How to Optimize Code During an Interview: A Comprehensive Guide
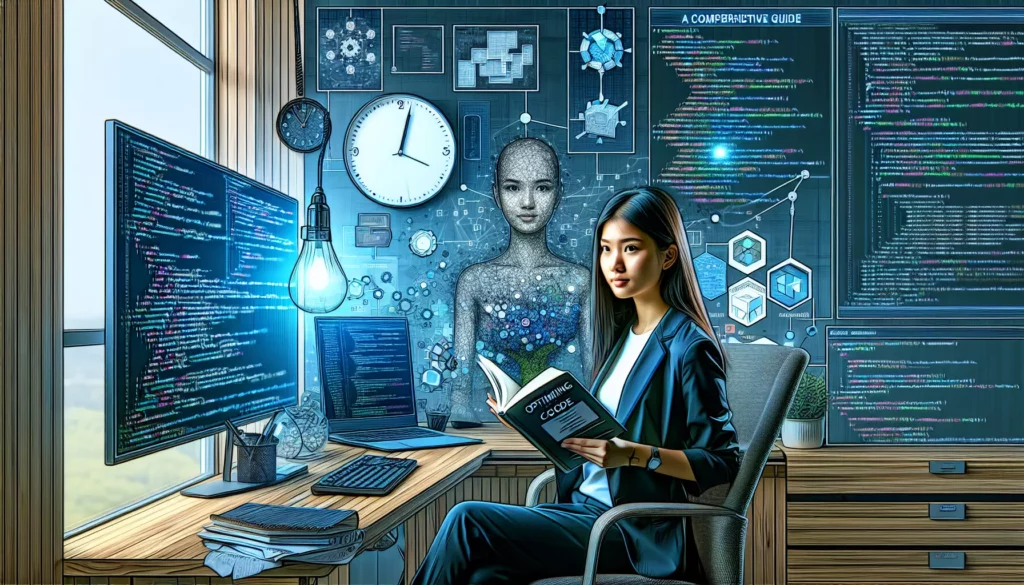
In the competitive landscape of technical interviews, particularly for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), the ability to optimize code is a crucial skill. This comprehensive guide will walk you through the process of code optimization during an interview, providing you with strategies, techniques, and best practices to showcase your problem-solving abilities and impress your interviewers.
Understanding the Importance of Code Optimization
Before diving into the specifics of how to optimize code during an interview, it’s essential to understand why this skill is so valued by employers:
- Efficiency: Optimized code runs faster and uses fewer resources, which is crucial for large-scale applications.
- Scalability: Well-optimized code can handle increased loads and growing datasets more effectively.
- Cost-effectiveness: Efficient code can lead to reduced infrastructure costs for companies.
- Problem-solving skills: The ability to optimize code demonstrates your capacity for analytical thinking and creative problem-solving.
- Industry standards: Many tech companies, especially FAANG, place a high value on code optimization skills.
Preparing for Code Optimization Questions
To excel in code optimization during an interview, you need to be well-prepared. Here are some key areas to focus on:
1. Master Data Structures and Algorithms
A solid understanding of various data structures and algorithms is fundamental to code optimization. Familiarize yourself with:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching Algorithms
- Dynamic Programming
- Greedy Algorithms
2. Understand Time and Space Complexity
Being able to analyze and discuss the time and space complexity of your solutions is crucial. Make sure you:
- Understand Big O notation
- Can calculate the time complexity of different algorithms
- Are familiar with space-time tradeoffs
3. Practice Problem-Solving
Regular practice is key to improving your code optimization skills. Use platforms like AlgoCademy, LeetCode, or HackerRank to:
- Solve a variety of coding problems
- Practice optimizing your initial solutions
- Learn from others’ solutions and explanations
Strategies for Code Optimization During an Interview
When faced with a coding problem during an interview, follow these strategies to effectively optimize your code:
1. Start with a Brute Force Solution
Begin by implementing a straightforward solution to the problem, even if it’s not the most efficient. This approach has several benefits:
- It demonstrates that you can solve the problem
- It provides a starting point for optimization
- It allows you to discuss tradeoffs between different approaches
2. Analyze and Identify Bottlenecks
Once you have a working solution, analyze it to identify areas for improvement:
- Look for redundant calculations or operations
- Identify any unnecessary data structures or variables
- Consider if there are more efficient algorithms or data structures you could use
3. Apply Optimization Techniques
Based on your analysis, apply appropriate optimization techniques. Some common approaches include:
- Memoization: Store results of expensive function calls and return the cached result when the same inputs occur again.
- Dynamic Programming: Break down a complex problem into simpler subproblems and store the results for future use.
- Two Pointers: Use two pointers to traverse data structures, often reducing time complexity from O(n^2) to O(n).
- Sliding Window: Maintain a subset of items as a window to process data streams efficiently.
- Binary Search: For sorted arrays, use binary search to reduce time complexity from O(n) to O(log n).
- Hash Tables: Use hash tables for quick lookups, often reducing time complexity from O(n) to O(1).
4. Optimize Space Usage
While time complexity is often the primary focus, don’t neglect space optimization:
- Consider in-place algorithms to reduce space complexity
- Use appropriate data structures to minimize memory usage
- Be mindful of creating unnecessary copies of data
5. Communicate Your Thought Process
Throughout the optimization process, clearly communicate your thoughts to the interviewer:
- Explain why you’re making specific optimizations
- Discuss the tradeoffs between different approaches
- Demonstrate your ability to think critically about performance
Common Code Optimization Techniques
Let’s explore some common code optimization techniques with examples:
1. Memoization
Memoization is particularly useful for recursive functions with overlapping subproblems. Here’s an example of optimizing a recursive Fibonacci function:
// Unoptimized recursive Fibonacci
function fib(n) {
if (n <= 1) return n;
return fib(n - 1) + fib(n - 2);
}
// Optimized Fibonacci with memoization
function fibOptimized(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibOptimized(n - 1, memo) + fibOptimized(n - 2, memo);
return memo[n];
}
The optimized version uses a memo object to store previously calculated values, significantly reducing the number of recursive calls.
2. Two Pointers Technique
The two pointers technique can be used to solve problems efficiently, often reducing time complexity from O(n^2) to O(n). Here’s an example of finding a pair of numbers in a sorted array that sum to a target:
// Unoptimized approach (O(n^2))
function findPairSum(arr, target) {
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] + arr[j] === target) {
return [arr[i], arr[j]];
}
}
}
return null;
}
// Optimized approach with two pointers (O(n))
function findPairSumOptimized(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left < right) {
let sum = arr[left] + arr[right];
if (sum === target) {
return [arr[left], arr[right]];
} else if (sum < target) {
left++;
} else {
right--;
}
}
return null;
}
The optimized version uses two pointers moving towards each other, reducing the time complexity to O(n).
3. Sliding Window Technique
The sliding window technique is useful for problems involving subarrays or substrings. Here’s an example of finding the maximum sum subarray of size k:
// Unoptimized approach (O(n*k))
function maxSubarraySum(arr, k) {
let maxSum = -Infinity;
for (let i = 0; i <= arr.length - k; i++) {
let sum = 0;
for (let j = 0; j < k; j++) {
sum += arr[i + j];
}
maxSum = Math.max(maxSum, sum);
}
return maxSum;
}
// Optimized approach with sliding window (O(n))
function maxSubarraySumOptimized(arr, k) {
if (k > arr.length) return null;
let maxSum = 0;
let windowSum = 0;
// Compute sum of first window
for (let i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
// Slide the window and update max sum
for (let i = k; i < arr.length; i++) {
windowSum = windowSum - arr[i - k] + arr[i];
maxSum = Math.max(maxSum, windowSum);
}
return maxSum;
}
The optimized version uses a sliding window to maintain the sum, reducing time complexity to O(n).
Advanced Optimization Techniques
As you progress in your coding skills, you may encounter more complex optimization scenarios. Here are some advanced techniques to consider:
1. Bit Manipulation
Bit manipulation can lead to significant optimizations in certain scenarios. For example, you can use bitwise operations to efficiently perform operations like checking if a number is even/odd, multiplying/dividing by 2, or setting/unsetting specific bits.
// Check if a number is even
function isEven(n) {
return (n & 1) === 0;
}
// Multiply by 2
function multiplyBy2(n) {
return n << 1;
}
// Divide by 2
function divideBy2(n) {
return n >> 1;
}
2. Precomputation and Caching
For problems involving repeated calculations or lookups, precomputing values and caching results can lead to significant performance improvements. This is particularly useful in scenarios where you have a fixed set of inputs or a limited range of possible values.
// Precompute factorials
const factorials = [1];
for (let i = 1; i <= 20; i++) {
factorials[i] = factorials[i-1] * i;
}
function factorial(n) {
if (n < 0 || n > 20) throw new Error("Input out of range");
return factorials[n];
}
3. Lazy Evaluation
Lazy evaluation involves delaying the evaluation of an expression until its value is needed. This can be particularly useful when dealing with large datasets or expensive computations.
function* lazyRange(start, end) {
for (let i = start; i <= end; i++) {
yield i;
}
}
// Usage
const range = lazyRange(1, 1000000);
for (const num of range) {
if (num % 10000 === 0) {
console.log(num);
if (num === 50000) break;
}
}
In this example, the lazyRange generator only computes values as they’re needed, rather than generating the entire range upfront.
Optimization in Different Programming Paradigms
Different programming paradigms may require different optimization approaches. Here’s a brief overview:
1. Functional Programming
In functional programming, optimization often involves:
- Using pure functions for easier memoization
- Leveraging lazy evaluation
- Utilizing tail recursion optimization
2. Object-Oriented Programming
In object-oriented programming, consider:
- Efficient use of inheritance and polymorphism
- Proper encapsulation to prevent unnecessary computations
- Using design patterns for optimized structures
3. Concurrent and Parallel Programming
When dealing with concurrent or parallel code:
- Optimize for thread safety without excessive locking
- Use appropriate synchronization mechanisms
- Consider load balancing in distributed systems
Balancing Readability and Optimization
While optimization is important, it’s crucial to maintain code readability and maintainability. Here are some tips:
- Comment your code, especially for complex optimizations
- Use meaningful variable and function names
- Break down complex optimizations into smaller, understandable steps
- Consider creating utility functions for common optimization patterns
Common Pitfalls in Code Optimization
Be aware of these common mistakes when optimizing code:
- Premature optimization: Don’t optimize before you have a working solution and have identified actual bottlenecks.
- Over-optimization: Sometimes, the performance gain doesn’t justify the increased complexity.
- Ignoring readability: Extremely optimized code can become difficult to understand and maintain.
- Not considering edge cases: Ensure your optimizations work for all possible inputs.
- Focusing only on time complexity: Remember to consider space complexity and other factors like readability and maintainability.
Tools for Code Optimization
Familiarize yourself with tools that can assist in code optimization:
- Profilers: Tools like Chrome DevTools (for JavaScript) or cProfile (for Python) can help identify performance bottlenecks.
- Static analyzers: Tools like ESLint (for JavaScript) or Pylint (for Python) can help identify potential optimization opportunities.
- Benchmarking tools: Use benchmarking libraries to compare the performance of different implementations.
Conclusion
Mastering code optimization is a valuable skill that can set you apart in technical interviews, especially for positions at major tech companies. By understanding fundamental concepts, practicing various optimization techniques, and effectively communicating your thought process, you can demonstrate your problem-solving abilities and technical prowess.
Remember, the goal in an interview is not just to produce optimized code, but to show your ability to think critically about performance, make informed decisions, and balance various factors such as time complexity, space complexity, and code readability.
Continue to practice and refine your optimization skills using platforms like AlgoCademy, and you’ll be well-prepared to tackle any coding challenge that comes your way in your next technical interview.