When and How to Use Heaps in Coding Problems
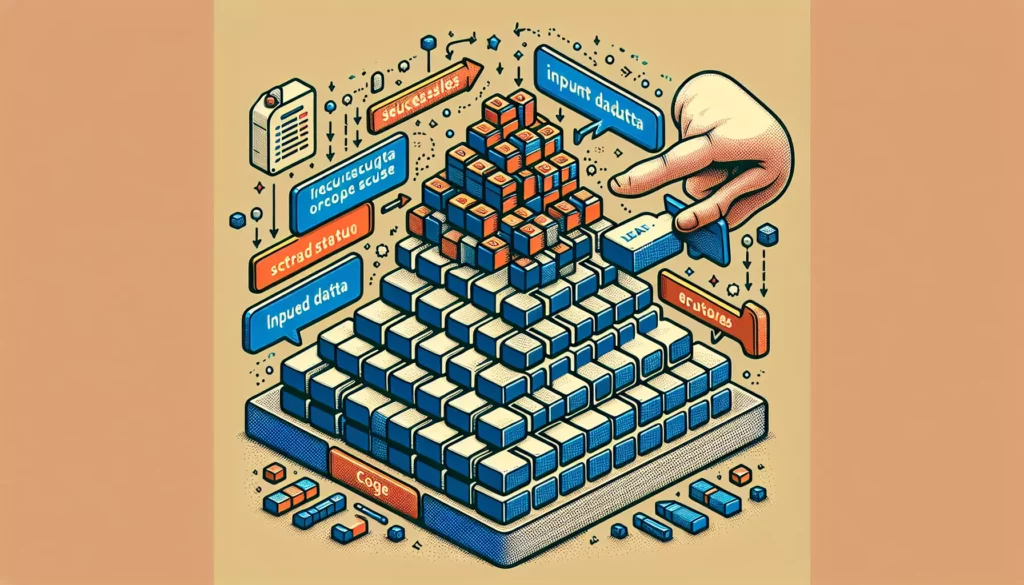
In the world of data structures and algorithms, heaps play a crucial role in solving various coding problems efficiently. As you progress from beginner-level coding to preparing for technical interviews at major tech companies, understanding when and how to use heaps can significantly boost your problem-solving skills. In this comprehensive guide, we’ll explore the concept of heaps, their types, implementation, and most importantly, when and how to leverage them in coding problems.
What is a Heap?
Before diving into the applications of heaps, let’s first understand what a heap is. A heap is a specialized tree-based data structure that satisfies the heap property. There are two types of heaps:
- Max Heap: In a max heap, for any given node I, the value of I is greater than or equal to the values of its children.
- Min Heap: In a min heap, the value of a node I is less than or equal to the values of its children.
Heaps are commonly implemented as binary trees, where each node has at most two children. The most important property of a heap is that it allows constant-time retrieval of the maximum element (in a max heap) or the minimum element (in a min heap), and logarithmic time insertion and deletion of elements.
Implementing a Heap
While heaps are conceptually tree-based structures, they are typically implemented using arrays for efficiency. Here’s a basic implementation of a min heap in Python:
class MinHeap:
def __init__(self):
self.heap = []
def parent(self, i):
return (i - 1) // 2
def left_child(self, i):
return 2 * i + 1
def right_child(self, i):
return 2 * i + 2
def swap(self, i, j):
self.heap[i], self.heap[j] = self.heap[j], self.heap[i]
def insert(self, key):
self.heap.append(key)
self._heapify_up(len(self.heap) - 1)
def _heapify_up(self, i):
parent = self.parent(i)
if i > 0 and self.heap[i] < self.heap[parent]:
self.swap(i, parent)
self._heapify_up(parent)
def extract_min(self):
if len(self.heap) == 0:
return None
if len(self.heap) == 1:
return self.heap.pop()
min_val = self.heap[0]
self.heap[0] = self.heap.pop()
self._heapify_down(0)
return min_val
def _heapify_down(self, i):
min_index = i
left = self.left_child(i)
right = self.right_child(i)
if left < len(self.heap) and self.heap[left] < self.heap[min_index]:
min_index = left
if right < len(self.heap) and self.heap[right] < self.heap[min_index]:
min_index = right
if min_index != i:
self.swap(i, min_index)
self._heapify_down(min_index)
This implementation provides the basic operations of a min heap: insertion and extraction of the minimum element. The time complexity for both these operations is O(log n), where n is the number of elements in the heap.
When to Use Heaps in Coding Problems
Now that we understand what heaps are and how to implement them, let’s explore when to use heaps in coding problems. Heaps are particularly useful in the following scenarios:
1. Finding the k-th Smallest/Largest Element
When you need to find the k-th smallest or largest element in an array, heaps can provide an efficient solution. For finding the k-th smallest element, you would use a max heap, and for the k-th largest element, you would use a min heap.
Example problem: Find the k-th largest element in an unsorted array.
import heapq
def findKthLargest(nums, k):
heap = []
for num in nums:
heapq.heappush(heap, num)
if len(heap) > k:
heapq.heappop(heap)
return heap[0]
# Example usage
nums = [3, 2, 1, 5, 6, 4]
k = 2
print(findKthLargest(nums, k)) # Output: 5
In this solution, we maintain a min heap of size k. By doing so, the k-th largest element will always be at the top of the heap.
2. Implementing Priority Queues
Heaps are the perfect data structure for implementing priority queues. In a priority queue, elements with higher priority are served before elements with lower priority. This is naturally achieved by the heap property.
Example problem: Implement a task scheduler where tasks with higher priority are executed first.
import heapq
class Task:
def __init__(self, priority, description):
self.priority = priority
self.description = description
def __lt__(self, other):
return self.priority > other.priority
class PriorityQueue:
def __init__(self):
self.queue = []
def add_task(self, task):
heapq.heappush(self.queue, task)
def execute_highest_priority_task(self):
if not self.queue:
return None
return heapq.heappop(self.queue)
# Example usage
scheduler = PriorityQueue()
scheduler.add_task(Task(3, "Low priority task"))
scheduler.add_task(Task(1, "High priority task"))
scheduler.add_task(Task(2, "Medium priority task"))
executed_task = scheduler.execute_highest_priority_task()
print(f"Executed: {executed_task.description}") # Output: Executed: High priority task
In this implementation, we use Python’s heapq module to create a priority queue. The Task class is defined with a custom comparison method to ensure that tasks with higher priority (lower numeric value) are at the top of the heap.
3. Merging k Sorted Lists
When you need to merge k sorted lists into a single sorted list, using a heap can provide an efficient solution. This problem is common in scenarios involving multiple data streams or in distributed systems.
Example problem: Merge k sorted linked lists into one sorted list.
import heapq
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def mergeKLists(lists):
heap = []
dummy = ListNode(0)
current = dummy
# Add the first node of each list to the heap
for i, lst in enumerate(lists):
if lst:
heapq.heappush(heap, (lst.val, i, lst))
while heap:
val, i, node = heapq.heappop(heap)
current.next = ListNode(val)
current = current.next
if node.next:
heapq.heappush(heap, (node.next.val, i, node.next))
return dummy.next
# Example usage
# Assume we have three sorted linked lists: 1->4->5, 1->3->4, 2->6
# We'll create these lists manually for demonstration
list1 = ListNode(1, ListNode(4, ListNode(5)))
list2 = ListNode(1, ListNode(3, ListNode(4)))
list3 = ListNode(2, ListNode(6))
merged = mergeKLists([list1, list2, list3])
# Print the merged list
while merged:
print(merged.val, end=" ")
merged = merged.next
# Output: 1 1 2 3 4 4 5 6
In this solution, we use a min heap to keep track of the smallest element among the heads of all lists. We continuously pop the smallest element from the heap and add it to the result list, then push the next element from the same list onto the heap if it exists.
4. Implementing Heap Sort
Heaps can be used to implement an efficient sorting algorithm called Heap Sort. While not as commonly used as QuickSort or MergeSort in practice, HeapSort has a guaranteed O(n log n) time complexity and is an in-place sorting algorithm.
Here’s an implementation of Heap Sort:
def heapify(arr, n, i):
largest = i
left = 2 * i + 1
right = 2 * i + 2
if left < n and arr[left] > arr[largest]:
largest = left
if right < n and arr[right] > arr[largest]:
largest = right
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
def heap_sort(arr):
n = len(arr)
# Build a max heap
for i in range(n // 2 - 1, -1, -1):
heapify(arr, n, i)
# Extract elements from the heap one by one
for i in range(n - 1, 0, -1):
arr[0], arr[i] = arr[i], arr[0]
heapify(arr, i, 0)
# Example usage
arr = [12, 11, 13, 5, 6, 7]
heap_sort(arr)
print("Sorted array:", arr)
# Output: Sorted array: [5, 6, 7, 11, 12, 13]
Heap Sort works by first building a max heap from the input array, then repeatedly extracting the maximum element from the heap and placing it at the end of the array.
5. Finding Median in a Stream of Numbers
Heaps can be effectively used to find the median in a stream of numbers. This problem is particularly interesting because it requires maintaining the median as new numbers are added to the stream.
Here’s an implementation using two heaps:
import heapq
class MedianFinder:
def __init__(self):
self.small = [] # max heap
self.large = [] # min heap
def addNum(self, num: int) -> None:
if len(self.small) == len(self.large):
heapq.heappush(self.large, -heapq.heappushpop(self.small, -num))
else:
heapq.heappush(self.small, -heapq.heappushpop(self.large, num))
def findMedian(self) -> float:
if len(self.small) == len(self.large):
return (-self.small[0] + self.large[0]) / 2.0
else:
return float(self.large[0])
# Example usage
mf = MedianFinder()
mf.addNum(1)
mf.addNum(2)
print(mf.findMedian()) # Output: 1.5
mf.addNum(3)
print(mf.findMedian()) # Output: 2.0
In this solution, we maintain two heaps: a max heap for the smaller half of the numbers and a min heap for the larger half. The median is either the average of the tops of both heaps (if they have equal size) or the top of the larger heap.
Best Practices When Using Heaps
When using heaps in your coding problems, keep these best practices in mind:
- Choose the right type of heap: Use a min heap when you need quick access to the smallest element, and a max heap when you need quick access to the largest element.
- Consider space complexity: While heaps provide efficient operations, they do require O(n) space. In memory-constrained environments, consider if an alternative approach might be more suitable.
- Use built-in implementations when available: Many programming languages provide built-in heap implementations (like Python’s heapq module) which are often more optimized than custom implementations.
- Be mindful of the heap property: Always ensure that the heap property is maintained after insertions and deletions. The heapify operation is crucial for this.
- Use heaps for top-k problems: Heaps are particularly efficient for problems involving finding the top k elements from a larger set of elements.
Common Pitfalls to Avoid
When working with heaps, be aware of these common pitfalls:
- Forgetting to heapify: After making changes to the heap, always remember to call the heapify function to maintain the heap property.
- Using the wrong type of heap: Using a min heap when you need a max heap (or vice versa) can lead to incorrect results.
- Inefficient implementation: Implementing heap operations inefficiently can negate the performance benefits of using a heap. Ensure your implementation maintains O(log n) time complexity for key operations.
- Not considering alternatives: While heaps are powerful, they’re not always the best solution. Consider other data structures like balanced binary search trees or arrays for simpler problems.
- Ignoring edge cases: Don’t forget to handle edge cases, such as empty heaps or heaps with a single element.
Conclusion
Heaps are a powerful data structure that can significantly improve the efficiency of many algorithms, especially those involving priority queues, sorting, and selection problems. By understanding when and how to use heaps, you can tackle a wide range of coding problems more effectively.
As you prepare for technical interviews and advance your coding skills, practice implementing heaps from scratch and solving problems that utilize heaps. This will not only deepen your understanding of the data structure but also sharpen your problem-solving skills.
Remember, the key to mastering heaps, like any other data structure or algorithm, is practice. Try to identify scenarios in your coding projects where heaps might be beneficial, and don’t hesitate to use them when appropriate. With time and experience, using heaps will become second nature, and you’ll find yourself reaching for this powerful tool whenever you encounter suitable problems.
Happy coding, and may the heap be with you!