51 Effective Ways to Practice Mock Interviews for Coding and Tech Jobs
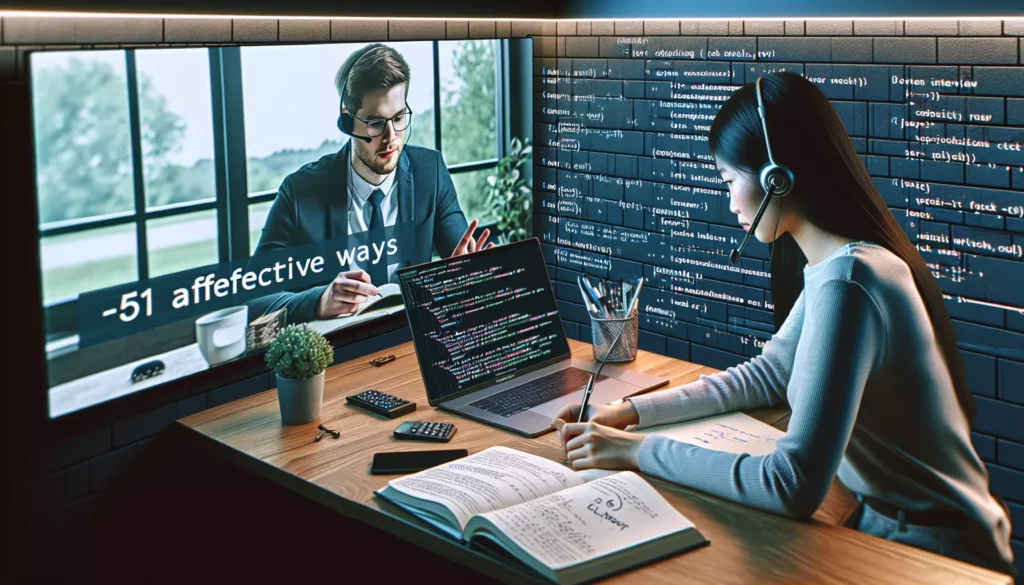
Preparing for coding interviews, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), requires dedication, strategy, and consistent practice. Mock interviews are an essential part of this preparation process, allowing you to simulate real interview conditions and improve your performance. In this comprehensive guide, we’ll explore 51 effective ways to practice mock interviews, helping you sharpen your coding skills, boost your confidence, and increase your chances of landing your dream tech job.
1. Utilize Online Platforms
There are numerous online platforms designed specifically for coding interview practice:
- LeetCode: Offers a vast collection of coding problems and mock interviews.
- HackerRank: Provides coding challenges and interview preparation kits.
- CodeSignal: Features a “Interview Practice” section with company-specific questions.
- Pramp: Allows you to practice live coding interviews with peers.
- InterviewBit: Offers a structured interview preparation course and mock interviews.
2. Join Coding Communities
Engage with other aspiring developers and experienced professionals:
- Reddit communities like r/cscareerquestions and r/leetcode
- Discord servers focused on coding and interview preparation
- Local meetup groups for developers
- Online forums like Stack Overflow
3. Practice with a Study Buddy
Find a friend or colleague who is also preparing for coding interviews. Take turns interviewing each other to simulate real interview conditions and provide feedback.
4. Record Your Mock Interviews
Use screen recording software to capture your mock interview sessions. Review the recordings to identify areas for improvement in your communication, problem-solving approach, and coding style.
5. Time Your Practice Sessions
Most coding interviews have time limits. Practice solving problems within similar time constraints to improve your speed and efficiency.
6. Use a Whiteboard or Paper
Some interviews may require you to write code on a whiteboard or paper. Practice this method to become comfortable with coding without an IDE or autocomplete features.
7. Verbalize Your Thought Process
Practice explaining your approach out loud as you solve problems. This helps interviewers understand your thinking and demonstrates your communication skills.
8. Focus on Core Data Structures
Ensure you have a solid understanding of fundamental data structures:
- Arrays
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
9. Master Essential Algorithms
Practice implementing and explaining key algorithms:
- Sorting algorithms (e.g., Quick Sort, Merge Sort)
- Searching algorithms (e.g., Binary Search)
- Graph algorithms (e.g., BFS, DFS)
- Dynamic Programming
- Greedy Algorithms
10. Study Company-Specific Questions
Research and practice questions that are frequently asked by the companies you’re targeting. Websites like Glassdoor and LeetCode often have company-specific problem sets.
11. Practice System Design Questions
For more senior positions, prepare for system design interviews by studying scalable architectures, distributed systems, and design patterns.
12. Implement a Mock Interview Schedule
Create a structured schedule for your mock interviews, gradually increasing the difficulty and complexity of the problems you tackle.
13. Use AI-Powered Interview Assistants
Explore AI-powered tools that can simulate interview scenarios and provide feedback on your performance.
14. Attend Virtual Career Fairs
Participate in virtual career fairs organized by companies or universities to gain exposure to real interview scenarios.
15. Practice Behavioral Questions
Don’t neglect the non-technical aspects of interviews. Prepare answers to common behavioral questions using the STAR method (Situation, Task, Action, Result).
16. Solve Problems in Multiple Languages
If you’re proficient in multiple programming languages, practice solving problems in each to demonstrate versatility.
17. Participate in Coding Competitions
Join online coding competitions or hackathons to challenge yourself and gain experience solving problems under pressure.
18. Create a GitHub Portfolio
Develop and showcase your projects on GitHub. This can serve as talking points during interviews and demonstrate your practical coding skills.
19. Practice Pair Programming
Some interviews involve pair programming. Practice collaborating with others on coding problems to improve your teamwork and communication skills.
20. Study Time and Space Complexity
Be prepared to analyze and discuss the time and space complexity of your solutions. Practice identifying and optimizing inefficient code.
21. Mock Interview with Industry Professionals
If possible, arrange mock interviews with experienced developers or hiring managers to get authentic feedback and insights.
22. Utilize Interactive Coding Platforms
Use platforms like Repl.it or CodePen for live coding practice, which can simulate the collaborative coding environments used in some interviews.
23. Practice Debugging
Develop your debugging skills by intentionally introducing bugs into your code and then fixing them. This demonstrates problem-solving abilities crucial for real-world scenarios.
24. Study Design Patterns
Familiarize yourself with common software design patterns and be prepared to discuss how and when to apply them.
25. Review Computer Science Fundamentals
Brush up on core CS concepts like operating systems, networking, and databases. These topics often come up in interviews, especially for more senior positions.
26. Practice Code Reviews
Review other people’s code and have your code reviewed. This improves your ability to read, understand, and critique code – a valuable skill in interviews and on the job.
27. Solve Problems on Paper First
Before coding, practice solving problems on paper. This helps you think through the logic without relying on IDE features.
28. Implement Data Structures from Scratch
Build common data structures (like linked lists or hash tables) from scratch to deepen your understanding of their inner workings.
29. Practice Refactoring Code
Take existing code and practice refactoring it to improve efficiency, readability, or design. This demonstrates your ability to work with and improve legacy code.
30. Study Clean Code Principles
Familiarize yourself with clean code principles and practice writing code that is not only functional but also maintainable and readable.
31. Mock Interviews in Different Formats
Practice different interview formats, such as phone screens, video interviews, and onsite interviews, as each requires slightly different skills and preparation.
32. Use Analog Clocks for Timing
Practice with an analog clock or watch to better manage your time without the stress of a digital countdown.
33. Practice Explaining Technical Concepts
Improve your ability to explain complex technical concepts in simple terms. This is crucial for demonstrating clear communication skills.
34. Study and Practice Testing
Learn about different testing methodologies and practice writing unit tests for your code. Many interviews include questions about testing strategies.
35. Explore Different Problem-Solving Techniques
Practice various problem-solving techniques like divide and conquer, sliding window, or two-pointer approach to tackle a wide range of coding challenges.
36. Implement a Personal Project
Work on a personal coding project that you can discuss in interviews. This demonstrates initiative and practical application of your skills.
37. Practice Handling Edge Cases
When solving problems, always consider and handle edge cases. This shows attention to detail and thorough thinking.
38. Study Software Development Methodologies
Familiarize yourself with different software development methodologies like Agile or Scrum. Be prepared to discuss your experience or understanding of these approaches.
39. Practice Estimating Time for Tasks
Improve your ability to estimate how long a coding task might take. This skill is often valuable in both interviews and real-world development scenarios.
40. Learn to Ask Clarifying Questions
Practice asking good clarifying questions before jumping into problem-solving. This demonstrates thoughtful analysis and attention to requirements.
41. Study Common Libraries and Frameworks
Familiarize yourself with popular libraries and frameworks in your chosen programming language. Be prepared to discuss their pros and cons.
42. Practice Coding Without an Internet Connection
Simulate conditions where you can’t quickly look up syntax or solutions online. This improves your recall and deep understanding of the language.
43. Implement Well-Known Algorithms
Practice implementing well-known algorithms like Dijkstra’s algorithm or the Knapsack problem. Understanding these can help in tackling more complex problems.
44. Study and Practice SQL Queries
Many software engineering roles require database knowledge. Practice writing complex SQL queries and understanding database design principles.
45. Mock Interviews with Time Pressure
Gradually decrease the time allowed for solving problems in your mock interviews to improve your ability to work under pressure.
46. Practice Explaining Your Resume
Be prepared to discuss any project or technology mentioned in your resume. Practice concise, impactful explanations of your experiences.
47. Study Computer Architecture
Understanding basic computer architecture can be beneficial, especially for low-level programming questions or system design discussions.
48. Practice Multithreading and Concurrency
For more advanced positions, study and practice concepts related to multithreading and concurrency. These topics often come up in system design questions.
49. Learn About Cloud Technologies
Familiarize yourself with basic cloud concepts and popular platforms like AWS, Google Cloud, or Azure. Many companies use cloud services extensively.
50. Practice Code Optimization
Take working solutions and practice optimizing them for better performance or memory usage. This demonstrates your ability to write efficient code.
51. Reflect and Iterate
After each mock interview or practice session, reflect on your performance. Identify areas for improvement and adjust your study plan accordingly.
Conclusion
Mastering the art of coding interviews takes time, dedication, and consistent practice. By incorporating these 51 strategies into your preparation routine, you’ll be well-equipped to tackle even the most challenging interview questions. Remember, the goal is not just to memorize solutions but to develop a deep understanding of problem-solving techniques and to communicate your thought process effectively.
As you progress through your interview preparation journey, don’t forget to take care of your mental and physical well-being. Regular breaks, exercise, and maintaining a balanced lifestyle are crucial for long-term success. Stay motivated, stay curious, and keep coding!
Good luck with your interviews, and may your hard work lead you to the tech career of your dreams!