How to Use Hash Tables to Simplify Your Solutions
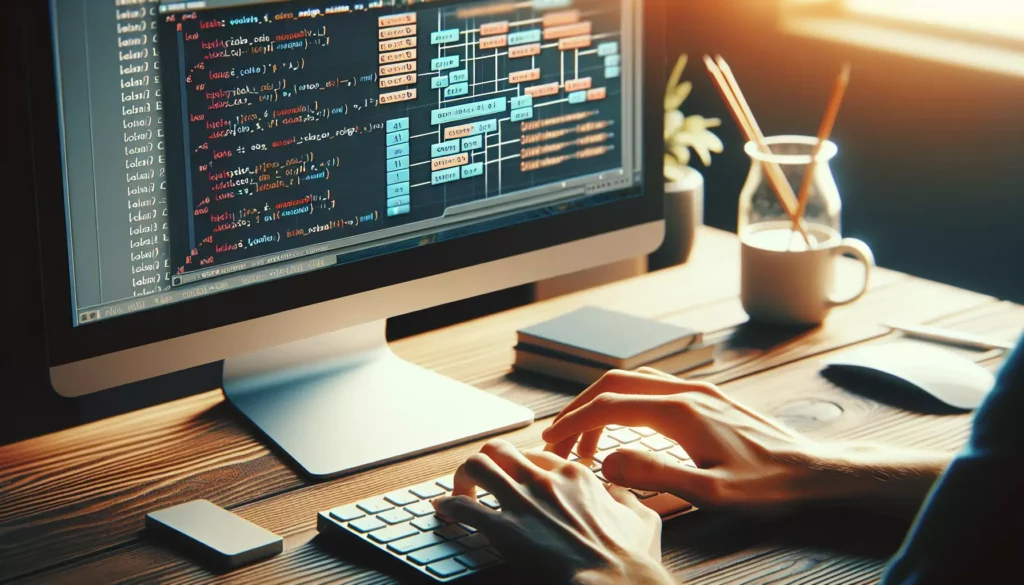
In the world of coding and algorithm design, efficiency is key. As developers, we’re constantly seeking ways to optimize our code and solve problems more effectively. One powerful tool that often comes to our rescue is the hash table. In this comprehensive guide, we’ll explore how hash tables can simplify your solutions and elevate your coding skills to the next level.
What are Hash Tables?
Before we dive into the practical applications of hash tables, let’s start with a clear understanding of what they are. A hash table, also known as a hash map, is a data structure that implements an associative array abstract data type. It’s a structure that can map keys to values, allowing for efficient lookup, insertion, and deletion operations.
The core idea behind a hash table is the use of a hash function. This function takes a key as input and returns an index (or address) where the corresponding value should be stored in the underlying array. The goal is to distribute the keys uniformly across the array to minimize collisions (when two keys hash to the same index).
Key Components of a Hash Table:
- Hash Function: Converts keys into array indices
- Array: Stores the key-value pairs
- Collision Resolution Method: Handles cases when two keys hash to the same index
Why Use Hash Tables?
Hash tables offer several advantages that make them an invaluable tool in a programmer’s arsenal:
- Fast Access: On average, hash tables provide O(1) time complexity for lookup, insertion, and deletion operations.
- Flexibility: They can store various types of data and are not limited to specific key types.
- Space Efficiency: Hash tables can be more space-efficient compared to other data structures for certain types of problems.
- Simplification: They can greatly simplify complex algorithms and make code more readable.
Implementing a Basic Hash Table
Let’s start by implementing a simple hash table in Python to understand its basic structure:
class HashTable:
def __init__(self, size=10):
self.size = size
self.table = [[] for _ in range(self.size)]
def _hash(self, key):
return hash(key) % self.size
def insert(self, key, value):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
item[1] = value
return
self.table[index].append([key, value])
def get(self, key):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
return item[1]
raise KeyError(key)
def remove(self, key):
index = self._hash(key)
for i, item in enumerate(self.table[index]):
if item[0] == key:
del self.table[index][i]
return
raise KeyError(key)
# Usage
ht = HashTable()
ht.insert("name", "John")
ht.insert("age", 30)
print(ht.get("name")) # Output: John
ht.remove("name")
# ht.get("name") # This would raise a KeyError
This implementation uses chaining for collision resolution, where each bucket in the array is a list that can hold multiple key-value pairs.
Simplifying Solutions with Hash Tables
Now that we have a basic understanding of hash tables, let’s explore how they can simplify solutions to common programming problems.
1. Two Sum Problem
The Two Sum problem is a classic example where hash tables shine. Given an array of integers and a target sum, find two numbers in the array that add up to the target.
Without a hash table, a naive solution might look like this:
def two_sum_naive(nums, target):
n = len(nums)
for i in range(n):
for j in range(i+1, n):
if nums[i] + nums[j] == target:
return [i, j]
return [] # No solution found
# Time complexity: O(n^2)
Using a hash table, we can simplify this to a single pass solution:
def two_sum_hash(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return [] # No solution found
# Time complexity: O(n)
The hash table solution reduces the time complexity from O(n^2) to O(n), dramatically improving efficiency for large inputs.
2. First Non-Repeating Character
Another problem where hash tables can simplify the solution is finding the first non-repeating character in a string.
A naive approach might involve nested loops:
def first_non_repeating_naive(s):
for i, char in enumerate(s):
if char not in s[:i] and char not in s[i+1:]:
return char
return None
# Time complexity: O(n^2)
Using a hash table, we can solve this in a single pass:
from collections import Counter
def first_non_repeating_hash(s):
char_count = Counter(s)
for char in s:
if char_count[char] == 1:
return char
return None
# Time complexity: O(n)
Again, we see a significant improvement in time complexity, from O(n^2) to O(n).
3. Grouping Anagrams
Hash tables are particularly useful when dealing with problems involving strings and character frequencies. Consider the problem of grouping anagrams from a list of words.
A solution using hash tables might look like this:
from collections import defaultdict
def group_anagrams(words):
anagram_groups = defaultdict(list)
for word in words:
# Sort the characters of the word to use as a key
sorted_word = ''.join(sorted(word))
anagram_groups[sorted_word].append(word)
return list(anagram_groups.values())
# Example usage
words = ["eat", "tea", "tan", "ate", "nat", "bat"]
result = group_anagrams(words)
print(result)
# Output: [["eat", "tea", "ate"], ["tan", "nat"], ["bat"]]
# Time complexity: O(n * k log k), where n is the number of words and k is the maximum length of a word
This solution efficiently groups anagrams without the need for comparing each word with every other word, which would result in a much higher time complexity.
Advanced Techniques with Hash Tables
As you become more comfortable with hash tables, you can start applying more advanced techniques to solve complex problems.
1. Rolling Hash for String Matching
Rolling hash is a technique used in string matching algorithms like Rabin-Karp. It allows for efficient computation of hash values for substrings, enabling quick comparisons.
class RollingHash:
def __init__(self, text, pattern_length):
self.text = text
self.pattern_length = pattern_length
self.base = 256
self.modulus = 101
self.h = pow(self.base, pattern_length - 1) % self.modulus
self.current_hash = self.calculate_initial_hash()
def calculate_initial_hash(self):
hash_value = 0
for i in range(self.pattern_length):
hash_value = (hash_value * self.base + ord(self.text[i])) % self.modulus
return hash_value
def next_hash(self, start_index):
if start_index + self.pattern_length >= len(self.text):
return None
self.current_hash = (self.current_hash - ord(self.text[start_index]) * self.h) % self.modulus
self.current_hash = (self.current_hash * self.base + ord(self.text[start_index + self.pattern_length])) % self.modulus
if self.current_hash < 0:
self.current_hash += self.modulus
return self.current_hash
# Usage in Rabin-Karp algorithm
def rabin_karp(text, pattern):
if not pattern:
return []
matches = []
pattern_hash = sum(ord(c) for c in pattern) % 101
rolling_hash = RollingHash(text, len(pattern))
for i in range(len(text) - len(pattern) + 1):
if i == 0:
current_hash = rolling_hash.current_hash
else:
current_hash = rolling_hash.next_hash(i - 1)
if current_hash == pattern_hash and text[i:i+len(pattern)] == pattern:
matches.append(i)
return matches
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABC"
print(rabin_karp(text, pattern)) # Output: [10]
This implementation of the Rabin-Karp algorithm uses a rolling hash to efficiently find pattern matches in a text string.
2. Bloom Filters
Bloom filters are a space-efficient probabilistic data structure that uses hash functions to test whether an element is a member of a set. They’re great for reducing the number of expensive disk (or network) lookups for non-existent keys.
import math
import mmh3
class BloomFilter:
def __init__(self, size, num_hashes):
self.size = size
self.num_hashes = num_hashes
self.bit_array = [0] * size
def add(self, item):
for seed in range(self.num_hashes):
index = mmh3.hash(item, seed) % self.size
self.bit_array[index] = 1
def contains(self, item):
for seed in range(self.num_hashes):
index = mmh3.hash(item, seed) % self.size
if self.bit_array[index] == 0:
return False
return True
@classmethod
def get_size(cls, n, p):
m = -(n * math.log(p)) / (math.log(2)**2)
return int(m)
@classmethod
def get_hash_count(cls, m, n):
k = (m/n) * math.log(2)
return int(k)
# Usage
n = 1000 # number of items to add
p = 0.01 # false positive probability
size = BloomFilter.get_size(n, p)
num_hashes = BloomFilter.get_hash_count(size, n)
bf = BloomFilter(size, num_hashes)
bf.add("apple")
bf.add("banana")
print(bf.contains("apple")) # True
print(bf.contains("banana")) # True
print(bf.contains("orange")) # False (probably)
Bloom filters are particularly useful in cases where you need to quickly check if an item might be in a set, and where false positives are acceptable but false negatives are not.
Common Pitfalls and How to Avoid Them
While hash tables are powerful, there are some common pitfalls to be aware of:
1. Collision Handling
Collisions occur when two different keys hash to the same index. There are two main strategies to handle collisions:
- Chaining: Each bucket contains a list of key-value pairs.
- Open Addressing: If a collision occurs, the algorithm searches for the next open slot in the array.
Choose the appropriate strategy based on your use case and expected data distribution.
2. Load Factor
The load factor is the ratio of the number of stored elements to the size of the hash table. As the load factor increases, so does the likelihood of collisions. Most hash table implementations automatically resize when the load factor exceeds a certain threshold (typically 0.75).
3. Poor Hash Functions
A poor hash function can lead to many collisions, degrading performance. Ensure your hash function distributes keys uniformly across the array.
4. Mutable Keys
Using mutable objects as keys can lead to unexpected behavior. If a key’s hash value changes after insertion, you may not be able to retrieve the associated value. Stick to immutable types for keys when possible.
Hash Tables in Different Programming Languages
Different programming languages implement hash tables with varying syntax and features. Let’s look at how hash tables (or their equivalents) are used in some popular languages:
Python (dict)
my_dict = {"apple": 1, "banana": 2}
my_dict["cherry"] = 3
print(my_dict.get("apple")) # Output: 1
JavaScript (Object or Map)
// Using Object
let obj = {apple: 1, banana: 2};
obj.cherry = 3;
console.log(obj.apple); // Output: 1
// Using Map
let map = new Map();
map.set("apple", 1);
map.set("banana", 2);
console.log(map.get("apple")); // Output: 1
Java (HashMap)
import java.util.HashMap;
HashMap<String, Integer> map = new HashMap<>();
map.put("apple", 1);
map.put("banana", 2);
System.out.println(map.get("apple")); // Output: 1
C++ (unordered_map)
#include <iostream>
#include <unordered_map>
#include <string>
int main() {
std::unordered_map<std::string, int> map;
map["apple"] = 1;
map["banana"] = 2;
std::cout << map["apple"] << std::endl; // Output: 1
return 0;
}
Real-world Applications of Hash Tables
Hash tables are not just theoretical constructs; they have numerous practical applications in real-world software systems:
1. Database Indexing
Hash tables are often used to create indexes in databases, allowing for fast retrieval of records based on key values.
2. Caching
Web browsers use hash tables to store cached objects, mapping URLs to locally stored content for quick access.
3. Symbol Tables in Compilers
Compilers and interpreters use hash tables to keep track of variable and function names and their associated information.
4. Password Storage
Secure systems store hashed passwords rather than plaintext, using hash tables to quickly verify login attempts.
5. Spell Checkers
Hash tables can be used to efficiently store and lookup words in a dictionary for spell-checking applications.
Conclusion
Hash tables are a powerful tool that can significantly simplify and optimize your coding solutions. By providing fast access times and flexible key-value associations, they enable efficient solutions to a wide range of problems.
As you continue your journey in coding and algorithm design, remember that mastering data structures like hash tables is key to becoming a proficient programmer. Practice implementing and using hash tables in various scenarios to solidify your understanding and improve your problem-solving skills.
Whether you’re preparing for technical interviews, working on personal projects, or developing large-scale applications, the ability to effectively use hash tables will serve you well. Keep exploring, keep practicing, and watch as your solutions become more elegant and efficient with the power of hash tables at your fingertips.