Understanding Client-Server Architecture for System Design
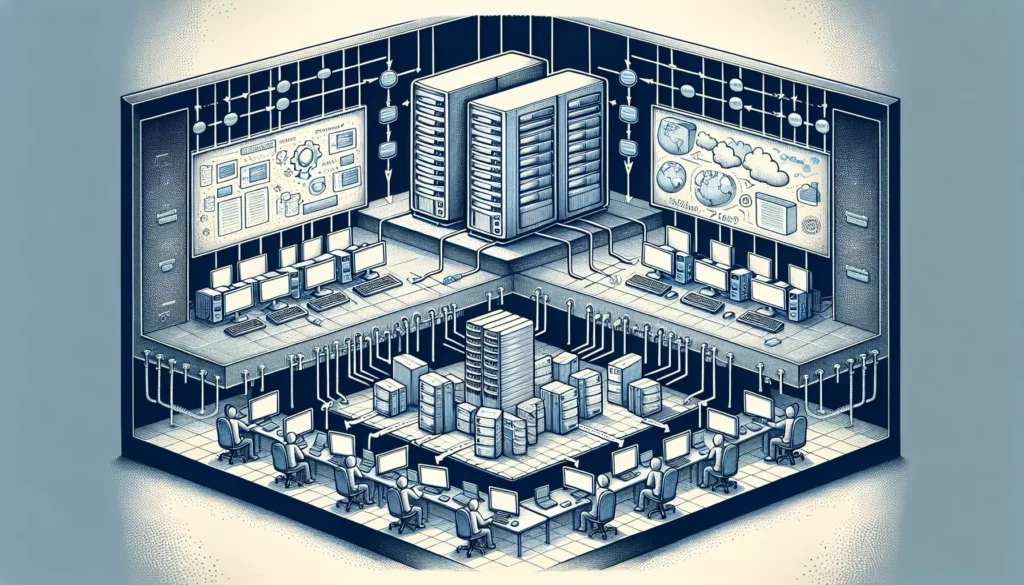
In the world of modern software development and system design, understanding client-server architecture is crucial. This fundamental concept forms the backbone of many applications and services we use daily, from web browsers to mobile apps and enterprise systems. As aspiring developers and software engineers, particularly those aiming for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), it’s essential to have a solid grasp of this architecture. In this comprehensive guide, we’ll dive deep into client-server architecture, exploring its components, benefits, challenges, and real-world applications.
What is Client-Server Architecture?
Client-server architecture is a computing model that divides tasks or workloads between providers of a resource or service, called servers, and service requesters, called clients. This model is widely used in network computing and forms the basis of many distributed systems.
In simple terms, the client is typically a user’s device or application that requests data or services, while the server is a powerful computer or system that stores, processes, and delivers the requested information or services to the client.
Key Components of Client-Server Architecture
- Client: The end-user device or application that initiates requests for data or services.
- Server: The system that receives requests, processes them, and sends back responses.
- Network: The communication medium that allows clients and servers to exchange information.
How Client-Server Architecture Works
The basic flow of client-server architecture can be broken down into the following steps:
- The client sends a request to the server over the network.
- The server receives and processes the request.
- The server generates a response based on the request.
- The server sends the response back to the client over the network.
- The client receives and processes the response, typically displaying the result to the user.
This process is often cyclical, with clients continuously sending requests and servers responding as needed.
Types of Client-Server Architecture
There are several types of client-server architectures, each with its own characteristics and use cases:
1. Two-Tier Architecture
In this simple model, the client directly communicates with the server without any intermediary. The client handles the user interface and business logic, while the server manages data storage and retrieval.
2. Three-Tier Architecture
This model introduces an additional layer between the client and the server, typically called the application server or middleware. The three tiers are:
- Presentation tier (client)
- Application tier (middleware)
- Data tier (server)
This architecture allows for better scalability and separation of concerns.
3. N-Tier Architecture
An extension of the three-tier model, N-tier architecture involves multiple layers of servers, each responsible for specific functions. This approach is often used in large-scale enterprise applications.
Advantages of Client-Server Architecture
Client-server architecture offers several benefits that make it a popular choice for many applications:
- Centralized data management: Servers can store and manage data centrally, ensuring consistency and easier backups.
- Enhanced security: Servers can implement robust security measures to protect sensitive data and control access.
- Scalability: It’s easier to scale server resources to handle increased load without affecting clients.
- Resource sharing: Multiple clients can access shared resources and services efficiently.
- Flexibility: Clients and servers can be upgraded independently, allowing for easier maintenance and updates.
Challenges in Client-Server Architecture
While client-server architecture has many advantages, it also comes with some challenges:
- Network dependence: The system relies heavily on network connectivity, which can be a single point of failure.
- Server overload: If not properly designed, servers can become overwhelmed by too many client requests.
- Complexity: As the system grows, managing multiple clients and servers can become complex.
- Cost: Implementing and maintaining robust server infrastructure can be expensive.
- Latency: Network latency can affect the responsiveness of client-server interactions, especially over long distances.
Real-World Applications of Client-Server Architecture
Client-server architecture is ubiquitous in modern computing. Here are some common applications:
1. Web Applications
Web browsers act as clients, sending requests to web servers that host websites and web applications. This is perhaps the most common example of client-server architecture in action.
2. Email Systems
Email clients (like Outlook or Gmail) communicate with email servers to send, receive, and store messages.
3. Database Management Systems
Database clients send queries to database servers, which process the requests and return the requested data.
4. File Servers
In corporate networks, file servers store and manage shared documents and files that can be accessed by multiple client computers.
5. Online Gaming
Game clients on players’ devices communicate with game servers that manage the game state, player interactions, and other game-related data.
Implementing Client-Server Architecture: Key Considerations
When designing systems using client-server architecture, there are several important factors to consider:
1. Protocol Selection
Choosing the right communication protocol is crucial. Common protocols include HTTP/HTTPS for web applications, SMTP for email, and custom protocols for specific applications. The choice depends on factors like security requirements, data format, and performance needs.
2. Load Balancing
To handle high traffic and ensure high availability, implementing load balancing techniques is essential. This involves distributing incoming client requests across multiple servers to optimize resource utilization and minimize response times.
3. Caching
Implementing caching mechanisms on both client and server sides can significantly improve performance by reducing the need for frequent data transfers.
4. Security Measures
Robust security measures are crucial in client-server systems. This includes encryption for data in transit, authentication and authorization mechanisms, and protection against common attacks like SQL injection and cross-site scripting (XSS).
5. Scalability Planning
Designing the system with scalability in mind is important. This might involve using cloud services, implementing microservices architecture, or designing for horizontal scaling.
Client-Server vs. Peer-to-Peer Architecture
It’s worth comparing client-server architecture with another popular model: peer-to-peer (P2P) architecture.
Client-Server Architecture:
- Centralized control and management
- Clear distinction between service providers and consumers
- Easier to secure and manage
- Can become a bottleneck if not properly scaled
Peer-to-Peer Architecture:
- Decentralized network where each node can be both client and server
- More resilient to failures as there’s no single point of failure
- Can be more challenging to manage and secure
- Often used in file-sharing applications and blockchain networks
Future Trends in Client-Server Architecture
As technology evolves, so does client-server architecture. Here are some trends shaping its future:
1. Serverless Computing
Serverless architectures abstract away server management, allowing developers to focus solely on writing code. While not truly “serverless,” this model changes how we think about client-server interactions.
2. Edge Computing
By moving computation closer to the data source or the end-user, edge computing aims to reduce latency and improve performance in client-server systems.
3. Microservices
Breaking down server-side applications into smaller, independently deployable services allows for more flexibility and scalability in client-server architectures.
4. AI and Machine Learning Integration
Incorporating AI and ML models into client-server systems is becoming more common, enabling more intelligent and adaptive applications.
Implementing a Simple Client-Server Application
To better understand client-server architecture, let’s look at a simple example using Python. We’ll create a basic server that responds to client requests with a “Hello, World!” message.
Server Code:
import socket
def start_server():
host = '127.0.0.1' # localhost
port = 12345 # Choose a port number
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind((host, port))
server_socket.listen(1)
print(f"Server listening on {host}:{port}")
while True:
client_socket, address = server_socket.accept()
print(f"Connection from {address}")
client_socket.send("Hello, World!".encode())
client_socket.close()
if __name__ == "__main__":
start_server()
Client Code:
import socket
def connect_to_server():
host = '127.0.0.1' # localhost
port = 12345 # Same port as the server
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect((host, port))
message = client_socket.recv(1024).decode()
print(f"Received from server: {message}")
client_socket.close()
if __name__ == "__main__":
connect_to_server()
In this example, the server listens for incoming connections and sends a “Hello, World!” message to any client that connects. The client connects to the server, receives the message, and prints it.
Best Practices for Client-Server Architecture
When working with client-server architecture, especially in the context of preparing for technical interviews at major tech companies, keep these best practices in mind:
- Separation of Concerns: Clearly define the responsibilities of the client and server components.
- Stateless Design: Whenever possible, design server-side components to be stateless, improving scalability and reliability.
- Efficient Communication: Optimize data transfer between client and server, using compression and efficient data formats when appropriate.
- Error Handling: Implement robust error handling and logging on both client and server sides.
- Security First: Always prioritize security in your design, considering aspects like data encryption, authentication, and authorization.
- Performance Optimization: Use techniques like caching, connection pooling, and asynchronous processing to improve system performance.
- Scalability Planning: Design your system with future growth in mind, considering how it will handle increased load and data volume.
Conclusion
Client-server architecture is a fundamental concept in system design, powering many of the applications and services we use daily. Understanding its principles, advantages, challenges, and best practices is crucial for any aspiring software engineer, especially those aiming for positions at major tech companies.
As you continue your journey in coding education and programming skills development, remember that client-server architecture is just one piece of the puzzle. It’s essential to also focus on algorithmic thinking, problem-solving, and practical coding skills. Platforms like AlgoCademy can provide valuable resources and interactive tutorials to help you master these concepts and prepare for technical interviews.
By combining a strong understanding of architectural principles like client-server with solid coding skills and problem-solving abilities, you’ll be well-equipped to tackle the challenges of modern software development and excel in your career as a software engineer.