Handling Ambiguous Questions in Coding Interviews
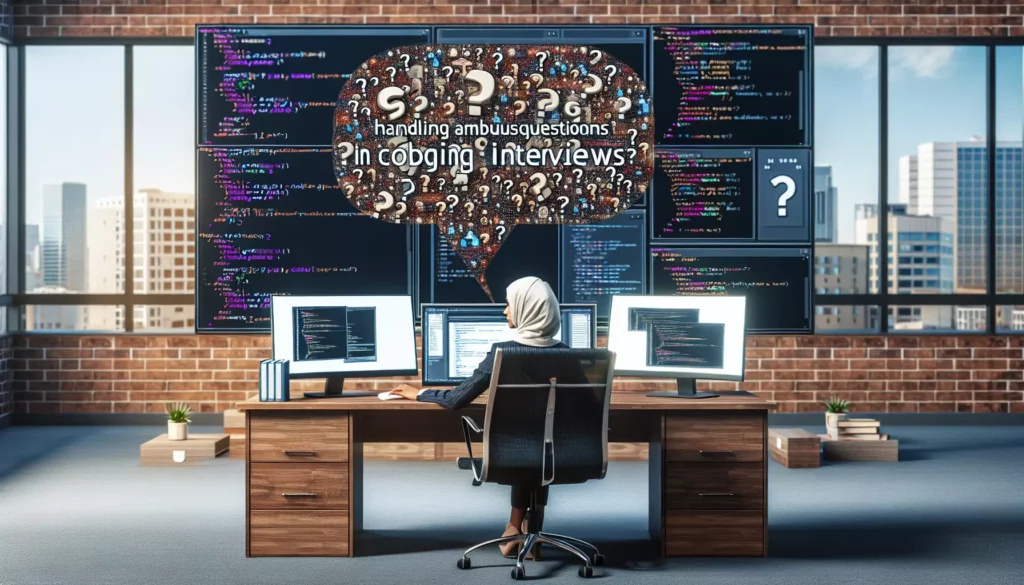
Coding interviews can be challenging, especially when you’re faced with ambiguous questions. These types of questions are often intentionally vague to test your problem-solving skills and ability to communicate effectively. In this comprehensive guide, we’ll explore strategies for handling ambiguous questions in coding interviews, providing you with the tools you need to succeed in even the most challenging interview scenarios.
Understanding Ambiguous Questions
Ambiguous questions in coding interviews are those that lack clear specifications or have multiple possible interpretations. They’re designed to mimic real-world scenarios where requirements may not always be well-defined. Interviewers use these questions to assess your:
- Ability to gather requirements
- Problem-solving skills
- Communication skills
- Adaptability
- Critical thinking
By learning how to handle ambiguous questions effectively, you’ll not only improve your performance in interviews but also develop skills that are valuable in real-world software development.
Strategies for Handling Ambiguous Questions
1. Don’t Panic
The first and most important step is to remain calm. Remember that ambiguity is often intentional, and the interviewer is more interested in your approach than in a perfect solution. Take a deep breath and approach the question methodically.
2. Clarify the Question
Before diving into a solution, make sure you understand the problem. Ask clarifying questions to gather more information and reduce ambiguity. Some examples of good clarifying questions include:
- “Can you provide an example input and expected output?”
- “What are the constraints on the input?”
- “Are there any performance requirements for the solution?”
- “Should I consider edge cases like null inputs or empty arrays?”
Don’t be afraid to ask questions – it shows that you’re thoughtful and thorough in your approach.
3. State Your Assumptions
After gathering information, clearly state any assumptions you’re making about the problem. This demonstrates your analytical skills and ensures that you and the interviewer are on the same page. For example:
“Based on our discussion, I’m assuming that:
- The input will always be a valid array of integers
- We need to optimize for time complexity rather than space complexity
- The solution should handle arrays of up to 10^6 elements”
4. Break Down the Problem
Once you have a clearer understanding of the problem, break it down into smaller, manageable components. This approach helps you tackle complex problems more effectively and demonstrates your ability to handle large-scale projects.
5. Think Aloud
As you work through the problem, verbalize your thought process. This gives the interviewer insight into your problem-solving approach and allows them to provide guidance if needed. It also demonstrates your ability to communicate technical concepts clearly.
6. Start with a Simple Solution
Begin with a basic, working solution before optimizing. This approach ensures that you have a functional solution and allows you to discuss potential improvements with the interviewer. For example:
def simple_solution(arr):
# Simple brute-force approach
result = []
for i in range(len(arr)):
for j in range(i+1, len(arr)):
if arr[i] + arr[j] == 0:
result.append([arr[i], arr[j]])
return result
7. Discuss Trade-offs
As you develop your solution, discuss the trade-offs between different approaches. This shows your ability to consider multiple perspectives and make informed decisions. For example:
“We could use a hash table to improve time complexity, but it would increase space complexity. Given the constraint of handling large arrays, I think the trade-off is worthwhile in this case.”
8. Be Adaptable
Be prepared to adjust your approach based on feedback from the interviewer. They may introduce new constraints or requirements as you progress, simulating real-world scenarios where requirements can change.
Common Types of Ambiguous Questions
While ambiguous questions can take many forms, some common types include:
1. Open-ended System Design Questions
Example: “Design a URL shortening service like bit.ly”
These questions are intentionally broad to test your ability to gather requirements, make design decisions, and consider scalability issues. When faced with such questions:
- Start by clarifying the scope and requirements
- Break down the system into components (e.g., frontend, backend, database)
- Discuss trade-offs between different architectural choices
- Consider scalability, reliability, and performance aspects
2. Algorithmic Questions with Unclear Constraints
Example: “Find the kth largest element in an unsorted array”
These questions often have multiple valid solutions depending on the constraints. To handle them effectively:
- Clarify the size of the array and the range of k
- Ask about the frequency of operations (one-time vs. repeated queries)
- Discuss time and space complexity trade-offs for different approaches
3. Real-world Scenario Questions
Example: “How would you implement a spell-checker?”
These questions test your ability to apply technical knowledge to practical problems. Approach them by:
- Clarifying the scope (e.g., supported languages, real-time vs. batch processing)
- Discussing different algorithms and data structures (e.g., Trie, Levenshtein distance)
- Considering practical aspects like dictionary updates and handling proper nouns
Example: Handling an Ambiguous Question
Let’s walk through an example of how to handle an ambiguous question in a coding interview:
Interviewer: “Implement a function to find all pairs of integers in an array that sum to zero.”
Candidate: “Thank you for the question. Before I start, I’d like to clarify a few things. Can you provide an example input and expected output?”
Interviewer: “Sure. For the input array [-1, 0, 2, -2, 1], the function should return pairs like [-1, 1] and [2, -2].”
Candidate: “I see. A few more questions:
- Should the function return all pairs or just unique pairs?
- Can the array contain duplicate elements?
- What’s the expected size of the input array?
- Are there any constraints on the integer values in the array?”
Interviewer: “Good questions. Let’s say we want unique pairs, the array can contain duplicates, the array size can be up to 10^5 elements, and the integers are within the range of -10^9 to 10^9.”
Candidate: “Thank you for the clarification. Based on this information, I’ll make the following assumptions:
- We need to return unique pairs of integers that sum to zero
- The input is a valid array of integers
- We should optimize for both time and space complexity due to the large input size
I’ll start with a simple solution and then optimize it. Here’s a basic approach using nested loops:”
def find_zero_sum_pairs(arr):
result = set()
for i in range(len(arr)):
for j in range(i+1, len(arr)):
if arr[i] + arr[j] == 0:
result.add(tuple(sorted([arr[i], arr[j]])))
return list(result)
“This solution has a time complexity of O(n^2) and space complexity of O(n) in the worst case. However, for large arrays, this might not be efficient enough. We can optimize it using a hash set:”
def find_zero_sum_pairs_optimized(arr):
seen = set()
result = set()
for num in arr:
if -num in seen:
result.add(tuple(sorted([num, -num])))
seen.add(num)
return list(result)
“This optimized solution has a time complexity of O(n) and space complexity of O(n). It trades some space for improved time complexity, which is a good trade-off given the large input size.”
Interviewer: “Good approach. Can you think of any edge cases we should consider?”
Candidate: “Certainly. We should consider the following edge cases:
- Empty array: We should return an empty list
- Array with all zeros: We should handle pairs of zeros correctly
- Array with no valid pairs: We should return an empty list
Let me update the function to handle these cases:”
def find_zero_sum_pairs_final(arr):
if not arr:
return []
seen = set()
result = set()
for num in arr:
if -num in seen:
result.add(tuple(sorted([num, -num])))
seen.add(num)
# Handle the case of multiple zeros
if arr.count(0) > 1:
result.add((0, 0))
return list(result)
“This final version handles the edge cases we discussed while maintaining the O(n) time and space complexity.”
Conclusion
Handling ambiguous questions in coding interviews is a valuable skill that can set you apart from other candidates. By following the strategies outlined in this guide – clarifying the question, stating assumptions, breaking down the problem, and communicating your thought process – you’ll be well-equipped to tackle even the most challenging interview questions.
Remember that the goal of these questions is not just to test your coding skills, but also to evaluate your problem-solving approach, communication abilities, and how you handle uncertainty. Embrace the ambiguity as an opportunity to showcase your skills and thought process.
As you prepare for coding interviews, practice with a variety of question types and focus on verbalizing your thought process. With time and practice, you’ll become more comfortable with ambiguous questions and better prepared for the challenges of real-world software development.
Additional Resources
To further improve your skills in handling ambiguous coding questions, consider exploring the following resources:
- AlgoCademy’s interactive coding tutorials and problem sets
- LeetCode’s problem-solving platform, which offers a wide range of algorithmic challenges
- “Cracking the Coding Interview” by Gayle Laakmann McDowell, which provides valuable insights into the interview process
- System design resources like “Designing Data-Intensive Applications” by Martin Kleppmann for tackling open-ended system design questions
- Mock interview platforms that allow you to practice with real interviewers and receive feedback
By combining the strategies discussed in this guide with consistent practice and a willingness to learn from each experience, you’ll be well-prepared to handle ambiguous questions and excel in your coding interviews. Remember, the key is not just to solve the problem, but to demonstrate your problem-solving process and communication skills along the way. Good luck with your interviews!