Utilizing Data Structures Effectively in Solutions: A Comprehensive Guide
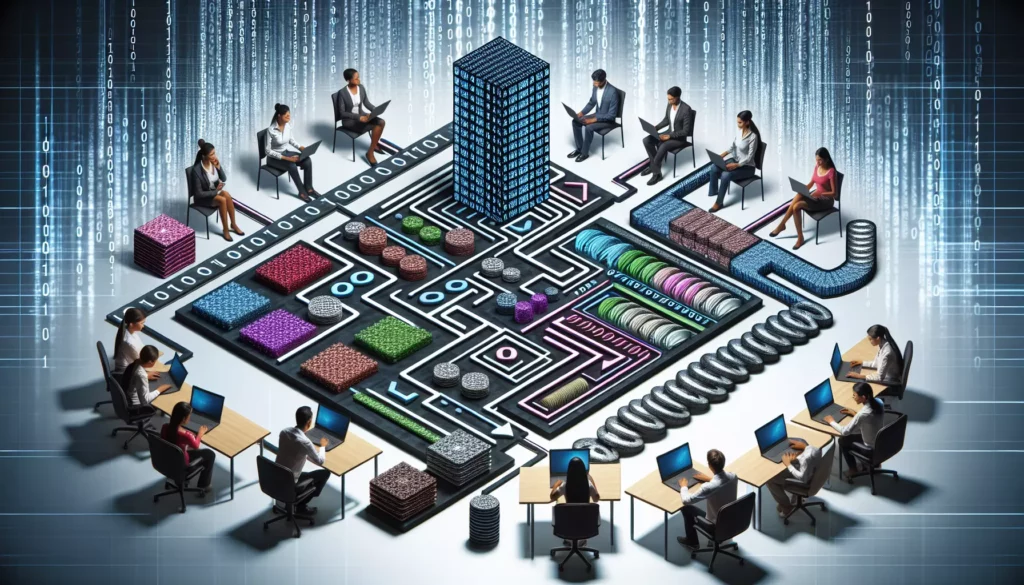
In the world of programming and algorithm design, data structures play a crucial role in creating efficient and scalable solutions. Whether you’re a beginner learning to code or an experienced developer preparing for technical interviews at top tech companies, understanding how to utilize data structures effectively is essential. This comprehensive guide will explore various data structures, their applications, and how to leverage them to solve complex problems.
1. Introduction to Data Structures
Data structures are specialized formats for organizing, processing, retrieving, and storing data. They provide a way to manage large amounts of information efficiently, allowing for faster processing and better memory utilization. Some common data structures include:
- Arrays
- Linked Lists
- Stacks
- Queues
- Trees
- Graphs
- Hash Tables
Each data structure has its own strengths and weaknesses, making them suitable for different types of problems and scenarios. Choosing the right data structure can significantly impact the performance and efficiency of your solution.
2. Arrays: The Foundation of Data Structures
Arrays are one of the most fundamental data structures in programming. They provide a contiguous block of memory to store elements of the same data type. Arrays offer constant-time access to elements using their indices, making them ideal for situations where you need quick random access to data.
When to Use Arrays:
- When you need constant-time access to elements
- When the size of the data is known in advance
- For implementing other data structures like stacks, queues, and hash tables
Example: Using Arrays for Two-Sum Problem
Let’s consider the classic “Two Sum” problem, where we need to find two numbers in an array that add up to a target sum.
public static int[] twoSum(int[] nums, int target) {
Map<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (map.containsKey(complement)) {
return new int[] { map.get(complement), i };
}
map.put(nums[i], i);
}
throw new IllegalArgumentException("No two sum solution");
}
In this solution, we use an array to store the input numbers and a hash map to keep track of complements, allowing for an O(n) time complexity solution.
3. Linked Lists: Dynamic Data Storage
Linked lists are a dynamic data structure consisting of nodes, where each node contains data and a reference (or link) to the next node in the sequence. Unlike arrays, linked lists can grow or shrink in size during runtime, making them flexible for scenarios where the data size is unknown or frequently changing.
When to Use Linked Lists:
- When frequent insertions and deletions are required
- When the size of the data is unknown or dynamic
- For implementing other data structures like stacks, queues, and hash tables
Example: Reversing a Linked List
Reversing a linked list is a common interview question that demonstrates the power of linked lists.
public ListNode reverseList(ListNode head) {
ListNode prev = null;
ListNode current = head;
while (current != null) {
ListNode nextTemp = current.next;
current.next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
This solution efficiently reverses a linked list in-place, showcasing the flexibility of linked list operations.
4. Stacks: Last-In-First-Out (LIFO) Structure
Stacks are a linear data structure that follows the Last-In-First-Out (LIFO) principle. Elements are added to the top of the stack and removed from the top as well. Stacks are particularly useful in scenarios where you need to keep track of state or perform operations in reverse order.
When to Use Stacks:
- For function call management (call stack)
- Undo mechanisms in applications
- Expression evaluation and syntax parsing
Example: Validating Parentheses
A common use case for stacks is validating balanced parentheses in a string.
public boolean isValid(String s) {
Stack<Character> stack = new Stack<>();
for (char c : s.toCharArray()) {
if (c == '(' || c == '[' || c == '{') {
stack.push(c);
} else {
if (stack.isEmpty()) return false;
char top = stack.pop();
if ((c == ')' && top != '(') ||
(c == ']' && top != '[') ||
(c == '}' && top != '{')) {
return false;
}
}
}
return stack.isEmpty();
}
This solution uses a stack to keep track of opening brackets and ensures that closing brackets match the most recent opening bracket.
5. Queues: First-In-First-Out (FIFO) Structure
Queues are another linear data structure that follows the First-In-First-Out (FIFO) principle. Elements are added to the rear of the queue and removed from the front. Queues are ideal for scenarios where you need to process items in the order they were received.
When to Use Queues:
- Task scheduling in operating systems
- Breadth-First Search (BFS) in graphs
- Print job spooling
Example: Level Order Traversal of a Binary Tree
Queues are commonly used in level order traversal of trees, also known as breadth-first traversal.
public List<List<Integer>> levelOrder(TreeNode root) {
List<List<Integer>> result = new ArrayList<>();
if (root == null) return result;
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(root);
while (!queue.isEmpty()) {
int levelSize = queue.size();
List<Integer> currentLevel = new ArrayList<>();
for (int i = 0; i < levelSize; i++) {
TreeNode node = queue.poll();
currentLevel.add(node.val);
if (node.left != null) queue.offer(node.left);
if (node.right != null) queue.offer(node.right);
}
result.add(currentLevel);
}
return result;
}
This solution uses a queue to process nodes level by level, resulting in a breadth-first traversal of the tree.
6. Trees: Hierarchical Data Structure
Trees are non-linear data structures that represent hierarchical relationships between elements. They consist of nodes connected by edges, with a single root node at the top. Trees are widely used in various applications, including file systems, organization charts, and search algorithms.
When to Use Trees:
- Representing hierarchical data
- Efficient searching and sorting (e.g., Binary Search Trees)
- Expression parsing and evaluation
Example: Implementing a Binary Search Tree (BST)
Binary Search Trees are a special type of binary tree that maintain a sorted order, making them efficient for searching, insertion, and deletion operations.
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int x) { val = x; }
}
class BinarySearchTree {
private TreeNode root;
public void insert(int value) {
root = insertRec(root, value);
}
private TreeNode insertRec(TreeNode root, int value) {
if (root == null) {
root = new TreeNode(value);
return root;
}
if (value < root.val)
root.left = insertRec(root.left, value);
else if (value > root.val)
root.right = insertRec(root.right, value);
return root;
}
public boolean search(int value) {
return searchRec(root, value);
}
private boolean searchRec(TreeNode root, int value) {
if (root == null || root.val == value)
return root != null;
if (value < root.val)
return searchRec(root.left, value);
return searchRec(root.right, value);
}
}
This implementation demonstrates the basic structure and operations of a Binary Search Tree, showcasing how trees can be used for efficient data management and retrieval.
7. Graphs: Representing Complex Relationships
Graphs are versatile data structures used to represent complex relationships between entities. They consist of vertices (or nodes) connected by edges. Graphs can be directed or undirected and can have weighted or unweighted edges. They are extensively used in various applications, including social networks, transportation systems, and recommendation engines.
When to Use Graphs:
- Modeling relationships in social networks
- Representing road networks for navigation
- Dependency resolution in software packages
Example: Depth-First Search (DFS) in a Graph
Depth-First Search is a fundamental graph traversal algorithm used to explore nodes in a graph.
import java.util.*;
class Graph {
private int V;
private LinkedList<Integer>[] adj;
Graph(int v) {
V = v;
adj = new LinkedList[v];
for (int i = 0; i < v; ++i)
adj[i] = new LinkedList();
}
void addEdge(int v, int w) {
adj[v].add(w);
}
void DFSUtil(int v, boolean visited[]) {
visited[v] = true;
System.out.print(v + " ");
Iterator<Integer> i = adj[v].listIterator();
while (i.hasNext()) {
int n = i.next();
if (!visited[n])
DFSUtil(n, visited);
}
}
void DFS(int v) {
boolean visited[] = new boolean[V];
DFSUtil(v, visited);
}
}
// Usage
Graph g = new Graph(4);
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 2);
g.addEdge(2, 0);
g.addEdge(2, 3);
g.addEdge(3, 3);
System.out.println("Depth First Traversal starting from vertex 2:");
g.DFS(2);
This implementation demonstrates how graphs can be represented using adjacency lists and how depth-first search can be performed to traverse the graph.
8. Hash Tables: Fast Data Retrieval
Hash tables, also known as hash maps, are data structures that provide fast insertion, deletion, and lookup operations. They use a hash function to compute an index into an array of buckets or slots, from which the desired value can be found. Hash tables are widely used for implementing associative arrays, database indexing, and caching.
When to Use Hash Tables:
- Fast data retrieval based on keys
- Implementing caches
- Detecting duplicates in a large dataset
Example: Implementing a Simple Hash Table
Let’s implement a basic hash table with string keys and integer values:
class HashNode<K, V> {
K key;
V value;
HashNode<K, V> next;
public HashNode(K key, V value) {
this.key = key;
this.value = value;
}
}
class MyHashMap<K, V> {
private ArrayList<HashNode<K, V>> bucketArray;
private int numBuckets;
private int size;
public MyHashMap() {
bucketArray = new ArrayList<>();
numBuckets = 10;
size = 0;
for (int i = 0; i < numBuckets; i++)
bucketArray.add(null);
}
private int getBucketIndex(K key) {
int hashCode = key.hashCode();
return Math.abs(hashCode % numBuckets);
}
public void put(K key, V value) {
int bucketIndex = getBucketIndex(key);
HashNode<K, V> head = bucketArray.get(bucketIndex);
while (head != null) {
if (head.key.equals(key)) {
head.value = value;
return;
}
head = head.next;
}
size++;
head = bucketArray.get(bucketIndex);
HashNode<K, V> newNode = new HashNode<K, V>(key, value);
newNode.next = head;
bucketArray.set(bucketIndex, newNode);
}
public V get(K key) {
int bucketIndex = getBucketIndex(key);
HashNode<K, V> head = bucketArray.get(bucketIndex);
while (head != null) {
if (head.key.equals(key))
return head.value;
head = head.next;
}
return null;
}
}
// Usage
MyHashMap<String, Integer> map = new MyHashMap<>();
map.put("John", 25);
map.put("Jane", 30);
System.out.println(map.get("John")); // Output: 25
System.out.println(map.get("Jane")); // Output: 30
This implementation demonstrates a basic hash table with separate chaining for collision resolution. It provides constant-time average case complexity for basic operations like insertion and retrieval.
9. Choosing the Right Data Structure
Selecting the appropriate data structure for a given problem is crucial for developing efficient solutions. Here are some guidelines to help you choose:
- Arrays: Use when you need fast random access and know the size in advance.
- Linked Lists: Ideal for frequent insertions and deletions, especially at the beginning or end.
- Stacks: Perfect for managing function calls, undo operations, or any last-in-first-out scenarios.
- Queues: Use for first-in-first-out processing, like breadth-first search or task scheduling.
- Trees: Great for hierarchical data and when you need efficient searching and sorting.
- Graphs: Use when representing complex relationships between entities.
- Hash Tables: Ideal for fast key-based data retrieval and detecting duplicates.
Consider the following factors when choosing a data structure:
- The type of operations you’ll be performing (insertion, deletion, search, etc.)
- The frequency of these operations
- The amount of data you’re working with
- Memory constraints of your system
- The nature of the data (sorted, unsorted, hierarchical, etc.)
10. Optimizing Data Structure Usage
To make the most of data structures in your solutions, consider these optimization techniques:
- Use built-in data structures: Most programming languages provide optimized implementations of common data structures. Use these when possible instead of reinventing the wheel.
- Understand time and space complexity: Know the big O notation for various operations on different data structures to make informed decisions.
- Combine data structures: Sometimes, using a combination of data structures can lead to more efficient solutions. For example, using a hash table with a linked list for LRU cache implementation.
- Preprocess data: If you’re working with static data, consider preprocessing it into a more efficient structure for your specific use case.
- Use lazy initialization: Only create data structures when they’re needed to save memory and initialization time.
- Consider concurrent data structures: If your application is multi-threaded, use thread-safe data structures or implement proper synchronization.
11. Practice and Application
To truly master the art of utilizing data structures effectively, practice is key. Here are some ways to improve your skills:
- Solve coding challenges: Platforms like LeetCode, HackerRank, and CodeSignal offer a wide range of problems that require efficient use of data structures.
- Implement data structures from scratch: This helps deepen your understanding of how they work under the hood.
- Analyze existing code: Study open-source projects to see how experienced developers use data structures in real-world applications.
- Participate in coding competitions: These often require quick thinking and efficient use of data structures under time pressure.
- Work on personal projects: Apply your knowledge to build applications that solve real problems, forcing you to make practical decisions about data structure usage.
Conclusion
Mastering the art of utilizing data structures effectively is a crucial skill for any programmer, especially those aiming for positions at top tech companies. By understanding the strengths and weaknesses of various data structures and practicing their application in diverse scenarios, you’ll be well-equipped to tackle complex problems and design efficient solutions.
Remember, the key to becoming proficient with data structures is not just knowing their implementations but understanding when and how to apply them in real-world situations. As you continue to practice and refine your skills, you’ll develop an intuition for choosing the right data structure for each unique problem you encounter.
Keep exploring, practicing, and challenging yourself with new problems and scenarios. With time and dedication, you’ll find that effective utilization of data structures becomes second nature, allowing you to create elegant and efficient solutions to even the most complex programming challenges.