Mastering Techniques for Solving Binary Tree Problems
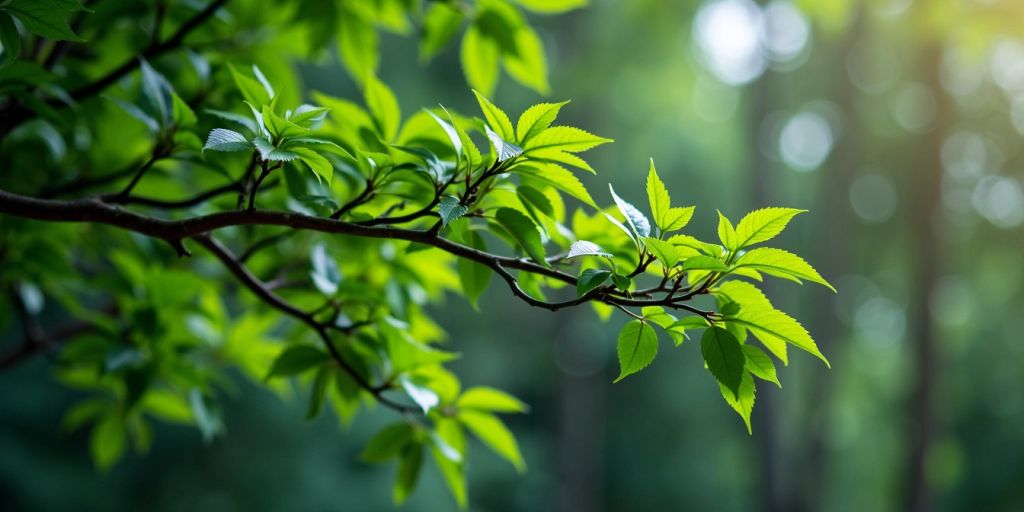
Binary trees are an essential concept in computer science, often appearing in coding interviews and real-world applications. Understanding how to navigate and manipulate these structures can greatly enhance your problem-solving skills. This article will guide you through the key techniques for mastering binary tree problems, from basic definitions to advanced strategies.
Key Takeaways
- Binary trees have unique properties and types that are crucial for solving problems.
- Traversal methods like in-order, pre-order, and post-order are key to accessing tree data.
- Recursion is often the most effective way to solve tree-related issues due to its natural fit with tree structures.
- Iterative solutions are useful in some cases, offering a different approach to tree problems.
- Balancing a binary tree is essential for maintaining efficiency in operations like searching and inserting.
Understanding Binary Trees
Definition and Properties
A binary tree is a data structure where each node can have at most two children, known as the left and right child. The main properties of binary trees include:
- Each node contains a value.
- The left child must have a value less than its parent node.
- The right child must have a value greater than its parent node.
Types of Binary Trees
There are several types of binary trees, including:
- Full Binary Tree: Every node has 0 or 2 children.
- Complete Binary Tree: All levels are fully filled except possibly the last level.
- Perfect Binary Tree: All internal nodes have two children, and all leaf nodes are at the same level.
Common Operations on Binary Trees
Binary trees support various operations, such as:
- Insertion: Adding a new node to the tree.
- Deletion: Removing a node from the tree.
- Traversal: Visiting all nodes in a specific order.
Understanding binary trees is crucial for mastering data structures and algorithms. They are widely used in various applications, including databases and file systems.
In summary, binary trees are fundamental structures in computer science, and their properties and operations are essential for solving complex problems. Mastering these concepts will greatly enhance your programming skills.
Traversing Binary Trees
Tree traversal techniques are essential for working with binary trees. Tree traversal refers to the process of visiting or accessing each node of the tree exactly once in a certain order. There are several methods to traverse a binary tree, each serving different purposes.
In-Order Traversal
In-Order Traversal visits nodes in the following order:
- Left subtree
- Current node
- Right subtree
This method is commonly used to retrieve values in a sorted manner for Binary Search Trees (BST).
Pre-Order Traversal
In Pre-Order Traversal, the order is:
- Current node
- Left subtree
- Right subtree
This technique is useful for creating a copy of the tree or for serialization.
Post-Order Traversal
Post-Order Traversal follows this order:
- Left subtree
- Right subtree
- Current node
This method is often used for deleting a tree or evaluating expressions.
Level-Order Traversal
Level-Order Traversal visits nodes level by level, starting from the root. It uses a queue to keep track of nodes to visit next. This method is particularly useful for finding the shortest path in unweighted trees.
Traversal Type | Order of Visit | Use Case |
---|---|---|
In-Order | Left, Node, Right | Sorted output for BSTs |
Pre-Order | Node, Left, Right | Copying trees, serialization |
Post-Order | Left, Right, Node | Deleting trees, evaluating expressions |
Level-Order | Level by level | Finding shortest paths |
Traversing a binary tree is a fundamental skill that helps in solving various problems efficiently.
Understanding these traversal methods will greatly enhance your ability to tackle binary tree problems effectively.
Solving Binary Tree Problems with Recursion
Why Recursion is Effective
Recursion is a powerful tool for solving binary tree problems. It allows us to break down complex problems into smaller, manageable parts. This method is particularly useful because:
- It simplifies the code, making it easier to read and maintain.
- It naturally fits the structure of binary trees, where each node can be treated as a smaller tree.
- Recursive solutions often require less code than their iterative counterparts.
Common Recursive Patterns
When working with binary trees, several common recursive patterns emerge:
- Depth-First Search (DFS): This involves exploring as far down a branch as possible before backtracking.
- Post-Order Traversal: This visits the left child, then the right child, and finally the node itself.
- Pre-Order Traversal: This visits the node first, then the left child, and finally the right child.
Examples of Recursive Solutions
Here are a few examples of how recursion can be applied to binary tree problems:
- Flattening a Binary Tree: This involves rearranging the tree into a linked list format. The recursive approach is efficient and avoids unnecessary storage.
- Inverting a Binary Tree: This flips the tree upside down, swapping left and right children recursively.
- Finding the Lowest Common Ancestor: This identifies the lowest node that is an ancestor to two given nodes, using recursion to traverse the tree.
Recursion is not just a technique; it’s a way of thinking about problems. By recognizing patterns in binary trees, we can develop elegant solutions that are both efficient and easy to understand.
In summary, mastering recursion is essential for tackling binary tree problems effectively. By understanding the recursive patterns and applying them, you can solve complex problems with ease. For instance, you can merge two binary trees by doing node sum using a recursive approach, which is often more intuitive than iterative methods.
Iterative Approaches to Binary Tree Problems
When to Use Iteration
Using iteration can be beneficial in several scenarios:
- Avoiding recursion depth limits: Iterative methods can handle larger trees without hitting stack overflow errors.
- Improved performance: In some cases, iterative solutions can be faster than their recursive counterparts.
- Memory efficiency: Iterative solutions often use less memory since they don’t require additional stack space.
Implementing Iterative Solutions
To implement an iterative solution for binary tree problems, follow these steps:
- Use a stack or queue: These data structures help manage the nodes as you traverse the tree.
- Process nodes: Visit each node and perform the required operation (like printing or modifying values).
- Push children onto the stack: Ensure that you add the left and right children of each node to the stack for further processing.
Comparing Iterative and Recursive Methods
Method | Time Complexity | Space Complexity | Pros | Cons |
---|---|---|---|---|
Recursive | O(n) | O(h) | Simple and clear | Can hit recursion limits |
Iterative | O(n) | O(h) | Avoids recursion depth issues | Slightly more complex |
Iterative solutions can be a powerful alternative to recursion, especially when dealing with large binary trees.
By understanding when and how to use iterative methods, you can effectively tackle various binary tree problems without the limitations of recursion.
Balancing Binary Trees
Definition of a Balanced Tree
A balanced binary tree is one where the height of the tree is O(log n), where n is the number of nodes. This means that the tree is structured in a way that keeps it efficient for operations like searching and inserting.
Techniques to Balance a Tree
To ensure a binary tree remains balanced, you can use several techniques:
- AVL Trees: These trees automatically adjust their height after insertions and deletions.
- Red-Black Trees: These maintain balance through color-coding nodes and ensuring certain properties are met.
- Splay Trees: These move frequently accessed elements closer to the root, helping maintain balance over time.
Checking if a Tree is Balanced
To determine if a binary tree is balanced, follow these steps:
- Check if the tree is empty. If it is, it is balanced.
- Calculate the height of the left and right subtrees.
- If the difference in height is more than 1, the tree is unbalanced.
- Recursively check the left and right subtrees.
A balanced binary tree is crucial for maintaining efficient operations. If a tree becomes unbalanced, it can lead to performance issues.
By understanding these concepts, you can effectively manage and maintain balanced binary trees in your applications.
Binary Search Trees (BST)
Properties of BSTs
A Binary Search Tree (BST) is a special type of tree structure where each node can have up to two children, known as the left and right child. The key features of a BST include:
- Left Subtree: Contains only nodes with values less than the parent node’s value.
- Right Subtree: Contains only nodes with values greater than the parent node’s value.
- Subtree Validity: Both left and right subtrees must also be BSTs.
These properties allow for efficient operations like searching, inserting, and deleting nodes.
Inserting and Deleting Nodes
When working with BSTs, inserting and deleting nodes follows specific rules:
- Inserting: Start at the root. If the new value is less, go left; if greater, go right. Repeat until you find an empty spot.
- Deleting: There are three cases:
- Node with no children: Simply remove it.
- Node with one child: Remove the node and connect its child to its parent.
- Node with two children: Find the in-order successor (smallest in the right subtree), replace the node’s value with it, and delete the successor.
Validating a BST
To check if a tree is a valid BST, you can use a simple method:
- Check each node: Ensure that each node’s value is within a valid range defined by its ancestors. The initial range for the root is (-∞, ∞).
Validating a BST is crucial for ensuring that the tree maintains its properties, which directly impacts the efficiency of operations performed on it.
Summary
In summary, understanding the properties and operations of Binary Search Trees is essential for efficiently managing data in various applications. Searching in a BST can be thought of as looking in a sorted array, making it a powerful tool in computer science.
Advanced Binary Tree Problems
Flattening a Binary Tree
Flattening a binary tree into a linked list is a common problem. The goal is to rearrange the tree so that all nodes follow a specific order. This process can be tricky but is essential for understanding tree structures. Here’s a simple approach:
- Perform a pre-order traversal of the tree.
- Store the values of each node in a list.
- Rebuild the tree using the stored values, linking nodes in the correct order.
Inverting a Binary Tree
Inverting a binary tree means swapping the left and right children of all nodes. This can be done using a recursive function:
- Start at the root node.
- Swap the left and right children.
- Recursively apply the same process to the left and right subtrees.
This method is efficient and straightforward, making it a favorite among programmers.
Finding the Lowest Common Ancestor
The lowest common ancestor (LCA) of two nodes in a binary tree is the deepest node that is an ancestor of both nodes. To find the LCA:
- Start from the root and traverse the tree.
- If you find either of the nodes, return that node.
- If both nodes are found in different subtrees, the current node is the LCA.
This problem is crucial in many applications, especially in databases and hierarchical data structures.
Understanding these advanced problems helps in mastering binary trees. They are not just academic exercises but have real-world applications in various fields.
Summary Table of Advanced Problems
Problem | Description | Key Technique |
---|---|---|
Flattening a Binary Tree | Rearranging tree nodes into a linked list | Pre-order Traversal |
Inverting a Binary Tree | Swapping left and right children | Recursion |
Finding the Lowest Common Ancestor | Identifying the deepest common ancestor of two nodes | Tree Traversal |
Optimizing Binary Tree Solutions
Improving Time Complexity
To enhance the performance of binary tree operations, consider the following strategies:
- Use efficient algorithms: Opt for algorithms with lower time complexity, such as O(n) for traversals.
- Avoid unnecessary traversals: Minimize the number of times you visit nodes by using techniques like memoization.
- Leverage properties of binary trees: Utilize the characteristics of specific types of trees, like Binary Search Trees (BSTs), to speed up searches and insertions.
Reducing Space Complexity
Reducing the amount of memory used can be crucial. Here are some tips:
- Use iterative methods: Instead of recursion, which can consume stack space, use loops where possible.
- In-place modifications: Modify the tree structure directly instead of creating new nodes or arrays.
- Avoid auxiliary data structures: Try to solve problems without using extra space for storing values.
Using Memoization
Memoization can significantly speed up recursive solutions. Here’s how:
- Store results of expensive function calls: Keep track of previously computed results to avoid redundant calculations.
- Use a hash map: This can help in storing results based on unique tree structures or node values.
- Apply it to overlapping subproblems: Identify parts of the problem that repeat and cache their results.
Optimizing binary tree solutions is essential for improving performance and efficiency. By focusing on time and space complexity, you can create solutions that are not only effective but also scalable.
Conclusion
By applying these optimization techniques, you can enhance the performance of your binary tree solutions, making them faster and more efficient. Remember, the goal is to balance performance with resource usage, ensuring that your algorithms run smoothly even with large datasets.
Highlight
- Balanced binary trees: Ensuring equilibrium in data structures is crucial for efficiency in operations like insertion and deletion.
Common Mistakes in Solving Binary Tree Problems
Ignoring Edge Cases
When working with binary trees, many people forget to consider edge cases. Here are some common ones to keep in mind:
- Empty trees: Always check if the tree is empty before performing operations.
- Single-node trees: Ensure your solution handles trees with only one node correctly.
- Unbalanced trees: Be aware that not all trees are balanced, which can affect your algorithms.
Misunderstanding Tree Properties
Understanding the properties of trees is crucial. Here are some key points:
- Depth vs. Height: Depth is the distance from the root to a node, while height is the distance from a node to its deepest leaf.
- Leaf Nodes: Leaf nodes are nodes without children. They play a significant role in many algorithms.
- Subtrees: Each node has a left and right subtree, which can be treated as separate trees.
Overcomplicating Solutions
Sometimes, solutions can become overly complex. Here are tips to simplify:
- Break down the problem: Divide the problem into smaller parts and solve each one.
- Use recursion wisely: Recursion can simplify many tree problems, but ensure you understand the base case.
- Avoid unnecessary data structures: Only use additional structures when absolutely necessary.
Remember: Understanding tree properties is essential for solving problems effectively. Simplifying your approach can lead to better solutions and less confusion.
By avoiding these common mistakes, you can improve your skills in solving binary tree problems and enhance your overall programming abilities.
Practical Applications of Binary Trees
Binary trees are not just theoretical concepts; they have real-world applications that make them essential in various fields. Here are some key areas where binary trees are used:
Binary Trees in Databases
- Efficient Searching: Binary trees, especially binary search trees (BST), allow for quick data retrieval, making them ideal for database indexing.
- Data Organization: They help in organizing data in a structured way, which improves performance during data operations.
- Dynamic Data Handling: Binary trees can easily adapt to changes, such as adding or removing data, without significant performance loss.
Binary Trees in File Systems
- Hierarchical Structure: File systems often use binary trees to represent directories and files, allowing for efficient navigation and management.
- Quick Access: They enable fast access to files and directories, improving user experience.
- Space Efficiency: Binary trees help in optimizing space by organizing files in a way that minimizes wasted storage.
Binary Trees in Network Routing
- Path Optimization: Binary trees can be used to determine the most efficient paths for data packets in a network.
- Load Balancing: They help in distributing network traffic evenly, preventing overload on any single route.
- Dynamic Routing: Binary trees allow for quick adjustments in routing paths as network conditions change.
In summary, binary trees play a crucial role in enhancing efficiency and organization in various applications. Their ability to manage and retrieve data quickly makes them invaluable in technology today.
Binary trees are not just a concept in computer science; they have real-world uses that can make a difference in how we manage data. From organizing information in databases to optimizing search algorithms, binary trees help in making processes faster and more efficient. If you’re eager to learn more about how to apply these concepts in coding, visit our website and start your journey today!
Conclusion
In summary, mastering binary tree problems requires understanding key techniques and strategies. By practicing recursive and iterative methods, you can tackle a variety of challenges effectively. Remember, recognizing patterns in tree structures is crucial. Whether you’re flattening a tree or checking if it’s balanced, these skills will help you excel in coding interviews and real-world applications. Keep exploring and practicing, and you’ll become proficient in solving binary tree problems!
Frequently Asked Questions
What is a binary tree?
A binary tree is a type of data structure that has nodes. Each node can have up to two children, which are called the left and right child.
What are the different types of binary trees?
There are several types of binary trees, including full binary trees, complete binary trees, and balanced binary trees, each with its own rules about how nodes are arranged.
How do you traverse a binary tree?
You can traverse a binary tree in several ways: in-order, pre-order, post-order, and level-order. Each method visits the nodes in a different order.
Why is recursion often used with binary trees?
Recursion is helpful with binary trees because it allows you to easily visit each node and its children without needing to keep track of everything manually.
What does it mean for a binary tree to be balanced?
A balanced binary tree is one where the left and right subtrees of every node differ in height by no more than one, ensuring efficient operations.
What is a binary search tree (BST)?
A binary search tree is a special kind of binary tree where each node’s left child is less than the node, and the right child is greater.
How can I check if a binary tree is balanced?
To check if a binary tree is balanced, you can calculate the height of the left and right subtrees for each node and see if their heights differ by more than one.
What are common mistakes when working with binary trees?
Common mistakes include ignoring edge cases, misunderstanding how trees work, and making solutions more complex than necessary.