Managing Your Time Wisely in a Coding Interview
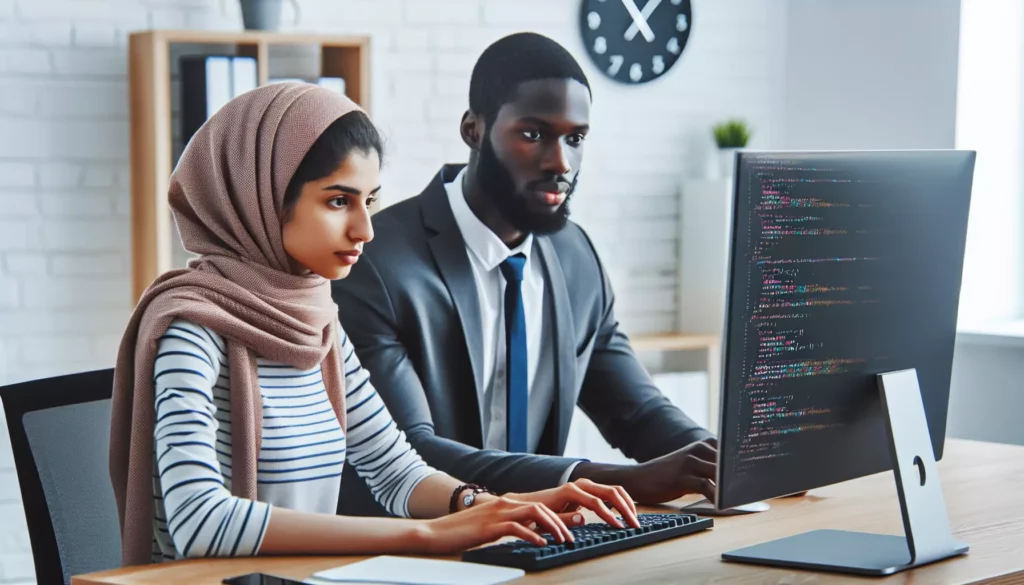
When it comes to coding interviews, particularly those for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), time management is crucial. The ability to solve complex problems efficiently within a limited timeframe can make or break your chances of landing that dream job. In this comprehensive guide, we’ll explore strategies and techniques to help you manage your time wisely during a coding interview, ensuring you showcase your skills effectively and maximize your chances of success.
Understanding the Importance of Time Management in Coding Interviews
Before diving into specific strategies, it’s essential to understand why time management is so critical in coding interviews:
- Limited Duration: Most coding interviews have a fixed time limit, typically ranging from 45 minutes to an hour.
- Multiple Components: Interviews often include various elements such as problem-solving, coding implementation, and discussions about your approach.
- Pressure Simulation: Time constraints simulate real-world pressure, allowing interviewers to assess how you perform under stress.
- Efficiency Evaluation: Your ability to manage time effectively reflects on your potential performance in actual work scenarios.
Pre-Interview Preparation
Effective time management during the interview starts long before you enter the room or join the video call. Here are some key preparation steps:
1. Practice Timed Problem-Solving
Regularly solve coding problems under timed conditions to improve your speed and accuracy. Platforms like AlgoCademy offer timed coding challenges that simulate interview conditions, helping you get comfortable with the pressure.
2. Review Common Algorithms and Data Structures
Familiarize yourself with frequently used algorithms and data structures. This knowledge will help you quickly identify appropriate solutions during the interview, saving valuable time.
3. Study Time Complexities
Understanding the time and space complexities of different algorithms is crucial. This knowledge will help you make quick decisions about which approach to use based on the problem’s constraints.
4. Mock Interviews
Participate in mock interviews with peers or mentors. This practice will help you get accustomed to explaining your thought process while coding, a skill that can save time during the actual interview.
Strategies for Time Management During the Interview
Now that you’re prepared, let’s explore strategies to manage your time effectively during the coding interview:
1. Clarify the Problem (2-3 minutes)
Start by ensuring you fully understand the problem. Ask clarifying questions about:
- Input format and constraints
- Expected output
- Edge cases or special considerations
- Any assumptions you can make
This initial investment of time can save you from going down the wrong path later.
2. Outline Your Approach (3-5 minutes)
Before diving into coding, briefly outline your approach:
- Describe the high-level algorithm you plan to use
- Discuss potential data structures
- Mention any trade-offs or alternative approaches you’re considering
This step allows the interviewer to provide early feedback and potentially guide you towards a more optimal solution.
3. Start with a Brute Force Solution (5-10 minutes)
If the optimal solution isn’t immediately apparent, start with a brute force approach:
- Quickly implement a basic solution that solves the problem
- Discuss the time and space complexity of this approach
- Use this as a foundation to optimize further
Having a working solution, even if not optimal, is better than no solution at all.
4. Optimize Your Solution (10-15 minutes)
Once you have a basic solution, look for ways to optimize:
- Identify bottlenecks in your current approach
- Consider using more efficient data structures
- Look for opportunities to reduce time complexity
- Discuss trade-offs between time and space complexity
Remember to think out loud during this process, as your problem-solving approach is often as important as the final solution.
5. Implement the Optimized Solution (15-20 minutes)
Now, implement your optimized solution:
- Write clean, readable code
- Use meaningful variable and function names
- Add comments to explain complex parts of your code
- Handle edge cases and potential errors
If you’re running short on time, it’s okay to use pseudocode for parts of your solution, explaining how you would implement it in detail.
6. Test Your Code (5-10 minutes)
Always allocate time for testing:
- Run through a few test cases, including edge cases
- Explain your testing approach to the interviewer
- Fix any bugs you find during testing
Demonstrating your ability to test and debug your own code is a valuable skill.
7. Discuss Improvements and Extensions (Remaining time)
If you have time left:
- Discuss potential further optimizations
- Consider how your solution might scale with larger inputs
- Think about how you might extend the solution for related problems
This shows your ability to think beyond the immediate problem and consider broader implications.
Time Management Tips and Tricks
Here are some additional tips to help you manage your time effectively during a coding interview:
1. Use the “Think Aloud” Technique
Constantly verbalize your thoughts and reasoning. This not only helps the interviewer understand your problem-solving process but also keeps you focused and on track.
2. Set Mental Checkpoints
As you progress through the interview, set mental checkpoints for yourself. For example:
- By 10 minutes: Have a clear understanding of the problem and a high-level approach
- By 20 minutes: Have at least a brute force solution implemented
- By 40 minutes: Have an optimized solution implemented and partially tested
These checkpoints can help you gauge your progress and adjust your pace if necessary.
3. Use Pseudocode Strategically
If you’re running short on time, it’s acceptable to use pseudocode for parts of your solution. Explain your approach in detail and focus on implementing the most critical or complex parts of the algorithm.
4. Ask for Hints Wisely
If you’re stuck, don’t hesitate to ask for hints. However, be strategic about when and how you ask:
- First, explain what you’ve tried and why you think it didn’t work
- Ask specific questions rather than general ones
- Use hints as a guide, not a complete solution
5. Prioritize Correctness Over Perfection
While optimization is important, having a working solution is crucial. If time is running short, focus on implementing a correct solution, even if it’s not the most efficient. You can discuss potential optimizations afterwards.
6. Use Built-in Functions and Libraries
Don’t waste time reinventing the wheel. If your chosen programming language has built-in functions or libraries that can help solve part of the problem, use them. Just make sure to explain why you’re using them and understand their time complexity.
7. Practice Time-Boxing
Time-boxing is a technique where you allocate a fixed amount of time to a task. Practice this technique during your preparation:
def time_box_example(task, time_limit):
start_time = time.time()
result = None
while time.time() - start_time < time_limit:
# Work on the task
result = do_work(task)
if result is not None:
break
return result
This approach can help you avoid getting stuck on a single aspect of the problem for too long.
Common Time Management Pitfalls to Avoid
Being aware of common time management mistakes can help you avoid them during your interview:
1. Diving into Coding Too Quickly
Resist the urge to start coding immediately. Spending a few minutes understanding the problem and planning your approach can save you time in the long run.
2. Getting Stuck on Optimization
While it’s important to consider efficiency, don’t get bogged down trying to find the perfect solution. Start with a working solution and optimize incrementally.
3. Neglecting to Test
Always allocate time for testing, even if it means having a slightly less optimized solution. Demonstrating your testing skills is valuable to interviewers.
4. Losing Track of Time
It’s easy to lose track of time when you’re focused on a problem. Consider using a small timer or asking the interviewer for time updates.
5. Over-Explaining Simple Concepts
While it’s important to explain your thought process, don’t waste time explaining basic programming concepts. Focus on the unique aspects of your solution.
6. Perfectionism
Remember that interviewers are more interested in your problem-solving process than a perfect solution. It’s okay if your code isn’t production-ready.
Adapting to Different Interview Formats
Time management strategies may need to be adjusted based on the interview format:
In-Person Interviews
- Use the whiteboard effectively to outline your approach
- Practice writing code by hand to improve speed and legibility
- Be mindful of your body language and maintain engagement with the interviewer
Remote Interviews
- Familiarize yourself with the coding platform beforehand
- Ensure your internet connection is stable
- Use screen sharing effectively to explain your thought process
Take-Home Coding Assignments
- Set a personal time limit to simulate interview conditions
- Focus on code quality and documentation, as you have more time
- Include a detailed explanation of your approach and any assumptions made
Post-Interview Reflection
After each interview or practice session, take time to reflect on your time management:
- Which parts of the problem took longer than expected?
- Were there any areas where you could have saved time?
- How well did you balance explanation and coding?
- Did you manage to cover all aspects of the problem in the given time?
Use these reflections to refine your approach for future interviews.
Conclusion
Effective time management in coding interviews is a skill that can be developed with practice and the right strategies. By preparing thoroughly, structuring your approach, and staying mindful of time during the interview, you can showcase your problem-solving and coding abilities to their fullest potential.
Remember, the goal is not just to solve the problem, but to demonstrate your thought process, coding skills, and ability to work under pressure. With these time management strategies in your toolkit, you’ll be well-equipped to tackle even the most challenging coding interviews at top tech companies.
Keep practicing, stay confident, and remember that each interview is an opportunity to learn and improve. Good luck with your coding interviews!