Applying Object-Oriented Principles in Coding Interviews
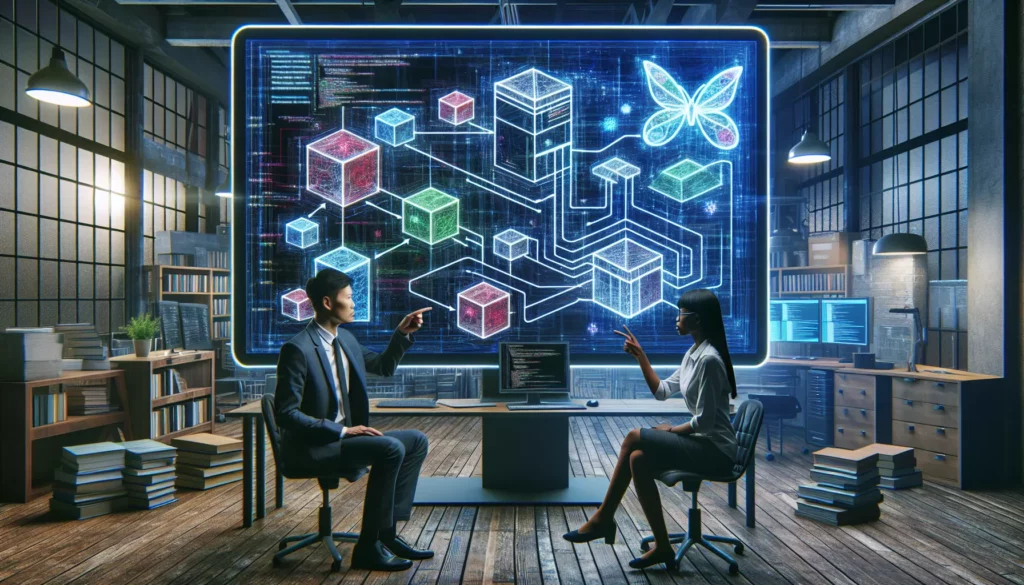
In the world of software development, object-oriented programming (OOP) has become a cornerstone of modern coding practices. As aspiring developers prepare for technical interviews, particularly those targeting prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), understanding and applying OOP principles is crucial. This article will delve into the importance of OOP in coding interviews, explore key concepts, and provide practical examples to help you ace your next technical interview.
Why OOP Matters in Coding Interviews
Object-oriented programming is not just a paradigm; it’s a way of thinking about and structuring code that can significantly impact the quality and maintainability of software. Interviewers at top tech companies often assess candidates’ ability to apply OOP principles for several reasons:
- Code Organization: OOP helps in organizing code into logical, reusable components.
- Scalability: Well-designed OOP systems are more scalable and easier to extend.
- Maintainability: OOP principles lead to code that is easier to understand and maintain.
- Problem-Solving: OOP concepts can be powerful tools in solving complex programming challenges.
- Industry Standard: Many large-scale applications are built using OOP, making it an essential skill for professional developers.
Key OOP Concepts to Master
Before diving into interview scenarios, let’s review the fundamental OOP concepts that you should be comfortable with:
1. Encapsulation
Encapsulation is the bundling of data and the methods that operate on that data within a single unit or object. It restricts direct access to some of an object’s components, which is a means of preventing accidental interference and misuse of the methods and data.
Example of Encapsulation:
class BankAccount {
private:
double balance;
public:
void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
bool withdraw(double amount) {
if (amount > 0 && balance >= amount) {
balance -= amount;
return true;
}
return false;
}
double getBalance() {
return balance;
}
};
In this example, the balance
is private and can only be accessed or modified through public methods, ensuring controlled access and data integrity.
2. Inheritance
Inheritance is a mechanism where a new class is derived from an existing class. The new class inherits properties and behaviors from the existing class, allowing for code reuse and the creation of a hierarchy of classes.
Example of Inheritance:
class Animal {
protected:
string name;
public:
void setName(string n) { name = n; }
virtual void makeSound() = 0;
};
class Dog : public Animal {
public:
void makeSound() override {
cout << name << " says Woof!" << endl;
}
};
class Cat : public Animal {
public:
void makeSound() override {
cout << name << " says Meow!" << endl;
}
};
Here, Dog
and Cat
classes inherit from the Animal
base class, demonstrating how common attributes and behaviors can be shared.
3. Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common base class. It enables you to write code that can work with objects of multiple types, providing flexibility and extensibility.
Example of Polymorphism:
void animalSound(Animal* animal) {
animal->makeSound();
}
int main() {
Dog dog;
Cat cat;
dog.setName("Buddy");
cat.setName("Whiskers");
animalSound(&dog); // Output: Buddy says Woof!
animalSound(&cat); // Output: Whiskers says Meow!
return 0;
}
This example demonstrates how polymorphism allows us to use a single function animalSound
to work with different types of animals.
4. Abstraction
Abstraction is the process of hiding the complex implementation details and showing only the necessary features of an object. It helps in reducing programming complexity and effort.
Example of Abstraction:
class Shape {
public:
virtual double area() = 0;
virtual double perimeter() = 0;
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() override {
return 3.14 * radius * radius;
}
double perimeter() override {
return 2 * 3.14 * radius;
}
};
class Rectangle : public Shape {
private:
double length, width;
public:
Rectangle(double l, double w) : length(l), width(w) {}
double area() override {
return length * width;
}
double perimeter() override {
return 2 * (length + width);
}
};
The Shape
class provides an abstraction for different shapes, hiding the specific implementation details of each shape.
Applying OOP in Coding Interviews
Now that we’ve covered the fundamental concepts, let’s explore how to apply these principles in coding interview scenarios. Here are some strategies and examples:
1. Designing a System
Interviewers often ask candidates to design a simple system or application. This is an excellent opportunity to showcase your OOP skills.
Example: Design a Library Management System
class Book {
private:
string title;
string author;
string ISBN;
bool isAvailable;
public:
Book(string t, string a, string isbn) : title(t), author(a), ISBN(isbn), isAvailable(true) {}
void borrowBook() {
if (isAvailable) {
isAvailable = false;
cout << "Book borrowed successfully." << endl;
} else {
cout << "Book is not available." << endl;
}
}
void returnBook() {
isAvailable = true;
cout << "Book returned successfully." << endl;
}
string getTitle() { return title; }
string getAuthor() { return author; }
string getISBN() { return ISBN; }
bool checkAvailability() { return isAvailable; }
};
class Library {
private:
vector<Book> books;
public:
void addBook(Book book) {
books.push_back(book);
}
void searchBook(string title) {
for (const auto& book : books) {
if (book.getTitle() == title) {
cout << "Book found: " << book.getTitle() << " by " << book.getAuthor() << endl;
return;
}
}
cout << "Book not found." << endl;
}
void borrowBook(string ISBN) {
for (auto& book : books) {
if (book.getISBN() == ISBN) {
book.borrowBook();
return;
}
}
cout << "Book not found." << endl;
}
void returnBook(string ISBN) {
for (auto& book : books) {
if (book.getISBN() == ISBN) {
book.returnBook();
return;
}
}
cout << "Book not found." << endl;
}
};
This design demonstrates encapsulation (private member variables), abstraction (hiding complex implementation details), and the use of classes to model real-world entities.
2. Implementing Design Patterns
Knowledge of common design patterns can be a significant advantage in coding interviews. Design patterns are reusable solutions to common problems in software design.
Example: Implementing the Singleton Pattern
class DatabaseConnection {
private:
static DatabaseConnection* instance;
string connectionString;
DatabaseConnection() : connectionString("default_connection_string") {}
public:
static DatabaseConnection* getInstance() {
if (instance == nullptr) {
instance = new DatabaseConnection();
}
return instance;
}
void connect() {
cout << "Connected to database with " << connectionString << endl;
}
void setConnectionString(string cs) {
connectionString = cs;
}
};
DatabaseConnection* DatabaseConnection::instance = nullptr;
This Singleton pattern ensures that only one instance of DatabaseConnection
is created, which can be useful for managing resources like database connections.
3. Solving Algorithm Problems with OOP
While many algorithm problems can be solved without explicitly using OOP, incorporating object-oriented principles can lead to more organized and maintainable solutions.
Example: Implementing a Queue using Two Stacks
class Queue {
private:
stack<int> inbox;
stack<int> outbox;
void transferIfNeeded() {
if (outbox.empty()) {
while (!inbox.empty()) {
outbox.push(inbox.top());
inbox.pop();
}
}
}
public:
void enqueue(int x) {
inbox.push(x);
}
int dequeue() {
transferIfNeeded();
if (outbox.empty()) {
throw runtime_error("Queue is empty");
}
int front = outbox.top();
outbox.pop();
return front;
}
int front() {
transferIfNeeded();
if (outbox.empty()) {
throw runtime_error("Queue is empty");
}
return outbox.top();
}
bool isEmpty() {
return inbox.empty() && outbox.empty();
}
};
This implementation encapsulates the complex logic of using two stacks to implement a queue, providing a clean and easy-to-use interface.
Best Practices for Applying OOP in Interviews
To effectively demonstrate your OOP skills during coding interviews, consider the following best practices:
- Start with a Clear Class Structure: Begin by identifying the main entities in the problem and create appropriate classes for them.
- Use Encapsulation: Keep data private and provide public methods for interaction. This demonstrates your understanding of data protection and interface design.
- Apply Inheritance Wisely: Use inheritance to model “is-a” relationships and avoid deep inheritance hierarchies.
- Leverage Polymorphism: Use polymorphism to create flexible and extensible designs, especially when dealing with collections of objects.
- Implement Interfaces: Use interfaces (or abstract classes) to define contracts and promote loose coupling between components.
- Follow SOLID Principles: Familiarize yourself with SOLID principles (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion) and apply them in your designs.
- Use Design Patterns: Recognize situations where common design patterns can be applied and implement them appropriately.
- Write Clean, Readable Code: Use meaningful variable and method names, keep methods short and focused, and follow consistent coding style.
- Explain Your Thought Process: As you design and implement your solution, explain your reasoning for choosing certain OOP structures and patterns.
- Be Prepared to Discuss Trade-offs: Be ready to discuss the advantages and potential drawbacks of your OOP design choices.
Common OOP Interview Questions
To help you prepare, here are some common OOP-related questions you might encounter in coding interviews:
- What are the four main principles of OOP, and can you explain each one?
- What is the difference between an interface and an abstract class?
- Can you explain the concept of method overloading and method overriding?
- What is the difference between composition and inheritance? When would you use one over the other?
- Can you explain the Liskov Substitution Principle?
- What is the difference between association, aggregation, and composition in OOP?
- How does encapsulation contribute to the concept of data hiding?
- Can you give an example of how you would use polymorphism to solve a real-world problem?
- What are some common design patterns, and when would you use them?
- How does OOP support code reusability?
Conclusion
Mastering object-oriented programming principles is crucial for success in coding interviews, especially when targeting top tech companies. By understanding and applying concepts like encapsulation, inheritance, polymorphism, and abstraction, you can create more robust, maintainable, and scalable solutions to interview problems.
Remember that OOP is not just about writing code in a certain way; it’s a paradigm that helps you think about problems in terms of objects and their interactions. As you practice for interviews, try to approach problems with an OOP mindset, considering how you can model the problem domain using classes and objects.
Lastly, while OOP is important, it’s equally crucial to have a strong foundation in algorithms, data structures, and problem-solving techniques. A well-rounded skill set that combines these elements with strong OOP principles will greatly enhance your chances of success in coding interviews and your career as a software developer.
Keep practicing, stay curious, and always be ready to explain your thought process. With dedication and the right approach, you’ll be well-prepared to showcase your OOP skills in your next coding interview.