time complexity.
When to Use:
- Searching in a sorted array
- Finding the first or last occurrence of an element
- Implementing efficient algorithms for various problems on sorted data
Example: Binary Search Implementation
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid; // Target found
} else if (arr[mid] < target) {
left = mid + 1; // Search right half
} else {
right = mid - 1; // Search left half
}
}
return -1; // Target not found
}
console.log(binarySearch([1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 7)); // Output: 6
6. Depth-First Search (DFS)
Depth-First Search is a graph traversal algorithm that explores as far as possible along each branch before backtracking. It’s widely used for exploring tree and graph structures.
When to Use:
- Traversing tree structures
- Finding paths in a graph
- Detecting cycles in a graph
- Topological sorting
Example: DFS on a Binary Tree
class TreeNode {
constructor(value) {
this.value = value;
this.left = null;
this.right = null;
}
}
function dfs(root) {
if (!root) return;
console.log(root.value); // Process current node
dfs(root.left); // Traverse left subtree
dfs(root.right); // Traverse right subtree
}
// Example usage
let root = new TreeNode(1);
root.left = new TreeNode(2);
root.right = new TreeNode(3);
root.left.left = new TreeNode(4);
root.left.right = new TreeNode(5);
dfs(root);
// Output: 1 2 4 5 3
7. Breadth-First Search (BFS)
Breadth-First Search is another graph traversal algorithm that explores all vertices of a graph in breadth-first order, i.e., it visits all the neighbor nodes at the present depth before moving to nodes at the next depth level.
When to Use:
- Finding the shortest path in an unweighted graph
- Level-order traversal of a tree
- Finding all nodes within one connected component
Example: BFS on a Binary Tree
function bfs(root) {
if (!root) return;
const queue = [root];
while (queue.length > 0) {
const node = queue.shift();
console.log(node.value); // Process current node
if (node.left) queue.push(node.left);
if (node.right) queue.push(node.right);
}
}
// Using the same tree structure as in the DFS example
bfs(root);
// Output: 1 2 3 4 5
8. Dynamic Programming
Dynamic Programming is a method for solving complex problems by breaking them down into simpler subproblems. It is particularly useful for optimization problems and can significantly improve time complexity in many cases.
When to Use:
- Optimization problems with overlapping subproblems
- Problems with optimal substructure
- Counting problems
Example: Fibonacci Sequence using Dynamic Programming
function fibonacci(n) {
if (n <= 1) return n;
let dp = new Array(n + 1);
dp[0] = 0;
dp[1] = 1;
for (let i = 2; i <= n; i++) {
dp[i] = dp[i - 1] + dp[i - 2];
}
return dp[n];
}
console.log(fibonacci(10)); // Output: 55
9. Backtracking
Backtracking is an algorithmic technique that considers searching every possible combination in order to solve a computational problem. It builds candidates to the solution incrementally and abandons a candidate as soon as it determines that the candidate cannot lead to a valid solution.
When to Use:
- Solving puzzles (e.g., Sudoku)
- Generating all possible permutations or combinations
- Finding all possible solutions to a problem
Example: Generating All Permutations of a String
function generatePermutations(str) {
const result = [];
function backtrack(current, remaining) {
if (remaining.length === 0) {
result.push(current);
return;
}
for (let i = 0; i < remaining.length; i++) {
backtrack(
current + remaining[i],
remaining.slice(0, i) + remaining.slice(i + 1)
);
}
}
backtrack('', str);
return result;
}
console.log(generatePermutations("abc"));
// Output: ["abc", "acb", "bac", "bca", "cab", "cba"]
10. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step with the hope of finding a global optimum. While they don’t always yield the best solution, they are often used for optimization problems and can provide good approximations in many cases.
When to Use:
- Optimization problems where local optimal choices lead to global optimal solution
- Problems requiring fast, approximate solutions
Example: Activity Selection Problem
function activitySelection(start, finish) {
const n = start.length;
const activities = Array.from({ length: n }, (_, i) => ({
start: start[i],
finish: finish[i]
}));
// Sort activities by finish time
activities.sort((a, b) => a.finish - b.finish);
const selected = [activities[0]];
let lastFinish = activities[0].finish;
for (let i = 1; i < n; i++) {
if (activities[i].start >= lastFinish) {
selected.push(activities[i]);
lastFinish = activities[i].finish;
}
}
return selected;
}
const start = [1, 3, 0, 5, 8, 5];
const finish = [2, 4, 6, 7, 9, 9];
console.log(activitySelection(start, finish));
// Output: [{ start: 1, finish: 2 }, { start: 3, finish: 4 }, { start: 5, finish: 7 }, { start: 8, finish: 9 }]
Conclusion
Understanding these common algorithm patterns is essential for any programmer looking to enhance their problem-solving skills and prepare for technical interviews. Each pattern has its own strengths and is suited for different types of problems. By mastering these patterns, you’ll be better equipped to tackle a wide range of coding challenges efficiently.
Remember, the key to becoming proficient with these patterns is practice. Try implementing these algorithms in different scenarios, solve related problems, and analyze their time and space complexities. As you gain more experience, you’ll start recognizing which pattern to apply to new problems you encounter.
Keep in mind that while these patterns are powerful tools, they’re not silver bullets. Sometimes, problems may require a combination of patterns or entirely novel approaches. Always strive to understand the problem thoroughly before deciding on an approach.
Happy coding, and may these algorithm patterns serve you well in your programming journey!