How to Handle Failures and Exceptions in Code: A Comprehensive Guide
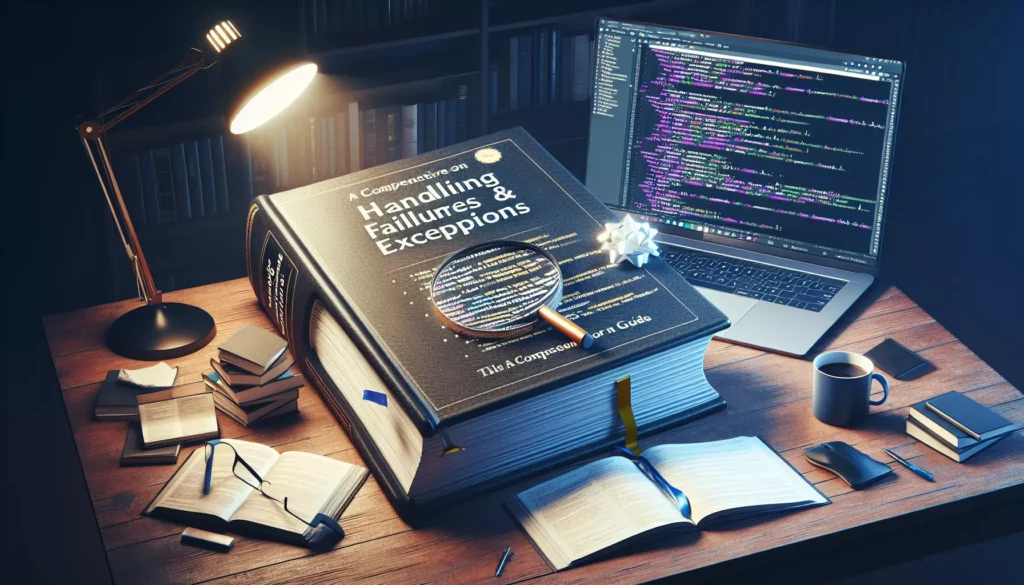
In the world of programming, things don’t always go as planned. Errors occur, unexpected situations arise, and our code needs to be prepared to handle these scenarios gracefully. This is where failure and exception handling come into play. As aspiring developers or those preparing for technical interviews at top tech companies, understanding how to effectively manage errors and exceptions is crucial. In this comprehensive guide, we’ll explore various strategies and best practices for handling failures and exceptions in your code.
Table of Contents
- Understanding Failures and Exceptions
- Types of Exceptions
- Using Try-Catch Blocks
- The Finally Clause
- Creating Custom Exceptions
- Exception Propagation
- Best Practices for Exception Handling
- Language-Specific Exception Handling
- Logging and Monitoring Exceptions
- Testing Exception Handling
- Real-World Examples
- Conclusion
1. Understanding Failures and Exceptions
Before diving into the specifics of handling failures and exceptions, it’s important to understand what they are and why they occur.
What is a Failure?
A failure is a general term that refers to any situation where a program doesn’t perform as expected. This could be due to various reasons, such as invalid input, resource unavailability, or logical errors in the code.
What is an Exception?
An exception is a specific type of failure that occurs during the execution of a program. It’s an event that disrupts the normal flow of the program’s instructions. When an exception occurs, it creates an exception object containing information about the error, which can be caught and handled by the program.
2. Types of Exceptions
Exceptions can be broadly categorized into two types:
Checked Exceptions
These are exceptions that are checked at compile-time. In languages like Java, the compiler requires that these exceptions be either caught or declared in the method signature. Examples include IOException and SQLException.
Unchecked Exceptions
These exceptions occur at runtime and don’t need to be explicitly caught or declared. They usually indicate programming errors that are not easily recoverable. Examples include NullPointerException and ArrayIndexOutOfBoundsException.
3. Using Try-Catch Blocks
The most common way to handle exceptions is by using try-catch blocks. Here’s a basic structure:
try {
// Code that may throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
}
Let’s look at a more concrete example:
public static void divideNumbers(int a, int b) {
try {
int result = a / b;
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Error: Division by zero is not allowed.");
}
}
In this example, if b is zero, an ArithmeticException will be thrown. The catch block catches this exception and prints an error message instead of crashing the program.
4. The Finally Clause
The finally clause is used to execute code regardless of whether an exception was thrown or not. It’s typically used for cleanup operations like closing files or releasing resources.
try {
// Code that may throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
} finally {
// Code that will always execute
}
Here’s an example using file handling:
FileInputStream file = null;
try {
file = new FileInputStream("example.txt");
// Read from file
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
} finally {
if (file != null) {
try {
file.close();
} catch (IOException e) {
System.out.println("Error closing file: " + e.getMessage());
}
}
}
5. Creating Custom Exceptions
Sometimes, built-in exceptions don’t adequately describe the error condition in your application. In such cases, you can create custom exceptions. Here’s how you might create a custom exception in Java:
public class InvalidAgeException extends Exception {
public InvalidAgeException(String message) {
super(message);
}
}
public class Person {
private int age;
public void setAge(int age) throws InvalidAgeException {
if (age < 0 || age > 150) {
throw new InvalidAgeException("Age must be between 0 and 150");
}
this.age = age;
}
}
Now you can use this custom exception in your code:
try {
Person person = new Person();
person.setAge(200);
} catch (InvalidAgeException e) {
System.out.println("Error: " + e.getMessage());
}
6. Exception Propagation
Exception propagation refers to the process of an exception being passed up through the call stack until it’s caught by an appropriate exception handler. If an exception is not caught, it will eventually reach the top of the call stack and terminate the program.
Here’s an example to illustrate exception propagation:
public void method1() throws Exception {
method2();
}
public void method2() throws Exception {
method3();
}
public void method3() throws Exception {
throw new Exception("Exception in method3");
}
public static void main(String[] args) {
try {
method1();
} catch (Exception e) {
System.out.println("Caught exception: " + e.getMessage());
}
}
In this example, the exception is thrown in method3, propagates through method2 and method1, and is finally caught in the main method.
7. Best Practices for Exception Handling
Here are some best practices to follow when handling exceptions:
- Be specific: Catch the most specific exception possible. Avoid catching generic exceptions like Exception unless absolutely necessary.
- Don’t catch exceptions you can’t handle: If you can’t do anything meaningful with an exception, let it propagate up the call stack.
- Log exceptions: Always log exceptions with enough context to understand what went wrong and where.
- Clean up resources: Use try-with-resources or finally blocks to ensure resources are properly cleaned up.
- Don’t use exceptions for flow control: Exceptions should be used for exceptional circumstances, not for normal program flow.
- Provide informative error messages: Exception messages should be clear and provide useful information about what went wrong.
8. Language-Specific Exception Handling
Different programming languages handle exceptions in slightly different ways. Let’s look at a few examples:
Python
Python uses a try-except block for exception handling:
try:
x = 1 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
else:
print("Division successful")
finally:
print("This always executes")
JavaScript
JavaScript uses try-catch-finally:
try {
throw new Error("Something went wrong");
} catch (error) {
console.error(error.message);
} finally {
console.log("This always executes");
}
C#
C# uses a try-catch-finally structure similar to Java:
try
{
int result = 10 / int.Parse("0");
}
catch (DivideByZeroException ex)
{
Console.WriteLine($"Caught divide by zero exception: {ex.Message}");
}
catch (FormatException ex)
{
Console.WriteLine($"Caught format exception: {ex.Message}");
}
finally
{
Console.WriteLine("This always executes");
}
9. Logging and Monitoring Exceptions
Proper logging of exceptions is crucial for debugging and maintaining software. Here are some tips for effective exception logging:
- Log the full stack trace, not just the exception message
- Include contextual information (e.g., user ID, transaction ID)
- Use appropriate log levels (e.g., ERROR for exceptions, WARN for caught and handled exceptions)
- Consider using a logging framework like Log4j or SLF4J
Here’s an example using Java’s built-in logging:
import java.util.logging.*;
public class ExceptionLoggingExample {
private static final Logger logger = Logger.getLogger(ExceptionLoggingExample.class.getName());
public static void main(String[] args) {
try {
throw new RuntimeException("An example exception");
} catch (RuntimeException e) {
logger.log(Level.SEVERE, "An exception occurred", e);
}
}
}
10. Testing Exception Handling
Testing exception handling is an important part of ensuring your code behaves correctly in error scenarios. Most testing frameworks provide ways to test for expected exceptions. Here’s an example using JUnit in Java:
import org.junit.Test;
import static org.junit.Assert.*;
public class DivisionTest {
@Test(expected = ArithmeticException.class)
public void testDivisionByZero() {
int result = 10 / 0;
}
}
This test will pass if an ArithmeticException is thrown, and fail otherwise.
11. Real-World Examples
Let’s look at some real-world scenarios where exception handling is crucial:
File Processing
public static List<String> readLinesFromFile(String filename) {
List<String> lines = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new FileReader(filename))) {
String line;
while ((line = reader.readLine()) != null) {
lines.add(line);
}
} catch (FileNotFoundException e) {
System.err.println("File not found: " + filename);
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
return lines;
}
Database Operations
public void updateUserEmail(int userId, String newEmail) {
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DriverManager.getConnection("jdbc:mysql://localhost/mydb", "user", "password");
pstmt = conn.prepareStatement("UPDATE users SET email = ? WHERE id = ?");
pstmt.setString(1, newEmail);
pstmt.setInt(2, userId);
pstmt.executeUpdate();
} catch (SQLException e) {
System.err.println("Database error: " + e.getMessage());
} finally {
try {
if (pstmt != null) pstmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
System.err.println("Error closing database connection: " + e.getMessage());
}
}
}
API Calls
public String fetchDataFromApi(String url) {
try {
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create(url))
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
return response.body();
} catch (IOException e) {
System.err.println("Network error: " + e.getMessage());
} catch (InterruptedException e) {
System.err.println("Request interrupted: " + e.getMessage());
Thread.currentThread().interrupt();
}
return null;
}
12. Conclusion
Handling failures and exceptions is a crucial skill for any programmer. It’s not just about preventing your program from crashing; it’s about creating robust, reliable software that can gracefully handle unexpected situations. By following the practices outlined in this guide, you’ll be well-equipped to write code that’s more resilient and easier to maintain.
Remember, exception handling is not just about catching errors—it’s about anticipating potential issues, providing meaningful feedback, and ensuring your application can recover or fail gracefully. As you prepare for technical interviews or work on real-world projects, keep these principles in mind, and you’ll be well on your way to writing more robust and reliable code.
Practice implementing these concepts in your own projects, and don’t be afraid to dive deeper into language-specific exception handling features. The more you work with exceptions, the more natural it will become to write code that’s prepared for the unexpected.