24 Techniques for Handling String Manipulation Problems
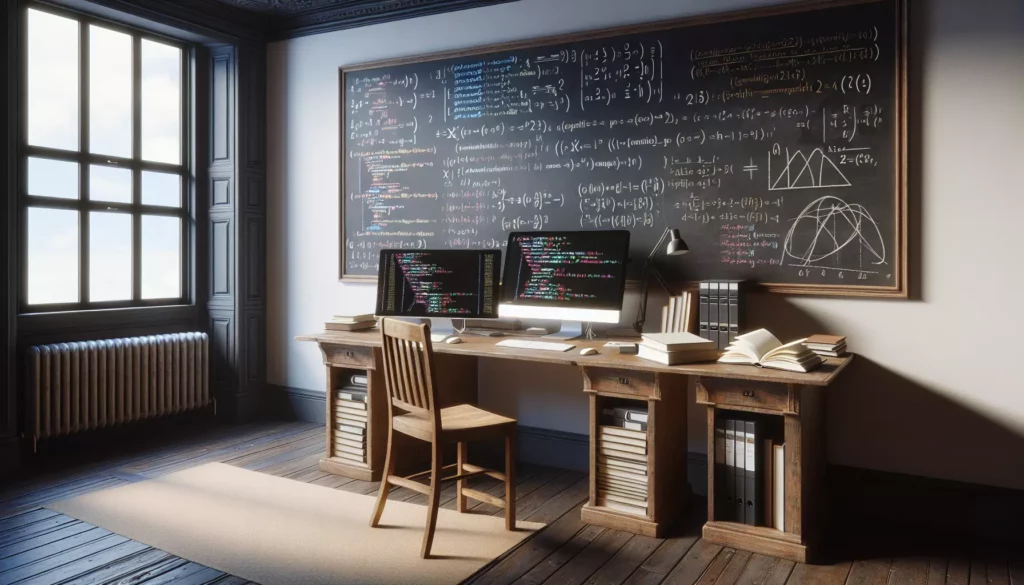
String manipulation is a fundamental skill in programming that every developer should master. Whether you’re preparing for technical interviews at major tech companies or working on real-world projects, the ability to efficiently manipulate strings is crucial. In this comprehensive guide, we’ll explore 24 essential techniques for handling string manipulation problems, providing you with the tools you need to tackle a wide range of coding challenges.
1. Two-Pointer Technique
The two-pointer technique is a powerful method for solving string manipulation problems, especially those involving palindromes, reversals, or comparisons.
Example: Reverse a String
def reverse_string(s):
left, right = 0, len(s) - 1
s = list(s)
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return "".join(s)
print(reverse_string("hello")) # Output: "olleh"
2. Sliding Window
The sliding window technique is useful for problems involving substrings or subarrays, allowing you to efficiently process contiguous sequences of characters.
Example: Find the Longest Substring Without Repeating Characters
def longest_substring_without_repeats(s):
char_set = set()
max_length = 0
left = right = 0
while right < len(s):
if s[right] not in char_set:
char_set.add(s[right])
max_length = max(max_length, right - left + 1)
right += 1
else:
char_set.remove(s[left])
left += 1
return max_length
print(longest_substring_without_repeats("abcabcbb")) # Output: 3
3. String Concatenation
String concatenation is a basic operation, but it’s important to understand the performance implications of different methods.
Example: Efficient String Concatenation
def efficient_concatenation(strings):
return "".join(strings)
print(efficient_concatenation(["Hello", " ", "World"])) # Output: "Hello World"
4. String Splitting
Splitting strings is a common operation when parsing input or processing text data.
Example: Split a String into Words
def split_into_words(s):
return s.split()
print(split_into_words("Hello World! How are you?"))
# Output: ["Hello", "World!", "How", "are", "you?"]
5. Regular Expressions
Regular expressions are powerful tools for pattern matching and text processing in strings.
Example: Extract Email Addresses from Text
import re
def extract_emails(text):
pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
return re.findall(pattern, text)
text = "Contact us at info@example.com or support@example.org"
print(extract_emails(text))
# Output: ["info@example.com", "support@example.org"]
6. String Formatting
Proper string formatting is essential for creating readable and maintainable code.
Example: Using f-strings in Python
def greet(name, age):
return f"Hello, {name}! You are {age} years old."
print(greet("Alice", 30)) # Output: "Hello, Alice! You are 30 years old."
7. Character Counting
Counting occurrences of characters is a common task in string manipulation problems.
Example: Count Character Frequency
from collections import Counter
def count_chars(s):
return Counter(s)
print(count_chars("hello")) # Output: Counter({"l": 2, "h": 1, "e": 1, "o": 1})
8. String Comparison
Comparing strings is fundamental to many string manipulation problems, including anagram detection and sorting.
Example: Check if Two Strings are Anagrams
def are_anagrams(s1, s2):
return sorted(s1) == sorted(s2)
print(are_anagrams("listen", "silent")) # Output: True
9. String Transformation
Transforming strings from one format to another is a common requirement in data processing and text manipulation tasks.
Example: Convert String to Title Case
def to_title_case(s):
return s.title()
print(to_title_case("hello world")) # Output: "Hello World"
10. Substring Search
Efficiently searching for substrings within a larger string is crucial for many text processing applications.
Example: Implement KMP Algorithm for Pattern Matching
def kmp_search(text, pattern):
def compute_lps(pattern):
lps = [0] * len(pattern)
length = 0
i = 1
while i < len(pattern):
if pattern[i] == pattern[length]:
length += 1
lps[i] = length
i += 1
elif length != 0:
length = lps[length - 1]
else:
lps[i] = 0
i += 1
return lps
lps = compute_lps(pattern)
i = j = 0
results = []
while i < len(text):
if text[i] == pattern[j]:
i += 1
j += 1
if j == len(pattern):
results.append(i - j)
j = lps[j - 1]
elif j != 0:
j = lps[j - 1]
else:
i += 1
return results
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
print(kmp_search(text, pattern)) # Output: [10]
11. String Encoding and Decoding
Encoding and decoding strings is essential for data compression, encryption, and serialization tasks.
Example: Run-Length Encoding
def run_length_encode(s):
if not s:
return ""
result = []
count = 1
for i in range(1, len(s)):
if s[i] == s[i-1]:
count += 1
else:
result.append(f"{count}{s[i-1]}")
count = 1
result.append(f"{count}{s[-1]}")
return "".join(result)
print(run_length_encode("AABBBCCCC")) # Output: "2A3B4C"
12. String Parsing
Parsing strings to extract structured information is a common task in data processing and text analysis.
Example: Parse CSV String
import csv
from io import StringIO
def parse_csv(csv_string):
csv_file = StringIO(csv_string)
csv_reader = csv.reader(csv_file)
return list(csv_reader)
csv_data = "Name,Age,City\nAlice,30,New York\nBob,25,San Francisco"
print(parse_csv(csv_data))
# Output: [["Name", "Age", "City"], ["Alice", "30", "New York"], ["Bob", "25", "San Francisco"]]
13. String Validation
Validating strings against specific criteria is crucial for ensuring data integrity and security in applications.
Example: Validate Email Address
import re
def is_valid_email(email):
pattern = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
return re.match(pattern, email) is not None
print(is_valid_email("user@example.com")) # Output: True
print(is_valid_email("invalid.email")) # Output: False
14. String Compression
Compressing strings can be useful for reducing storage space or transmission size.
Example: Basic String Compression
def compress_string(s):
if not s:
return ""
compressed = []
count = 1
for i in range(1, len(s)):
if s[i] == s[i-1]:
count += 1
else:
compressed.append(s[i-1] + str(count))
count = 1
compressed.append(s[-1] + str(count))
compressed_str = "".join(compressed)
return compressed_str if len(compressed_str) < len(s) else s
print(compress_string("aabcccccaaa")) # Output: "a2b1c5a3"
15. String Rotation
Rotating strings is a common operation in cryptography and certain algorithmic problems.
Example: Check if One String is a Rotation of Another
def is_rotation(s1, s2):
if len(s1) != len(s2):
return False
return s2 in s1 + s1
print(is_rotation("waterbottle", "erbottlewat")) # Output: True
print(is_rotation("hello", "olleh")) # Output: False
16. String Interleaving
Interleaving strings is a technique used in various string manipulation problems and can be useful in certain coding challenges.
Example: Check if a String is Interleaving of Two Other Strings
def is_interleave(s1, s2, s3):
if len(s1) + len(s2) != len(s3):
return False
dp = [[False] * (len(s2) + 1) for _ in range(len(s1) + 1)]
dp[0][0] = True
for i in range(1, len(s1) + 1):
dp[i][0] = dp[i-1][0] and s1[i-1] == s3[i-1]
for j in range(1, len(s2) + 1):
dp[0][j] = dp[0][j-1] and s2[j-1] == s3[j-1]
for i in range(1, len(s1) + 1):
for j in range(1, len(s2) + 1):
dp[i][j] = (dp[i-1][j] and s1[i-1] == s3[i+j-1]) or \
(dp[i][j-1] and s2[j-1] == s3[i+j-1])
return dp[len(s1)][len(s2)]
print(is_interleave("aabcc", "dbbca", "aadbbcbcac")) # Output: True
print(is_interleave("aabcc", "dbbca", "aadbbbaccc")) # Output: False
17. String Matching with Wildcards
Matching strings with wildcards is a common problem in file system operations and text search applications.
Example: Implement Wildcard Matching
def wildcard_match(s, p):
s_len, p_len = len(s), len(p)
dp = [[False] * (p_len + 1) for _ in range(s_len + 1)]
dp[0][0] = True
for j in range(1, p_len + 1):
if p[j-1] == "*":
dp[0][j] = dp[0][j-1]
for i in range(1, s_len + 1):
for j in range(1, p_len + 1):
if p[j-1] == "*":
dp[i][j] = dp[i][j-1] or dp[i-1][j]
elif p[j-1] == "?" or s[i-1] == p[j-1]:
dp[i][j] = dp[i-1][j-1]
return dp[s_len][p_len]
print(wildcard_match("adceb", "*a*b")) # Output: True
print(wildcard_match("acdcb", "a*c?b")) # Output: False
18. String Permutations
Generating permutations of a string is a classic problem that tests understanding of recursion and combinatorics.
Example: Generate All Permutations of a String
def generate_permutations(s):
if len(s) <= 1:
return [s]
perms = []
for i, char in enumerate(s):
rest = s[:i] + s[i+1:]
for perm in generate_permutations(rest):
perms.append(char + perm)
return perms
print(generate_permutations("abc"))
# Output: ["abc", "acb", "bac", "bca", "cab", "cba"]
19. String Alignment
String alignment is crucial in bioinformatics for comparing DNA sequences and in natural language processing for text comparison.
Example: Implement Longest Common Subsequence
def longest_common_subsequence(s1, s2):
m, n = len(s1), len(s2)
dp = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if s1[i-1] == s2[j-1]:
dp[i][j] = dp[i-1][j-1] + 1
else:
dp[i][j] = max(dp[i-1][j], dp[i][j-1])
return dp[m][n]
print(longest_common_subsequence("ABCDGH", "AEDFHR")) # Output: 3
20. String Editing
String editing problems, such as calculating edit distance, are important in spell checking and DNA sequence analysis.
Example: Calculate Levenshtein Distance
def levenshtein_distance(s1, s2):
m, n = len(s1), len(s2)
dp = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(m + 1):
dp[i][0] = i
for j in range(n + 1):
dp[0][j] = j
for i in range(1, m + 1):
for j in range(1, n + 1):
if s1[i-1] == s2[j-1]:
dp[i][j] = dp[i-1][j-1]
else:
dp[i][j] = min(dp[i-1][j], dp[i][j-1], dp[i-1][j-1]) + 1
return dp[m][n]
print(levenshtein_distance("kitten", "sitting")) # Output: 3
21. String Sorting
Sorting strings efficiently is important for many applications, including dictionary creation and search functionality.
Example: Custom String Sort
def custom_sort(arr, order):
order_map = {c: i for i, c in enumerate(order)}
return sorted(arr, key=lambda x: [order_map.get(c, len(order)) for c in x])
arr = ["cba", "abcd", "cbad", "efg"]
order = "abc"
print(custom_sort(arr, order))
# Output: ["abcd", "cba", "cbad", "efg"]
22. String Hashing
String hashing is a technique used to quickly compare strings and is the basis for many string matching algorithms.
Example: Implement Rabin-Karp Algorithm
def rabin_karp(text, pattern):
n, m = len(text), len(pattern)
p = 31
mod = 10**9 + 9
p_pow = [1]
for i in range(1, max(n, m)):
p_pow.append((p_pow[-1] * p) % mod)
def compute_hash(s):
h = 0
for i, c in enumerate(s):
h = (h + (ord(c) - ord("a" + 1)) * p_pow[i]) % mod
return h
pattern_hash = compute_hash(pattern)
text_hash = [0] * (n + 1)
for i in range(n):
text_hash[i+1] = (text_hash[i] + (ord(text[i]) - ord("a" + 1)) * p_pow[i]) % mod
result = []
for i in range(n - m + 1):
curr_hash = (text_hash[i+m] - text_hash[i] + mod) % mod
if curr_hash == pattern_hash * p_pow[i] % mod:
if text[i:i+m] == pattern:
result.append(i)
return result
text = "abababab"
pattern = "ab"
print(rabin_karp(text, pattern)) # Output: [0, 2, 4, 6]
23. String Trie
A trie is an efficient data structure for storing and searching strings, particularly useful for prefix matching and autocomplete features.
Example: Implement a Trie
class TrieNode:
def __init__(self):
self.children = {}
self.is_end = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
trie = Trie()
trie.insert("apple")
print(trie.search("apple")) # Output: True
print(trie.search("app")) # Output: False
print(trie.starts_with("app")) # Output: True
24. String Compression with Dictionary Encoding
Dictionary encoding is a powerful technique for compressing strings, especially when dealing with repetitive text or large datasets.
Example: Implement LZW Compression
def lzw_compress(s):
dictionary = {chr(i): i for i in range(256)}
result = []
current_string = ""
code = 256
for char in s:
current_string += char
if current_string not in dictionary:
result.append(dictionary[current_string[:-1]])
dictionary[current_string] = code
code += 1
current_string = char
if current_string:
result.append(dictionary[current_string])
return result
def lzw_decompress(compressed):
dictionary = {i: chr(i) for i in range(256)}
next_code = 256
result = ""
current_code = compressed.pop(0)
result = dictionary[current_code]
for code in compressed:
if code in dictionary:
entry = dictionary[code]
elif code == next_code:
entry = result + result[0]
else:
raise ValueError("Invalid compressed code")
result += entry
dictionary[next_code] = result + entry[0]
next_code += 1
return result
original = "TOBEORNOTTOBEORTOBEORNOT"
compressed = lzw_compress(original)
decompressed = lzw_decompress(compressed)
print("Original:", original)
print("Compressed:", compressed)
print("Decompressed:", decompressed)
print("Compression ratio:", len(compressed) / len(original))
# Output:
# Original: TOBEORNOTTOBEORTOBEORNOT
# Compressed: [84, 79, 66, 69, 79, 82, 78, 79, 84, 256, 258, 260, 265, 259, 261, 263]
# Decompressed: TOBEORNOTTOBEORTOBEORNOT
# Compression ratio: 0.6153846153846154
Conclusion
Mastering these 24 techniques for handling string manipulation problems will significantly enhance your coding skills and prepare you for a wide range of programming challenges. From basic operations like concatenation and splitting to advanced algorithms like Rabin-Karp and LZW compression, these techniques cover the spectrum of string manipulation tasks you’re likely to encounter in technical interviews and real-world projects.
Remember that practice is key to internalizing these concepts. Try implementing these techniques in your preferred programming language and apply them to various coding problems. As you become more comfortable with these string manipulation techniques, you’ll find yourself better equipped to tackle complex algorithmic challenges and develop efficient solutions in your software development journey.