The Importance of Writing Readable Code Under Pressure
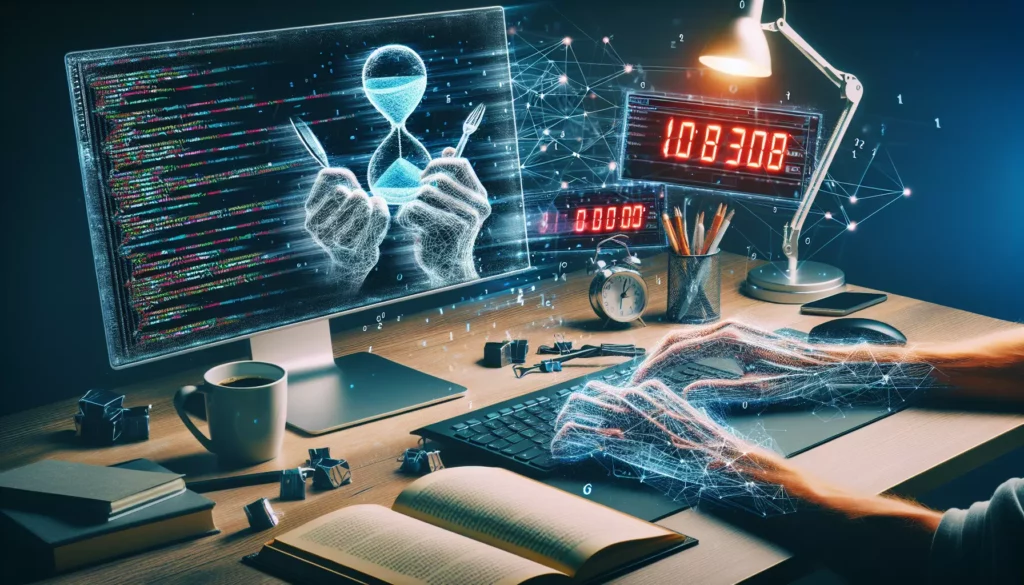
In the fast-paced world of software development, writing clean and readable code is a crucial skill that often gets overlooked, especially when working under pressure. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, the ability to produce clear and maintainable code is paramount. In this comprehensive guide, we’ll explore why writing readable code matters, even when time is of the essence, and how you can improve this essential skill.
Why Readable Code Matters
Before diving into the techniques for writing readable code under pressure, it’s important to understand why this skill is so valuable:
- Maintainability: Code that is easy to read is easier to maintain and update in the future, both for you and your colleagues.
- Debugging: When issues arise, readable code makes it much simpler to identify and fix problems quickly.
- Collaboration: In team environments, clear code facilitates better collaboration and knowledge sharing among developers.
- Code Reviews: Readable code speeds up the code review process, leading to faster iterations and improved overall quality.
- Interview Performance: During technical interviews, especially at FAANG companies, the clarity of your code can be just as important as its functionality.
Strategies for Writing Readable Code Under Pressure
Now that we understand the importance of readable code, let’s explore some strategies to help you write clean code even when you’re under time constraints:
1. Use Meaningful Variable and Function Names
One of the simplest yet most effective ways to improve code readability is to use descriptive names for variables and functions. Even when you’re in a rush, take a moment to choose names that clearly convey the purpose or content of what you’re working with.
Instead of:
function calc(a, b) {
return a + b;
}
let x = 5;
let y = 10;
let z = calc(x, y);
Write:
function calculateSum(firstNumber, secondNumber) {
return firstNumber + secondNumber;
}
let baseValue = 5;
let increment = 10;
let totalSum = calculateSum(baseValue, increment);
2. Keep Functions Small and Focused
Even when time is tight, try to keep your functions small and focused on a single task. This not only makes your code more readable but also more modular and easier to test.
// Instead of one large function
function processUserData(userData) {
// Validate user input
if (!userData.name || !userData.email) {
throw new Error("Invalid user data");
}
// Format user data
let formattedName = userData.name.trim().toLowerCase();
let formattedEmail = userData.email.trim().toLowerCase();
// Save user to database
let user = {
name: formattedName,
email: formattedEmail
};
saveToDatabase(user);
// Send welcome email
sendWelcomeEmail(formattedEmail);
}
// Break it down into smaller, focused functions
function validateUserData(userData) {
if (!userData.name || !userData.email) {
throw new Error("Invalid user data");
}
}
function formatUserData(userData) {
return {
name: userData.name.trim().toLowerCase(),
email: userData.email.trim().toLowerCase()
};
}
function processUserData(userData) {
validateUserData(userData);
let formattedData = formatUserData(userData);
saveToDatabase(formattedData);
sendWelcomeEmail(formattedData.email);
}
3. Use Comments Wisely
While it might be tempting to skip comments when you’re in a hurry, strategic use of comments can greatly enhance code readability. Focus on explaining the “why” rather than the “what” in your comments.
// Bad comment (explains the obvious)
// Increment counter by 1
counter++;
// Good comment (explains the reason)
// Increment the retry counter to track failed attempts
retryCounter++;
4. Consistent Formatting and Indentation
Maintaining consistent formatting and indentation is crucial for readability, even when you’re working quickly. Most modern IDEs offer auto-formatting features, so take advantage of these tools to keep your code clean and consistent.
// Inconsistent formatting
function calculateArea(length,width){
if(length<0||width<0){
return 0;
}
return length*width;
}
// Consistent formatting
function calculateArea(length, width) {
if (length < 0 || width < 0) {
return 0;
}
return length * width;
}
5. Use Built-in Methods and Libraries
Leverage built-in methods and libraries whenever possible. They not only save time but also make your code more readable and less prone to errors.
// Instead of writing your own implementation
function isPalindrome(str) {
let left = 0;
let right = str.length - 1;
while (left < right) {
if (str[left] !== str[right]) {
return false;
}
left++;
right--;
}
return true;
}
// Use built-in methods for cleaner code
function isPalindrome(str) {
return str === str.split('').reverse().join('');
}
6. Avoid Deep Nesting
Deep nesting can make code hard to read and understand. Try to limit nesting and use early returns or guard clauses to simplify your logic.
// Deeply nested code
function processOrder(order) {
if (order) {
if (order.items && order.items.length > 0) {
if (order.paymentMethod) {
if (order.paymentMethod === 'credit') {
// Process credit payment
} else if (order.paymentMethod === 'debit') {
// Process debit payment
} else {
// Handle unknown payment method
}
} else {
// Handle missing payment method
}
} else {
// Handle empty order
}
} else {
// Handle null order
}
}
// Flattened code with early returns
function processOrder(order) {
if (!order) {
return handleNullOrder();
}
if (!order.items || order.items.length === 0) {
return handleEmptyOrder();
}
if (!order.paymentMethod) {
return handleMissingPaymentMethod();
}
switch (order.paymentMethod) {
case 'credit':
return processCreditPayment(order);
case 'debit':
return processDebitPayment(order);
default:
return handleUnknownPaymentMethod(order);
}
}
Practicing Readable Code in Coding Interviews
When preparing for coding interviews, especially for top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), it’s crucial to practice writing clean and readable code under time pressure. Here are some tips specifically for interview scenarios:
1. Start with a Clear Plan
Before you start coding, take a minute to outline your approach. This not only helps you organize your thoughts but also demonstrates to the interviewer that you think before you code.
// Example: Solving Two Sum problem
// 1. Outline approach:
// - Use a hash map to store complements
// - Iterate through the array once
// - Check if complement exists, if so return indices
// - If not, add current number and index to hash map
// 2. Implement solution
// 3. Test with example cases
2. Communicate While Coding
As you write your code, explain your thought process. This helps the interviewer understand your approach and can earn you points even if you make minor mistakes.
function twoSum(nums, target) {
// Explain: "I'm using a Map to store complements for O(1) lookup"
const complementMap = new Map();
for (let i = 0; i < nums.length; i++) {
// Explain: "For each number, I'll check if its complement exists"
const complement = target - nums[i];
if (complementMap.has(complement)) {
// Explain: "If it does, we've found our pair"
return [complementMap.get(complement), i];
}
// Explain: "If not, add this number and its index to the map"
complementMap.set(nums[i], i);
}
// Explain: "If we've gone through the entire array without finding a pair, return null"
return null;
}
3. Use Helper Functions for Complex Logic
If a part of your solution involves complex logic, consider breaking it out into a helper function. This keeps your main function clean and shows that you can write modular code.
function isValidSudoku(board) {
for (let i = 0; i < 9; i++) {
if (!isValidUnit(board[i])) return false; // Check row
if (!isValidUnit(board.map(row => row[i]))) return false; // Check column
}
for (let i = 0; i < 9; i += 3) {
for (let j = 0; j < 9; j += 3) {
if (!isValidUnit(getBox(board, i, j))) return false; // Check 3x3 box
}
}
return true;
}
function isValidUnit(unit) {
const seen = new Set();
for (let num of unit) {
if (num !== '.' && seen.has(num)) return false;
seen.add(num);
}
return true;
}
function getBox(board, startRow, startCol) {
const box = [];
for (let i = 0; i < 3; i++) {
for (let j = 0; j < 3; j++) {
box.push(board[startRow + i][startCol + j]);
}
}
return box;
}
4. Use Descriptive Variable Names, Even in Short Functions
In interview settings, it’s tempting to use short variable names to save time. However, using descriptive names can actually save time in explaining your code and shows that you prioritize readability.
// Instead of:
function f(s) {
let m = new Map();
for (let c of s) {
m.set(c, (m.get(c) || 0) + 1);
}
return [...m.entries()].sort((a, b) => b[1] - a[1])[0][0];
}
// Write:
function findMostFrequentChar(str) {
let charFrequency = new Map();
for (let char of str) {
charFrequency.set(char, (charFrequency.get(char) || 0) + 1);
}
return [...charFrequency.entries()].sort((a, b) => b[1] - a[1])[0][0];
}
5. Handle Edge Cases Gracefully
Showing that you consider edge cases demonstrates attention to detail and robustness in your code. Handle these cases at the beginning of your function for clarity.
function findKthLargest(nums, k) {
// Handle edge cases
if (!nums || nums.length === 0) return null;
if (k <= 0 || k > nums.length) return null;
// Main logic
return quickSelect(nums, 0, nums.length - 1, nums.length - k);
}
function quickSelect(nums, left, right, kSmallest) {
// Implementation details...
}
Tools and Techniques for Improving Code Readability
To consistently write readable code, even under pressure, it’s helpful to leverage tools and techniques that support this practice:
1. IDE Features and Extensions
Most modern Integrated Development Environments (IDEs) offer features that can help improve code readability:
- Auto-formatting: Use your IDE’s built-in formatter or install extensions like Prettier for consistent code formatting.
- Linting: Tools like ESLint can help identify potential issues and enforce coding standards.
- Code snippets: Create and use code snippets for common patterns to save time and ensure consistency.
2. Code Review Practices
Regular code reviews, even for personal projects, can help you develop a habit of writing readable code:
- Conduct self-reviews before submitting code.
- Participate in peer code reviews to learn from others and share knowledge.
- Use code review checklists that include readability criteria.
3. Refactoring Techniques
Familiarize yourself with common refactoring techniques that can improve code readability:
- Extract Method: Break down large methods into smaller, more focused ones.
- Rename Variable/Method: Improve naming for better clarity.
- Replace Conditional with Polymorphism: Use object-oriented principles to simplify complex conditional logic.
4. Documentation Tools
Use documentation tools and practices to enhance code understandability:
- JSDoc for JavaScript: Use JSDoc comments to document functions and classes.
- README files: Maintain clear and concise README files for projects.
- Inline documentation: Write clear comments for complex logic or algorithms.
5. Version Control Best Practices
Leverage version control systems like Git to support code readability:
- Write clear and descriptive commit messages.
- Use feature branches for organized development.
- Regularly review your own changes before committing.
The Long-Term Benefits of Writing Readable Code
Developing the habit of writing readable code, even under pressure, offers numerous long-term benefits:
1. Improved Collaboration
Readable code facilitates better teamwork and knowledge sharing. When your code is easy to understand, it’s easier for your colleagues to work with you, review your code, and build upon your work.
2. Faster Onboarding
For new team members or when you’re onboarding to a new project, readable code significantly reduces the learning curve. This leads to faster integration and productivity.
3. Reduced Technical Debt
By consistently writing clean, readable code, you reduce the accumulation of technical debt. This means less time spent on refactoring and bug fixing in the future, allowing more time for new feature development.
4. Enhanced Problem-Solving Skills
The process of writing readable code often involves breaking down complex problems into simpler, more manageable parts. This practice enhances your overall problem-solving skills, benefiting you in various aspects of software development.
5. Career Advancement
Developers known for writing clean, readable code are often highly valued in the industry. This skill can set you apart in job interviews, performance reviews, and when seeking leadership roles in technical teams.
Conclusion
Writing readable code under pressure is a valuable skill that can significantly impact your effectiveness as a developer and your career prospects. By implementing the strategies and techniques discussed in this guide, you can improve your ability to produce clean, maintainable code even in high-pressure situations like coding interviews or tight project deadlines.
Remember, the goal is not just to solve the problem at hand, but to create solutions that can be easily understood, maintained, and built upon in the future. As you continue to practice and refine your skills, writing readable code will become second nature, allowing you to tackle complex problems with confidence and clarity.
Whether you’re just starting your coding journey or preparing for technical interviews at top tech companies, prioritizing code readability will serve you well throughout your career in software development. Keep practicing, stay mindful of these principles, and watch as your code—and your skills—continue to improve.