27 Strategies for Memory Management and Avoiding Leaks
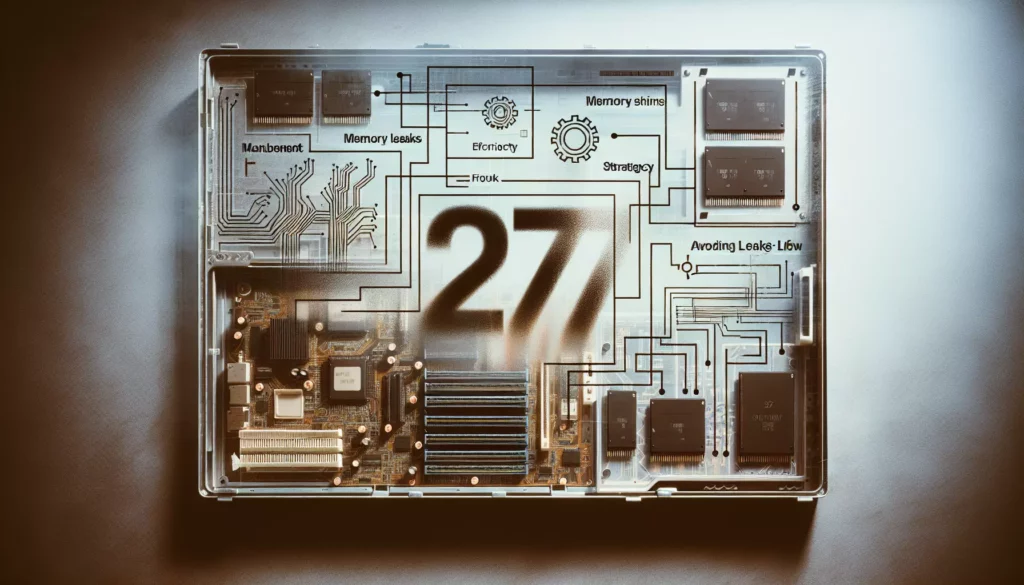
In the world of software development, efficient memory management is crucial for creating high-performance, stable, and reliable applications. As programmers, we must be vigilant about how our code allocates, uses, and releases memory to prevent issues like memory leaks, fragmentation, and excessive resource consumption. This comprehensive guide will explore 27 essential strategies for effective memory management and avoiding memory leaks, providing you with the knowledge and tools to write more efficient and robust code.
1. Understand the Basics of Memory Allocation
Before diving into specific strategies, it’s essential to have a solid understanding of how memory allocation works in your programming language of choice. Different languages handle memory management differently:
- Manual memory management (e.g., C, C++)
- Automatic garbage collection (e.g., Java, Python, JavaScript)
- Hybrid approaches (e.g., Rust with ownership system)
Knowing the underlying mechanisms will help you make informed decisions about memory usage in your code.
2. Use Smart Pointers (C++)
In C++, smart pointers provide automatic memory management for dynamically allocated objects. They help prevent memory leaks by automatically deallocating memory when it’s no longer needed. The three main types of smart pointers in C++ are:
- std::unique_ptr: For exclusive ownership
- std::shared_ptr: For shared ownership
- std::weak_ptr: For temporary ownership without affecting the object’s lifetime
Here’s an example of using std::unique_ptr:
#include <memory>
void example() {
std::unique_ptr<int> ptr = std::make_unique<int>(42);
// ptr will be automatically deleted when it goes out of scope
}
3. Implement RAII (Resource Acquisition Is Initialization)
RAII is a programming technique that ties the lifecycle of a resource (like memory) to the lifetime of an object. This ensures that resources are properly released when they’re no longer needed. In C++, this is often achieved through constructors and destructors:
class ResourceManager {
private:
Resource* resource;
public:
ResourceManager() : resource(new Resource()) {}
~ResourceManager() { delete resource; }
};
void example() {
ResourceManager manager;
// Resource is automatically released when manager goes out of scope
}
4. Use Object Pools for Frequent Allocations
Object pools can significantly improve performance and reduce memory fragmentation for applications that frequently allocate and deallocate objects of the same type. Instead of creating and destroying objects repeatedly, you can reuse objects from a pre-allocated pool:
class ObjectPool {
private:
std::vector<Object*> pool;
public:
Object* getObject() {
if (pool.empty()) {
return new Object();
}
Object* obj = pool.back();
pool.pop_back();
return obj;
}
void returnObject(Object* obj) {
pool.push_back(obj);
}
};
5. Implement Custom Memory Allocators
For performance-critical applications, implementing custom memory allocators can provide fine-grained control over memory usage. This approach allows you to optimize allocation strategies for specific use cases:
class CustomAllocator {
private:
char* memory;
size_t size;
size_t used;
public:
CustomAllocator(size_t size) : size(size), used(0) {
memory = new char[size];
}
void* allocate(size_t bytes) {
if (used + bytes > size) {
return nullptr; // Out of memory
}
void* result = &memory[used];
used += bytes;
return result;
}
void deallocate(void* ptr) {
// Implement deallocation logic
}
~CustomAllocator() {
delete[] memory;
}
};
6. Use Weak References in Garbage-Collected Languages
In languages with garbage collection, weak references can help prevent memory leaks caused by circular references. They allow you to reference an object without preventing it from being collected by the garbage collector:
import java.lang.ref.WeakReference;
class Example {
private WeakReference<LargeObject> weakRef;
public void setObject(LargeObject obj) {
weakRef = new WeakReference<>(obj);
}
public LargeObject getObject() {
return weakRef.get();
}
}
7. Implement Dispose Patterns
For languages that don’t have deterministic destruction (like C#), implementing a dispose pattern can ensure that resources are properly released when they’re no longer needed:
public class ResourceManager : IDisposable {
private bool disposed = false;
private Resource resource;
public void Dispose() {
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing) {
if (!disposed) {
if (disposing) {
resource.Dispose();
}
disposed = true;
}
}
~ResourceManager() {
Dispose(false);
}
}
8. Use Memory Profiling Tools
Memory profiling tools can help identify memory leaks, excessive allocations, and other memory-related issues in your application. Some popular tools include:
- Valgrind (for C and C++)
- Java VisualVM (for Java)
- Memory Profiler in Visual Studio (for .NET)
- Chrome DevTools Memory tab (for JavaScript)
Regularly profiling your application can help catch memory issues early in the development process.
9. Implement Reference Counting
Reference counting is a memory management technique where objects keep track of how many references point to them. When the count reaches zero, the object can be safely deallocated. While many languages implement this automatically, you might need to implement it manually in some cases:
class RefCounted {
private:
int refCount;
public:
RefCounted() : refCount(0) {}
void addRef() { ++refCount; }
void release() {
if (--refCount == 0) {
delete this;
}
}
virtual ~RefCounted() {}
};
10. Use Static Code Analysis Tools
Static code analysis tools can help identify potential memory leaks and other issues before runtime. Some popular tools include:
- Clang Static Analyzer (for C, C++, and Objective-C)
- SonarQube (for multiple languages)
- Coverity (for multiple languages)
Integrating these tools into your development process can catch many memory-related issues early.
11. Implement Copy-on-Write Semantics
Copy-on-write is an optimization technique that delays copying of data until it’s modified. This can significantly reduce memory usage in scenarios where data is often read but rarely modified:
template <typename T>
class CopyOnWrite {
private:
std::shared_ptr<T> data;
public:
CopyOnWrite(T value) : data(std::make_shared<T>(std::move(value))) {}
T& get() {
if (!data.unique()) {
data = std::make_shared<T>(*data);
}
return *data;
}
const T& get() const {
return *data;
}
};
12. Use Memory-Mapped Files for Large Datasets
When working with large datasets, memory-mapped files can provide efficient access without loading the entire file into memory:
#include <fcntl.h>
#include <sys/mman.h>
#include <unistd.h>
int main() {
int fd = open("large_file.dat", O_RDONLY);
off_t size = lseek(fd, 0, SEEK_END);
void* mapped = mmap(NULL, size, PROT_READ, MAP_PRIVATE, fd, 0);
// Use mapped memory...
munmap(mapped, size);
close(fd);
return 0;
}
13. Implement Lazy Initialization
Lazy initialization delays the creation of an object until it’s first needed. This can help reduce memory usage, especially for objects that are expensive to create or may not be used in every program execution:
class LazyInitialized {
private:
std::unique_ptr<ExpensiveObject> object;
public:
ExpensiveObject& getObject() {
if (!object) {
object = std::make_unique<ExpensiveObject>();
}
return *object;
}
};
14. Use Flyweight Pattern for Shared State
The Flyweight pattern is useful when you need to create a large number of similar objects. It separates the intrinsic state (shared) from the extrinsic state (unique), reducing memory usage:
class Flyweight {
private:
std::string sharedState;
public:
Flyweight(const std::string& shared) : sharedState(shared) {}
void operation(const std::string& unique) {
std::cout << "Flyweight: Shared (" << sharedState
<< ") and unique (" << unique << ") state.\n";
}
};
15. Implement Memory Pools
Memory pools can improve performance and reduce fragmentation by allocating a large block of memory upfront and managing it internally:
class MemoryPool {
private:
char* memory;
size_t size;
size_t used;
public:
MemoryPool(size_t size) : size(size), used(0) {
memory = new char[size];
}
void* allocate(size_t bytes) {
if (used + bytes > size) return nullptr;
void* result = &memory[used];
used += bytes;
return result;
}
void reset() { used = 0; }
~MemoryPool() { delete[] memory; }
};
16. Use Stack Allocation When Possible
Stack allocation is generally faster and more efficient than heap allocation. When dealing with small, short-lived objects, prefer stack allocation:
void example() {
// Stack allocation
int stackArray[100];
// Instead of:
// int* heapArray = new int[100];
// ...
// delete[] heapArray;
}
17. Implement Object Caching
Object caching can reduce memory allocation and deallocation overhead for frequently used objects:
template <typename T>
class ObjectCache {
private:
std::vector<std::unique_ptr<T>> cache;
public:
T* get() {
if (cache.empty()) {
return new T();
}
T* obj = cache.back().release();
cache.pop_back();
return obj;
}
void release(T* obj) {
cache.push_back(std::unique_ptr<T>(obj));
}
};
18. Use Memory Barriers in Multi-threaded Applications
Memory barriers ensure proper synchronization between threads, preventing issues related to memory visibility and ordering:
#include <atomic>
std::atomic<int> sharedData(0);
void producer() {
sharedData.store(42, std::memory_order_release);
}
void consumer() {
int value = sharedData.load(std::memory_order_acquire);
// Use value...
}
19. Implement Custom Delete Functions
Custom delete functions can help ensure proper cleanup of resources, especially when dealing with polymorphic types:
class Base {
public:
virtual ~Base() = default;
};
class Derived : public Base {
public:
void* operator new(size_t size) {
return ::operator new(size);
}
void operator delete(void* ptr, size_t size) {
// Custom cleanup logic
::operator delete(ptr);
}
};
20. Use Placement New for Custom Memory Management
Placement new allows you to construct objects at a specific memory address, which can be useful for custom memory management schemes:
char buffer[sizeof(MyClass)];
MyClass* obj = new (buffer) MyClass();
// Use obj...
obj->~MyClass(); // Call destructor manually
21. Implement Circular Buffers for Streaming Data
Circular buffers can efficiently manage streaming data without frequent allocations and deallocations:
template <typename T, size_t Size>
class CircularBuffer {
private:
std::array<T, Size> buffer;
size_t head = 0;
size_t tail = 0;
bool full = false;
public:
void push(const T& item) {
buffer[head] = item;
if (full) {
tail = (tail + 1) % Size;
}
head = (head + 1) % Size;
full = head == tail;
}
T pop() {
if (empty()) {
throw std::runtime_error("Buffer is empty");
}
T item = buffer[tail];
full = false;
tail = (tail + 1) % Size;
return item;
}
bool empty() const {
return (!full && (head == tail));
}
};
22. Use Memory-Efficient Data Structures
Choose data structures that are appropriate for your use case and memory constraints. For example, use std::vector instead of std::list when you need contiguous memory and don’t require frequent insertions/deletions in the middle of the container.
23. Implement Custom Allocators for STL Containers
Custom allocators allow you to control how STL containers allocate and deallocate memory:
template <typename T>
class CustomAllocator {
public:
using value_type = T;
CustomAllocator() = default;
template <typename U>
CustomAllocator(const CustomAllocator<U>&) {}
T* allocate(std::size_t n) {
return static_cast<T*>(::operator new(n * sizeof(T)));
}
void deallocate(T* p, std::size_t n) {
::operator delete(p);
}
};
std::vector<int, CustomAllocator<int>> vec;
24. Use Move Semantics to Avoid Unnecessary Copies
Move semantics in C++11 and later can significantly reduce unnecessary copying of objects, improving performance and reducing memory usage:
class MyClass {
private:
std::vector<int> data;
public:
MyClass(MyClass&& other) noexcept
: data(std::move(other.data)) {}
MyClass& operator=(MyClass&& other) noexcept {
if (this != &other) {
data = std::move(other.data);
}
return *this;
}
};
25. Implement Memory Compaction
For long-running applications, implementing periodic memory compaction can help reduce fragmentation:
class MemoryManager {
private:
std::vector<char> memory;
std::vector<std::pair<size_t, size_t>> allocations;
public:
void* allocate(size_t size) {
// Allocation logic...
}
void deallocate(void* ptr) {
// Deallocation logic...
}
void compact() {
std::sort(allocations.begin(), allocations.end());
std::vector<char> newMemory;
for (const auto& alloc : allocations) {
size_t offset = alloc.first;
size_t size = alloc.second;
newMemory.insert(newMemory.end(),
memory.begin() + offset,
memory.begin() + offset + size);
}
memory = std::move(newMemory);
// Update allocation offsets...
}
};
26. Use Memory-Mapped I/O for Efficient File Handling
Memory-mapped I/O can provide efficient access to file contents, especially for large files:
#include <fcntl.h>
#include <sys/mman.h>
#include <unistd.h>
int main() {
int fd = open("file.txt", O_RDONLY);
struct stat sb;
fstat(fd, &sb);
char* mapped = (char*)mmap(NULL, sb.st_size, PROT_READ, MAP_PRIVATE, fd, 0);
// Use mapped memory...
munmap(mapped, sb.st_size);
close(fd);
return 0;
}
27. Implement Generational Garbage Collection
For languages that allow custom garbage collection, implementing a generational collector can improve performance by focusing on short-lived objects:
class GenerationalGC {
private:
std::vector<Object*> youngGeneration;
std::vector<Object*> oldGeneration;
public:
void collectYoungGeneration() {
// Mark and sweep young generation
// Promote surviving objects to old generation
}
void collectOldGeneration() {
// Less frequent full collection of old generation
}
void* allocate(size_t size) {
// Allocation logic with generations
}
};
Conclusion
Effective memory management is a critical skill for any programmer, regardless of the language or platform they work with. By implementing these 27 strategies, you can significantly improve the performance, stability, and efficiency of your applications. Remember that different strategies may be more or less applicable depending on your specific use case, language, and performance requirements.
As you continue to develop your skills in memory management, it’s important to stay updated with the latest best practices and tools in your chosen programming languages. Regular profiling, testing, and optimization of your code will help you identify and address memory-related issues before they become problematic in production environments.
By mastering these memory management techniques, you’ll be well-equipped to write high-performance, memory-efficient code that can handle complex tasks and large-scale applications. Keep practicing and experimenting with these strategies to find the best approaches for your specific projects and development needs.