Approaching Coding Problems You’ve Never Seen Before: A Comprehensive Guide
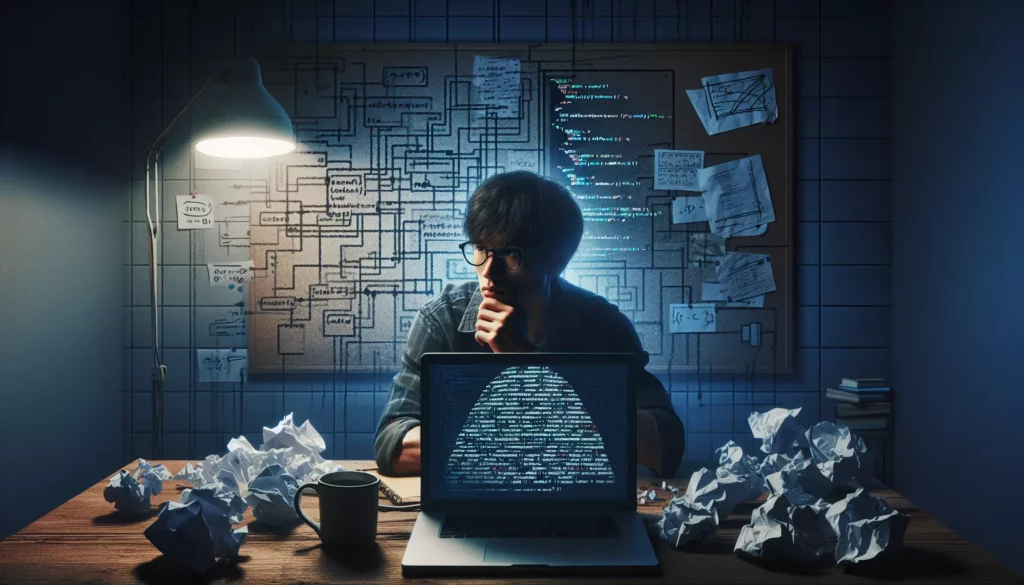
In the ever-evolving world of programming, encountering new and unfamiliar coding problems is a common occurrence. Whether you’re a beginner taking your first steps into the world of algorithms or an experienced developer preparing for technical interviews at top tech companies, the ability to tackle novel challenges is a crucial skill. This comprehensive guide will walk you through effective strategies for approaching coding problems you’ve never seen before, helping you build confidence and problem-solving prowess.
1. Understanding the Importance of Problem-Solving Skills
Before diving into specific strategies, it’s essential to recognize why being able to approach unfamiliar coding problems is so valuable:
- Career Growth: Technical interviews, especially at FAANG companies (Facebook, Amazon, Apple, Netflix, Google), often involve solving unique problems to assess a candidate’s problem-solving abilities.
- Adaptability: The tech industry is constantly evolving, and new challenges arise regularly. Being able to tackle unfamiliar problems helps you stay relevant and adaptable.
- Confidence Building: Successfully solving new problems boosts your confidence and reinforces your ability to learn and grow as a programmer.
- Creativity Enhancement: Dealing with novel challenges fosters creativity and helps you think outside the box, leading to innovative solutions.
2. The Problem-Solving Framework
When faced with a new coding problem, following a structured approach can significantly improve your chances of success. Here’s a step-by-step framework to guide you:
2.1. Read and Understand the Problem
The first and most crucial step is to thoroughly read and understand the problem statement. This involves:
- Identifying the input and output requirements
- Noting any constraints or special conditions
- Recognizing the problem’s core objective
Take your time with this step, as a misunderstanding of the problem can lead you down the wrong path.
2.2. Analyze the Given Examples
Most coding problems come with example inputs and outputs. Analyze these carefully to:
- Verify your understanding of the problem
- Identify patterns or relationships between inputs and outputs
- Consider edge cases or special scenarios
2.3. Break Down the Problem
Complex problems can be overwhelming. Break them down into smaller, manageable sub-problems. This approach, known as “divide and conquer,” makes the overall task less daunting and helps you focus on solving one piece at a time.
2.4. Brainstorm Potential Approaches
Before diving into coding, take some time to brainstorm different approaches to solve the problem. Consider:
- Brute force solutions
- Optimized algorithms
- Data structures that might be useful
- Any similar problems you’ve encountered before
2.5. Choose an Approach and Plan Your Solution
Select the most promising approach based on your brainstorming session. Then, create a high-level plan or pseudocode outlining the steps of your solution. This serves as a roadmap for your implementation.
2.6. Implement Your Solution
With your plan in place, start coding your solution. Focus on writing clean, readable code, even if it’s not the most optimized version. You can always refine it later.
2.7. Test and Debug
Once you have a working solution, test it thoroughly. Use the provided examples, create your own test cases, and consider edge cases. Debug any issues you encounter.
2.8. Optimize and Refine
If time allows, look for ways to optimize your solution. This might involve improving the time or space complexity, enhancing readability, or adding comments for clarity.
3. Strategies for Tackling Unfamiliar Problems
While the framework above provides a solid foundation, here are some additional strategies to help you approach new coding problems effectively:
3.1. Start with What You Know
Even if a problem seems entirely new, there’s often something familiar you can use as a starting point. Look for elements that remind you of problems you’ve solved before or concepts you’re comfortable with.
3.2. Use Visualization Techniques
Drawing diagrams, flowcharts, or visual representations of the problem can help you understand it better and spark ideas for solutions. Tools like pen and paper or digital whiteboarding apps can be invaluable.
3.3. Think Aloud
Verbalizing your thought process, even if you’re working alone, can help clarify your thinking and reveal new insights. This technique is particularly useful in interview settings, where communicating your approach is as important as solving the problem.
3.4. Leverage Data Structures and Algorithms Knowledge
A strong foundation in common data structures (arrays, linked lists, trees, graphs, etc.) and algorithms (sorting, searching, dynamic programming, etc.) can provide valuable tools for tackling new problems. Often, unfamiliar problems can be solved using familiar concepts in novel ways.
3.5. Use the Process of Elimination
If you’re unsure which approach to take, start by eliminating options that definitely won’t work. This can help narrow down your choices and guide you towards a viable solution.
3.6. Break Constraints
Sometimes, temporarily ignoring certain constraints can help you see the problem from a new perspective. Once you have a basic solution, you can then work on meeting all the requirements.
3.7. Look for Patterns and Analogies
Try to identify patterns in the problem or draw analogies to real-world scenarios. This can often lead to insights about potential solutions.
4. Common Pitfalls to Avoid
When approaching new coding problems, be aware of these common pitfalls:
- Rushing to Code: Resist the urge to start coding immediately. Proper planning can save time in the long run.
- Ignoring Edge Cases: Always consider extreme or unusual inputs that might break your solution.
- Overcomplicating the Solution: Sometimes, the simplest approach is the best. Don’t overcomplicate things unnecessarily.
- Failing to Communicate: In interview settings, remember to explain your thought process clearly, even if you’re struggling.
- Getting Stuck: If you’re truly stuck, don’t be afraid to ask for hints or clarification. In real-world scenarios, utilizing resources like documentation or asking colleagues for input is a valuable skill.
5. Practical Examples
Let’s walk through two examples of approaching unfamiliar coding problems using the strategies discussed above.
Example 1: Finding the Longest Palindromic Substring
Problem Statement: Given a string s, find the longest palindromic substring in s.
Approach:
- Understand the Problem: We need to find the longest substring that reads the same forwards and backwards.
- Analyze Examples: Input: “babad” Output: “bab” or “aba” (both are valid)
- Break Down the Problem: We need to check all substrings and determine if they’re palindromes.
- Brainstorm Approaches: Brute force (check all substrings), dynamic programming, or expand around center.
- Choose an Approach: Let’s use the “expand around center” approach for its simplicity and efficiency.
- Implement the Solution:
def longestPalindrome(s: str) -> str:
def expand_around_center(left: int, right: int) -> str:
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return s[left+1:right]
result = ""
for i in range(len(s)):
# Odd length palindromes
palindrome1 = expand_around_center(i, i)
if len(palindrome1) > len(result):
result = palindrome1
# Even length palindromes
palindrome2 = expand_around_center(i, i+1)
if len(palindrome2) > len(result):
result = palindrome2
return result
Test and Debug: Test with various inputs, including edge cases like empty strings or single-character strings.
Optimize: This solution has a time complexity of O(n^2) and space complexity of O(1), which is already quite efficient for this problem.
Example 2: Merge K Sorted Lists
Problem Statement: Merge k sorted linked lists and return it as one sorted list.
Approach:
- Understand the Problem: We need to combine multiple sorted linked lists into a single sorted linked list.
- Analyze Examples: Input: [[1,4,5],[1,3,4],[2,6]] Output: [1,1,2,3,4,4,5,6]
- Break Down the Problem: We need to compare the heads of all lists and repeatedly select the smallest element.
- Brainstorm Approaches: Brute force (compare all heads), use a min-heap, or merge lists two at a time.
- Choose an Approach: Let’s use a min-heap for its efficiency in finding the minimum element.
- Implement the Solution:
import heapq
from typing import List, Optional
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def mergeKLists(lists: List[Optional[ListNode]]) -> Optional[ListNode]:
# Custom comparison for ListNode objects
ListNode.__lt__ = lambda self, other: self.val < other.val
dummy = ListNode(0)
current = dummy
heap = []
# Add the head of each list to the heap
for i, lst in enumerate(lists):
if lst:
heapq.heappush(heap, (lst.val, i, lst))
while heap:
val, i, node = heapq.heappop(heap)
current.next = node
current = current.next
if node.next:
heapq.heappush(heap, (node.next.val, i, node.next))
return dummy.next
Test and Debug: Test with various inputs, including edge cases like empty lists or lists of different lengths.
Optimize: This solution has a time complexity of O(N log k), where N is the total number of nodes and k is the number of lists. The space complexity is O(k) for the heap.
6. Leveraging AI-Powered Assistance
In today’s tech landscape, AI-powered coding assistants can be valuable tools when approaching unfamiliar problems. Platforms like AlgoCademy offer AI-driven features that can provide hints, suggest optimal data structures, or even break down complex problems into more manageable steps.
While these tools can be incredibly helpful, it’s important to use them judiciously:
- Use AI as a Guide, Not a Crutch: AI assistants should supplement your problem-solving skills, not replace them.
- Verify AI Suggestions: Always understand and verify any suggestions or solutions provided by AI.
- Learn from AI Explanations: Use AI-generated explanations to deepen your understanding of algorithms and problem-solving techniques.
- Practice Independent Problem-Solving: While AI can be helpful, make sure to also practice solving problems independently to build your skills.
7. Continuous Improvement and Learning
Approaching unfamiliar coding problems is a skill that improves with practice. Here are some strategies for continuous improvement:
- Regular Practice: Solve coding problems regularly, even when you’re not preparing for interviews.
- Diverse Problem Types: Expose yourself to a wide range of problem types and difficulty levels.
- Code Reviews: Have your solutions reviewed by peers or mentors to gain new perspectives.
- Study Optimal Solutions: After solving a problem, study alternative or more optimal solutions to expand your problem-solving toolkit.
- Analyze Real-World Code: Study open-source projects to see how experienced developers approach complex problems.
- Participate in Coding Competitions: Platforms like LeetCode, HackerRank, or Codeforces offer competitive coding environments that can sharpen your skills.
8. Conclusion
Approaching coding problems you’ve never seen before can be challenging, but with the right strategies and mindset, it can also be an exciting opportunity for growth. By following a structured problem-solving framework, leveraging your existing knowledge, and continuously expanding your skills, you’ll be well-equipped to tackle even the most unfamiliar coding challenges.
Remember that struggling with new problems is a natural part of the learning process. Embrace the challenge, stay persistent, and view each unfamiliar problem as an opportunity to expand your coding repertoire. With practice and dedication, you’ll develop the confidence and skills needed to approach any coding problem, whether in a technical interview, a professional setting, or your personal projects.
As you continue your journey in coding education and skills development, platforms like AlgoCademy can provide valuable resources, interactive tutorials, and AI-powered assistance to support your growth. By combining these tools with your own determination and problem-solving strategies, you’ll be well on your way to mastering the art of approaching unfamiliar coding problems.