Mastering Tree Traversal Techniques for Interviews
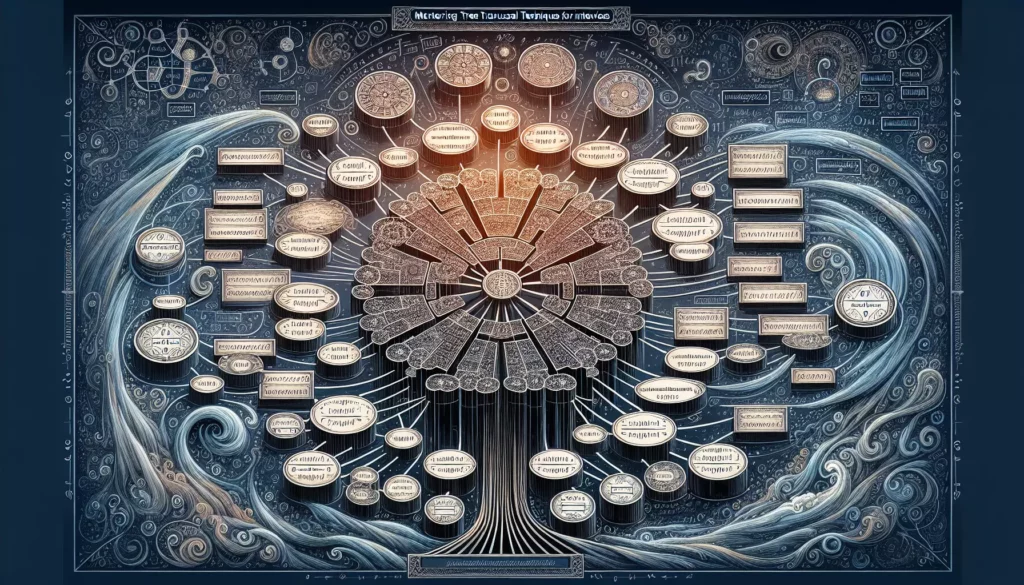
Tree traversal is a fundamental concept in computer science and a common topic in technical interviews, especially for positions at major tech companies. Understanding various tree traversal techniques is crucial for any programmer looking to excel in algorithm-based interviews. In this comprehensive guide, we’ll explore different tree traversal methods, their implementations, and practical applications to help you master this essential skill.
Table of Contents
- Introduction to Tree Traversal
- Types of Tree Traversal
- Depth-First Traversal Techniques
- Breadth-First Traversal
- Recursive vs. Iterative Implementations
- Practical Applications of Tree Traversal
- Interview Tips and Common Questions
- Advanced Tree Traversal Techniques
- Conclusion
1. Introduction to Tree Traversal
Tree traversal refers to the process of visiting each node in a tree data structure exactly once. It’s a fundamental operation in working with trees and is essential for various algorithms and problem-solving techniques. Before diving into specific traversal methods, let’s review the basic structure of a tree:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
This simple Python class represents a binary tree node, which contains a value and references to its left and right child nodes. Understanding this structure is crucial as we explore different traversal techniques.
2. Types of Tree Traversal
Tree traversal techniques can be broadly categorized into two main types:
- Depth-First Traversal (DFS): In this approach, we explore as far as possible along each branch before backtracking. DFS can be further divided into three subtypes based on the order of visiting the root node:
- Preorder (Root, Left, Right)
- Inorder (Left, Root, Right)
- Postorder (Left, Right, Root)
- Breadth-First Traversal (BFS): Also known as level-order traversal, this method explores all nodes at the present depth before moving to the nodes at the next depth level.
Each of these traversal methods has its own use cases and advantages, which we’ll explore in detail.
3. Depth-First Traversal Techniques
3.1 Preorder Traversal
In preorder traversal, we visit the root node first, then the left subtree, and finally the right subtree. This is often used when you need to explore the roots before inspecting any leaves.
Here’s a recursive implementation of preorder traversal:
def preorder_traversal(root):
if root:
print(root.val) # Visit the root
preorder_traversal(root.left) # Traverse left subtree
preorder_traversal(root.right) # Traverse right subtree
And here’s an iterative version using a stack:
def preorder_traversal_iterative(root):
if not root:
return
stack = [root]
while stack:
node = stack.pop()
print(node.val)
if node.right:
stack.append(node.right)
if node.left:
stack.append(node.left)
3.2 Inorder Traversal
Inorder traversal visits the left subtree, then the root node, and finally the right subtree. For binary search trees, this traversal gives nodes in non-decreasing order.
Recursive implementation of inorder traversal:
def inorder_traversal(root):
if root:
inorder_traversal(root.left) # Traverse left subtree
print(root.val) # Visit the root
inorder_traversal(root.right) # Traverse right subtree
Iterative implementation using a stack:
def inorder_traversal_iterative(root):
stack = []
current = root
while current or stack:
while current:
stack.append(current)
current = current.left
current = stack.pop()
print(current.val)
current = current.right
3.3 Postorder Traversal
In postorder traversal, we visit the left subtree, then the right subtree, and finally the root node. This is useful in scenarios where you need to delete the tree or perform operations on child nodes before the parent.
Recursive implementation of postorder traversal:
def postorder_traversal(root):
if root:
postorder_traversal(root.left) # Traverse left subtree
postorder_traversal(root.right) # Traverse right subtree
print(root.val) # Visit the root
Iterative implementation using two stacks:
def postorder_traversal_iterative(root):
if not root:
return
stack1 = [root]
stack2 = []
while stack1:
node = stack1.pop()
stack2.append(node)
if node.left:
stack1.append(node.left)
if node.right:
stack1.append(node.right)
while stack2:
print(stack2.pop().val)
4. Breadth-First Traversal
Breadth-First Traversal, also known as level-order traversal, visits all the nodes of a level before going to the next level. This is particularly useful when you need to find the shortest path between two nodes or when you want to traverse the tree level by level.
Here’s an implementation of BFS using a queue:
from collections import deque
def breadth_first_traversal(root):
if not root:
return
queue = deque([root])
while queue:
node = queue.popleft()
print(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
BFS is often used in scenarios where you need to find the shortest path or the minimum number of steps to reach a target node.
5. Recursive vs. Iterative Implementations
As we’ve seen, tree traversal can be implemented both recursively and iteratively. Each approach has its advantages and trade-offs:
Recursive Approach:
- Pros:
- More intuitive and easier to understand
- Code is usually more concise
- Follows the natural recursive structure of trees
- Cons:
- Can lead to stack overflow for very deep trees
- May be less efficient due to function call overhead
Iterative Approach:
- Pros:
- More efficient in terms of space complexity
- Avoids potential stack overflow issues
- Often faster in practice due to reduced function call overhead
- Cons:
- Can be more complex to implement and understand
- May require explicit stack or queue management
In interview situations, it’s valuable to be familiar with both approaches. Start with the method you’re most comfortable with, and if time allows, discuss or implement the alternative approach to demonstrate your versatility.
6. Practical Applications of Tree Traversal
Tree traversal techniques have numerous practical applications in computer science and software development. Here are some common use cases:
- Expression Evaluation: Inorder traversal of expression trees can generate infix notation, while postorder traversal can be used to evaluate the expression.
- File System Navigation: Traversing directory structures in file systems often uses tree traversal algorithms.
- HTML/XML Parsing: The Document Object Model (DOM) is often represented as a tree, and traversal is used for parsing and manipulation.
- Compiler Design: Abstract Syntax Trees (ASTs) are traversed during various phases of compilation.
- Game AI: Decision trees in game AI often use traversal techniques to determine the best move.
- Database Indexing: B-trees and other tree-based data structures used in database indexing rely on efficient traversal methods.
Understanding these applications can help you relate tree traversal to real-world scenarios during interviews.
7. Interview Tips and Common Questions
When preparing for interviews that may involve tree traversal, keep these tips in mind:
- Practice implementing all traversal methods both recursively and iteratively.
- Be prepared to explain the time and space complexity of your solutions.
- Understand when to use each traversal method based on the problem requirements.
- Be familiar with common tree-related problems that involve traversal, such as:
- Finding the maximum depth of a binary tree
- Checking if a binary tree is balanced
- Serializing and deserializing a binary tree
- Finding the lowest common ancestor of two nodes
Here are some common interview questions related to tree traversal:
- “Implement a function to check if two binary trees are identical.”
- “Given a binary tree, find its maximum depth.”
- “Convert a binary search tree to a sorted doubly linked list in-place.”
- “Implement a function to print all paths from root to leaf nodes in a binary tree.”
- “Given a binary tree, find the maximum path sum between two leaves.”
For each of these questions, consider which traversal method would be most appropriate and be prepared to explain your reasoning.
8. Advanced Tree Traversal Techniques
Once you’ve mastered the basic traversal techniques, you can explore more advanced concepts:
8.1 Morris Traversal
Morris Traversal is an in-order traversal technique that uses threading to achieve O(1) space complexity without using a stack or recursion. Here’s an implementation:
def morris_traversal(root):
current = root
while current:
if not current.left:
print(current.val)
current = current.right
else:
pre = current.left
while pre.right and pre.right != current:
pre = pre.right
if not pre.right:
pre.right = current
current = current.left
else:
pre.right = None
print(current.val)
current = current.right
8.2 Threaded Binary Trees
Threaded binary trees use the unused right pointers in leaf nodes to create “threads” that link to the in-order successor, allowing for efficient traversal without recursion or a stack.
8.3 N-ary Tree Traversal
While we’ve focused on binary trees, many real-world problems involve n-ary trees. Here’s an example of preorder traversal for an n-ary tree:
class Node:
def __init__(self, val=None, children=None):
self.val = val
self.children = children or []
def preorder_nary(root):
if not root:
return
print(root.val)
for child in root.children:
preorder_nary(child)
8.4 Vertical Order Traversal
Vertical order traversal involves visiting nodes top to bottom, column by column. This can be useful in scenarios where you need to process tree nodes based on their horizontal distance from the root.
from collections import defaultdict, deque
def vertical_order_traversal(root):
if not root:
return []
column_table = defaultdict(list)
queue = deque([(root, 0)])
min_column = max_column = 0
while queue:
node, column = queue.popleft()
column_table[column].append(node.val)
min_column = min(min_column, column)
max_column = max(max_column, column)
if node.left:
queue.append((node.left, column - 1))
if node.right:
queue.append((node.right, column + 1))
return [column_table[i] for i in range(min_column, max_column + 1)]
9. Conclusion
Mastering tree traversal techniques is essential for excelling in coding interviews, especially when applying to major tech companies. By understanding the various traversal methods, their implementations, and practical applications, you’ll be well-equipped to tackle a wide range of tree-related problems.
Remember to practice regularly, implement both recursive and iterative solutions, and focus on understanding the underlying principles rather than just memorizing code. As you become more comfortable with these techniques, you’ll find that many complex tree problems become more approachable.
Keep in mind that tree traversal is just one aspect of working with tree data structures. To fully prepare for interviews, also study tree construction, balancing, and various types of trees like AVL trees, Red-Black trees, and B-trees.
With dedication and consistent practice, you’ll be well on your way to mastering tree traversal and improving your overall coding skills. Good luck with your interview preparation!