How to Prepare for Whiteboard Coding Sessions: A Comprehensive Guide
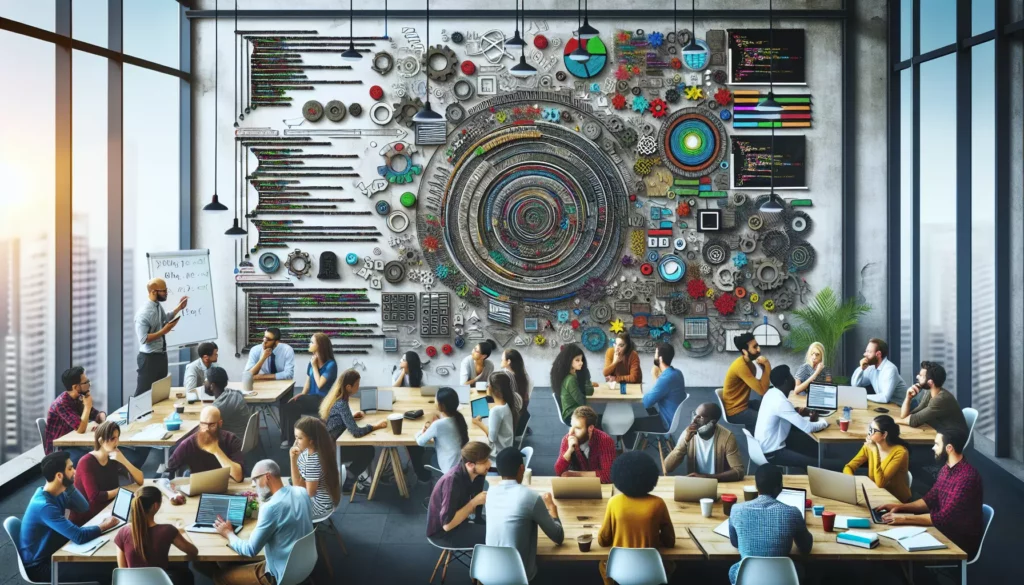
Whiteboard coding sessions are a crucial part of many technical interviews, especially for software engineering positions at top tech companies. These sessions can be intimidating, but with proper preparation, you can significantly improve your performance and boost your chances of landing your dream job. In this comprehensive guide, we’ll explore effective strategies to prepare for whiteboard coding sessions, covering everything from fundamental concepts to advanced techniques.
Understanding Whiteboard Coding Sessions
Before diving into preparation strategies, it’s essential to understand what whiteboard coding sessions entail. These interviews typically involve solving coding problems or algorithmic challenges on a whiteboard (or sometimes a shared online document) while explaining your thought process to the interviewer. The goal is not just to arrive at the correct solution but to demonstrate your problem-solving skills, coding ability, and communication skills.
1. Master the Fundamentals
A strong foundation in computer science fundamentals is crucial for success in whiteboard coding sessions. Focus on the following areas:
1.1 Data Structures
Understand and be comfortable working with common data structures, including:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
1.2 Algorithms
Familiarize yourself with fundamental algorithms and their implementations:
- Sorting algorithms (e.g., Quicksort, Mergesort, Heapsort)
- Searching algorithms (e.g., Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Recursion and Backtracking
1.3 Time and Space Complexity
Learn to analyze and discuss the time and space complexity of your solutions using Big O notation. This skill is crucial for optimizing your code and demonstrating your understanding of efficiency.
2. Practice, Practice, Practice
Consistent practice is key to improving your whiteboard coding skills. Here are some effective ways to practice:
2.1 Solve Coding Problems Regularly
Dedicate time each day to solving coding problems. Use platforms like:
- LeetCode
- HackerRank
- CodeSignal
- Project Euler
Start with easier problems and gradually increase the difficulty as you improve.
2.2 Simulate Whiteboard Conditions
Practice solving problems on an actual whiteboard or using a simple text editor without auto-completion or syntax highlighting. This will help you get used to coding without the assistance of an IDE.
2.3 Time Yourself
Most whiteboard coding sessions have time constraints. Practice solving problems within a set time limit to improve your speed and efficiency.
2.4 Explain Your Thought Process
Get comfortable explaining your approach out loud as you solve problems. This will help you practice clear communication, which is crucial during the actual interview.
3. Develop a Problem-Solving Approach
Having a structured approach to problem-solving can help you tackle unfamiliar problems more effectively. Follow these steps:
3.1 Understand the Problem
- Read the problem statement carefully
- Ask clarifying questions
- Identify the inputs and expected outputs
- Consider edge cases and constraints
3.2 Plan Your Approach
- Break down the problem into smaller subproblems
- Consider multiple approaches and their trade-offs
- Choose the most appropriate data structures and algorithms
3.3 Implement the Solution
- Write clean, readable code
- Use meaningful variable and function names
- Comment your code when necessary
3.4 Test and Optimize
- Test your solution with sample inputs
- Consider edge cases and potential bugs
- Analyze the time and space complexity
- Optimize your solution if possible
4. Learn Common Patterns and Techniques
Many coding problems follow specific patterns or can be solved using common techniques. Familiarize yourself with the following:
4.1 Two Pointers
This technique involves using two pointers to traverse an array or string, often moving in opposite directions. It’s useful for problems like reversing a string or finding a pair of elements with a specific sum.
Example: Two Sum II – Input Array Is Sorted
def twoSum(numbers, target):
left, right = 0, len(numbers) - 1
while left < right:
current_sum = numbers[left] + numbers[right]
if current_sum == target:
return [left + 1, right + 1]
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
4.2 Sliding Window
This technique is used to process subarrays or substrings of a given size or with specific properties. It’s efficient for problems involving contiguous sequences.
Example: Maximum Sum Subarray of Size K
def maxSubarraySum(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
4.3 Binary Search
Binary search is an efficient algorithm for searching sorted arrays. It’s also useful in various other problem-solving scenarios.
Example: Binary Search Implementation
def binarySearch(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = left + (right - left) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
4.4 Depth-First Search (DFS) and Breadth-First Search (BFS)
These graph traversal algorithms are essential for solving problems involving trees, graphs, and matrices.
Example: DFS for a Binary Tree
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def dfs(root):
if not root:
return
print(root.val) # Process the current node
dfs(root.left) # Traverse left subtree
dfs(root.right) # Traverse right subtree
4.5 Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems by breaking them down into simpler subproblems.
Example: Fibonacci Sequence with Dynamic Programming
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
5. Improve Your Coding Style
Clean, readable code is important in whiteboard coding sessions. Follow these best practices:
5.1 Use Meaningful Variable Names
Choose descriptive names for variables and functions that clearly convey their purpose.
5.2 Write Modular Code
Break your solution into smaller, reusable functions when appropriate.
5.3 Comment Your Code
Add comments to explain complex logic or non-obvious implementation details.
5.4 Follow Consistent Indentation
Use proper indentation to improve code readability, especially on a whiteboard.
6. Enhance Your Problem-Solving Skills
Developing strong problem-solving skills goes beyond just coding. Consider these strategies:
6.1 Think Aloud
Practice verbalizing your thought process as you solve problems. This helps the interviewer understand your approach and allows them to provide hints if needed.
6.2 Start with a Brute Force Approach
If you’re stuck, begin with a simple, inefficient solution. This demonstrates your problem-solving ability and provides a starting point for optimization.
6.3 Use Examples and Visualizations
Draw diagrams or use specific examples to illustrate your approach. This can help clarify your thinking and communicate your ideas effectively.
6.4 Learn to Optimize
After implementing a solution, always consider ways to optimize it. Look for opportunities to reduce time complexity or space usage.
7. Mock Interviews and Peer Practice
Simulate real interview conditions to gain confidence and identify areas for improvement:
7.1 Conduct Mock Interviews
Practice with friends, colleagues, or use online platforms that offer mock interview services.
7.2 Join Coding Communities
Participate in coding forums, Discord servers, or local meetups to connect with other developers and share experiences.
7.3 Code Reviews
Have experienced developers review your code and provide feedback on your solutions and coding style.
8. Manage Interview Anxiety
Whiteboard coding sessions can be stressful. Use these techniques to manage anxiety:
8.1 Deep Breathing
Practice deep breathing exercises before and during the interview to stay calm and focused.
8.2 Positive Visualization
Imagine yourself successfully solving problems and performing well in the interview.
8.3 Embrace Mistakes
Remember that making mistakes is normal. Use them as learning opportunities and demonstrate your ability to adapt and correct errors.
9. Stay Updated with Industry Trends
Keep yourself informed about the latest developments in software engineering:
9.1 Follow Tech Blogs and News
Stay updated with industry trends, new technologies, and best practices.
9.2 Contribute to Open Source
Participating in open-source projects can improve your coding skills and expose you to different coding styles and practices.
9.3 Attend Tech Conferences and Webinars
These events can provide valuable insights into industry trends and networking opportunities.
10. Tailor Your Preparation
Customize your preparation based on the specific company and role you’re interviewing for:
10.1 Research the Company
Understand the company’s products, technologies, and engineering challenges.
10.2 Review the Job Description
Focus on the skills and technologies mentioned in the job posting.
10.3 Practice Company-Specific Questions
Some companies are known for asking certain types of questions. Research and practice these specific problem types.
Conclusion
Preparing for whiteboard coding sessions requires dedication, consistent practice, and a structured approach. By mastering the fundamentals, developing strong problem-solving skills, and simulating interview conditions, you can significantly improve your performance in these challenging interviews. Remember that the goal is not just to solve the problem but to demonstrate your thought process, communication skills, and ability to write clean, efficient code.
As you prepare, leverage resources like AlgoCademy, which offers interactive coding tutorials, AI-powered assistance, and step-by-step guidance to help you progress from beginner-level coding to mastering technical interviews. With the right preparation and mindset, you can approach whiteboard coding sessions with confidence and increase your chances of success in your job search.