Mastering Math and Number Theory Questions: Essential Skills for Coding Interviews
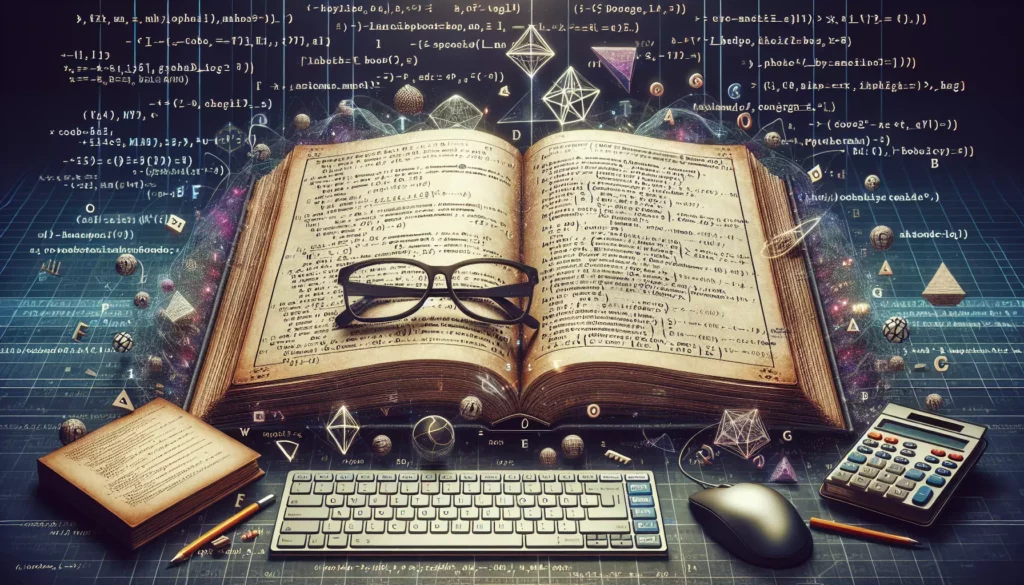
In the world of coding interviews, particularly for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering mathematical concepts and number theory is crucial. These topics form the foundation of many algorithmic problems and can be the key to unlocking efficient solutions. In this comprehensive guide, we’ll explore various mathematical and number theory concepts that frequently appear in coding interviews, along with strategies to tackle them effectively.
1. Prime Numbers and Primality Testing
Prime numbers are fundamental to number theory and often feature in coding interview questions. Understanding how to work with primes efficiently is essential.
Sieve of Eratosthenes
The Sieve of Eratosthenes is an efficient algorithm for finding all prime numbers up to a given limit. Here’s a Python implementation:
def sieve_of_eratosthenes(n):
primes = [True] * (n + 1)
primes[0] = primes[1] = False
for i in range(2, int(n**0.5) + 1):
if primes[i]:
for j in range(i*i, n+1, i):
primes[j] = False
return [i for i in range(2, n+1) if primes[i]]
# Example usage
print(sieve_of_eratosthenes(30))
# Output: [2, 3, 5, 7, 11, 13, 17, 19, 23, 29]
Primality Testing
For checking if a single number is prime, you can use a more efficient method:
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
# Example usage
print(is_prime(17)) # Output: True
print(is_prime(24)) # Output: False
2. Greatest Common Divisor (GCD) and Least Common Multiple (LCM)
GCD and LCM are frequently used in various mathematical problems. Understanding these concepts and their efficient implementation is crucial.
Euclidean Algorithm for GCD
def gcd(a, b):
while b:
a, b = b, a % b
return a
# Example usage
print(gcd(48, 18)) # Output: 6
LCM using GCD
def lcm(a, b):
return abs(a * b) // gcd(a, b)
# Example usage
print(lcm(12, 18)) # Output: 36
3. Modular Arithmetic
Modular arithmetic is essential for handling large numbers and is often used in cryptography-related problems.
Basic Operations
def mod_add(a, b, m):
return (a + b) % m
def mod_subtract(a, b, m):
return (a - b + m) % m
def mod_multiply(a, b, m):
return (a * b) % m
# Example usage
print(mod_add(15, 17, 7)) # Output: 4
print(mod_subtract(15, 17, 7)) # Output: 5
print(mod_multiply(15, 17, 7)) # Output: 3
Modular Exponentiation
For efficiently calculating large powers modulo a number:
def mod_pow(base, exponent, modulus):
result = 1
base = base % modulus
while exponent > 0:
if exponent % 2 == 1:
result = (result * base) % modulus
exponent = exponent // 2
base = (base * base) % modulus
return result
# Example usage
print(mod_pow(2, 20, 1000)) # Output: 376
4. Combinatorics
Combinatorics problems often appear in coding interviews, especially when dealing with permutations and combinations.
Calculating nCr (Combinations)
def nCr(n, r):
if r > n:
return 0
r = min(r, n - r)
numerator = 1
denominator = 1
for i in range(r):
numerator *= (n - i)
denominator *= (i + 1)
return numerator // denominator
# Example usage
print(nCr(5, 2)) # Output: 10
Generating Permutations
def generate_permutations(arr):
if len(arr) == 0:
return [[]]
result = []
for i in range(len(arr)):
rest = arr[:i] + arr[i+1:]
for p in generate_permutations(rest):
result.append([arr[i]] + p)
return result
# Example usage
print(generate_permutations([1, 2, 3]))
# Output: [[1, 2, 3], [1, 3, 2], [2, 1, 3], [2, 3, 1], [3, 1, 2], [3, 2, 1]]
5. Fibonacci Sequence and Dynamic Programming
The Fibonacci sequence is a classic example used to introduce dynamic programming concepts.
Recursive Fibonacci (Inefficient)
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
# Example usage
print(fibonacci_recursive(10)) # Output: 55
Dynamic Programming Fibonacci (Efficient)
def fibonacci_dp(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
# Example usage
print(fibonacci_dp(100)) # Output: 354224848179261915075
6. Matrix Operations
Matrix operations are fundamental in many areas of computer science and often appear in coding interviews.
Matrix Multiplication
def matrix_multiply(A, B):
if len(A[0]) != len(B):
raise ValueError("Incompatible matrix dimensions")
result = [[0 for _ in range(len(B[0]))] for _ in range(len(A))]
for i in range(len(A)):
for j in range(len(B[0])):
for k in range(len(B)):
result[i][j] += A[i][k] * B[k][j]
return result
# Example usage
A = [[1, 2], [3, 4]]
B = [[5, 6], [7, 8]]
print(matrix_multiply(A, B))
# Output: [[19, 22], [43, 50]]
7. Probability and Expected Value
Understanding probability and expected value can be crucial for certain types of interview questions, especially those related to randomized algorithms or game theory.
Calculating Probability
def probability_of_sum(dice_count, target_sum):
total_outcomes = 6 ** dice_count
favorable_outcomes = count_favorable_outcomes(dice_count, target_sum)
return favorable_outcomes / total_outcomes
def count_favorable_outcomes(dice_count, target_sum, current_sum=0):
if dice_count == 0:
return 1 if current_sum == target_sum else 0
count = 0
for i in range(1, 7):
count += count_favorable_outcomes(dice_count - 1, target_sum, current_sum + i)
return count
# Example: Probability of getting a sum of 7 with 2 dice
print(probability_of_sum(2, 7)) # Output: 0.16666666666666666
8. Bit Manipulation
Bit manipulation is a powerful technique that can lead to highly efficient solutions for certain problems.
Common Bit Operations
def count_set_bits(n):
count = 0
while n:
count += n & 1
n >>= 1
return count
def is_power_of_two(n):
return n > 0 and (n & (n - 1)) == 0
def find_single_number(arr):
result = 0
for num in arr:
result ^= num
return result
# Example usage
print(count_set_bits(13)) # Output: 3 (13 is 1101 in binary)
print(is_power_of_two(16)) # Output: True
print(find_single_number([4, 1, 2, 1, 2])) # Output: 4
9. Number Theory Concepts
Advanced number theory concepts can be useful for solving complex mathematical problems efficiently.
Euler’s Totient Function
def euler_totient(n):
result = n
p = 2
while p * p <= n:
if n % p == 0:
while n % p == 0:
n //= p
result *= (1 - 1/p)
p += 1
if n > 1:
result *= (1 - 1/n)
return int(result)
# Example usage
print(euler_totient(10)) # Output: 4
Modular Multiplicative Inverse
def mod_inverse(a, m):
def extended_gcd(a, b):
if a == 0:
return b, 0, 1
else:
gcd, x, y = extended_gcd(b % a, a)
return gcd, y - (b // a) * x, x
gcd, x, _ = extended_gcd(a, m)
if gcd != 1:
raise Exception("Modular inverse does not exist")
else:
return x % m
# Example usage
print(mod_inverse(3, 11)) # Output: 4 (because (3 * 4) % 11 = 1)
10. Geometry and Spatial Problems
Geometric problems can appear in various forms in coding interviews, often requiring a mix of mathematical understanding and algorithmic thinking.
Calculating Distance Between Two Points
import math
def distance(x1, y1, x2, y2):
return math.sqrt((x2 - x1)**2 + (y2 - y1)**2)
# Example usage
print(distance(0, 0, 3, 4)) # Output: 5.0
Checking if Points Form a Straight Line
def are_collinear(x1, y1, x2, y2, x3, y3):
return (y2 - y1) * (x3 - x2) == (y3 - y2) * (x2 - x1)
# Example usage
print(are_collinear(1, 1, 2, 2, 3, 3)) # Output: True
Conclusion
Mastering these mathematical and number theory concepts is crucial for excelling in coding interviews, especially for top tech companies. These problems not only test your coding skills but also your ability to think abstractly and apply mathematical principles to solve complex problems efficiently.
Remember, the key to success in these areas is not just memorizing formulas or algorithms, but understanding the underlying principles and being able to apply them creatively to new problems. Practice regularly with a variety of problems, and don’t hesitate to dive deep into the mathematical foundations of these concepts.
As you prepare for your coding interviews, make sure to integrate these mathematical problem-solving skills with your algorithmic knowledge. Platforms like AlgoCademy offer a wealth of resources and practice problems that can help you hone these skills and prepare effectively for technical interviews at major tech companies.
Keep in mind that while these topics are important, they are just one aspect of coding interviews. Make sure to balance your preparation with other crucial areas such as data structures, algorithms, system design, and practical coding skills. With dedicated practice and a solid understanding of these mathematical concepts, you’ll be well-equipped to tackle even the most challenging interview questions and advance your career in the tech industry.